Loading and Error Handling
We'll learn how to handle loading and error states in our application
This lesson preview is part of the React Data Fetching: Beyond the Basics course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to React Data Fetching: Beyond the Basics, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
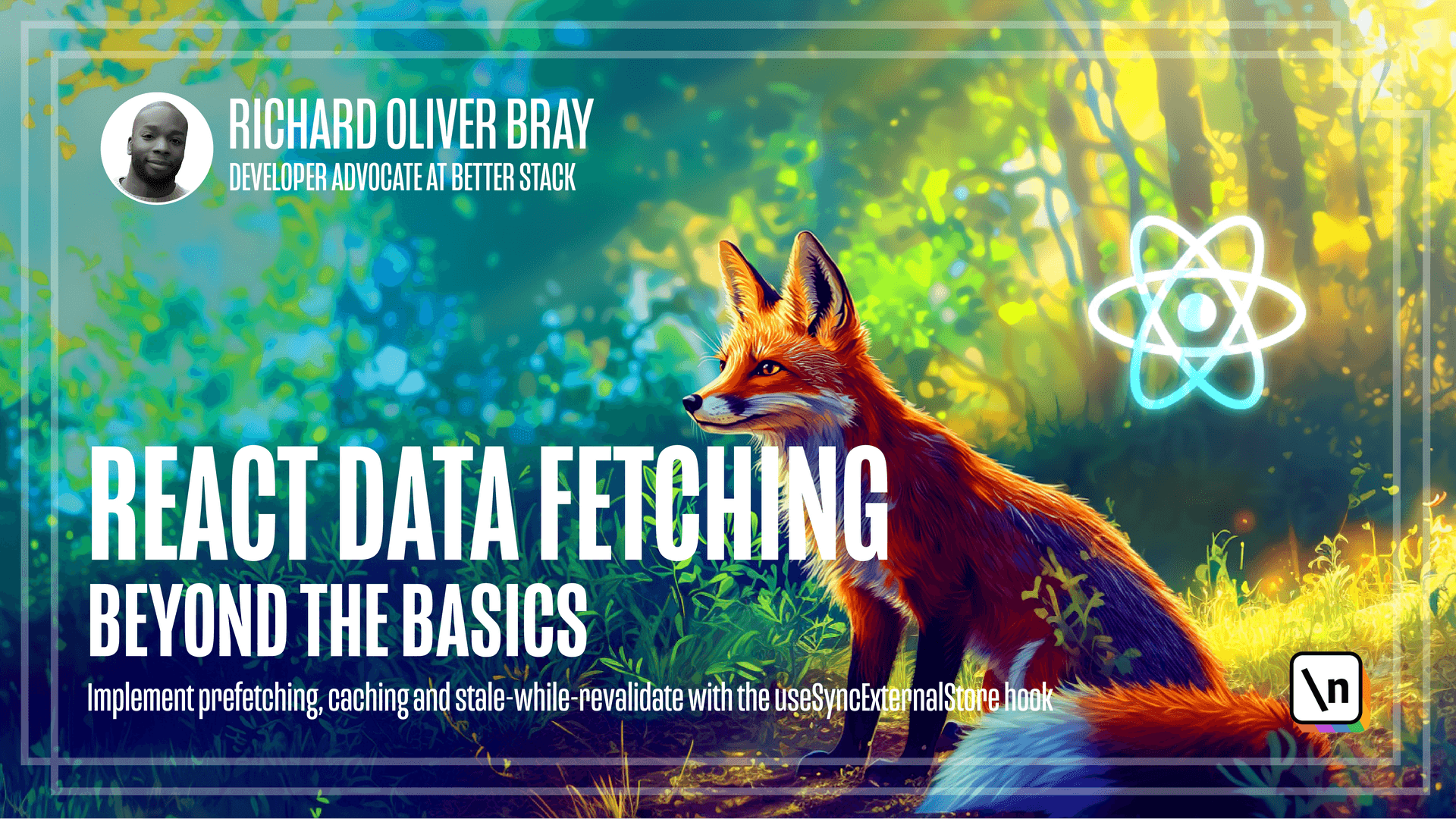
[00:00 - 00:09] In the last lesson, we went through how to fetch data on an event. But right now, we are manually handling the loading states and we're not even handling errors.
