Building a News Site - Fetching Articles
Fetch and render articles from the newsapi.org API
This lesson preview is part of the Sleek Next.JS Applications with shadcn/ui course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Sleek Next.JS Applications with shadcn/ui, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
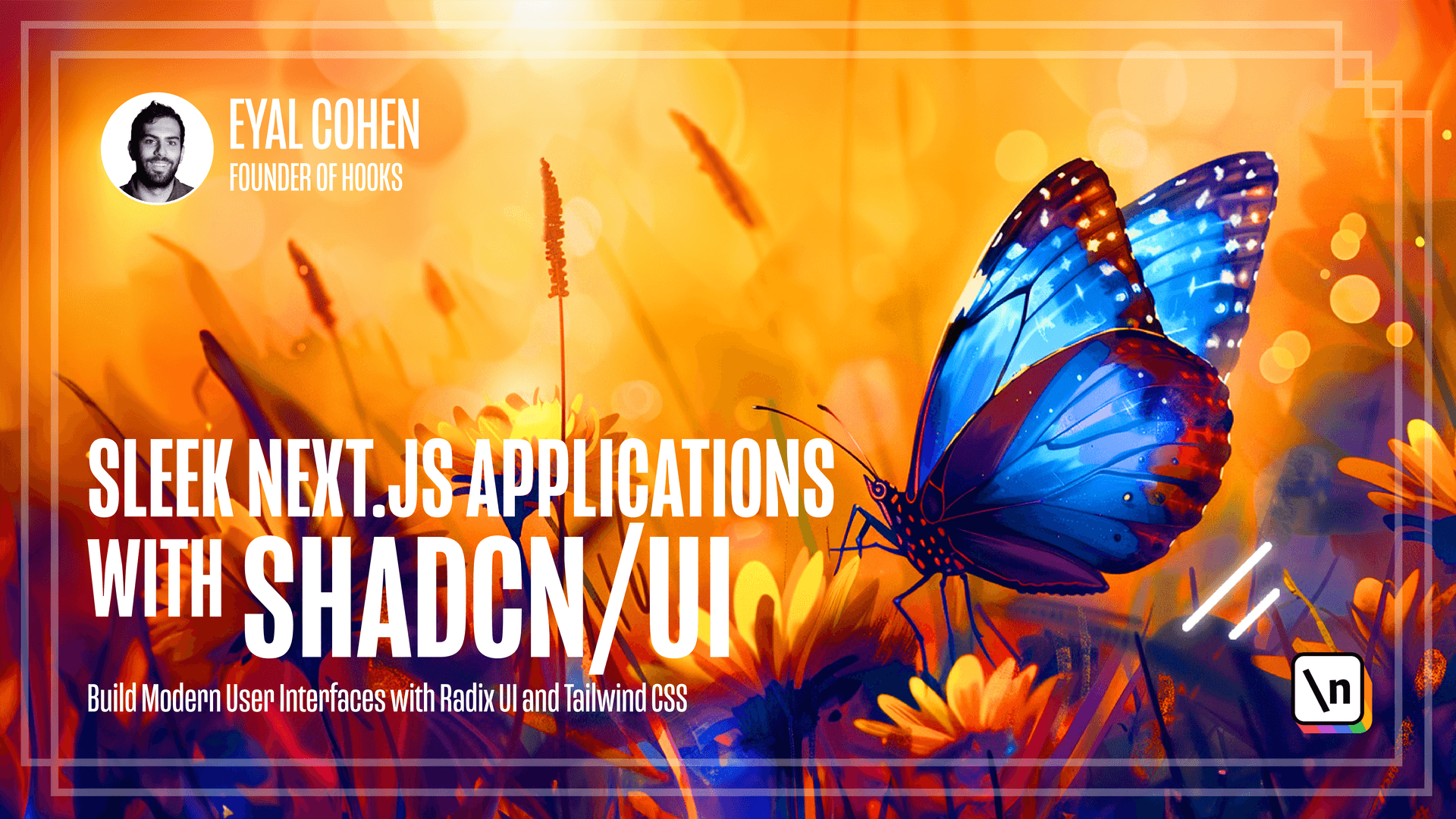
[00:00 - 00:10] In the previous lesson, you build the header category component. In this lesson, you are going to use the newsapi.org to fetch articles.
[00:11 - 00:21] The API is free to use, but there is a quick registration process you need to go through on their site. So navigate to newsapi.org and click on the "Get an API key".
[00:22 - 00:38] Fill in the details, and then you will be able to grab your API key. Now copy the API key and create a new file called .env in the root folder of the project.
[00:39 - 00:55] Now create a "News_API_Key" inside the file and paste the API key you've just copied. Now add the "News_API_URL" key as well and paste the following URL into that.
[00:56 - 01:10] https://newsapi.org/v2 Great. Now let's navigate to the newsapi.org documentation.
[01:11 - 01:20] The API endpoint you are going to fetch articles from is called "Top Headlines ". Return type of the API endpoint can be seen in the docs.
[01:21 - 01:36] So the article includes a source, author, title, description, URL, URL to image , publish that and content. I want to take this type and create a TypeScript type out of it that can be used in the app.
[01:37 - 02:05] Create a new file called "Type/article.ts" and add the following code to it. For type "article = source" with the name string inside of it, author, title, description, URL, URL to image, publishedAt and content.
[02:06 - 02:09] Then add the category type. So "Expert type" category.
[02:10 - 02:24] Equal the strings of the categories we previously added. Health, science, sports and technology.
[02:25 - 02:29] This will help making the requests type save. It's now time to fetch the article.
[02:30 - 02:53] So create a new file at lib/news-api.ts. Import the types you've just created.
[02:54 - 03:22] Then create the fetchheadlines function "export const fetchheadlines" = "n assing function" and the function receives a category as a param inside an object because we want to make it type. And the function returns a promise which is array of articles.
[03:23 - 03:33] Great. Now we want to make the request using fetch.
[03:34 - 03:42] The request will be to topheadlines. We want to pass in the API key.
[03:43 - 04:02] And we want to pass a country. And we want to pass a category but only if it exists.
[04:03 - 04:10] Now convert the request body into a JSON response. So const articles = await request.json.
[04:11 - 04:21] Then we want to tell type script it returns an article which is an array of the article type. Now we want to return the articles but we want to filter them.
[04:22 - 04:29] Sometimes the API return title = removed. And we don't want articles which doesn't have an image.
[04:30 - 04:37] So title is different then. Removed and URL to image exists.
[04:38 - 04:41] Perfect. It's now time to test the function.
[04:42 - 04:53] So inside app/page.tsx import the fetchHeadlines function first. Then turn the index page into a nesting component.
[04:54 - 05:03] And call the fetch_headlines function const_articles = fetch_headlines. And we want to console.log the articles.
[05:04 - 05:09] We will not pass a category. Or we will pass the business category instead.
[05:10 - 05:28] Now run the app. And navigate to localhost 3000.
[05:29 - 05:36] You should see in the server a list of the components. We forgot to await.
[05:37 - 05:45] So don't forget to inside the page to await the request so it returns a promise . So reload the page.
[05:46 - 05:54] And you can now see a list of the articles being returned from the server. In the next lesson you will use the articles to render the news page.