Creating an Efficient Data Cache
Here we'll learn how to create an efficient data cache
This lesson preview is part of the React Data Fetching: Beyond the Basics course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to React Data Fetching: Beyond the Basics, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
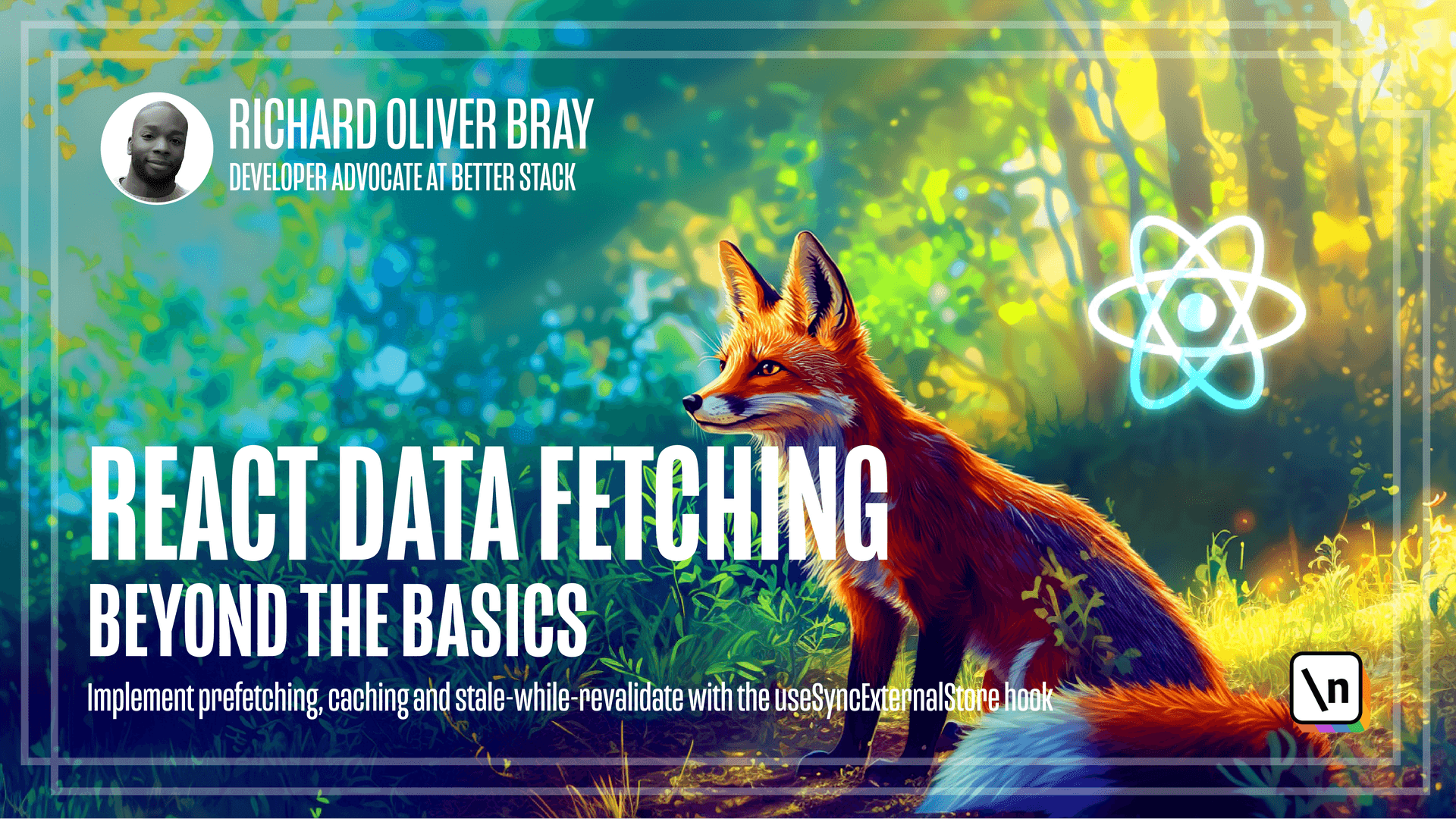
[00:00 - 00:17] In this lesson, we're going to look at how we can create an efficient data cache, but let's first go through why we need a cache in the first place. In the last lesson, we implemented some basic prefetching to get all the Pokemon data and load it into the home component.
