Getting Started with React - Building Your First App
Now that we've introduced our very first app, the Greeting App, this lesson will cover the actual building of it, step by step.
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Beginner's Guide to Real World React course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Beginner's Guide to Real World React, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
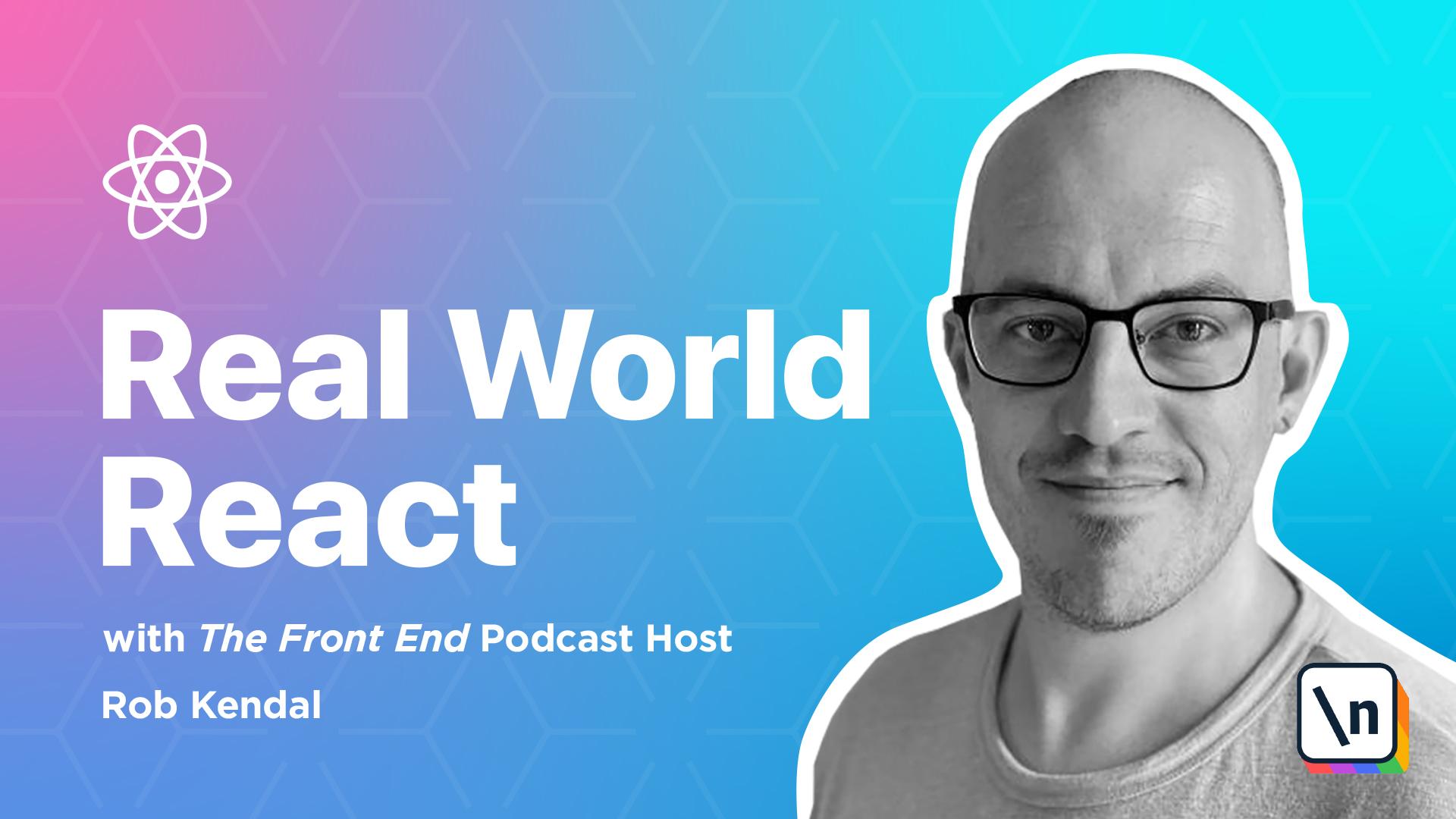
[00:00 - 00:13] Hello and welcome to our very first React demo, as part of the Beginner's Guide to Real World React course. The first real example we're going to build is a really simple greeting app that's going to accept a name from an input box and I'll put it back to the user as part of a welcoming message.
[00:14 - 00:21] So the first thing we need to do is to add the parcel bundler. Now you can just use React by loading it using a script tag for a package repository like Unpackage.
