Editing the resume content
Make the resume content editable by learning the v-model and the v-on directive, methods, and how to handle events in Vue.js.
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Interactive Vue.js Resume Builder course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Interactive Vue.js Resume Builder, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
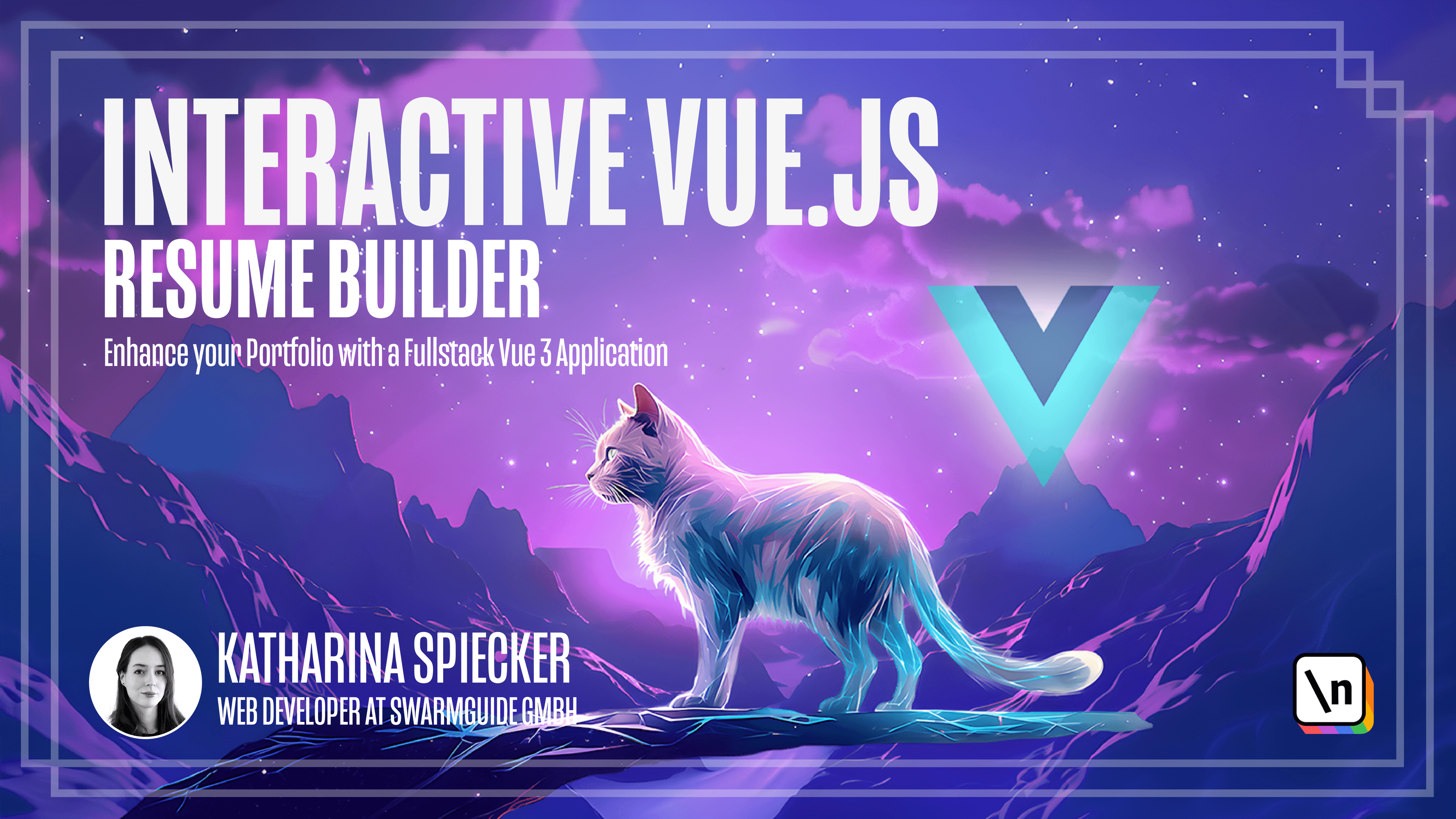