Getting Started
In this lesson, we'll begin installing the testing libraries we are to use on the client application.
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
Lesson Transcript
[00:00 - 00:15] So, in this lesson, we'll begin by just making sure we have all the libraries we need in our tests. And we may just do a quick check to create a simple test file and write some dummy tests to make sure our runner is working and everything is set up.
[00:16 - 00:35] If we head over to our package JSON file and just to mention, this is the client project from the latest point of our tiny house course. So assuming you've managed to go through the course, you've set up everything you needed to do, at this very moment in time, this is where we're picking up the testing module or the work we're going to do with testing.
[00:36 - 00:49] If we take a look at the package JSON file, we'll notice that there's already some type definitions installed for Jest. And this comes installed with the Create React app utility that we used to create our React application.
[00:50 - 00:59] Create React app uses Jest as its test runner. And they've set up all the necessary tooling that we may need such that there isn't really any other changes we'll need to do.
[01:00 - 01:25] Some of the cool things that already exist is the file name conventions that the test runner just will look for to actually run tests. If we create a file with the .js suffix in an underscore underscore test folder , or if we have files with the .test.js suffix or a file with the .spec.js suffix, if we were to run the command to run our tests, the runner will look through all these files to actually execute those tests.
[01:26 - 01:36] Notice that this .js suffix refers to the JavaScript portion. Similarly for us, we can have the TypeScript convention as well, .test.ts, etc.
[01:37 - 01:49] And from the package JSON file, we'll notice in our scripts, there is already a test command. This test command utilizes the React Script library that has Jest and all the tooling set up to run a test command.
[01:50 - 01:57] What does this NPM run test script do? Test would launch in Watch mode by doing so.
[01:58 - 02:09] Every single time we save a file, it would rerun the test to pick up the changes that were made to the tests to then rerun those tests again. As of this moment, we have no test set up, but we can still run this script.
[02:10 - 02:32] So if we head to our command line and if I was to run NPM run tests, don't tell me that it's running React Script test and it's smart enough to know that no tests were found related to files changed since the last commit. If I was interested to just run every test that might exist, I'd just press A to run all tests and it knows that there's no tests that have been found.
[02:33 - 02:43] And it shows that it's still in Watch mode. So I'm able to then go back and have it just ready for me to pick up any changes to any new tests that I add.
[02:44 - 02:55] So let's now go ahead and try to create a test file. If you head over to our sections folders, we'll see how we've neatly separated all the different sections or all the different high level components in our app.
[02:56 - 03:07] Assume we wanted to begin with the home components and we wanted to create tests just for this component alone. I personally like to have my tests grouped in a folder, so I'll create a folder called tests.
[03:08 - 03:18] And I'll follow along by having a file where we'll actually keep tests for this component. So I'll say home dot test, I can use dot spec or dot test dot TS.
[03:19 - 03:34] Since we actually would want to import components into this particular file, we would want the TypeScript JSX extension for our file name convention. So instead of test dot TS, we would most likely also want dot TSX.
[03:35 - 03:50] Okay. So since in this particular test file will most likely eventually move ahead to test the home component, we can go ahead and now import that particular component from its respective file.
[03:51 - 04:04] We won't use it just right away, but we'll import it regardless. And we've mentioned before, when it comes to setting up our test suites and our test files, the first thing we're going to do and use from just is the global function known as describe.
[04:05 - 04:11] Describe takes two arguments. The first argument is the name or the description of the test that one is trying to sort of compile here.
[04:12 - 04:17] In this case, we want to create tests for the home component. So we'll just say home.
[04:18 - 04:29] The second argument is a callback function that wraps the tests that we want to specify. Similarly, the very similar setup or a very similar number of arguments is the its blocks.
[04:30 - 04:42] Its blocks allow us to specify the actual unit tests that we want. So let's assume here just to get things started, we're going to specify a name for this particular block as first tests.
[04:43 - 04:50] It's not going to do anything with the home component. It's just for us to specify and make sure that our testing setup is working.
[04:51 - 04:57] And over here is where we're actually going to write our very first test. We've mentioned how just already comes with its own assertion library.
[04:58 - 05:14] And it gives us the expect method to give us all the different methods for us to write our assertions. So in this case, for example, I can just simply do a quick check and say, I expect one to equal one.
[05:15 - 05:23] Let's see if this works. We'll head back to our terminal and now we'll rerun the test command or the test script.
[05:24 - 05:32] It tells us that it's actually now picking up the fact that it's running tests from the home.test.tsx file. And it shows that this particular first test now passes.