[00:10 - 00:19] Wouldn't it be nice if we could let the hook handle the loading and error states for us? Well, that's what we're going to do in this lesson.
[00:20 - 00:32] The best way to do this is to assign a status or a state to the data depending on what's happening. So, if it's loading, we can set the state to loading and if it's error then we can set the state to error.
[00:33 - 00:48] We can manage these states with something like a switch statement or a JavaScript object, which will allow us to manage the states of data and return the correct state to the client. Cool! Let's go into the code and start to implement this.
[00:49 - 01:06] Let's first create a function below our prefect on event function called format data response. Let's press enter a few times after line 26 to give us some space and then on line 28, we're going to write function, format, data, response.
[01:07 - 01:13] We'll add parentheses and then curly braces. This is going to create the object that will contain the loading and error states.
[01:14 - 01:27] So what we're going to do is give each value in our data cache an object with a status value. This means each item will be an object that contains a status value and data which we'll call payload.
[01:28 - 01:52] Because of that, we can destructure the status and payload values as arguments in our format data response function. Then we can create an object which we're going to make a const called status response and this is going to return the correct object based on the status. It may sound a bit confusing now but once I write the code it will make much more sense.
[01:53 - 02:09] So if we have a status of loading then we want to return an object that's going to make is loading. True! Let's add another property. So after line 30 we'll hit enter and add a new property of error.
[02:10 - 02:44] This is going to have a value of error and this will return the error as a payload. And finally we're going to have one more. We'll actually duplicate the last line to do that and let's change error over here on line 32 to success. And then we can change the error here as well to data. Cool! And beneath that, so after our status response object we can return the status response that matches the status that is passed in as an argument.
[02:45 - 03:34] Cool! Now because we want to be able to de-structure the object for our used data hook we'll need to pass default values to other properties. For example, the loading status here on line 30 will also need to have a value for error and data and these can both be null but they have to have a value. So we can do this by creating a new variable which we're going to call default data and this is going to equal an object with all the default values. So is loading will we false error will be null and data will also be null. Nice! Now on line 31 32 and 33 we can actually spread the default data object so that only the value that we want to change will be changed.
[03:35 - 04:28] Let's copy the default data variable and here all we have to do is write a spread operator, paste a variable and add a comma. So what's going on on this line line 31 is that default data is spread out and only the is loading value here is changed from false to true. And we can do the same on line 32 as well. So here after the error and after the opening value brace we can do a spread, paste and add a comma as well. Similarly on line 31 before the word data we can do a spread, paste and add a comma as well. Cool! Now that we have this we can update our pre-veg data function to add the status and payload data to the cache. So let's scroll up in this file until we get to line 18 and over here we're going to change data to an object that will contain a status.
[04:29 - 04:42] In this case we want this to be success and you want to give it a payload of just data. And now that we have a status we also have a way to handle errors in the code.
[04:43 - 05:13] So while we're here we can add a catch block on line 20 so at the end of the parentheses we'll write catch, add parentheses and then inside these parentheses we're going to add another set of parentheses with an argument of error. Then we're going to add an error function, add curly braces and then hit enter. So what we want to do here when there's an error is the same as a success but we want to return the error in the payload. So let's copy all of line 18 and line 19 and we'll paste it on line 21.
[05:14 - 05:28] Then where it says success will change to error and where it says data will also change that to error. And while we're here we can add a console error just to tell the developer that there's been an error.
[05:29 - 06:23] So here I'm going to add some backtext and say error prefetching data for key and then add a dollar sign and curly braces and here we'll put the key in with a colon and add a comma then we'll paste an error message. Sweet! I've noticed I was spelled error incorrectly on line 21 so let's go ahead and change that. We'll select the text and write error. Now you can see that on line 19 and also on line 23 we've got the dispatch code twice. We can avoid this duplication by using the finally block. So I'm going to press command X to cut this code and at the end of line 23 I'm going to write finally add parentheses and inside these parentheses I'm going to add another parentheses with an error function and this is going to have curly braces and I'm going to paste in the dispatch event. This also means we can delete the code on line 22. Nice! This is looking good.
