How to Bulk Edit Data With React Query Builder
Using React Query Builder for a completely different purpose
This lesson preview is part of the Building Advanced Admin Reporting in React course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Building Advanced Admin Reporting in React, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
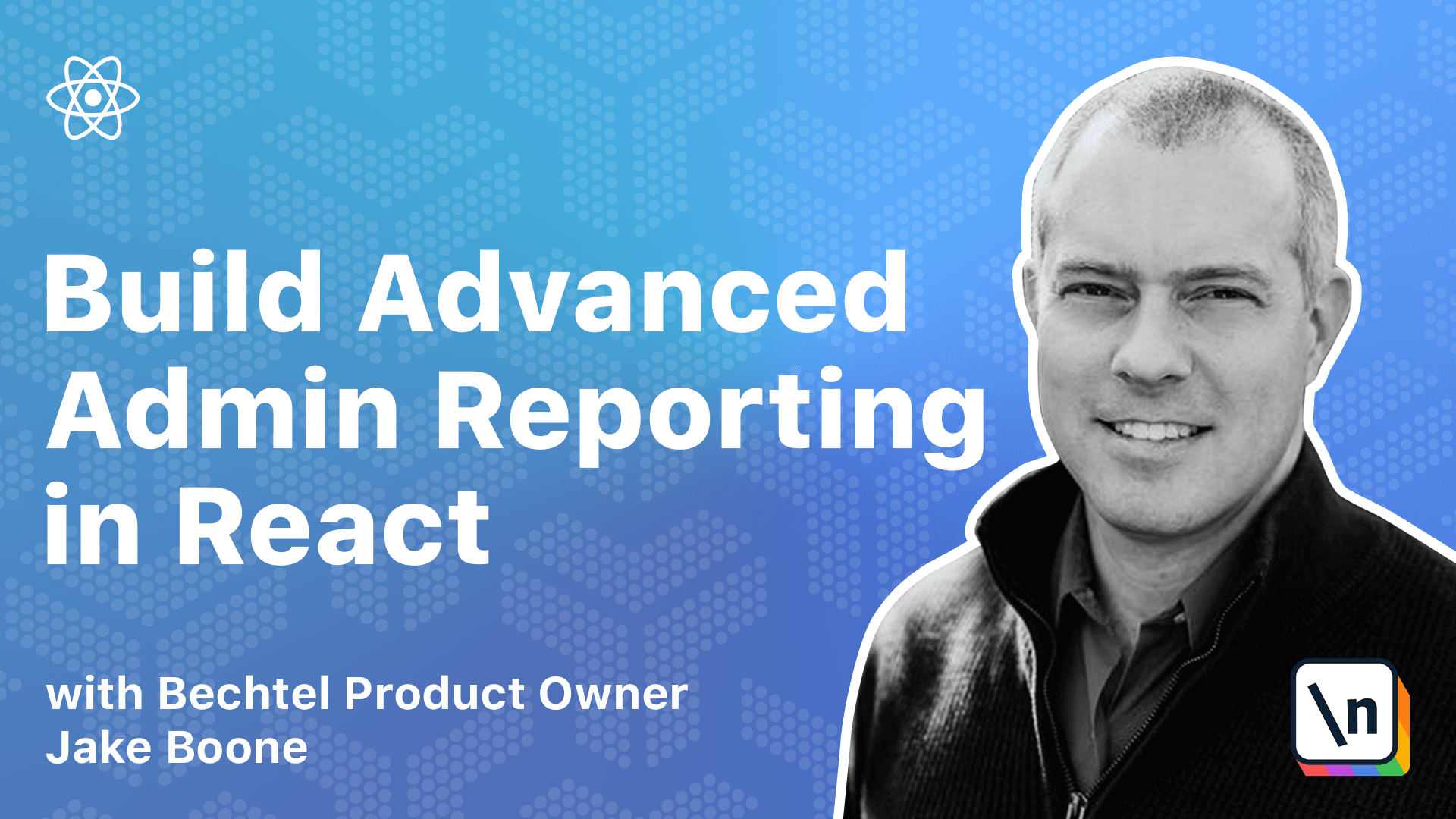
[00:00 - 00:12] Now we're going to use the query builder for a completely different purpose, bulk editing. This is useful when your data grid is updatable and you want to be able to define many changes at once.
[00:13 - 00:23] First we'll add a selection column to the data grid so users can determine exactly which rows they want to update. Make sure to set the row selection prop to multiple so we can select multiple rows.
[00:24 - 00:36] We'll also need to capture the grid API in a state variable for later use. So let's start out in app.tsx creating the state variable for the grid API.
[00:37 - 00:54] We'll call this grid API and the setter set grid API. That's going to equal use state of type grid API which we can get from a ggrid community.
[00:55 - 01:10] And we'll leave it as null for the default. Now down in our aggrid react component we want to add a selection column.
[01:11 - 01:26] So we want to leave the other columns alone. So what I'm going to do is wrap that in parentheses and then wrap that in square brackets and spread them.
