How to Fetch Data From a REST API With Python and Flask
Building an stock quote application that uses an external API
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
Tracking Stock Prices
You've now built your first Flask application! In this next section, we're going to build upon this to create a web application for more than just dice rolling which we will deploy to the internet. Over the next few chapters we are going to extend this application further to add more functionality and explore more parts of Flask.
What we're building
Some people like tracking the price of stocks, but if you go to many stock websites, there's a lot of clutter and the largest piece of information that people care about (the stock price) is not obvious. With our Python and Flask skills, we can make an application to solve that problem.
Setting up.
In our terminal, let's go back to our fullstack-flask folder and create a new folder for our app (which we can call stock-app
)
cd ~/fullstack-flask
mkdir stock-app
cd stock-app
Again, we're going to set up a virtual environment.
python3 -m venv env
source env/bin/activate
This time, to install Flask, we're going to create a file called requirements.txt where we list all of the dependencies of our project
touch requirements.txt
We're also going to use a Python library called requests
to interface with an external API to fetch the data we need about stocks.
Put these lines in your file requirements.txt
:
Flask
requests
Once we've set up this file, we can install the requirements using pip by running
pip install -r requirements.txt
Then we're going to create a server.py
to start out our Flask application.
touch server.py
code . # optional: to open the folder in VS Code
Within server.py, we're going to repeat the Flask imports to initialize our Flask application. This time in addition to importing Flask, we're going to import requests
, a Python library to make HTTP requests.
from flask import Flask
app = Flask(__name__)
We will also need a free API key from FinancialModelingPrep.com to get access to the raw data. You can register for your own API key for free at https://financialmodelingprep.com/developer/docs/.
warning
The free API provided by FinancialModelingPrep.com requires an API key for usage. When we pass the URL parameters of apikey=demo
, the API only works for Apple's stock.
This lesson preview is part of the Fullstack Flask: Build a Complete SaaS App with Flask course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack Flask: Build a Complete SaaS App with Flask with a single-time purchase.
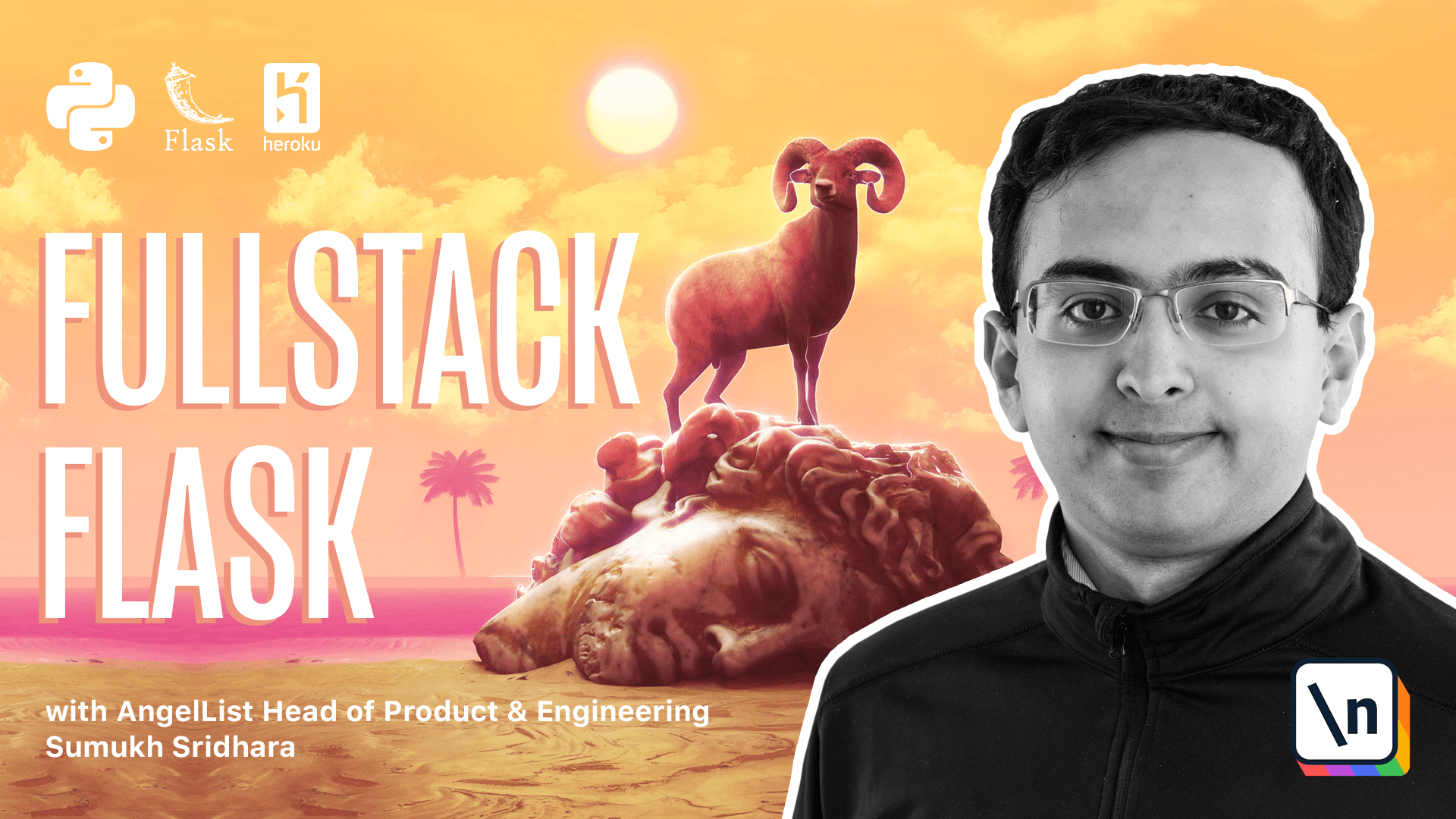