Beginner's Guide to React useRef Hook
Learn about the useRef Hook, its use cases and implementation.
This lesson preview is part of the Beginner's Guide to Real World React course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Beginner's Guide to Real World React, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
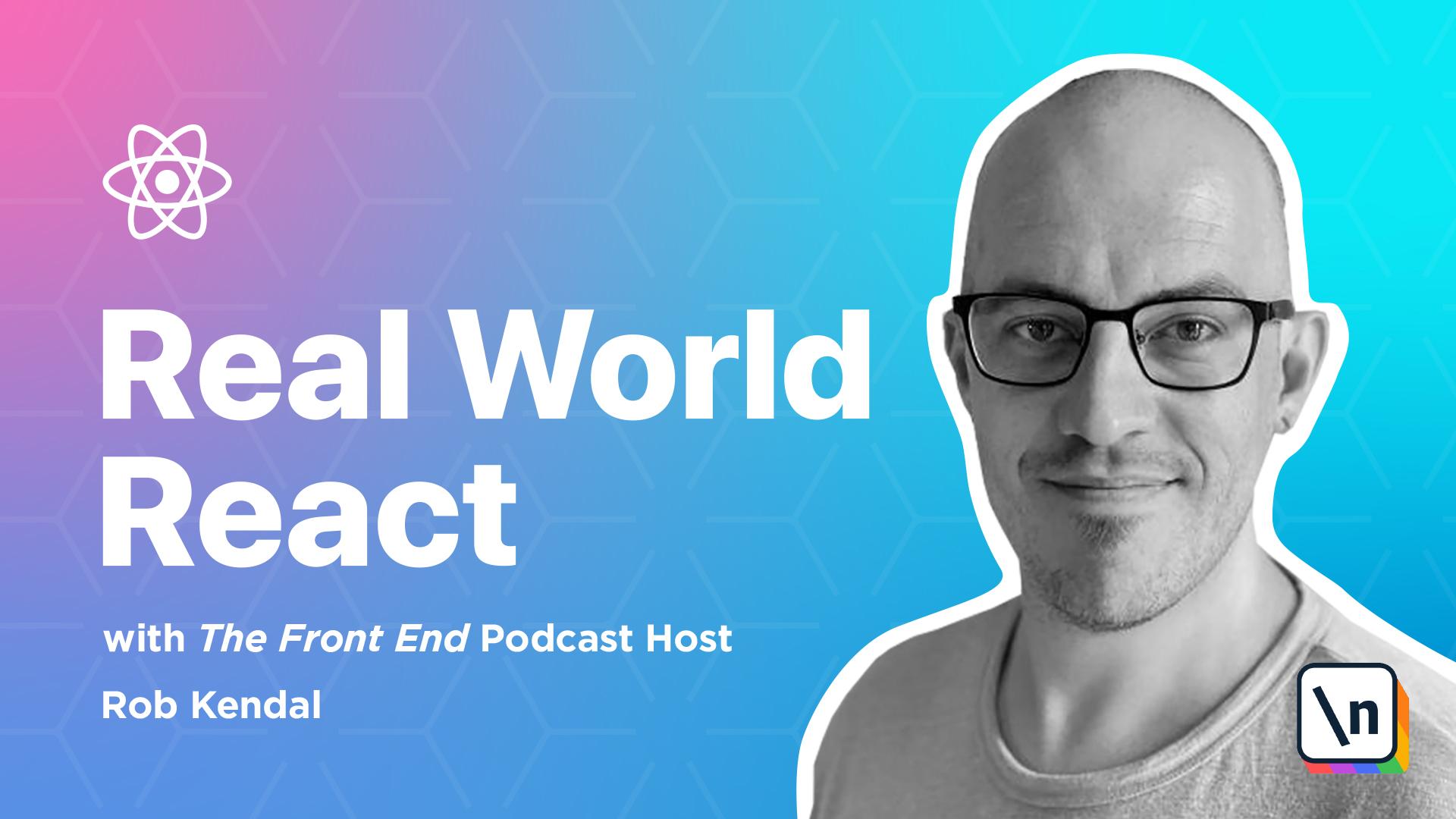
[00:00 - 00:11] Lesson 4 - The Use Ref Hook The Use Ref Hook provides us with a way to start and retrieve a mutable, that is, subject to change, reference object. It has a dot current property that can be initialized to a starting value.
[00:12 - 00:24] The object that's returned from the Use Ref will be persisted for the lifetime of the component, which is quite handy. From the official React Docs on Use Ref, essentially, Use Ref is like a box that can hold a mutable value in its dot current property.
[00:25 - 00:40] If you've come across React.createref function or seen those funny ref-style attributes about the place, especially in class components, then Use Ref Hook functions in a very similar way. One of the most common use cases for the Use Ref Hook is to attach a reference to the underlying DOM element that a ref is assigned to.
[00:41 - 00:54] For example, in our demo, in this lesson we're going to build a simple modal popup that when closed, returns focus to the corresponding input element in a form. We can use the Use Ref Hook to provide us with a way to access the input element in the React-generated DOM.
[00:55 - 01:02] With a reference to this element, we can access native properties and functions , such as the focus function. However, the Use Ref Hook isn't just for DOM refs.
[01:03 - 01:11] A ref object is a generic container with a mutable current property. This property can hold any value and is comparable to an instance property on a class.
