How to Install and Run ESLint When Creating React Libraries
Code linting and ensuring code style.
This lesson preview is part of the Creating React Libraries from Scratch course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Creating React Libraries from Scratch, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
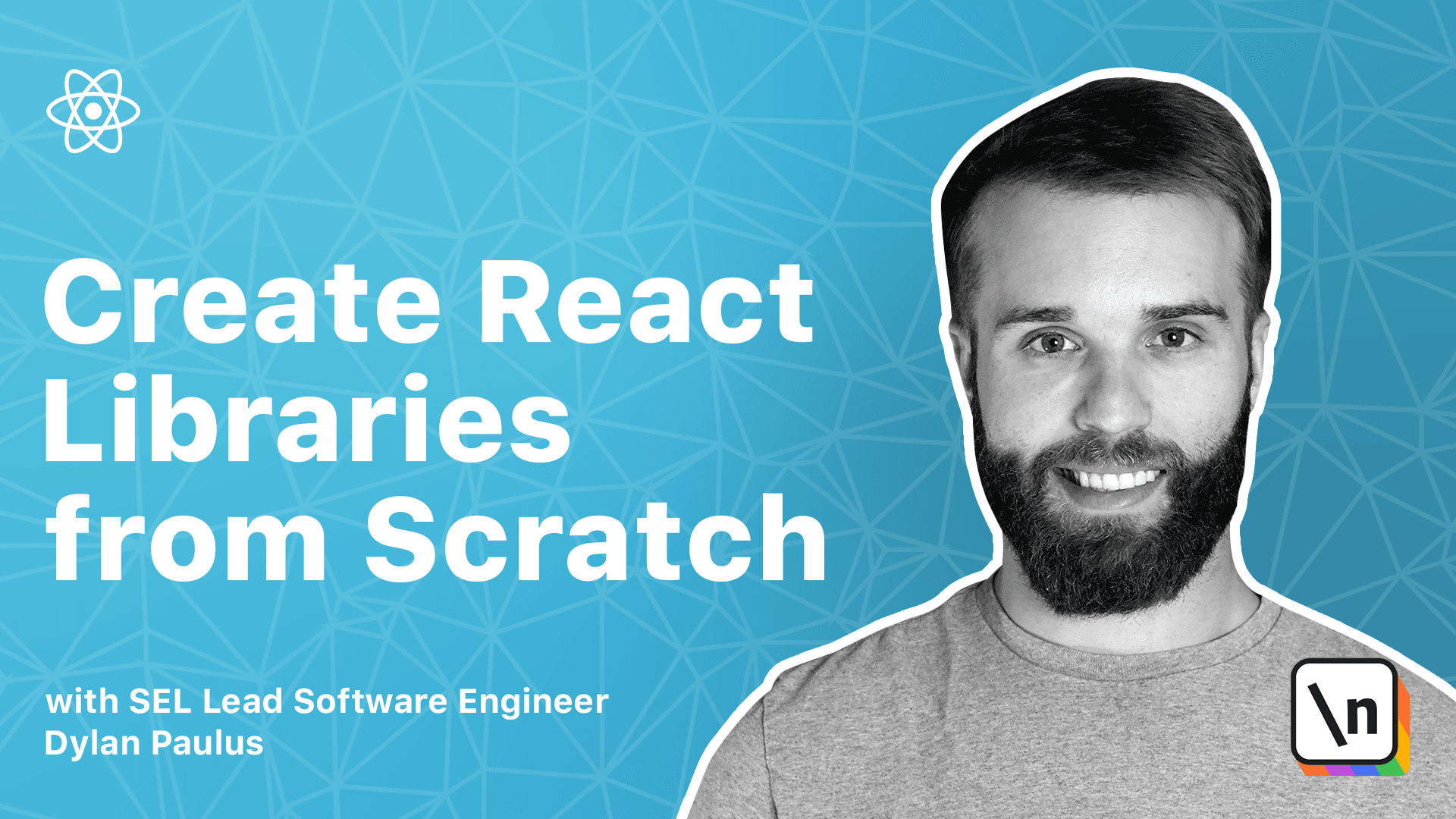
[00:00 - 00:09] Linters are used to analyze our source code to find common bugs, keep coding styles consistent, ensure we're using good practices. ESLint is the most popular Linter in the JavaScript community.
