How to Build Accessible Navigation Divs in React
Let's revisit the Hero section from Module 1 and build it for real using the primitives we have learned.
This lesson preview is part of the Composing Layouts in React course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Composing Layouts in React, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
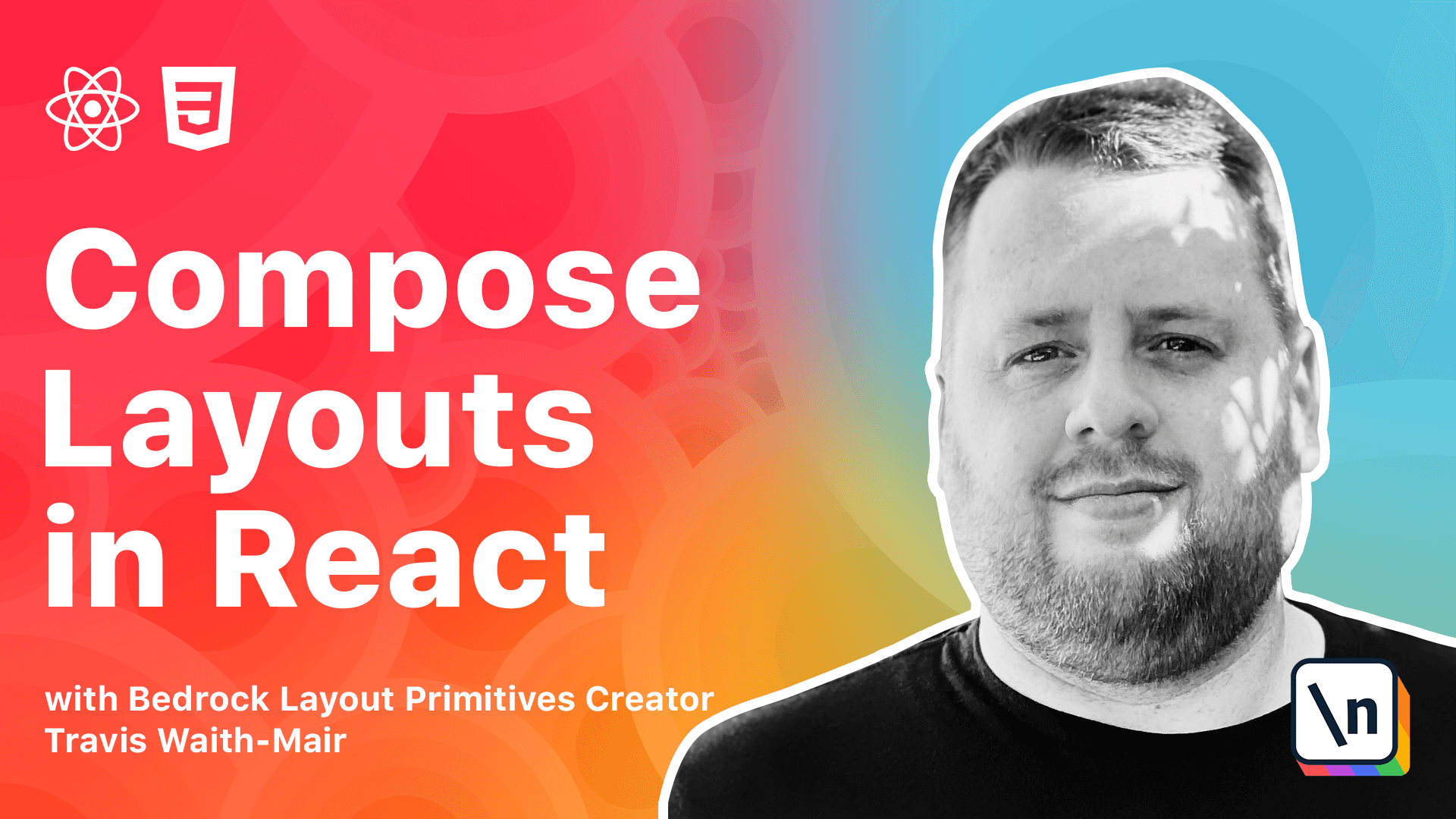
[00:00 - 00:11] What's up guys, Travis here with another lesson in composing layouts in React. In this lesson we are going to revisit the hero mockup from module 1.
[00:12 - 00:37] In module 1 we introduce the concept of composition by analyzing this hero mock up. Now at the time we gloss over implementation of how each layout primitive works , but now that we've built a complete set of layout primitives, I thought it would be good to revisit that hero section from module 1 and build it for real this time using primitives that we have learned.
[00:38 - 00:50] Now in this lesson we'll be using the bedrock layout primitive library. It has all the primitives that we built in this course, plus a couple other tools that help us layout our website.