[01:12 - 01:20] Using the Use Ref Hook couldn't be simpler. We call the Use Ref Hook passing in the value of null as the initial value, but this could be anything you want, of course.
[01:21 - 01:36] In the return function we'll define a standard HTML element and assign our input ref variable to the ref attribute. This essentially wires the underlying DOM input element to the Use Refs current property so that later on, in the handle something function, we can grab a reference to the input element.
[01:37 - 01:49] In our case we've gone with a common example of calling the focus function to bring the browser focus into the input element. Of course, if you want to change the value of the refs current property, you can do so as you would with any JavaScript assignment.
[01:50 - 02:03] e.g. input ref dot current equals and then whatever your value is. So, with our fundamental knowledge of the hook in place, let's build something to showcase it in action. For this demo we'll be creating a form in the modelled pop-up.
[02:04 - 02:16] Once the user clears the modelled we want to return focus to the correct area of the form, a specific input element. Back in our project in VS Code, open the styles.css file and add the following styles to the bottom.
[02:17 - 02:26] We already have some default styles form elements such as inputs. These styles are concerned with the modelled on-screen positioning and toggling its visibility.
[02:27 - 02:43] CSS's flex property gives us a super clean and simple way to position the element in the center of the screen, such as our modelled friend here. With our helper styles in place, open up the userefexample.jsx file and unsurprisingly we'll start by importing the Use Ref hook from React's library first.
[02:44 - 03:11] Next we're going to define a modelled box component that will house some dummy content to be displayed when we eventually click on the button to trigger a mod elled interview. It's a purely presentational component and receives two props, is open and handle modelled click.
[03:12 - 03:23] The isopen prop is used as a ternary if-stem into applying an isactive CSS class that will show or hide the modelled. The handle modelled click prop will be a function that we're just assigning to the modelled buttons.
[03:24 - 03:41] Ultimately our default parent component will handle the toggling of isopen prop value via the handle modelled click event, but for now our presentational mod elled box component doesn't need to know or care about this. When a user clicks either cancel or save the component simplifies handle mod elled click.
[03:42 - 04:03] Of course the modelled box component on its own isn't enough to do anything, so our default parent component is going to handle a few things for us. It will hold a modelled visibility toggle, a boolean value, it will create a ref object that will be tied to a form input element and it will handle togg ling of the modelled visibility via a click handling event that will be passed into the modelled box component.
[04:04 - 04:17] Underneath our modelled box component let's outline an empty scaffolded default export. And then first things first let's define a couple of variables.
[04:18 - 04:27] Notice our familiar use of the use state hook to define a modelled is open boolean value. We'll use this to keep track of the visibility status of the modelled.
[04:28 - 04:38] By default we want it to be closed so we'll initialize it to false. Under here we're creating a groups input ref variable that uses the use ref hook passing in null as we call it.
[04:39 - 04:53] We can assign this returned ref to the corresponding input element in a moment, but now we're just defining the ref and calling the hook to kick things off. Next we need a way to allow the modelled box component to effectively close itself or rather notifies that it needs to be closed.
[04:54 - 05:12] We'll do this by passing a function which we'll define now. The on modelled click function when called will accomplish two things.
[05:13 - 05:20] First it sets the modelled is open value back to false. This will then be passed back into the modelled box component which will re- render with the correct CSS class applied.
[05:21 - 05:35] Next it will call the focus function from the groups input ref variables current property. Remember the refs current property will be pointing to the underlying input element in the DOM, thus giving us access to its properties and values, focus being one of them.
[05:36 - 05:56] Next let's fill out the JSX inside the section element. We have a plain old form element here with some equally plain labels and inputs , note that in a real form we'd want to add a little more markup such as state syncing on change events and styling classes, maybe some accessibility attributes.
[05:57 - 06:03] We'd also want to handle the form submission. For example if you click the submit button now the form will submit to nowhere and the page will refresh.
[06:04 - 06:16] For now the main thing is to draw your attention to the select groups button which is wired up to an on click event. It's an inline anonymous error function that simply calls the set model is open state passing in a value of true.
[06:17 - 06:32] Underneath our form element let's add the modelled box component. We're passing in the modelled is open value and on modelled click function to the props is open and handle modelled click, as we've covered when we defined the modelled box component.
[06:33 - 06:38] And that's what's done with the code. Right, the best part, running the code.
[06:39 - 06:49] As with our previous example make sure to open up the app.js file and comment out all the other components in there except for the use ref example. Your app component should look like this.
[06:50 - 07:04] Update and save the file and then open up the terminal and run the trusty yarn start command viewing the result in the browser. So we have a simple form with a few fields, none of them are hooked up to anything so don't worry about what you put in there but the interesting thing is that you can't use the app.
[07:05 - 07:13] But the interesting part is that with the select groups button. Click on that and you'll see our modelled window appear in all its dimmed out glory.
[07:14 - 07:26] Again select whatever you want in the modelled, nothing's hooked up here either and then either click save or cancel from the modelled's footer. But when it closes notice how the input element under the groups label is highlighted and given focus.
[07:27 - 07:38] You might see this pattern a lot in enterprise applications or admin consoles where you open a new window, select multiple items and then get returned to the main form to continue editing. That's what we've achieved here.
[07:39 - 07:47] Once you've finished with the modelled the focus is automatically set back to the correct place in the form. This is all achieved via your friend of mine the use ref hook.
[07:48 - 07:58] Coming up in the next lesson we'll be exploring the use memo hook and I'll see you over there. over that.