How to Install Jest and Run Your First Unit Test
Testing our code through unit tests.
This lesson preview is part of the Creating React Libraries from Scratch course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Creating React Libraries from Scratch, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
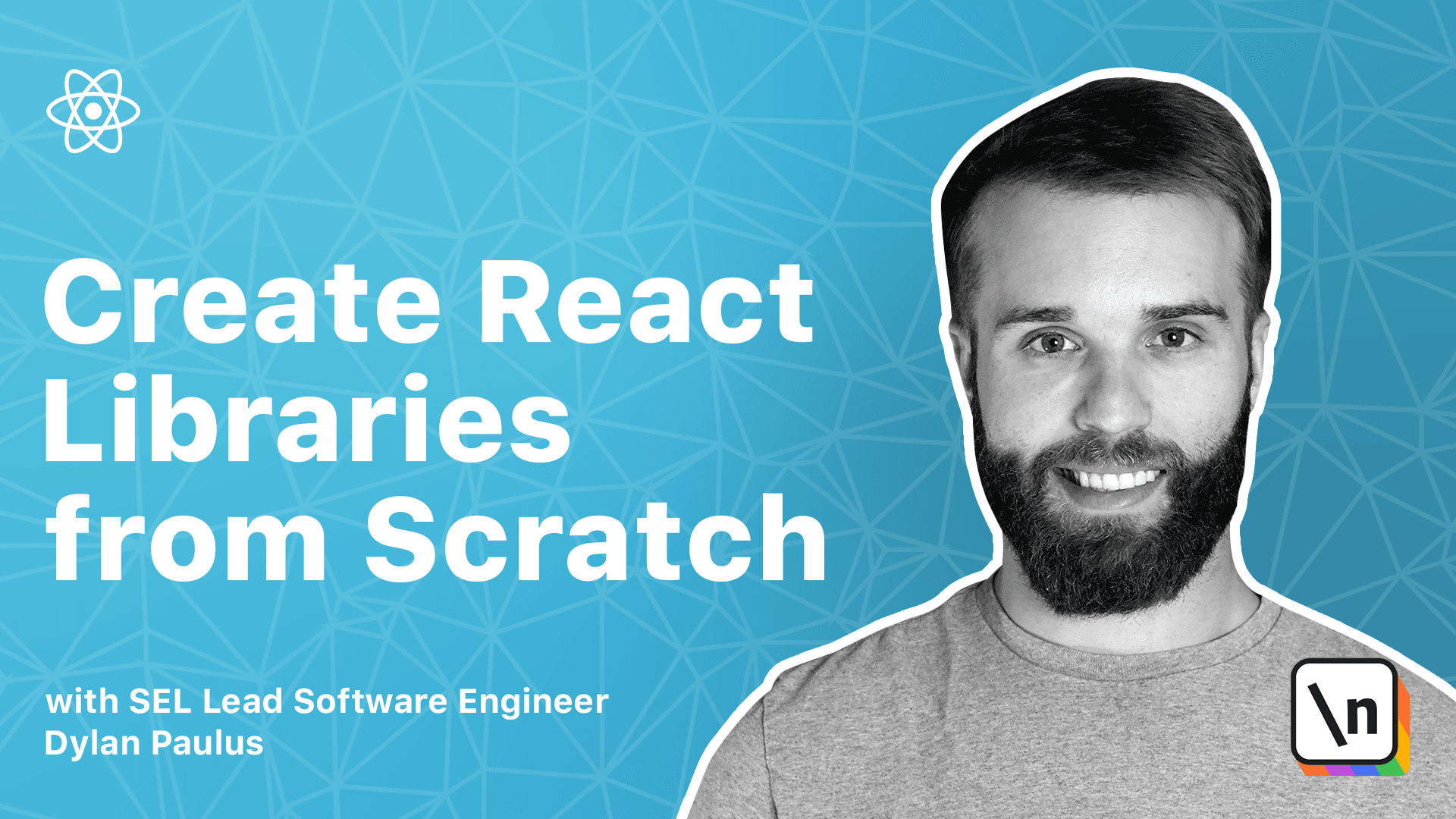
[00:00 - 00:32] As our library grows in popularity, contributors, and lines of code, it can become hard to ensure code is correct. There are many forms of code testing, such as unit tests, integration tests, end-to-end tests, smoke tests, etc. Just as a JavaScript testing framework that can run different forms of testing, but the most common is unit tests. Unit tests are automated tests run by the computer that check if a section of code is correct. Usually this is a functional method. Unit tests are quick, small tests that don't require outside systems, for example, databases.