[00:10 - 00:20] In this lesson, we'll take a look at implementing an ESLint configuration and running Linting on our code. ESLint can be installed as a dev dependency by running Yarn, Add, ESLint, Dash, Dash, Dev.
[00:21 - 00:25] ESLint comes with a nice initialization script to get started. Run Yarn, ESLint, Dash, and Net.
[00:26 - 00:30] We'll be asked a bunch of questions about our project. How would you like to use ESLint?
[00:31 - 00:35] We'll say it to check syntax, find problems, and enforce code stuff. What type of modules does your project use?
[00:36 - 00:38] JavaScript modules. Which framework does your project use?
[00:39 - 00:40] React? Does your project use TypeScript?
[00:41 - 00:43] Yes. Where does your code run?
[00:44 - 00:46] The browser. How would you like to define a style for your project?
[00:47 - 00:49] Use a popular style guide. Which style guide would you want to follow?
[00:50 - 00:53] Airbnb? What format do you want your config file to be in?
[00:54 - 00:57] We'll say JavaScript. And finally, would you like to install them now with npm?
[00:58 - 01:04] Say yes. .eslintrc.js file is created after initialization, which contains the configuration for ESLint.
[01:05 - 01:13] Update our package.json file to include a lint script. In the script section, add lint to be ESLint/star.
[01:14 - 01:27] To run linting on all files in the source directory, we need to escape the double quotes because your terminal will try to resolve the glob, the star itself. With quotes around source, we ignore the terminal's glob resolution and use no JS's instead, which we want.
[01:28 - 01:33] Now run yarn lint to check any linting errors. ESLint init provided a couple of default rule sets.
[01:34 - 01:37] The first is plugin react recommended. The recommended linting rules for react.
[01:38 - 01:44] And armbab, which is a popular code standard for the JavaScript language. The armbab configuration enforces single quotes when creating strings.
[01:45 - 01:54] But we've been using double quotes in Scroller. In the rule section of our .eslintrc.js file, add a new rule to create an error if we don't use double quotes instead of single quotes.
[01:55 - 02:03] Rules we specify will override the default rules given from the extends configuration. We also can turn rules off by adding rules with an off value.
[02:04 - 02:17] Override a few of armbab configurations to match our style. There could be files that are auto-generated in our project or files we don't want to check for code stylus.
[02:18 - 02:28] ESLint gives us a few escape hatches to ignore linting on whole files or individual files. To demonstrate this, we'll assume that the stories directory don't need linting , though you may consider linting stories yourself.
[02:29 - 02:44] ESLint has a .eslint ignore file, similar to .mpm ignore, which will tell ESL int to ignore the specific files. Read a new file called .eslint ignore and add stories as an entry.
[02:45 - 02:53] Run your own lint and you should find that the usescroller.stories.js will no longer give linting errors. There are cases when we may want to turn off linting for specific lines of code .
[02:54 - 03:01] We can do this by adding code comments. In source/usescroller.ts we have a linting error, functions usescroller expected no return value.
[03:02 - 03:18] Navigate to source/usescroller.ts and add /slint-disabled-next-line on line 21 before return function scroller, which will ignore linting on the line after this comment. We have errors in usescroller.test.ts.
[03:19 - 03:27] We have errors in usescroller.tslint.js to find its own global variables. Add ESLint plugin.js as a dev dependency by running yarn.
[03:28 - 03:36] Add ESLint-plugin-js-dev. Next, we will update our .eslint.rscjs file to apply the jest plugin.
[03:37 - 03:50] Add just/globels to be true in the emv property to tell ESLint about jest global variables. Finally, add jest as a plugin.
[03:51 - 03:58] Run yarn lint to see our test code having no more errors. To resolve the last two errors we need to tell ESLint how to handle importing TypeScript files.
[03:59 - 04:06] Another plugin is needed. So run yarn add ESLint-import-resolver-typescript-dev.
[04:07 - 04:27] To install the TypeScript Resolver, then update.eslint-rc to use our tsk config .json file at the root of scroller by adding a settings property. In send settings, have import/resolver and then have an empty object for Type Script.
[04:28 - 04:29] Now run yarn lint to see no linting errors.