[06:24 - 07:25] Now let's go ahead and update the code in our use data hook to make use of our format data response function. Let's quickly talk about that. So when we first call the use data hook if there's no data inside the cache we want to add a status of loading to the cache with an empty payload or a payload that has a value of null. This means that the loading object is returned to the user or client. Then when the data is fetched we want to update the status to success or error based on what's happened and add the relevant data to the payload and this will update the correct properties in the client. Cool! Let's go back to the code. Okay, at the top of our use data hook let's add an if statement to check if the key argument is in the cache. So at the end of line five I'm going to press enter right to if add parentheses and I'm going to add a space between the if and the opening parentheses and inside the parentheses I'm going to add an exclamation mark right data cache.
[07:26 - 07:48] .has add parentheses and here I'm going to write key then if it doesn't have the key inside the cache what we're going to do is set the cache or write data cache. sets and here we'll set the key to have an object of status which will be loading and it will have a payload of null.
[07:49 - 09:16] Cool! We can also change the default value of use date on line nine from null to something like data cache.get add parentheses and then write key. Since we're setting the payload to null on line seven then a key should always have a value so it makes sense to always fetch the data from the cache. Now beneath that we can change our if statement to only return data if the cache doesn't have a status of loading. So on line 11 we're going to change data cache has two data cache.get and we'll get the status and make sure it doesn't equal the string of loading which means it will be success or error. If that's the case then data has been added to the cache properly and we can just return that data but we want to return the formatted object. So let's highlight this code on line 12 and change it to format data response and we're going to add parentheses and give it an argument of data. Now there's one last thing to do in this file and that's on line 16. So beneath our event listener since we don't want to return the data from the cache we want to format it first let's change data to use our formatted data response function. So we 're going to write format data response and add parentheses and we'll give it an argument of data.
[09:17 - 10:24] Nice and that should be it. I know this code is getting quite complicated so let's have a quick recap of what we've done before we move on. If we scroll down you can see we've added a new function called format data response. What this is going to do is get the status and payload and return an object based on that status. We need to have default data since all we're doing is changing one property. So for loading we're just changing the is loading property for error we're changing the error and for success we're just changing data. Now if we scroll up we can see that we've changed what we're adding to the cache. So before we were just adding the data but now we're adding an object that contains a status and payload. So if this call was successful then the data cache will have a key of the key that's passed in so percumin or percumin details and the value will be an object with a status or data. So whenever we get a value from the cache it's going to be an object that contains status or payload.
[10:25 - 13:07] I said data but I meant payload and what we're going to do here with that status is we're going to format it with a function we wrote down here and based on the status we're going to return an object to the user. Nice let's go ahead and make use of this object in our relevant components. We'll first go to our home component. So here on line eight let's change the word percumin to an object that's destructured so we'll delete this word and add curly braces then we're going to write data and we'll change that value of data to pog c'mons and then we're going to have is loading and we'll also have error and now that we have our own is loading property what we can do is replace this if statement on line 10 to make use of that instead of pogmons so delete this code in the parentheses and paste in art is loading variable we can even add another if statement below that to check if there's an error so we'll write if add parentheses and then write error to make use of our error variable and then add curly braces and what we can do here as we can just return a div that will contain the error and also the error message from the catch method very cool. Now if we go to the browser and do a hard refresh so that will be shift command r or shift control r if you're on the windows we can see very briefly that the text loading appears this means that our loading status is working but what about our error status well let's go back to the code and update it to try and test that inside our api pokemon.js file let's update the get pokemon's function by selecting and commenting out line 17 and 18 then at the end of line 16 hit enter and what we're going to do is have a promise rejection so we're going to write return promise dot reject add parentheses and then we're going to write new error add parentheses and we're going to type something in here so we can write something has gone wrong cool now let's go back to the browser and we can see that our error state is being handled by the hook nice this definitely wasn't happening before all we have to do now is update the details component to make use of these new values but since it's not difficult to do i'm going to leave that for you to do after this lesson okay and this marks the end of the second module in the next lesson we're going to have a brief recap of what we've done before we move on