This lesson preview is part of the Blazing Fast Next.js with React Server Components course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Blazing Fast Next.js with React Server Components, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
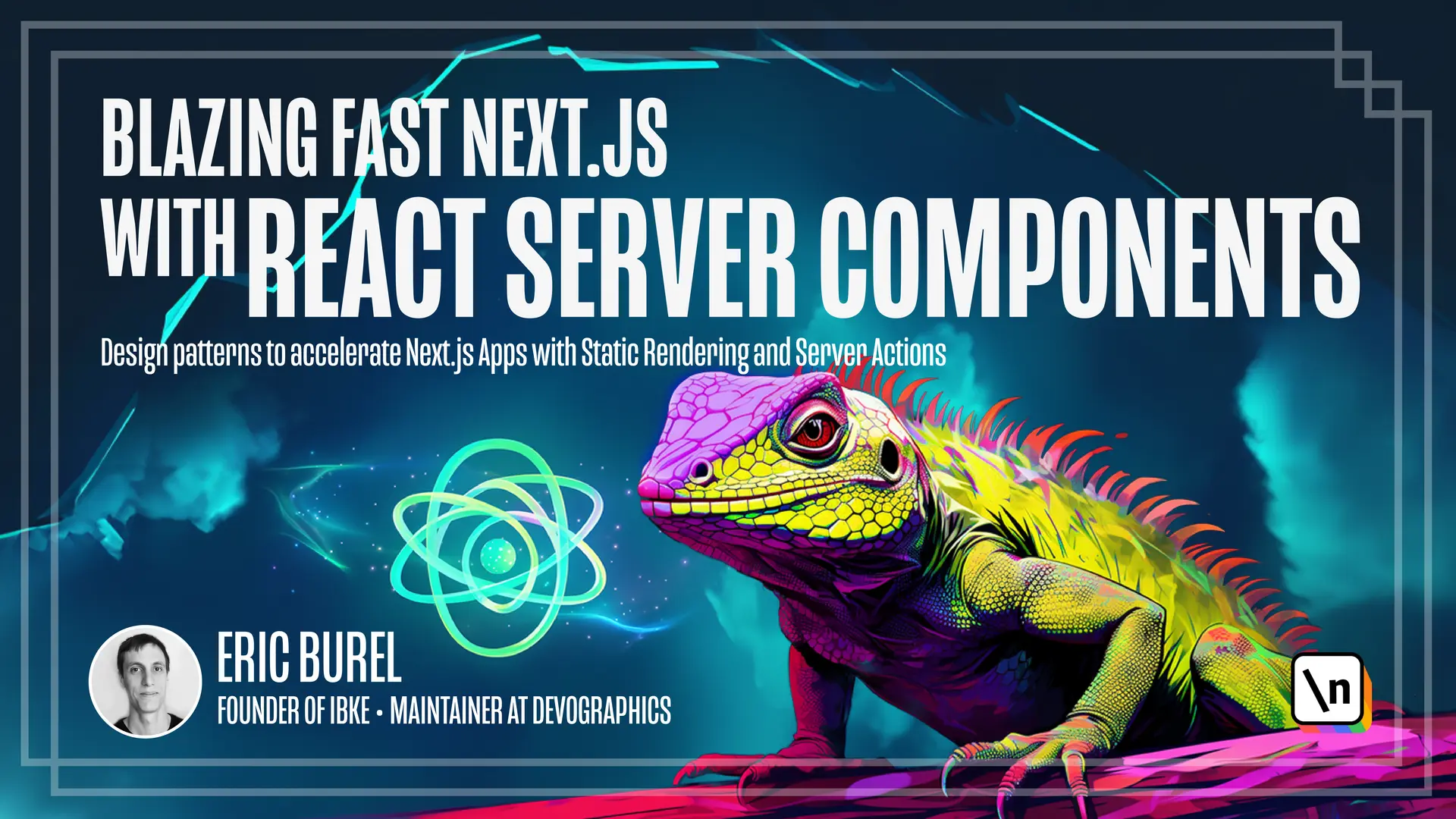
[00:00 - 00:09] Now let's say that we want a tiny glimpse of user-specific personalization on our website. We want to display the current user name.
