Storing Colors in a Pixel Grid
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
Lesson Transcript
[00:00 - 00:08] To create our page, we're going to be storing pixels in an Ethereum smart contract. To store colors, we have to pick a format to encode them in.
[00:09 - 00:17] Every byte we store on the Ethereum blockchain costs either, so it's important that we're careful about what format we use to store our pixels. Thankfully, we don't need to invent our own format.
[00:18 - 00:27] We're going to use 24-bit RGB color. What that means is that we're going to store one byte each for the red, green, and blue component of each pixel.
[00:28 - 00:36] For example, when we use the color picker in Chrome, we can specify colors in RGBA. RGBA stands for Red/Green/Blue/Alpha.
[00:37 - 00:46] Each one of these is called a channel, as in the red channel, or the alpha channel. The alpha specifies the transparency of the color, which we're not going to use , but you could if you wanted to.
[00:47 - 00:56] Notice that as we pick a different color, the values for red, green, and blue vary as a number between 0 and 255. That's because each channel is represented by one byte.
[00:57 - 01:04] I can understand if you haven't been working with bits and bytes recently, so recall that one byte is 8 bits. A bit can hold two values, either 1 or 0.
[01:05 - 01:13] And with 8 bits, we can hold 2 to the eighth values, which is 256 values. In JavaScript, we can convert numbers between bases like this.
[01:14 - 01:30] This converts 255 in base 10 into binary base 2. There's another common way to view the bytes of a color code, and that is hex encoding.
[01:31 - 01:35] If you've done any web development at all, you've probably seen this. This is just another way of encoding the same thing.
[01:36 - 01:44] Each RGB channel is encoded in two base 16 letters, which are then concatenated together in one string. So to convert our RGB value to hex, we could do this.
[01:45 - 02:06] We'll convert each base 10 string to hex. And then we'll concatenate them together.
[02:07 - 02:14] And notice that this is the same hex code we see in our browser for that same color. But there's one caveat here that's specific to our implementation.
[02:15 - 02:22] Conventional hex color codes require that the hex code have exactly six digits. But if our input channel is less than 16, we are only given a single digit.
[02:23 - 02:27] Here we're given a single digit C, and that's incorrect. It should be 0C.
[02:28 - 02:42] We can fix this by a JavaScript trick, where we prepend zeros and then slice them off. We can also convert colors the other direction, that is from hex back to base 10.
[02:43 - 02:54] In this case, we're taking a base 16 number and converting it to base 10. We'll use this sort of conversion throughout the course.
[02:55 - 03:05] We're going to store our pixels in our smart contract storage as three raw bytes. When we draw this pixel in our browser's canvas, we're going to convert from these raw bytes into base 10.
[03:06 - 03:17] When we upload pixels to our page from an image, we're going to convert the decimal colors back into hexadecimal. We're not going to do a lot of bit manipulation in this series, but because we 're passing pixels around, understanding this color encoding helps a lot.