[05:33 - 05:56] If we were to go back and try to make this test fail, let's say I expect one to equal two, but I was to go back to my test while this is still in watch mode, it would pick up that this test now fails. And another useful note to specify here is sometimes or most often actually the logs within just are usually pretty helpful and they'll actually specify where things have gone wrong.
[05:57 - 06:02] So here it's pretty apparent what's happening. They're saying we expected a value of two, but we received one.
[06:03 - 06:17] So something is wrong here or this test is failing. So now that we know our just runners working as expected, let's just install the two other utilities we'll be using shortly.
[06:18 - 06:26] We'll first install the React testing library. Now it's a quick note actually before I install this.
[06:27 - 06:40] If we go to the package JSON file, we mentioned this in the course before somewhere. With create React app, they tend to group all the dependencies into a single dependencies field as opposed to using a separate development dependencies for development libraries.
[06:41 - 06:59] I don't think this is necessarily a big deal and it usually comes a little bit more important when you're trying to deal with deployments, but for now we'll go ahead and install the testing tools or the testing libraries in development dependencies. So I'll go ahead and install the testing library react.
[07:00 - 07:08] Now this is installing. I'll grab the other library we're interested in installing, which is the Apollo react testing tool.
[07:09 - 07:35] Great. As a quick note, if you're using a project that you've just recently been working on, you would most likely not see this many vulnerabilities.
[07:36 - 07:39] I'm picking on from an older project that I had. So I'll just do this.
[07:40 - 07:57] This is not really related to what we're trying to do, but I'll just try to fix any of these vulnerabilities that NPM can allow us to fix them. Cool.
[07:58 - 08:12] Now if you go to the package JSON file, we'll notice the two new dependencies we've added, Apollo react testing and testing library react. These two libraries already have their type definitions, so we won't have to install any other additional type definitions.
[08:13 - 08:20] Awesome. So we'll stop here for now and in the next lesson we'll continue from where we are here and begin to write some tests for our very first components.
This lesson preview is part of the TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL - Part Two course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL - Part Two, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
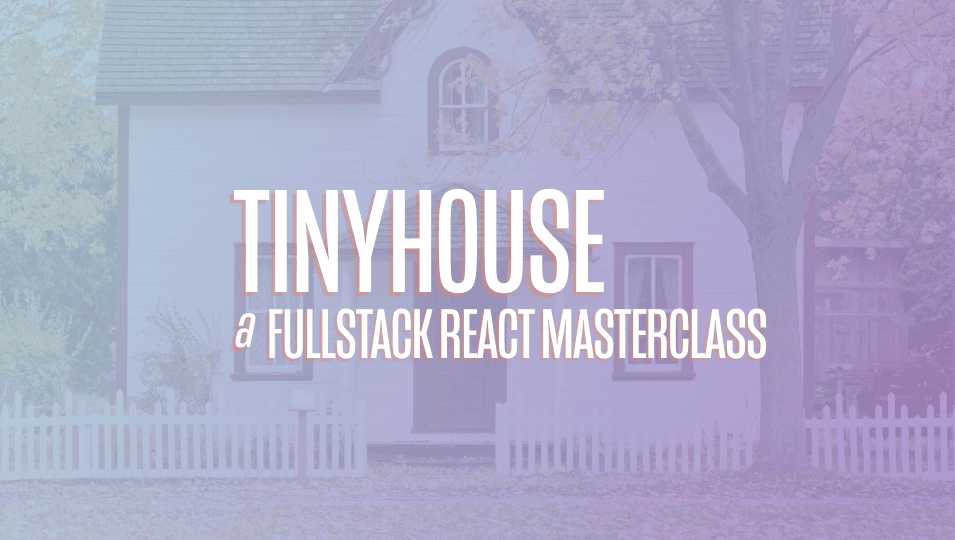
So, in this lesson, we'll begin by just making sure we have all the libraries we need in our tests. And we may just do a quick check to create a simple test file and write some dummy tests to make sure our runner is working and everything is set up. If we head over to our package JSON file and just to mention, this is the client project from the latest point of our tiny house course. So assuming you've managed to go through the course, you've set up everything you needed to do, at this very moment in time, this is where we're picking up the testing module or the work we're going to do with testing. If we take a look at the package JSON file, we'll notice that there's already some type definitions installed for Jest. And this comes installed with the Create React app utility that we used to create our React application. Create React app uses Jest as its test runner. And they've set up all the necessary tooling that we may need such that there isn't really any other changes we'll need to do. Some of the cool things that already exist is the file name conventions that the test runner just will look for to actually run tests. If we create a file with the .js suffix in an underscore underscore test folder , or if we have files with the .test.js suffix or a file with the .spec.js suffix, if we were to run the command to run our tests, the runner will look through all these files to actually execute those tests. Notice that this .js suffix refers to the JavaScript portion. Similarly for us, we can have the TypeScript convention as well, .test.ts, etc. And from the package JSON file, we'll notice in our scripts, there is already a test command. This test command utilizes the React Script library that has Jest and all the tooling set up to run a test command. What does this NPM run test script do? Test would launch in Watch mode by doing so. Every single time we save a file, it would rerun the test to pick up the changes that were made to the tests to then rerun those tests again. As of this moment, we have no test set up, but we can still run this script. So if we head to our command line and if I was to run NPM run tests, don't tell me that it's running React Script test and it's smart enough to know that no tests were found related to files changed since the last commit. If I was interested to just run every test that might exist, I'd