This lesson preview is part of the Rust For JavaScript Developers course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Rust For JavaScript Developers, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
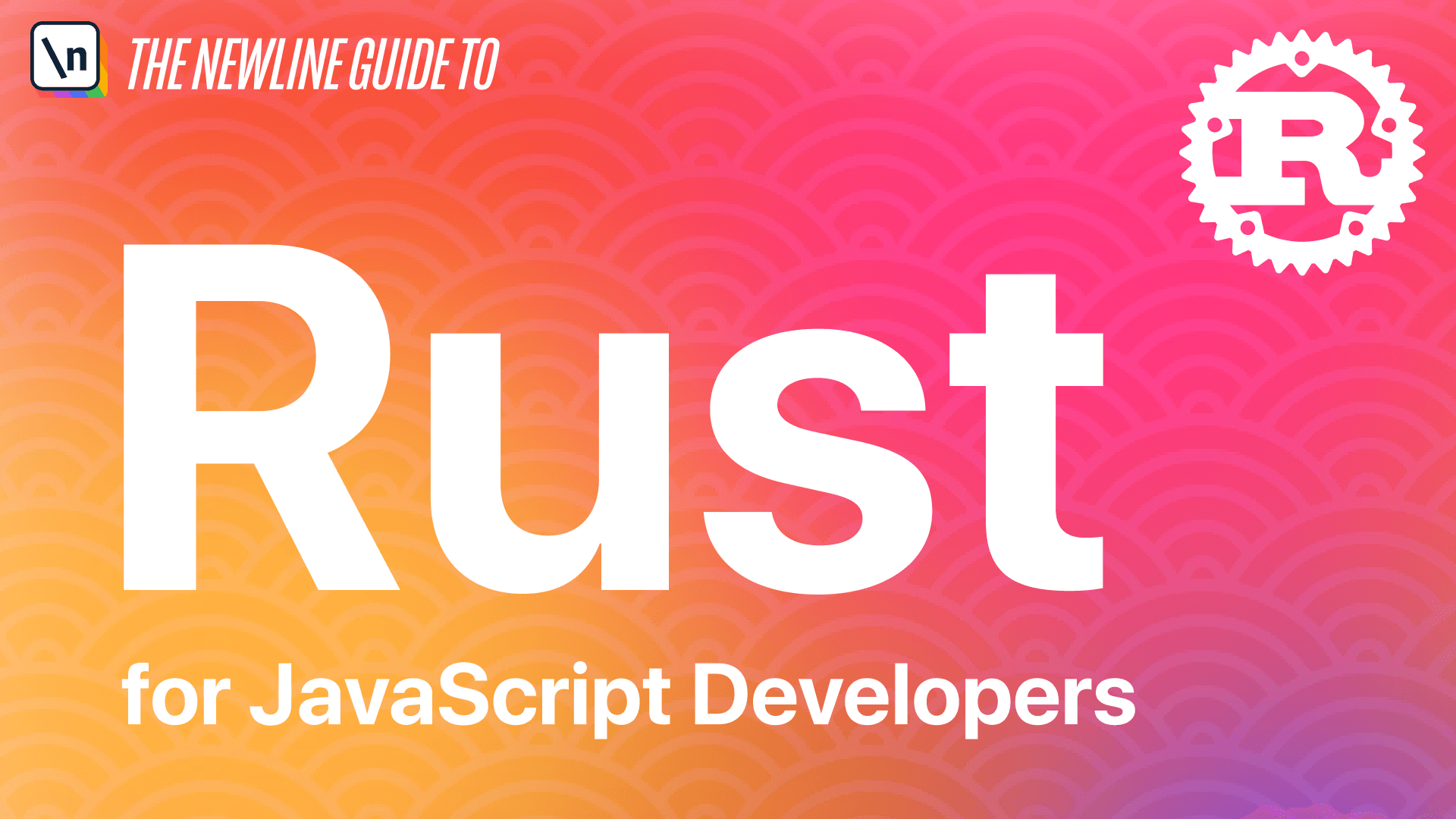
[00:00 - 01:24] In this module, we'll work through the process of building two command line utilities and rust. It's important to reiterate at this point that this course aims to be a very practical introduction to rust and doesn't aim to cover intro to programming. As assumed, you have a grasp on general programming constructs like conditional logic, control flow, various collection types, and some functional and object-oriented concepts, so I won't spend a lot of time explaining those as they come up. I will make sure to highlight the particular zone you need rust syntax or unique rust concepts as we uncover them through building programs in the lessons to come. So the first program we'll build is a clone of the WC utility. WC stands for Word Count and is a basic Unix utility that counts the words in a given file. We'll call our clone of WC Mini WC and we'll implement a subset of functionality that exists in the original WC program. So our Mini WC program will, one, be an executable that we can run from the command line, two, it'll take a file name as an argument via the command line, and three, it will return the total number of words that exists in that document. And for the purposes of Mini WC words will be defined as any string of characters that is separated by a space. So you won't worry for now about dealing with multiple files or what to do with files that don't contain words. The first thing we'll have to do is create a new rust project. And so we'll use the command cargo and the new and we'll call it Mini WC.