[00:22 - 00:41] However, what you'll find with most real life React projects is that it will be adding React libraries over NPM and usually some sort of code bundler such as webpack or even the Create React app starter project which uses webpack under the hood. We're going to do something similar to get you familiar with using a common means to build and run a React project and for this we're going to use Parcel JS.
[00:42 - 00:51] Parcel JS is a really simple code bundler that's very much like webpack but without a lot of the complex configuration setup. In its own words, Parcel JS is a blazing fast zero config web application bund ler.
[00:52 - 01:09] So you can see I've got the Parcel JS website open which you can find by navigating to Parcel JS.org and we're going to head over to the Getting Started section. We'll need to install it globally onto our machine and you can do that by using the commands in the course documentation.
[01:10 - 01:25] It should be NPMI-G, Parcel - bundler or if you're using yarn, yarn, dash global, add Parcel - bundler and you can see those here. Once you've done that we'll close this window up and we'll open a brand new project in VS code.
[01:26 - 01:36] So I've got a shiny new empty folder that I've opened in VS code. I've got a git ignore file in the readme in here because I've got this connected with a git repository but we're not going to use those files and you can ignore them for now.
[01:37 - 01:44] Because we're using Parcel we don't need to do any configuration to make it work but we do need to add React to our project. So you can see I'm in VS code.
[01:45 - 01:56] If you open up the terminal in VS code which you can find by clicking down here this will open up this kind of terminal and output bin. If we click on the terminal you'll see that it opens up in the current working directory of our project.
[01:57 - 02:03] From here we're going to need to do three steps to get our project up and running with everything we need. Number one we need to initialize our project.
[02:04 - 02:12] Two we need to add the React dependencies and three we need to add a shortcut script to build and run our code. So the first thing we're going to do is initialize our project.
[02:13 - 02:24] The first step to initialize our project is to get a package.js and file up and running. To do that we're going to run the command yarn init and dash y.
[02:25 - 02:40] We add the dash y flag which will automatically answer yes to all the initialization questions such as who's the author, what's the license and those sort of questions that we don't really care about for our quick example. You can see it's created as a package.js and file here.
[02:41 - 02:44] So step two we need to add the React dependencies. So now we're going to add React to the project.
[02:45 - 02:51] There's two packages we need that is React and React DOM. React is the star of the show and includes the core React library.
[02:52 - 03:01] React DOM is a secondary package that is responsible for rendering our components to the DOM in the browser. Back in our terminal we need to type a following command to add both packages to the project.
[03:02 - 03:13] That's yarn add react and react dash DOM. Yarn is very well cached and very fast so both of these packages should be added to the project in no time at all.
[03:14 - 03:21] And you can see there we go we've got React and React DOM. So the final step is to add the script for building and running the code.
[03:22 - 03:28] This is the final part of the setup and it involves the package.js and file that I've got open here. Notice the React and React DOM dependencies are in there.
[03:29 - 03:36] Now once we've built our app we need to call pastel to bundle everything up and run it. It's not a huge chore but we can add a simple shortcut to help us out.
[03:37 - 04:06] In our package.js and file we need to add a new property scripts and we need to add a new command property under this and we'll call it start. Now inside this start build command we need to add the following instructions, parcel, index.html, dash, open and we'll save that file.
[04:07 - 04:17] So the new part of the package.js and file should look like this. We've added a scripts tag and then we've added a start command with the command parcel index.html dash open.
[04:18 - 04:38] What we've just written there is a terminal shortcut that will allow us to type yarn start and have our parcel command open our starting file and the dash open flag tells parcel to open the running code in the browser once the files have been compiled and parcels development servers up and running. You notice I've made a slight mistake there we need to have two dashes before the open.
[04:39 - 04:55] Parcel works by giving it an entry point in this case ours is index.html which we'll be building next and from there Parcel works out which files it needs to include in the bundle based on your import and references. So coding the project files now we're on to the exciting part building out our example.
[04:56 - 05:04] Now for smaller projects like this I like to just start by creating all the files we need and filling them in as we go along. Now we're going to create four files so let's create them now.
[05:05 - 05:18] The first thing we'll need is a styles.css file. We'll just have some basic styles in here to make our app look just slightly more interested than the out of the box HTML.
[05:19 - 05:27] We need our parcels starting point index.html. This is going to be our first entry point the parcels going to look for to render our app.
[05:28 - 05:41] As a starting point for the JavaScript we need index.js and finally our main React component which we'll call app.js. This is our main entry point for the React side of things.
