Reactive Primitives in Signals
Here, you will get to learn about the different Reactive primitives available in Signals, which are writable signals, computed and effects.
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Demystifying Reactivity with Angular Signals course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Demystifying Reactivity with Angular Signals, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
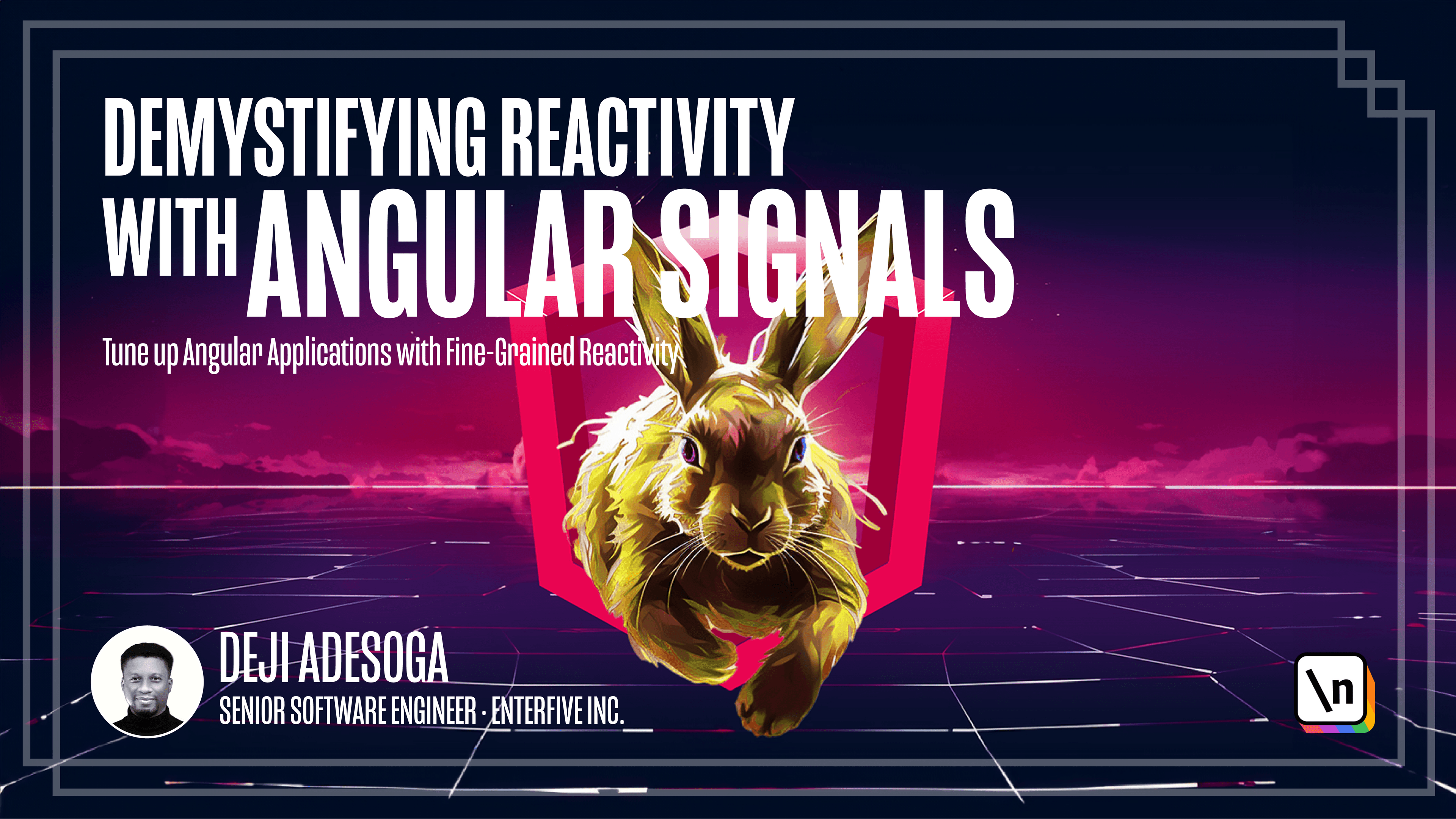