Planning Our First TypeScript Application: FinanceMe
Building FinanceMe#
You have been hired to build FinanceMe, a program that helps users manage their finances. Users of FinanceMe are able to create budgets, manage expenses, and view trends in their spending.
FinanceMe can be used via the commandline (we'll use a nifty tool, ts-node, to run our program). We'll build it in the form of a library that can be imported from other TypeScript and JavaScript projects.
By the end of the chapter, you'll be familiar with the headline features of TypeScript. Hopefully this chapter will spark your curiosity to learn more about the topics discussed, which will be covered in-depth throughout the rest of the book (along with so much more!).
For starters, we will make sure your development environment is set up correctly. Then we will devise a high-level plan for FinanceMe before we begin coding.
Planning FinanceMe#
Before we start coding, we should devise a high-level plan for FinanceMe. We'll refer to this plan as we build the various components of our application.
The first thing we should consider is how our program will be used from the user's perspective. Our client only needs FinanceMe to run on the command-line via ts-node, so we don't have to worry about configuring any compiler output settings.
A FinanceMe user will be able to do the following:
Create a user
Create budgets
Manage expenses
To fulfill these requirements, we decide to take an object-oriented approach and determine that our program will consist of four classes: User
, TrackedMonth
, Budget
, and Expense
.
Example Usage#
Below is a long block of code that shows how the finished product will work. We are about to dive into explaining it with diagrams and digging into the code. So go ahead and skim this code block to get an idea of where we're going, but if it seems a bit overwhelming, know that we will cover each step in detail!
We envision the finished product match the usage below:
import TrackedMonth from './FinanceMe/entities/TrackedMonth';
The code above gives us a preview of a typical usage of FinanceMe. The flow can be described in 4 steps:
Create expenses
Create budgets that contain expenses
Create "month" objects that contain budgets for that month
Create a user that contains a collection of "month" objects
As we build our program, we'll incrementally cover more use cases. For example, users should be able to call a method that lets them know if they are overbudget for a given year & month.
Class Diagram of FinanceMe#
For a visual depiction of our program, we've created a simple diagram that shows the relationship between the four classes:
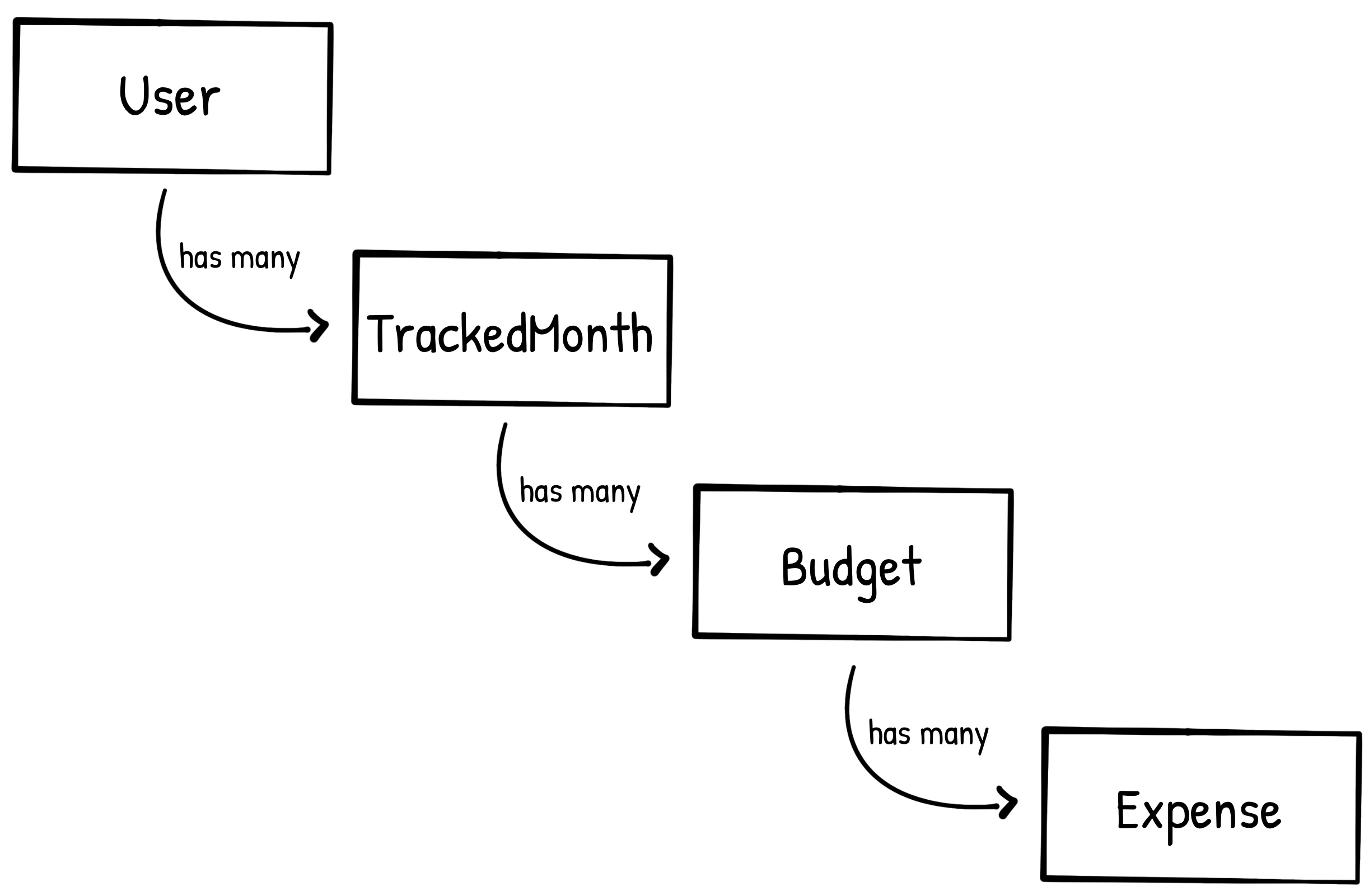
Using the diagram above we can deduce the dependency flow of our program: the User
class depends on TrackedMonth
, which depends on Budget
, which depends on Expense
. To satisfy this order, we will build FinanceMe from the bottom up, starting with Expenese
and ending with User
.
Not into object-oriented programming? You're not alone! We have a full chapter dedicated to functional programming with TypeScript.
The folder structure for our project will contain four top-level folders: entities
, enums
, types
, and utils
:
entities/
The entities
folder will hold our classes. The enums
folder will hold our enums (more on that later). The types
folder will hold global types. And the utils
folder will hold global utility functions that will be imported and used by the various entities of our program.
By the end of the next few sections, you will be acquainted with the following topics:
Annotating types for variables and functions
Exporting and importing reusable types
Using Classes
Using Enums
Using Union Types
Using Type Aliases
And most importantly, this chapter hopes to sell you on the tangible benefits of TypeScript. We quoted impressive statistics in the previous section, but knowing these numbers is half the battle--it's just as important to experience the benefits firsthand by building a real project.
Before we start coding, let's review the syntax for creating and using classes, the building blocks of our application.