[05:42 - 05:57] Once we have the files created let's start filling them out and we'll start with our styles.css file. Our styles file isn't exactly essential at this point but it's nice to have a few basic styles available to improve the built in look and feel the browser's give us by default.
[05:58 - 06:09] So you can see that I'm just going to copy in some really simple styles that just affect the body and the font size and the line height. Later on we'll be adding an input and a button to our app so I've added some nice styles in there for those as well.
[06:10 - 06:19] Give that a save and we'll move on to our entry point our index.html file. The next thing we want to do is add some HTML into our index.html file.
[06:20 - 06:44] I'm using Emmet that comes with VS Code because it allows me to just type the name of the HTML element like this rather than having to type it all out by hand so it's a nice little time saver. So what we'll add our HTML tag first inside that we're going to add a head tag and within that we'll add a title tag and I'm just going to give it a title of my first React example.
[06:45 - 06:50] We'll also need a body tag so we'll get that added. And within the body tag we need two things.
[06:51 - 07:08] Some kind of element to render our React output into and a script tag that references our JavaScript entry point index.js which we'll create once we're done here. So the first one I'm going to add a main element and I'm going to give it an ID of output and that'll get filled in for us.
[07:09 - 07:25] Finally I'll add the script tag and we'll just give that a source reference of our index.js file. So what happens here is that when Parcel runs it looks in this first file for any script tags.
[07:26 - 07:37] When it finds the one pointing to index.js it looks in there to see what other JavaScript files it needs to impart and chain together to bundle up into our working app. With that done we'll save the file and move on to the main JavaScript entry point index.js.
[07:38 - 07:46] Inside our index.js files where the magic happens. It's the first place that we really set up our React app to load and inject it into our HTML page.
[07:47 - 07:52] This is going to be quite a small file where we're going to add our first piece of React code. We'll need to do a few things inside this file.
[07:53 - 08:04] We need to import React, import our main app component, the starting point for our React app and we need to use React DOM to render the app to the browser. First off we need to import our two React packages, React and React DOM.
[08:05 - 08:17] So let's do that now. So we'll import React from React and import React DOM from React DOM.
[08:18 - 08:23] It's important to know that whenever you're doing anything with React you must import it at the top of the file. This is what's known as bringing React into scope.
[08:24 - 08:33] For this we also need the React DOM package which is responsible for rendering our React code to the browser. Finally we'll import our app component which will look after greeting the user.
[08:34 - 08:40] This is import app from app. Now we have all of our imports.
[08:41 - 08:57] We need to make sure our app gets rendered to the browser and to do that we're going to call the render method from the React DOM package that we imported. So we're going to use React DOM.render.
[08:58 - 09:05] So React DOM render accepts two arguments. The component that you want to render so for us that's app and where you want to render it.
[09:06 - 09:16] So in this case the HTML element from the index.html file. To add our app component into the React DOM render we'll first get our first glimpse of the JSX.
[09:17 - 09:23] React's XML like syntax. So we'll do that with app like this.
[09:24 - 09:33] It looks very similar to XML or HTML and indeed later on we'll be adding the paragraph tags and input elements into our components but it's important to know the difference. What we're using here is JSX which is a proprietary React syntax.
[09:34 - 09:50] For the second part I'm just going to use the document.querySelector to find the main element in our index.html file. We gave it an ID of output so we'll use that here to find it.
[09:51 - 10:00] Save that file and that's what's done onto our app component. So notice the name of the file has been capitalized to app.js with a capital A.
[10:01 - 10:11] This isn't 100% necessary but it's a convention used among React projects to capitalize any component file names. So the last piece of the puzzle for us is to build out our app component to actually make something happen and greet our user.
[10:12 - 10:23] As we already discussed if we use React we need to have React in scope so we'll import React at the top of the file. So we'll import React from React.
[10:24 - 10:32] Now what I like to do when we've done this is just outline the basics of the component and then export that component so it can be used in another file or another component. That'll look like this.
[10:33 - 10:56] So we will have class, give the name of our components app and extends React. component to do, implement component contents and then we export default app.
[10:57 - 11:05] For now we've got to use the class based component which is an older less preferable means to build the components. However, you'll see some of them out in the wild so it's useful to take a look at how they're built.
[11:06 - 11:14] For future lessons we'll be using the more modern functional components with React hooks which look a bit different. For class based components we need to extend React component class.
[11:15 - 11:26] We can do that by typing the extends keyword after our class declaration and extending the React component class in there. To make this look a little neater we can import the component class as part of our React import at the top of the file.