just press A to run all tests and it knows that there's no tests that have been found. And it shows that it's still in Watch mode. So I'm able to then go back and have it just ready for me to pick up any changes to any new tests that I add. So let's now go ahead and try to create a test file. If you head over to our sections folders, we'll see how we've neatly separated all the different sections or all the different high level components in our app. Assume we wanted to begin with the home components and we wanted to create tests just for this component alone. I personally like to have my tests grouped in a folder, so I'll create a folder called tests. And I'll follow along by having a file where we'll actually keep tests for this component. So I'll say home dot test, I can use dot spec or dot test dot TS. Since we actually would want to import components into this particular file, we would want the TypeScript JSX extension for our file name convention. So instead of test dot TS, we would most likely also want dot TSX. Okay. So since in this particular test file will most likely eventually move ahead to test the home component, we can go ahead and now import that particular component from its respective file. We won't use it just right away, but we'll import it regardless. And we've mentioned before, when it comes to setting up our test suites and our test files, the first thing we're going to do and use from just is the global function known as describe. Describe takes two arguments. The first argument is the name or the description of the test that one is trying to sort of compile here. In this case, we want to create tests for the home component. So we'll just say home. The second argument is a callback function that wraps the tests that we want to specify. Similarly, the very similar setup or a very similar number of arguments is the its blocks. Its blocks allow us to specify the actual unit tests that we want. So let's assume here just to get things started, we're going to specify a name for this particular block as first tests. It's not going to do anything with the home component. It's just for us to specify and make sure that our testing setup is working. And over here is where we're actually going to write our very first test. We've mentioned how just already comes with its own assertion library. And it gives us the expect method to give us all the different methods for us to write our assertions. So in this case, for example, I can just simply do a quick check and say, I expect one to equal one. Let's see if this works. We'll head back to our terminal and now we'll rerun the test command or the test script. It tells us that it's actually now picking up the fact that it's running tests from the home.test.tsx file. And it shows that this particular first test now passes. If we were to go back and try to make this test fail, let's say I expect one to equal two, but I was to go back to my test while this is still in watch mode, it would pick up that this test now fails. And another useful note to specify here is sometimes or most often actually the logs within just are usually pretty helpful and they'll actually specify where things have gone wrong. So here it's pretty apparent what's happening. They're saying we expected a value of two, but we received one. So something is wrong here or this test is failing. So now that we know our just runners working as expected, let's just install the two other utilities we'll be using shortly. We'll first install the React testing library. Now it's a quick note actually before I install this. If we go to the package JSON file, we mentioned this in the course before somewhere. With create React app, they tend to group all the dependencies into a single dependencies field as opposed to using a separate development dependencies for development libraries. I don't think this is necessarily a big deal and it usually comes a little bit more important when you're trying to deal with deployments, but for now we'll go ahead and install the testing tools or the testing libraries in development dependencies. So I'll go ahead and install the testing library react. Now this is installing. I'll grab the other library we're interested in installing, which is the Apollo react testing tool. Great. As a quick note, if you're using a project that you've just recently been working on, you would most likely not see this many vulnerabilities. I'm picking on from an older project that I had. So I'll just do this. This is not really related to what we're trying to do, but I'll just try to fix any of these vulnerabilities that NPM can allow us to fix them. Cool. Now if you go to the package JSON file, we'll notice the two new dependencies we've added, Apollo react testing and testing library react. These two libraries already have their type definitions, so we won't have to install any other additional type definitions. Awesome. So we'll stop here for now and in the next lesson we'll continue from where we are here and begin to write some tests for our very first components.