Fetching and Displaying Data
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Mastering RxJS: A Compact Journey from Beginner to Pro course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Mastering RxJS: A Compact Journey from Beginner to Pro, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
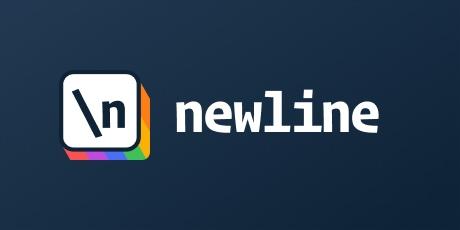
Welcome back. So in the previous lesson, we have created the product service, which does an HTTP call to our fake backend and gets a list of products. Now, we want to display that list in the UI. Let me start the app again. So, we want to get this list in here. But before we do that, we need to understand a few concepts. The first one is thinking in streams. So what is a stream? The way I like to think about streams is the same way I think about time. Let's represent the time as an arrow. And each second represents a data point like this. Whenever a second passes, a new data point is being made available, just like that. But, where does the data come from? The way I like to think about it is let's say there is a data pool somewhere. So if we think about space and time, actually, what's on the left side, let's say this everything is beyond the observable universe. In other words, we don't care what's on the left side. We only care what's on the right side, meaning that we don't care where our data comes from. We only need to think about the stream of data. So a stream is a sequence of data elements made available over time. So whenever a second passes, we'll get a new data element, just like this. So how do we interact with a stream? RxJS has a structure called Observable. In order to get data from this stream, we need to work with an Observable. And to get the data, we need to, let me visualize it this way. This is the same flow of time. And, let's say I want to get data from this stream. To do that, I need to subscribe at a certain point in time. And when I subscribe to this stream, I start getting this data points in here as well, just like this. And if I want to stop getting data, I will need to unsubscribe at some point in time. Now that we've visualized it, let's see how it works in code. First we need to get rid of all of this in here. Let me stop this as well. OK, now I want to create a product list component. Let's add a container. And inside this container, let's add our product list component that we have just created and it's complaining because we didn't add the product list component in here already. We've added it inside our product list component. Let's create an input product. Then the product that we have created. OK, that will be it. What are we missing? We need to do our HTTP call in here. Let's create our product service. OK, and we also need to add ngOnInit implements OnInit and inside ngOnInit we want to make a call to this observable. Now we want to pass the data to our product list. Let's go into our product list. Oh, I forgot to pass it in here. And let me paste that in here. I'm missing the common module. OK, there. I think we're set up. How do we get the product list from the products observable into this products list? As you remember, I told you about subscribing to an Observable. What we will get from there is the data that we want to use. Now we can actually assign it to our products. If we look carefully in here, fetchProducts has an Observable, you can view it as a wrapper around product list. Let me add a type to make it clearer. There we have it. Let's run it and see what we get. Let me start it in here as well. OK, I forgot to add HttpClientModule in here for it to work. OK, so now let me start it and nothing happens. OK, I figured out why nothing happens, it's because I forgot to set the port where it should start at. I will set it to 5000. And in here I'll set it to 5000 as well. What I did here is in order to make the debugger work correctly, we need to set up the port. So from here, you can see the command, which is running, which is npm start. And when we look at here, the start command will run ng serve on port 5000. This way we'll get a consistent debugging session. Let me restart it. Let's start the debugger. So it looks like I forgot to add some imports in here. It is complaining about this product service. And from the looks of it, I forgot to add it into the provider survey. There we have it. And here we go. We get our first list in here. If we look at the network tab, refresh, let's enable preserve logging in here and in here. This way, whenever we refresh the page, we still preserve the previous request as well. Otherwise, if we don't have it, our networking tab will be cleared. So we can see we have successfully gotten the data from our observable. Fortunately, that's not it, because, let me remove that, because whenever we create a subscription, it gets stored in memory. So if we navigate away from the page, the subscription will still live in memory. Now, let's assume we have about 1000 or 10,000 subscriptions. The browser will start using a lot of memory. That's why we need to unsubscribe when we are done working with it. In order to unsubscribe, we need to implement OnDestroy those two are Angular component lifecycles. You can search them, if you want to know more about it. First, let's create a subscription. Let's put it in here. Let's assign it. And now in ngOnDestroy. Let's unsubscribe from it. Now we are safe. We don't have any more memory leaks and we are good to go. To summarize what we have done so far is we are doing an HTTP call, to get a list of products and then we are displaying these products, we are subscribing to an Observable. And then when we don't need it anymore, we are unsubscribing from it.