[11:27 - 11:39] To do that we'll add it as a named import like so. By doing that we can replace the React component part with just component.
[11:40 - 11:48] Finally we want to add a default x-box this component at the bottom which is just app. We'll add this right after our class declaration.
[11:49 - 11:55] We're not quite finished yet but we've got the building blocks in there. If we run this now nothing would happen because our component doesn't return anything.
[11:56 - 12:03] So let's add a title. All React components have to provide some sort of return which is usually a block of JSX.
[12:04 - 12:13] With class based components we have to prefer to provide a render method and then add a return statement within that render method. To get us started and to test our app we'll just return a heading.
[12:14 - 12:41] Add a render method and a return statement within that they will add a simple message using a h1 tag. So we're going to add a render method and within that we'll add a return statement and we'll add a heading level 1 and just type welcome to the app just like that.
[12:42 - 12:45] Great right now the fun part. Let's head over to our terminal.
[12:46 - 12:55] I'm going to clear mine. Great so let's head over to our terminal and we'll type yarn start to start parcel building and running our app.
[12:56 - 13:18] What's going to find our index.html file, follow it up to our index.js file and we'll load our app component and then parcel will bundle our ladder, optimize it and start a local development server that runs at localhost port 1234 which we can visit now. So we'll see our lovely welcome message welcome to the app.
[13:19 - 13:36] Another nice thing about parcels local development servers is that it hot reloads responding to file changes on save and refreshing the browser. For example if I change this to welcome to the jungle and saved it you can see that it automatically hot reloads and updates it but we'll leave it as app for now.
[13:37 - 14:05] An important thing to note about JSX is that you can only return one top level element to highlight this if we add a paragraph tag under here and say this is a paragraph and give that a save we should see and that's the error message. So the error message states adjacent JSX elements must be wrapped in an encl osing tag did you want to use a fragment.
[14:06 - 14:20] So you can see we've got two adjacent top level elements returned in our return statements. To fix this we need to wrap them in a div and hit save.
[14:21 - 14:36] When the app reloads we'll see our message and a paragraph and importantly no error. However adding an additional div is fine for our example here but you might have situations where you don't want to add additional markup and output to the browser and wrapping everything in a div or some sort of other element won't be sufficient.
[14:37 - 14:57] For these cases you can use the react.fragments syntax. So we can replace our containing div element and either use react.fragments.
[14:58 - 15:12] But the shorthand version is to just have an opening and closing bracket corresponding opening /closing bracket like that. So we save that you can see exactly the same output.
[15:13 - 15:24] So react.fragments and the opening and closing bracket are functionally equivalent they do the same thing but the shorthand version just looks a little bit neater. React uses its own copy of the DOM called the virtual DOM.
[15:25 - 15:42] React handles changes to this internal copy of the DOM and the real DOM in the browser for us to keep everything in sync. Although JSX looks almost identical to the same HTML that we type into .html file it's important to understand that it doesn't map directly to the HTML and the eventual output to the browser.
[15:43 - 15:49] So it's time to expand our app by adding a dynamic message. In the first paragraph we're going to return our user's name once they've typed it in.
[15:50 - 16:10] To do that we need to modify our paragraph tag to include some executable code. So we'll edit that to say hi there, this.state.displainam.
[16:11 - 16:25] If you did this in HTML you'd just get a paragraph with the text hi there curly bracket this.state.displainam closing curly brace. But because we're using it in JSX anything between the curly braces will be interpreted as executable JavaScript by react and evaluated as such.
[16:26 - 16:38] And also notice a call to a class property called state here. Every component has access to its own state, its own local storage as it were where you can store values instead as well as retrieving them which is what we're doing here.
[16:39 - 16:50] So this line this.state.displainam we're really saying get me the display name value out of this component state. We haven't added any state yet so let's do that now.
[16:51 - 17:15] So within a class based component like this just like a regular JavaScript class you'll want to initialize any state values in the constructor. So we'll add a constructor here.
[17:16 - 17:33] This.state it equals an object and we'll type in our display name property and we'll just leave it as an empty string for now. So react automatically wires up our constructor when the component is created and passes it the properties object called props for short.
[17:34 - 17:41] You'll see this a lot when we're dealing with react. And the first thing we have to do in our constructor is call the parent class's constructor using the super method.
[17:42 - 17:51] The parent class is the component class here that we extended right the beginning. So we're using the super method and we're passing in the props object that's passed to this app component.