[00:18 - 00:27] But when we revisited that component, no data showed. This is because the data we previously fetched and put in a store wasn't being loaded.
[00:28 - 00:41] To fix this, we need to implement a cache for the data. This involves giving each saved piece of data a key that we can use to check if the data already exists before retrieving it.
[00:42 - 00:50] That may not make sense now, but it will make more sense later on. Okay, let's go ahead and implement this in code.
[00:51 - 01:06] Let's first replace our store array on line 3 with a map which will call data cache. So let's get rid of the code on line 3 and write constant data cache and we'll make this equal a new map and add parentheses.
[01:07 - 01:21] Maps in JavaScript are much more performant than objects when lots of data is being stored. And they also provide useful methods like get, set and has which we'll use to make our code easier to read.
[01:22 - 01:39] Let's change the code in our prefetch data function to make use of our data cache. Our first double click on the word data cache on line 3 and then delete all the code on line 13, paste data cache in place and write docs sets, add parentheses and here we're going to give it two values.
[01:40 - 01:45] First the key and then the data as the value. Maps are a bit like objects.
[01:46 - 01:53] They can store key value pairs, but the keys can be anything. So they can be strings, numbers, objects, you name it.
[01:54 - 02:03] But for now we're keeping our keys as strings. We're making use of the key value on line 13, but we don't have a key argument inside our prefetch data.
[02:04 - 02:11] So let's go ahead and add that before the FN arguments will write key and add a comma. We'll also need to pass the key in our use data hook.
[02:12 - 02:22] So inside the parentheses on line 5, let's write key. And on line 7, let's update our event listener to get data from our cache instead of the store.
[02:23 - 02:39] So inside the parentheses, we're going to delete store and write data cache.get add parentheses and we're going to put in our key. So what this is doing here on line 7 is basically getting the data from the cache that matches the key that's passed into the use data hook.
[02:40 - 02:54] So when the events gets dispatched on line 14, there should be data inside our map on line 7 to add to our set data hook. This also means that if data already exists in our cache, we can check this with the key.
[02:55 - 03:06] So if our key already exists in our data cache, that means it must have data and we can return that instead of fetching data again. We can do that with an if statement.
[03:07 - 03:28] So at the end of line 6, hit enter and write if add parentheses and write data cache dot has add parentheses and inside the parentheses will give it a value of key. If it does have the key, then we can return the data cache dot get our parentheses and inside the parentheses, we're going to add key.
[03:29 - 03:34] So if the key exists, then return the data that's inside the cache that has that key. Cool.
[03:35 - 03:45] I know I've put this code on one line on line 7, but if you want to wrap the if statement around clearly braces, you're more than welcome to do so. In fact, let's go ahead and do that.
[03:46 - 03:57] I'm going to select all the code from return onwards and I'm going to wrap them in clearly braces and press enter on line 7 and also at the end of line 8 to format the code a bit. Nice.
[03:58 - 04:12] Now, before we test this in the browser, let's update our home component and the main.jsx file. So, on line 9, where we call our use data hook, we can give it a key which would be a string of per commands.
[04:13 - 04:24] But of course, you can make the key whatever you want. While we're here, we can get rid of the console log on line 10 and also on line 11, since they've served their purpose.
[04:25 - 04:33] And at the top of this file, we can get rid of the input on line 1 and on line 2. Now let's go to our main.jsx file.
[04:34 - 04:47] And over here, let's update our prefetch data function and give it a key. So, before we get a Pokemon function, let's add a string of per commands and add a comma to separate the arguments.
[04:48 - 04:52] Nice. Now let's go ahead and test this in the browser.
[04:53 - 05:06] Okay, so if we refresh the page, we can see that Pokemon data is being fetched. And if we click on view details and then click on the back button, we can see data isn't being fetched again because it's already in our cache.
[05:07 - 05:29] So no matter how often we click on the back button, it doesn't refetch the data , which is the complete opposite of what's happening with our details component, which seems to be fetching data each time we visit it. In the next lesson, let's go through how to fetch data on an event like hover, submit, click, scroll, etc.
[05:30 - 05:36] And hopefully, by doing that, we'll also avoid the issue of making multiple calls when visiting the same page.