Promise
Explanation of promises in JavaScript
Promises introduced in ES2015 have transformed the way we handle asynchronous code in JavaScript. Promises are meant to address the problems we discussed with callbacks.
A promise represents an object that acts as a placeholder for a value that is typically produced as a result of an asynchronous operation. In other words, a promise object represents the successful completion or failure of an asynchronous operation. There is a common misconception among beginners that promises to make our code asynchronous; they do not. Think of promises as a notification mechanism that notifies us about the success or failure of some operation that is already asynchronous. Promises wrap asynchronous operations and allow us to execute code when an asynchronous operation is successfully completed or when it fails. That's all a promise does. Nothing more, nothing less. It is only meant to observe the asynchronous operation and notify us when that operation is completed.
Before we learn how we can create promise objects, let us first learn how we can deal with promises using the built-in fetch
function that allows us to make HTTP requests from the JavaScript code running in the browser.
When the fetch
function is called, instead of making the calling code wait for the HTTP request to complete, it returns a promise object. We can associate callback functions with the returned promise object to execute code when the HTTP request is complete. We still use callbacks with promises, but the problems we discussed with callbacks in an earlier lesson in this module don't exist when using promises. Compared to callbacks, promises provide a clean and structured way to handle asynchronous operations in JavaScript.
const p1 = fetch(/* some url */);
The promise returned by the fetch
function can be thought of as the fetch
function promising us to supply a value when the HTTP request completes some time in the future. In the meantime, the main thread is free to do other things.
What can we do with the returned promise? We can register callbacks with the promise object that will be invoked when the network request completes. We can register separate callbacks to handle the success or failure of the network request.
Promise states#
Promises can be in one of the following three states in their lifecycle:
pending: the initial state in which promises typically start when they are created. It indicates that the asynchronous operation associated with the promise is in progress.
fulfilled: means that the asynchronous operation associated with the promise has been completed successfully.
rejected: means that the asynchronous operation associated with the promise has failed.
During the lifecycle of a promise, its state changes from pending to either fulfilled or rejected. The state of a promise is saved in the hidden internal slot named [[PromiseState]].
A promise in the pending state is considered unsettled. Once the promise transitions from the pending state into either a fulfilled or rejected state, it is said to have settled.
Promise instance methods#
There are three instance methods we can call on promise instances:
Promise.prototype.then()
Promise.prototype.catch()
Promise.prototype.finally()
then method#
The then
method is used to register a callback that is invoked asynchronously once the promise is fulfilled, i.e., the asynchronous operation wrapped by the promise completes successfully. This method allows us to execute code upon the successful completion of an asynchronous operation. Consider the following code example:
// code to execute if the promise fulfills
The then
method accepts two callback functions as arguments: the fulfillment handler and the rejection handler. The fulfillment handler is the first argument, as shown in the code example above. The rejection handler is the optional second argument that is invoked if the promise on which the then
method is called gets rejected.
// code to execute if the promise is rejected
The fulfillment handler is passed the result of the asynchronous operation as an argument. In other words, the fulfillment handler receives the value with which the promise is fulfilled. In the case of an HTTP request, the promise is fulfilled with the server response, so the fulfillment handler receives the server response as an argument. On the other hand, the rejection handler receives the rejection reason as an argument if the promise is rejected.
catch method#
We learned in the previous section that we can pass the rejection handler as the second argument to the then
method to handle the promise rejection. There is another option to register the rejection handler, and that is through the catch
method. Instead of passing the rejection handler to the then
method, we can call the catch
method on the promise to register the rejection handler.
This lesson preview is part of the Advanced JavaScript Unleashed course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Advanced JavaScript Unleashed, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
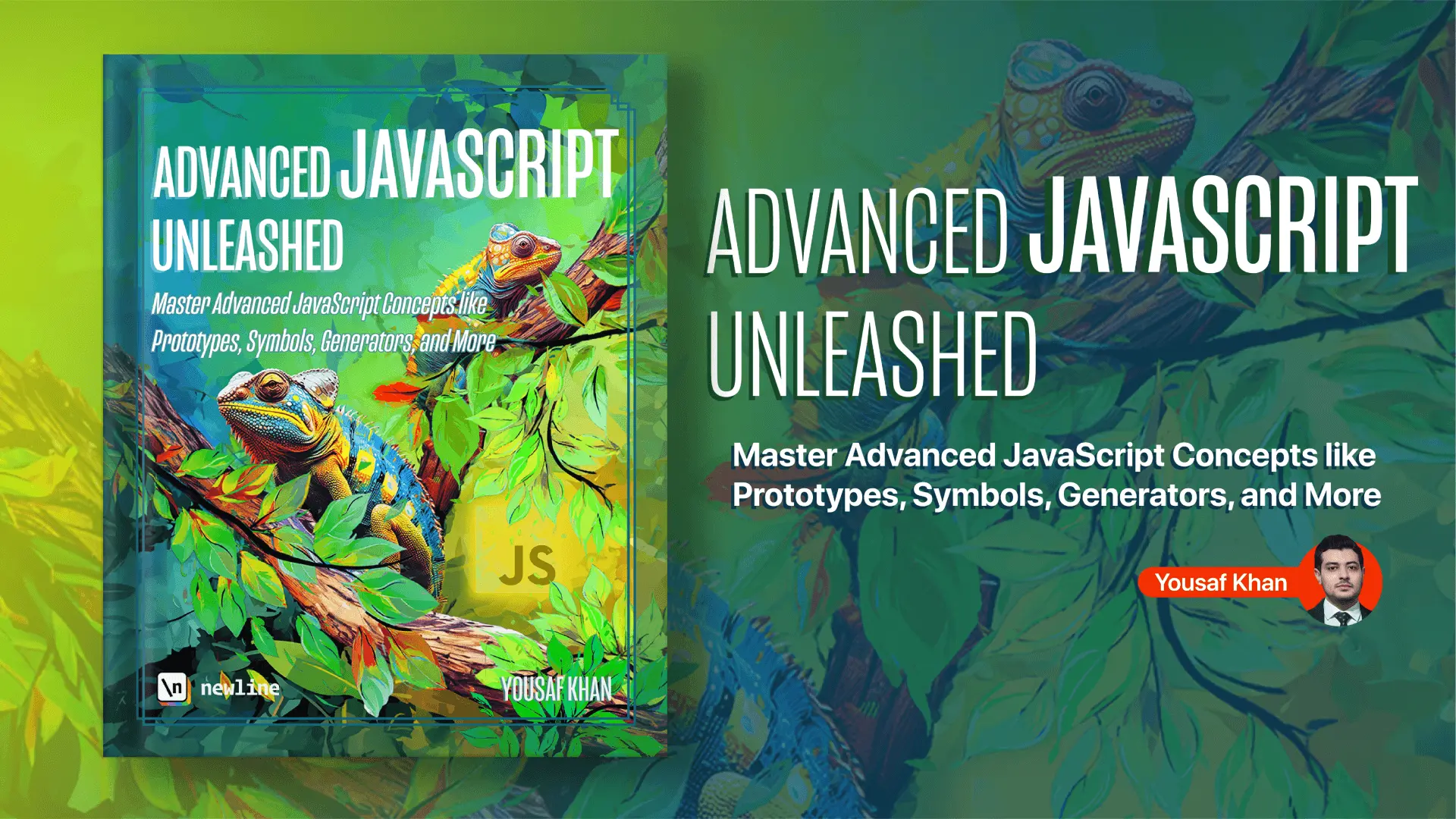