[17:52 - 18:03] To add our component state property we simply call this.state and set it to an object that contains whatever data you need. So for now we've just added the display name property and set it to an empty string.
[18:04 - 18:11] It's important thing to know about state is that it is immutable. This means that whilst it can be updated using react.setState method we never edit it directly.
[18:12 - 18:29] The only time we ever edit it directly is inside of a constructor function. You don't have to initialize state in the constructor as we're doing here but it's a good habit to get into so that anyone coming into the class can see what property should be expected to exist on the state object and what their value should be.
[18:30 - 18:43] So we've got a dynamic welcome message but we really need to allow the user to enter their name so we can greet them personally. Let's add some instructions and an input box.
[18:44 - 18:53] So we'll add an input element. We want to add a button.
[18:54 - 19:02] We set our input value to another state variable, this.state.name. By doing this we're turning it into what's known as a controlled component.
[19:03 - 19:19] HTML form elements like inputs and selects and text areas are typically uncontrolled components because they handle their own state and values. However by letting react handle our input's value from state it becomes a controlled component which means that react is responsible for maintaining the state of any value that we assign to it.
[19:20 - 19:30] Since we've added a new state value reference here let's update our component state in the constructor. So we'll add the name value and set that to an empty string.
[19:31 - 19:44] If we stop there however the value of the input will always be an empty string we need a way to update the value instead whenever someone updates the text. We do that by adding an event handler to the input element and the perfect place to do this will be as part of the on change event.
[19:45 - 19:57] This is where we have this on change event and we assign it to this.handle Change. So we add the on change event to the input and add a handle function handle Change which we'll define now.
[19:58 - 20:09] So we'll add the handleChange just before the render method. So we'll define handleChange.
[20:10 - 20:18] So the handle function is a simple arrow function that accepts one argument which is an event object that react automatically passes in for us. This is known as a synthetic event.
[20:19 - 20:27] We're calling it EVT here but you might see it called E or event. In this function we just need to update our state's name value with whatever text the user has entered.
[20:28 - 20:39] So to do that we'll use react built in state updating method, setState. We'll call setState and we pass it an object that contains any property value pair that we want to update instead.
[20:40 - 21:00] In our case we want to update the name property and we want to update it with the value of our text input which we can grab from the EVT argument using evt.target.value. In order for this to really be a true app we'll give our user some way of triggering a greeting message update once it updated their name in the input box.
[21:01 - 21:14] To do that we've added this button element just under our input element with a similar event handler but this time the event has been named on click instead of on change. We'll also give it some text update name.
[21:15 - 21:59] So we're going to add another event handler underneath our handle change and when this one we'll call handle click which matches the name that we've passed in to the on click event on the button. So within handle click it's very similar to on change and again it receives a synthetic event argument that we'll just call evt once more and you can see our click handler method is going to do something very similar so we're going to setState but this time we're going to pass in an object with the property of display name and the value is going to be set to this.state.name.
[22:00 - 22:13] The display name value here is going to be the same as the final value they've entered in our input box which we've already set to the state value name. That's what we'll use to update a state in our handle click method.
[22:14 - 22:33] So now that we've got everything we need let's save our app.js file and let's take a look in the browser. If we enter a name into the input box and click update name you can see that instead of high there and blank we get high there Rob Kendall.
[22:34 - 22:39] The very last part of our greeting app is just to add a little bit of spit and polish. Remember those styles we added right at the start.
[22:40 - 22:54] Now we're going to add them by imparting them just before the class component. Let me do that by typing import and then simply the reference to the file.
[22:55 - 23:05] Give that a save and we'll take a look in the browser. So you can see it's not an award winning app just yet but you can see it already looks a little bit nicer.
[23:06 - 23:15] And last but not least we have an initial message high there which is blank and then just nothing. We can make this a little more interesting by adding a default message we haven 't been introduced.
[23:16 - 23:30] So we'll do this by amending our initial greeting message using the logical or statement. It sounds quite fancy but it's really just a shorthand if statement in JavaScript that you'll see used a lot in React as a way to say evaluate both sides of the argument and return whichever is true.
[23:31 - 23:47] So we can do that here. Because our state value this.state.display name is empty which is falsey by default.
[23:48 - 23:53] React will look at this and return the right hand side of the argument our default string. And that's it.
[23:54 - 24:03] We'll give it a save and you can see it's updated. So we've got high there we haven't been introduced by name and update name.
[24:04 - 24:13] You can see that that default string gets replaced with what I've entered in the input box. In the next lesson we're going to refactor our app component into smaller components and introduce some React hooks.