[00:10 - 00:23] That's a nice thing to do to tell the user that we are thankful to have them, that we recognize them as a recurring user of our application. Those are the kinds of little touches that make a website great.
[00:24 - 00:31] This is achieved using your component named UserInfo. It is displayed there in the header.
[00:32 - 00:40] And we can see here the component implementation. Like for our product list, we have actually two components, the asynchronous component that will actually fetch the data.
[00:41 - 00:53] And a wrapper component that is the exported version that will be actually used in the application that wraps this component with a Suspense. This way we can under the loading step when the asynchronous call is running.
[00:54 - 01:00] Let's take a closer look. Our UserInfo component has to use cookies because it has to use the token.
[01:01 - 01:15] The authentification token stored in the cookies to tell who the user is and if there is even an authenticated user at the moment, maybe the token is empty and it's just a visit. Then it uses the transcription token to get the user.
[01:16 - 01:21] You might notice something wrong here. I'm using a getUserFromToken function directly.
[01:22 - 01:32] In a React Server Component, I should be using an rscGetUserFromToken function for instance. In order to immediately recognize that this function is safe to use in React server code.
[01:33 - 01:39] Let me fix that. I've defined rscGetUser which is just a cache version of my getUserFromToken function.
[01:40 - 01:51] It is safe to use in React server components and if I want to audit the security of my application, I just need to take a look at this "rsc" function. This function gets the user and then I can display them.
[01:52 - 02:04] The problem is that cookies are part of the HTTP request and we don't know the current HTTP request right ahead of time before the request happens, during build time. So this component cannot be statically rendered.
[02:05 - 02:08] It's specific to the current user. I just want to make a side-note here.
[02:09 - 02:14] Static rendering is not advised here because the content is user-specific. It is specific to a single user.
[02:15 - 02:27] But often I see is mistake that is static rendering is limited to public content that is the same for all users. Actually static rendering is also a good option when you have a group of users that are using the same value.
[02:28 - 02:40] If instead I wanted to display a flag indicating that the user is coming from a certain country so there are multiple users from the same country. I could use static rendering and a pattern that I call Segmented Rendering.
[02:41 - 02:51] It is out of scope for this course but I will link an article that I've written for Smashing Magazine that explains this pattern in details if you are interested. Anyway, here this component is specific to the current user.
[02:52 - 03:06] So we have to use per-request rendering, dynamic rendering because we need to access information from the request. Until very recently having one component that is dynamic would have forced the whole page to be dynamic.
[03:07 - 03:20] And at the time I'm writing this course this is actually still true. This feature will be probably released in the days or weeks to come with Next 14.1, while I'm currently using next 14.0.
[03:21 - 03:34] And this feature is named Partial Prendering. Before diving into it, let's take a look at what happens in the browser because I think, in this course, you may have noticed that I often open the network DevTools.
[03:35 - 03:45] This is really my source of truth to understand static and dynamic rendering. The other source is the terminal where I see new renders at runtime and renders that happen at built-time.
[03:46 - 04:00] It's important to log when I'm rendering the component to see why others. Here I see a few logs because my page is open and there are client-side requests from SWR so I can keep track of what happens in the application.
[04:01 - 04:09] It's very important to do so when you are learning, when you are discovering static and server-side dynamic rendering. Now if I go to my home page I see "Hello John doe!"
[04:10 - 04:20] I've added my UserInfo component and if I check the network tab, reload the page, I will see that this value is server-side rendered. I need to check the first request.
[04:21 - 04:26] Here and I see "Hello John Doe!" So this is indeed server-side rendered.
[04:27 - 04:34] But this is actually dynamically rendered. This is rendered for every request and I can see that in the terminal logs.
[04:35 - 04:40] Let me open my text editor. Here I see "Rendering UserInfo" back to the browser.
[04:41 - 04:48] I see it refresh. Back to the text editor I see again "Rendering UserInfo" but also "Rendering the home page".
