Prototype chain
Explore the prototype chain
Now that we know what prototypal inheritance is let us explore the prototype chain.
Object.prototype - parent of all objects#
At the top of the prototypal inheritance hierarchy is the Object.prototype
object. It is the root object or parent of all objects. When we create an object literal, its prototype is the Object.prototype
object.
xxxxxxxxxx
const obj = {};
​
console.log(Object.getPrototypeOf(obj) === Object.prototype);
// true
You can run the above code in the Replit below:
We didn't define any properties on the obj
object. But we can still call some methods on it.
xxxxxxxxxx
const obj = {};
​
console.log(obj.toString()); // [object Object]
You can run the above code in the Replit below:
We didn't define a method named toString
on the obj
object; how is it accessible in the code example above? You guessed it: it is defined on the Object.prototype
object, and as Object.prototype
is the prototype of obj
, the properties defined on Object.prototype
are inherited by obj
, toString
being one of them.
Objects created in this way are instances of the Object
constructor function. We can also define obj
as shown below:
xxxxxxxxxx
const obj = new Object();
This has the same effect: it creates an empty object. As discussed in the previous lesson, functions have a prototype property that points to an object that serves as the "prototype" of all instances of that function when that function is invoked as a "constructor". So, the Object.prototype
object serves as the "prototype" of all objects created via new Object()
or through object literal notation.
At this point, you might ask: isn't toString
callable on all objects? Yes, it is; some objects inherit it from the Object.prototype
object, while other objects, such as arrays, inherit it from their prototype, i.e., the Array.prototype
object, which overrides the toString
implementation defined in Object.prototype
.
This lesson preview is part of the Advanced JavaScript Unleashed course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Advanced JavaScript Unleashed, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
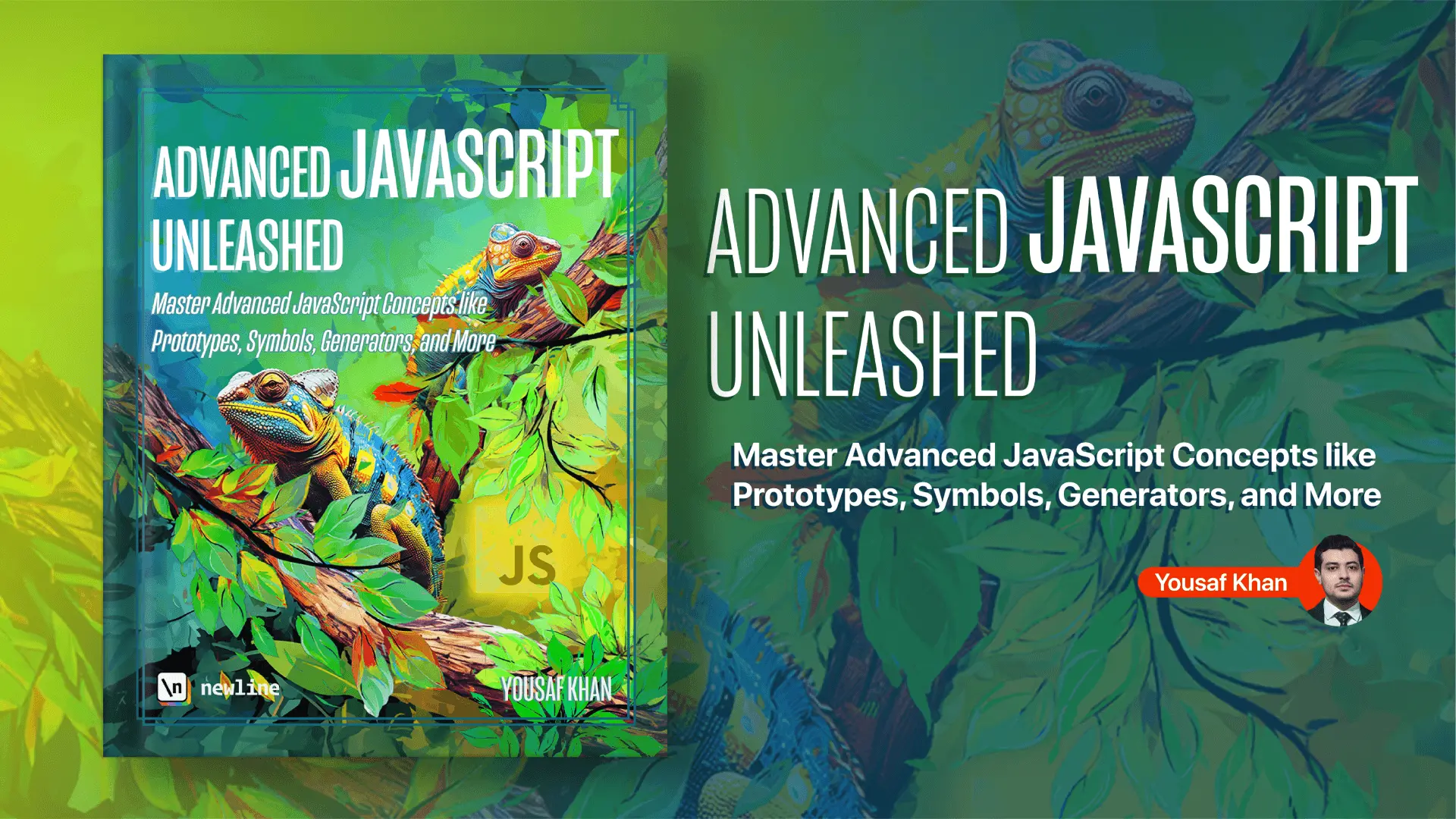