How to Mock Storybook App Loading Scenarios With MSW
Let's mock the API scenarios with MSW.
This lesson preview is part of the Storybook for React Apps course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Storybook for React Apps, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
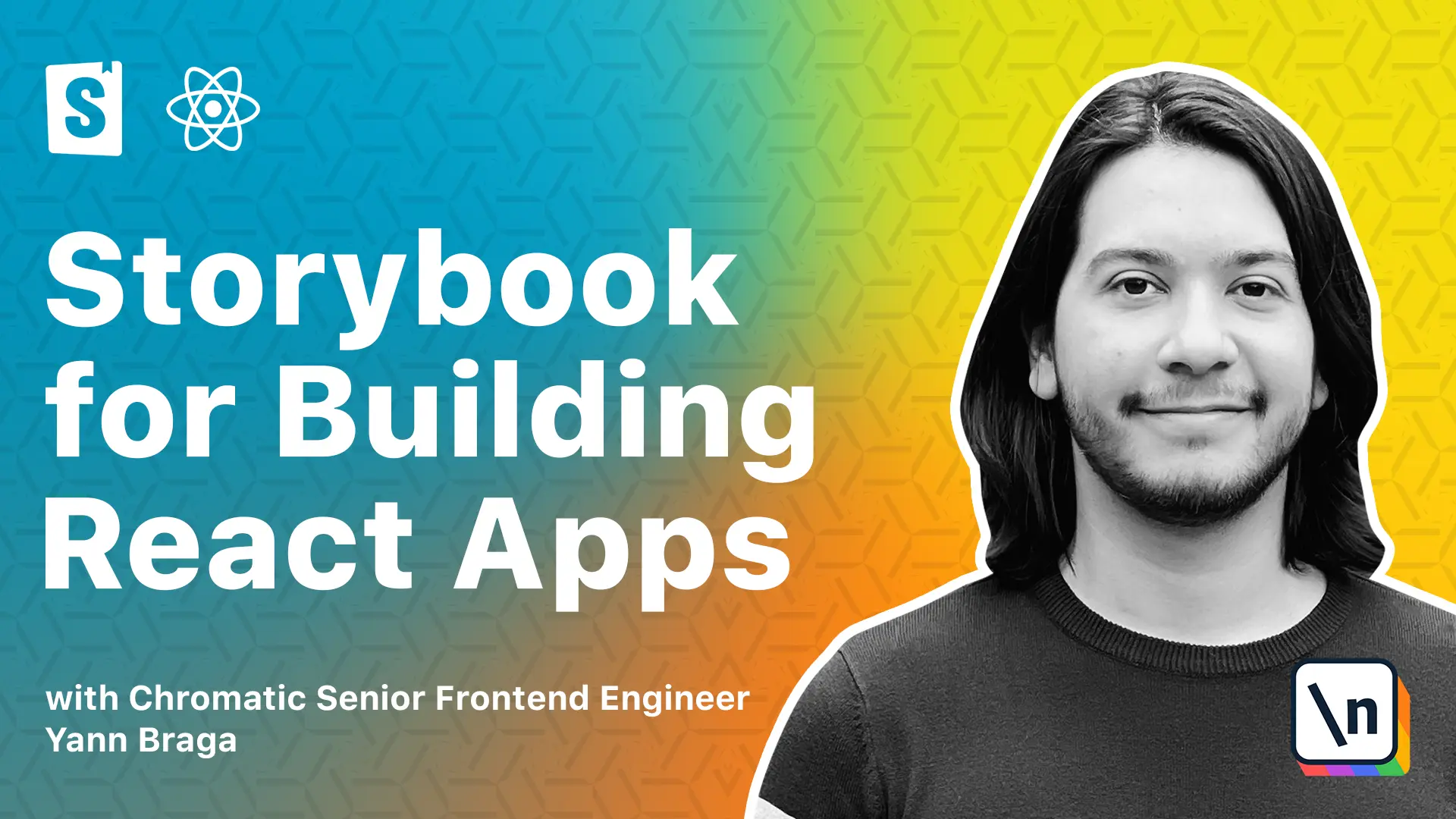
[00:00 - 00:10] Alright, now it's time to add the loading error in 404 scenarios to our stories . So let's go back to the Storycode and we learned from previous lessons that we can use mock service worker to mock the data.
[00:11 - 00:26] So what we can do here is, first of all, we need to import a REST operator from mock service worker. We also need to import a base URL from the API, which is two levels above.
[00:27 - 00:46] And lastly, we need to import from stubs, we need to get the restaurant's mock data. So once we have all of these necessary pieces, we can go under the success story and add a new parameter, which is a sw and the MSW receives a property called handlers, which is an array.
[00:47 - 01:07] And then we call rest dot get, which is the operation we want to mock with the base URL that we're passing, and then this receives a callback, which is going to receive the request, the response and the context. And then that essentially returns a response with a context dot JSON of our restaurant's data.
[01:08 - 01:12] And let's just use the first one. So when we check Storybook, it's actually just rendering the mock data.
[01:13 - 01:23] Regardless, if we refresh the browser, it's not going to fetch any data. And if we look into the implementation of the restaurant detail page, we're essentially going through the success scenario.
[01:24 - 01:34] But we've also got a loading scenario, a 404 and 500. So let's use the mock service worker to also reach all of these scenarios as different stories.
[01:35 - 01:40] And because I don't want to be typing too much, I'm just going to paste the entire code for the stories here. And let's go through them.
[01:41 - 01:50] So we have a loading story, which has its own design. And it's got a mock service worker, which is using context dot delay with the infinite property.
[01:51 - 02:00] And that essentially will never resolve the request, which is being sent. And then we've got not found, which is using context dot status, passing the 404 status as a response.
[02:01 - 02:09] And then the error one, which is context dot status 500. So if we look back into our storybook, we have all of these different states available as stories.
[02:10 - 02:19] We have the loading state, which is actually really good to have a story because it's pretty difficult to reproduce in the real app. We've got to not found, which is showing this animation.
[02:20 - 02:24] And then we've got the error, which is also showing an animation. And that's it.
[02:25 - 02:35] Because we've added support for a bunch of functionality along the way, adding any component, feature or page to storybook will be a piece of cake. This is the last component we'll be adding to storybook in this course.
[02:36 - 02:47] And as an exercise, you can add other components or even create new ones from scratch directly in storybook. You can always refer to the meal drop repository to see a complete storybook with every single component in it.
[02:48 - 02:58] And hopefully, you can use it as a showcase for your colleagues to show all the interesting value that storybook can bring to your project. In fact, there's even more testing value, which we'll be talking about in the next module.
[02:59 - 02:59] So I'll see you then.