[00:33 - 01:00] To learn how to integrate testing and testing our project, we'll add a unit test for the use-scroller hook. Testing is only done in a development environment, so we'll install just as a dev dependency. Run YarnAddJust-dev. Continue from the last lesson, we'll be using TypeScript to write our tests. To include types for just, we need to install the TypeScript package. Run YarnAddAt types/just-dev. Just natively doesn't know how to validate types for types.
[01:01 - 01:34] To do this, we'll need to install a plugin called TSGest by running YarnAddTST ASGest-dev. Next, we'll tell Jess to use TSGest by using TSGest init script. Run YarnTSTASG est-config-colon init, which will create a new jest.config.js file. Open this file and change the test environment from node to jest-dom. Jest-dom will allow us to use browser-specific functionality inside the testing node environment. For example, the window object we'll be using.
[01:35 - 02:10] Finally, in our package.json file, add a new script called test, which runs jest. In the source directory, create a new file called use-scroller.test.ts. Jest will automatically run files with a .test in their file extension. Enter the following code. Don't worry, we'll be breaking down this test real soon. But for now, let's check out what each section is doing. Most tests follow a similar pattern . Set up the environment, run the code under test, then make sure we get the correct outcome . Arrange, act, assert, or you'll see it as AAA is a pattern to help write good tests, which mirror this behavior.
[02:11 - 02:32] Before writing any code, I like to create comments for each action to ensure I follow Arrange, act, assert. The Arrange step sets up the test, mocking, creating variables, and setting up our initial React.js structure should all live here. Act is where we execute the code in our test. In the code above, this is our scroll function being called. Assert is to run any assertions.
[02:33 - 02:55] To make sure our code, we run and act, behaves as we expect. In use scroll.test .ts, we want to test that calling the scroll function causes the window to scroll. To keep our test fast, we don't want to require a browser instance to test if our code actually scrolls a screen. This is more suited to end-to-end testing. Instead, we'll create a spy to stab or replace browser functionality.
[02:56 - 03:41] We apply a spy on the window object to intercept calls to scroll to. When using spy and jask, call in window.scroll2 will first execute the spy stub, then call the original implementation of the function work spying on. To prevent the actual window.scroll2 function from being called, we need to provide a mock implementation for a spy. If we wanted to change what window.scroll2 returned after being called, we could provide a function as an argument to mock implementation. Instead, we just want to prevent the original implementation from being called, so we'll call mock implementation without arguments. Next, we set up the usescr oll2 hook with arbitrary parameters we want to test. Since usescroll2 can return early if no window object exists, we have to check if scroll is undefined, though with a spy defined this should never be true.
[03:42 - 04:14] Moving to the axe section, we only need to call the scroll function return from the usescroll hook. In the asserts section, we'll run assertions to make sure window.scroll2 is called correctly from the scroll function. We can access the spy mock to CMA times window.scroll 2 was called, in which parameters were used. The to be called with matcher will check if a spy or mock is called with a given parameters. Finally, in the test, we need to make sure we restore our spy mock. Test should be good neighbors. It is good practice to revert any mocks to the original implementation.
[04:15 - 04:30] This will make sure our tests are predictable, don't rely on each other, and can be random parallel. spy.mock restore will turn off any mock and recreate it through the spy object, and restore the object to its original implementation. Run yarn test. Congrats, we've written our first unit test.