[01:27 - 01:47] That'll leave them the same as they were before but we're going to stick another column here on the front. And it's going to have a field name of what is called this selection column.
[01:48 - 02:03] And then we want to set header checkbox selection to true and checkbox selection to true. And then so it doesn't take up too much room.
[02:04 - 02:18] We're going to set the width to 40. We also want to set the ongrid ready function or event.
[02:19 - 02:26] It's going to take a grid ready event parameter. So we'll call it GRE.
[02:27 - 02:46] And we're going to set the grid API to GRE.API. And remember we also need to set the rows selection prop equal to multiple so that we're able to select multiple rows.
[02:47 - 03:04] I'm going to format that real quick and save it. For the query builder configuration we can reuse the fields from our SQL generating query builder setup but we'll use different operators.
[03:05 - 03:15] The value editor component will also need to be different but we'll cover that in a subsequent lesson. Set up a new state variable to hold the update query and place a new query builder component just above the data grid.
[03:16 - 03:27] Place the button below the new query builder labeled update. So let's start out with our state variable to hold the update query.
[03:28 - 03:44] And what we can do is just copy the query state variable and setter. And we'll call this update query.
[03:45 - 04:03] Oops, what did I do? And set update query and we'll use the same defaults.
[04:04 - 04:27] And then down just above the data grid below our get data button we'll set up a new query builder. The fields are going to be the same as our SQL generating query builder.
[04:28 - 04:50] So fields data set. And our on query change prop is going to take the query and set update query to that parameter q.
[04:51 - 05:09] Our query prop is going to be our update query state variable that we declared just a minute ago. Now for get operators we want these operators to be different than the SQL generating query builder.
[05:10 - 05:32] So we're going to define a new function called get operators for update which we'll define in just a minute. Since we don't need the and or a combinator picker and we don't want the user to create groups of rules the query builder should not have combinator selector or ad group action components.
[05:33 - 05:54] So like we did before we'll just set those to return null. So control elements is going to be an object where ad group action is a function that just returns null.
[05:55 - 06:16] Copy that down and combinator selector is the same thing the function that returns null. Now just below the query builder we want to have a button labeled update.
[06:17 - 06:47] It's going to be of type button and it will have an on click handler of the function on click update which we'll define in just a minute. Now create a file called get operators for update.ts that exports a function called get operators for update.
[06:48 - 06:57] This method will return the operators we need to use for the bulk editor. The default operator will be set to which will set the field to the value provided.
[06:58 - 07:13] The numeric fields will also get increased by and decreased by which will respectively perform addition and subtraction on the field. Date fields will get the operator extend by number of days which will add days to the date in the field.
[07:14 - 07:43] Now I've already got this typed up so I'm just going to save this as get operators for update.ts. In the client source folder and then back in app.tsx we can import it.
[07:44 - 07:56] Now we have our get operators for update. The on click update function will loop through the rules provided and update the selected rows based on the rules.
[07:57 - 08:07] For now we'll only support the set to operator by setting everything equal to the value of the rule. We'll enhance this function in the next lesson to handle more operators and field types.
[08:08 - 08:29] So let's just above the return statement let's set up our on click update function. That's going to be a function that takes no parameters and what it's going to do is loop through the update query.rules property.
[08:30 - 08:52] I'm going to put this in parentheses you'll find out why in just a second. So update query.rules as rule type which we need to get from react query builder array .forEach.
[08:53 - 09:02] Let me see if I can import that. There we go.
[09:03 - 09:28] So for each rule now the reason we cast this as rule type array is because we know that the rules are not going to have any rule groups within them because we removed the add group button from the query builder. So for each are or each rule now we want to loop through the selected nodes in the grid.
[09:29 - 09:46] So we'll use the grid API for that. Grid API we need a question mark because it might not exist.getSelected nodes and that will return an array of the selected nodes.
[09:47 - 10:12] So .forEach in for node we're going to take that node and set the data value and this function takes in a field name and a value. So we get those from the rule r.field and r.value.
[10:13 - 10:27] And format that and save it and build the application again and check that everything is working as intended. So back in our terminal on the client folder we'll run npm run build.
[10:28 - 10:47] So when this is finished we should be able to see two query builders in our application. One to interact with the API and one to update the grid once the data is there.
[10:48 - 10:59] So this should take just a second to run. Okay, that's done.
[11:00 - 11:15] Let's go back up to our react application refresh and you can see the two query builders here and here. So let's get some data in.
[11:16 - 11:28] Let's try. Well, we'll just do order order ID is greater than zero to get everything.
[11:29 - 11:45] So we'll do get data and there we have our data. And now let's say we want to update the for some reason we want to update the country to United States for all of them.
[11:46 - 12:02] So I would do add a rule to our update query builder country set it to United States and hit update. Now it's not going to do anything because we don't have any items selected.
[12:03 - 12:08] So let's select all the items. Select update and there we go.
[12:09 - 12:12] Country is updated to United States for all of our selected records.