An Introduction to Creating Django Models [with Examples]
Let's enhance our project a bit
This lesson preview is part of the Serverless Django with Zappa course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Serverless Django with Zappa, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
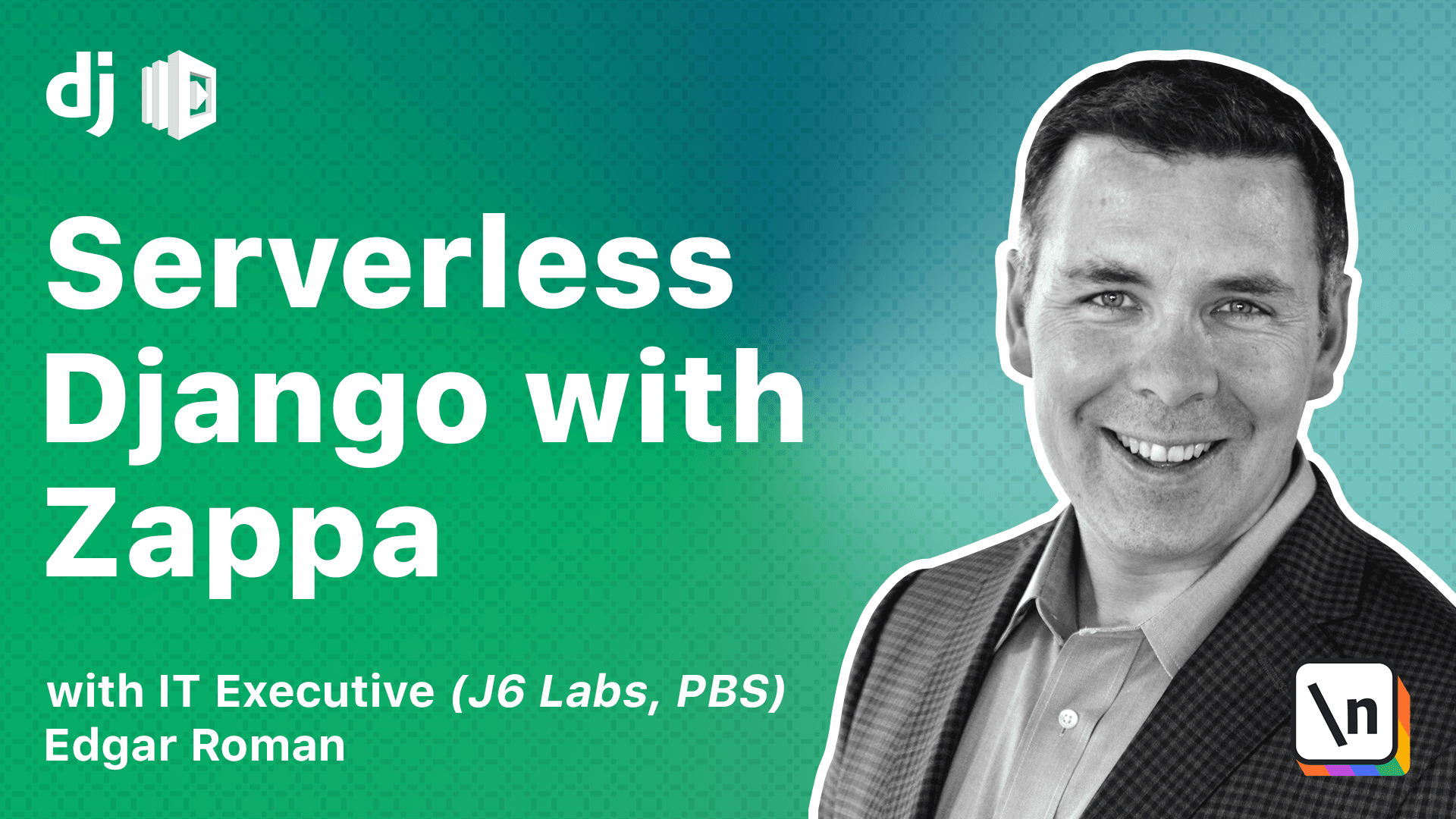
[00:00 - 00:11] All right, folks, so we have verified that our application can connect to the database. We are going to enhance our app a little bit to make it a little more interesting.
[00:12 - 00:23] Let's take our Django app and make it into a micro-blogging site. We can have a micro-blog about our progress of learning to play the guitar.