[04:49 - 05:00] That's a shame the whole page is rerendering just because I need to rerender my UserInfo with potentially a new user. Because maybe I've logged out and signed in again with a different account.
[05:01 - 05:09] So every time for every request I have to check that the current user is still John Doe. This is because at this time, Partial Prerendering is not yet enabled.
[05:10 - 05:17] But still, let's discover this feature because it will be released anytime soon . So let's take a look at Next.js official documentation.
[05:18 - 05:27] I'm using the Next.js Learn tutorial as the source of truth here to understand this feature. It is not yet released so I have to trust the documentation.
[05:28 - 05:42] I can't run an experiment yet. By the way, I must tell that this tutorial is absolutely awesome and if you didn't follow it, even if you are an experienced Next.js developer, I really, really advise you to take a look at this tutorial and it's really a great tool.
[05:43 - 05:49] So Partial Prerendering is one of the modules of this tutorial. It's an experimental feature and produced in Next.js 14.
[05:50 - 05:58] Actually, not even 14.0 but in later minor versions of next. So make sure that your Next.js applications are up to date.
[05:59 - 06:08] If you notice that the whole page is dynamic if only one component is dynamic, it's probably because you are using another version of Next.js. And according to this documentation, we have nothing to do.
[06:09 - 06:26] Partial Prerendering will be most probably used automatically whenever we have a dynamic component within a static page. When we have a more technical explanation in the documentation, Partial Prerend ering is introduced as a compiler optimization that allows to have a static shell and within it dynamic components.
[06:27 - 06:37] So the static shell will appear immediately and the dynamic components will apply later on when they are rendered by the server dynamically. This is quite similar to client-side rendering.
[06:38 - 06:44] You have the static page and then client-side will relay when all the JavaScript is loaded. Except that it happens on the server.
[06:45 - 06:48] So it doesn't need client-side JavaScript anymore. It might be faster, it might be not.
[06:49 - 06:57] It depends on the user network connection and your budget because keep in mind that dynamic rendering is something that you pay. You need to render the page for every request.
[06:58 - 07:09] So keep in mind that it's not totally free but usually the cost structure is similar with client-side rendering because with client-side rendering you need to fetch the data from an API route. So you still pay the API route.
[07:10 - 07:16] So anywhere client-side and dynamic are slightly more expensive than static rendering. So keep that in mind.
[07:17 - 07:24] You might want to discuss with your infrastructure team to compare cost if you have a big application. With significant cost.
[07:25 - 07:35] You won't see the difference for a small application with a limited number of users. The documentation tells that the static part is considered a shell so it could be basically for a dashboard.
[07:36 - 07:52] It could be the structure of the dashboard with no data in it. But here in our application we go much further because we have virtually the whole e-commerce page that is static except for the tiny dynamic part that displays the current user name.
[07:53 - 08:00] And it makes sense in this situation to use static for the most part and dynamic for the little part. But this is actually complicated to implement.
[08:01 - 08:18] That's why only the most recent version of Next.js can propose that. This is because this feature relies also on new features of React like concurrent rendering that allows to have one part of the application that are rendered and other smaller part that are rendered concurrently.
[08:19 - 08:28] My understanding is that it first needed a raw refactor or rethink of React to make that possible in Next.js. At the moment it's not possible to do the reverse.
[08:29 - 08:39] Have a fully dynamic page or client-side render at page and static component. It's not possible to clear on only certain components of the application.
[08:40 - 08:50] And I don't think we can explain this feature to appear anytime soon because that would be a huge change in the way of React sees a page. In React a page is a tree.
[08:51 - 09:03] You have a root, a page component that will then render components that can then render more components and so on. And it's difficult to have parts of this tree that are statically rendered.
[09:04 - 09:21] It's not possible at the moment. But still, thanks to this module and the previous one, we are able to mix static rendering for most of the page with client-side rendering to update the number of pre- orders and dynamic rendering to display the current user name.
[09:22 - 09:42] We can keep static as far as possible whenever it's relevant and it's great because remember that static is fast, static is cheap, so we want static as much as possible and we want to scope client-side rendering and dynamic server-side rendering to where it belongs to the components where they make the most sense.