This lesson preview is part of the Million Ether Homepage course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Million Ether Homepage, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
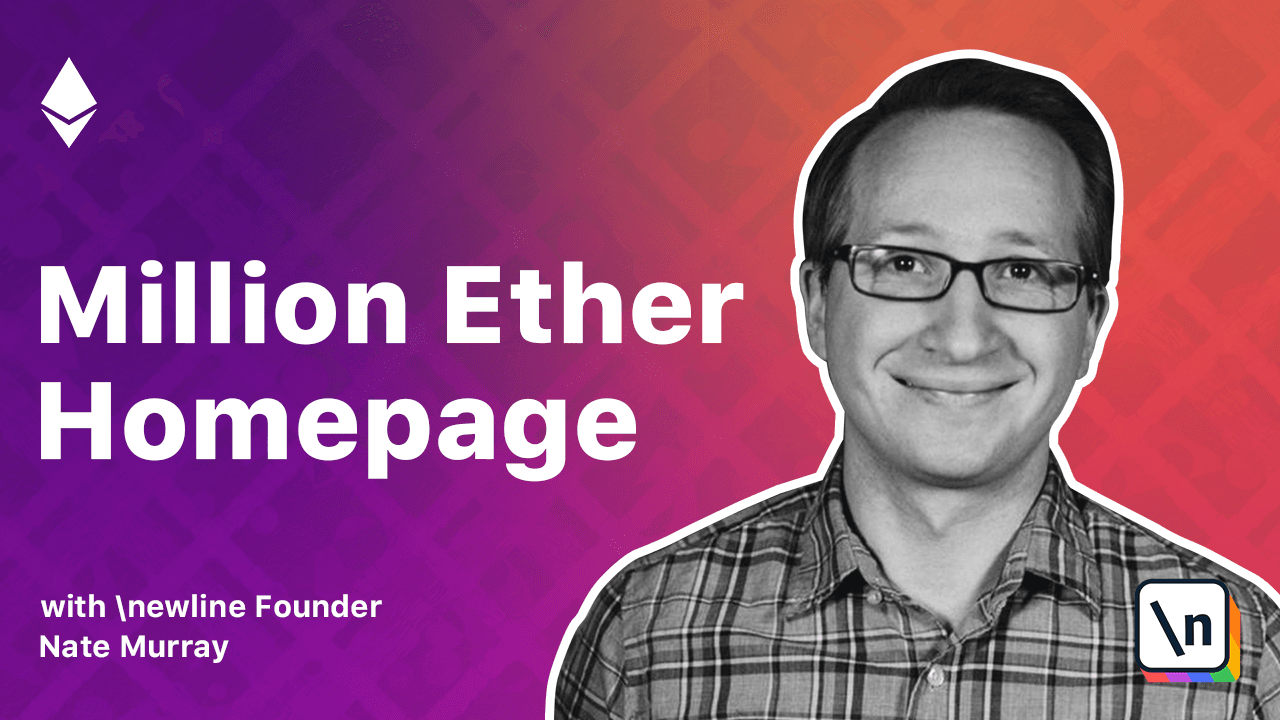
To create our page, we're going to be storing pixels in an Ethereum smart contract. To store colors, we have to pick a format to encode them in. Every byte we store on the Ethereum blockchain costs either, so it's important that we're careful about what format we use to store our pixels. Thankfully, we don't need to invent our own format. We're going to use 24-bit RGB color. What that means is that we're going to store one byte each for the red, green, and blue component of each pixel. For example, when we use the color picker in Chrome, we can specify colors in RGBA. RGBA stands for Red/Green/Blue/Alpha. Each one of these is called a channel, as in the red channel, or the alpha channel. The alpha specifies the transparency of the color, which we're not going to use , but you could if you wanted to. Notice that as we pick a different color, the values for red, green, and blue vary as a number between 0 and 255. That's because each channel is represented by one byte. I can understand if you haven't been working with bits and bytes recently, so recall that one byte is 8 bits. A bit can hold two values, either 1 or 0. And with 8 bits, we can hold 2 to the eighth values, which is 256 values. In JavaScript, we can convert numbers between bases like this. This converts 255 in base 10 into binary base 2. There's another common way to view the bytes of a color code, and that is hex encoding. If you've done any web development at all, you've probably seen this. This is just another way of encoding the same thing. Each RGB channel is encoded in two base 16 letters, which are then concatenated together in one string. So to convert our RGB value to hex, we could do this. We'll convert each base 10 string to hex. And then we'll concatenate them together. And notice that this is the same hex code we see in our browser for that same color. But there's one caveat here that's specific to our implementation. Conventional hex color codes require that the hex code have exactly six digits. But if our input channel is less than 16, we are only given a single digit. Here we're given a single digit C, and that's incorrect. It should be 0C. We can fix this by a JavaScript trick, where we prepend zeros and then slice them off. We can also convert colors the other direction, that is from hex back to base 10. In this case, we're taking a base 16 number and converting it to base 10. We'll use this sort of conversion throughout the course. We're going to store our pixels in our smart contract storage as three raw bytes. When we draw this pixel in our browser's canvas, we're going to convert from these raw bytes into base 10. When we upload pixels to our page from an image, we're going to convert the decimal colors back into hexadecimal. We're not going to do a lot of bit manipulation in this series, but because we 're passing pixels around, understanding this color encoding helps a lot.