[00:24 - 00:41] So if we go back to our Django settings file, we are going to enable the guitar app that we initially created way back in earlier lessons. All right, so this activates the application.
[00:42 - 00:55] We're going to go to the models because without models, we'll have no reason to connect to the database. Now I'm going to just cut and paste some models here.
[00:56 - 01:00] This is a pretty simple model here. So this journal entry is pretty straightforward.
[01:01 - 01:12] It has a title, timestamp, and the content of our micro-blog. We're not really going to dive deep into how to structure models for Django.
[01:13 - 01:17] We're focusing this course on Zappa. So I'm going to skim over some of these configurations.
[01:18 - 01:30] Now we're going to enable the admin site to view this model. So I'm going to go to admin here and paste.
[01:31 - 01:45] Very simple administration widget so we can view our entries and that should do it. This is the absolute bare minimum we need to enable models in our Django app.
[01:46 - 02:01] And now I'm going to update our cloud-based instance. Cool.
[02:02 - 02:14] Now that that's finished, we're going to move on to initializing our database. If you're familiar with Django, Django will automatically create schema files for you from your models.
[02:15 - 02:31] And so all we have to do is run the make migrations management command, which I 'm going to go ahead and run right now. It's going to create some files locally that will insert the schema into our Postgres database.
[02:32 - 02:52] Now that we have the migrations ready, I just have to actually run migrate. Now this command will connect to our RDS instance and insert the schema into our database.
[02:53 - 03:04] Now you'll note that there is only really one schema for our application guitar . There's a bunch of other migrations that are used for the admin console.
[03:05 - 03:24] So that's what most of this output is for. The last thing we need to do is create our super user so we can log into the Django admin.
[03:25 - 03:28] Here we're creating our super user. You can use any login you'd like.
[03:29 - 03:44] I traditionally use admin and I have a fake email address here. Okay.
[03:45 - 03:53] And our super user is created successfully. Now I can log in using this super user.
[03:54 - 04:00] So let's go to our browser. Here we are at our website.
[04:01 - 04:04] I've been admin. And here we are.
[04:05 - 04:13] We are in the admin. You'll notice in addition to the standard Django admin widgets here, you have our application and we have our journal entries here.
[04:14 - 04:28] And this is a fully functional widget here. I can add some journal entries.
[04:29 - 04:45] So this is bought my first guitar. And I put the timestamp as maybe Saturday and I went to the store about my first guitar.
[04:46 - 04:54] I'm going to save and add another. Put some of this already in here.
[04:55 - 05:06] Add another. The content is not important.
[05:07 - 05:18] Quick spin through the admin shows now I have three micro entries on my micro blog. And things are working just fine.
[05:19 - 05:40] At this point though, if I go back to just the main site, we haven't invested any time or effort into our front end website. So what I'm going to do for completeness and I'm going to enhance our Django application to actually retrieve the blog entries and display them.
[05:41 - 05:49] So let's do that now. Once again, I'm not going to spend a lot of time fixing up the front end and making it look nice, but I'm going to make it look decent.
[05:50 - 05:59] All right, so we go back to our views. And now I can replace the views with.
[06:00 - 06:22] Okay, so I just enhanced our view to have the importer models from journal entry and I've done a quick query to get all blog entries and return them in the context. Then I need a quick template for my application.
[06:23 - 06:36] So let me create the template application under templates. I can insert index dot html.
[06:37 - 06:46] I'm going to paste a bunch of code here. So very simple HTML document as a very simple head section.
[06:47 - 07:12] I am using the semantic UI CDN delivery here for my style sheets because it looks nice and I don't need to invest a lot into this. Okay, for the body itself, I have a quick H1 class, my guitar journal, and then my template will loop through all the entries that are returned from the database and just print them out.
[07:13 - 07:27] Nothing super fancy. Now I just need to push all this code up to the cloud.
[07:28 - 07:54] Okay, now that that's complete, I go back to my website and refresh. We have our micro blog site with our three journal entries that we added in.
[07:55 - 08:04] Now you notice it's not very fancy. This is not a course on UI design for sure, but I wanted to clean it up just a little bit to make it look nice.
[08:05 - 08:11] Okay, so then in this lesson, we enhance our Django application to have models. We initialize the database.
[08:12 - 08:25] We created a super user and we were able to log into the admin to create and manipulate database objects. In the next lesson, we'll wrap up the database lessons that we've learned so far.