[01:25 - 01:38] And we'll change into there and open our code editor. So by default, we get our print Hello World. And we're ready to get started.
[01:39 - 03:44] So at this point, we have a default executable that we can run. And we can just double check on that by showing cargo run, run. And we can see the prints out there. So the next step is that we need to figure out how to accept arguments from the command line. So we can allow users to supply a file name to the program. And so to do that, we can use the Rust standard library, specifically the standard ENV module. So in JavaScript, we don't really have a standard library. We have core functionality, like number and math. And then we also have runtime dependent global utilities, like process and node. And then the web API is like window.fetch in the browser. Rust on the other hand, has a core set of primitives and syntax that are available everywhere, which is generally called rust core. And additionally, Rust exposes a standard library that's available by default in a rust project. So you can access any of the standard library models and rust via the STD namespace. And so we can see that here, STD, and then colon colon is rust syntax for going deeper into a nested path, a module path in this case. So on standard, we have a bunch of different utilities. And then on there, we have ENV. And within ENV, we have this ARGS function. And so ARGS operates very similarly to the process.argv in the node ecosystem, meaning that it just takes the arguments that are supplied to the program, when you run it from the command line. And then that's what the output of this function is. So we can test that by changing our format string here, and putting our standard ENV ARGS function. So there's a small caveat here. And that's that the default format string here, string here that has the curly braces.
[03:45 - 04:05] By default, will not show the output from ARGS. So specifically, ARGS returns this ARGS type. And you can see that in the denoted by the single line, AR, the hyphen carrot syntax, which is the return syntax and rust.
[04:06 - 04:48] And by default, these two curly braces can't display that type. So we have to change this to the debug format string type. And this will be able to show a lot more types and rust that you typically wouldn't want to display unless you're doing a some debugging. And so now we can save this and run. And we should see our output. So we can see that there's this ARGS thing with the inner. And then we have what looks to be like an array with target debug mini WC.
[04:49 - 05:34] And so by default, and this holds true for node dot, or processed ARG via node, the first argument in any program will always be the path to that program. So the name of the program in WC, and that exists in the target debug mini WC right here. So if we supply it with an actual argument, and so we can separate cargo run and hyphen hyphen, and then we can put supply some arguments to our actual our program mini WC. And so then we can do like ARG 1, ARG 2. Now we can see that both of those were printed or were added to that array.
[05:35 - 05:57] Cool. So using the fully qualified name like this is is possible. We can do that, no problem. But to make things a little bit nicer, we can also shorten that up a little bit by using the standard ENV module. And so use will just bring a certain path into scope.
[05:58 - 06:58] And so in this case, we want to bring standard ENV into scope. And so now instead of having to prefix everything with the standard colon colon syntax, we just have ENV readily accessible to us . We could also do this and pull ARG's in itself. And then we don't even have to have the ENV colon colon. But typically it's a little bit more idiomatic to if you're pulling in code from some external source, that you at least have the parent module that it's coming from. Makes it a little bit clearer to see, especially once you have more lines of code in your file, to see, okay, that this ARG's function is not something that I defined in my program, or that's defined in this program. Rather, it's defined in this ENV module, which we can see that we're pulling in at the top here. So this ENV ARG's function returns a value that we can iter ate over using a for loop. And so for loops in Rust are very similar to for loops in JavaScript.
[06:59 - 08:03] The syntax is very similar, as we'll see in a moment. And so we can, instead of printing out all of the ARG's like this, we can go ahead and print them out one at a time by nesting them inside of a for loop. So we can say for ARG in ENV and then ARG's. And then open up our brackets here, remove this and just go back to our original curly brackets. And so for ARG and ENV ARG's, let's print out ARG. And so since that value inside of, since this ARG's function returns something that we can iterate over using this for loop, that internal value here is actually something that's a string. And so it's something that's displayed more easily or more readily. And so we don't have to use a debug format string here. And so running this, we should see our ARG's printed out.
[08:04 - 08:23] And there we go. So we have the first one, which is that default path to the executable, and then ARG1 and ARG2. So in the next section, we'll figure out how to handle the arguments that have been passed in and actually tell our program what to do.