[00:51 - 01:00] So in this lesson we are going to build this hero layout. We have a menu on the top and two equal width areas below it.
[01:01 - 01:14] The area on the left will have a vertically centered call to action section, and the right side will have an image that will cover the area and maintain an aspect ratio. There will also be a small gutter in between each of these areas.
[01:15 - 01:24] So before we get into the solution here, I want to just bring up an important point that gets overlooked very often. In fact, I've kind of brushed over it in this course already.
[01:25 - 01:36] And that's a word on accessibility. And specifically I want to talk about using the correct semantic markup.
[01:37 - 02:03] Because whole courses could be written on accessibility, I'm not trying to get into this, but one of the easiest mistakes that we can fix really easily is using the correct markup to denote the correct item. And it's a problem, unfortunately, not just with what we've been doing, but with anything that's been component based, as it gets really easy to put everything inside of a div.
[02:04 - 02:33] And we don't want to forget that these semantic markups, like the nav or sections, headings, all have meanings, and as used, especially by screen readers, to help people with, can't see our websites who are using screen readers to not just know what's going on, but also to navigate. So from this point on, we're actually going to emphasize, make sure that we don't forget to use the correct semantic markup going forward .
[02:34 - 02:45] And to do that, we're going to make sure we heavily take use of that as prop so that we can make sure that we're using the correct markup. So here we are.
[02:46 - 02:51] This is the beginning of our hero section. This will, everything's wrapped in a header.
[02:52 - 03:12] This is going to be our menu with a nav and an unordered list, and then we have the bottom half, which consists of two parts, a section with a call to action section over here and an image. And that's how that works.
[03:13 - 03:23] Now, we already know what we need, so we need to bring in. We're going to need to bring in a couple things from bedrock layout.
[03:24 - 03:36] So I'm just going to preemptively bring those in. We're going to need to bring in a stack from that bedrock layout stack.
[03:37 - 03:48] And you can see I've already installed pretty much everything we need. We're going to need a split.
[03:49 - 04:16] I know we're going to need both an inline and a -- I'm going to spell the inline cluster. Did that wrong?
[04:17 - 04:20] That's an inline. Oops.
[04:21 - 04:27] And inline cluster. I think that's good for now.
[04:28 - 04:36] So let's go ahead. We know we need -- everything's going to be wrapped in a stack as a header with a smoke.
[04:37 - 04:43] Got her, so let's do that. And you see that happen already.
[04:44 - 04:57] And then we're going to go ahead and wrap the menu bar in the inline. With the large gutter, we're going to align everything center.
[04:58 - 05:06] We're going to stretch that middle. This nav, we're going to use an inline cluster as an unordered list with an extra-large gutter.
[05:07 - 05:19] And everything's going to be centered horizontally. And then finally, the right-hand side is going to have a large gutter with it vertically centered as well.
[05:20 - 05:28] Awesome. See how fast we were able to make that menu bar just with these components?
[05:29 - 05:47] Now, let's go ahead and split our bottom half. And we're like halfway there already.
[05:48 - 06:06] So we know we need a cover and we know we need a frame. So let's import those.
[06:07 - 06:29] Now, the cover has a natural height of 100 view heights. But we don't want to be 100 view heights because we have this menu bar that's taking up some space plus this small gap here.
[06:30 - 06:45] So what we're going to do instead, with the power of copying and painting, we 're going to just go over that. And using cover, we're going to use gutter none.
[06:46 - 06:59] But the min height, we're going to pass in this string here. We're going to calculate 100 view heights minus 48 pixels, which I already measured ahead, is the height of this menu bar.
[07:00 - 07:11] And also minus the size of the gap, this small gutter. And that gets us the rest of the remaining space.
[07:12 - 07:27] That's what's really cool about this calculation function. Perfect. So the only thing left we need to do is we need to wrap the image in a frame.
[07:28 - 07:43] So let's go ahead and once again, just take advantage of copying paste. We're using frame without a ratio.
[07:44 - 08:07] And then the frame will still keep the aspect ratio of that image and not distort it. And as we change that height, it will keep the image exactly the way it's aspect ratio and move it to the height it needs.
[08:08 - 08:12] And there we go. And that's it's done.
[08:13 - 08:27] We've achieved exactly what we need to. And if we go to different size screens here, it will keep its look and do exactly what we need.
[08:28 - 08:39] It was that simple. So we're going to do more of this in the next module. We're going to actually build a full on page, not just a section or a widget.
[08:40 - 08:49] We're going to build a whole setting page from scratch using just bedrock layouts, just using these layout primitives. You'll see just how amazing it is and how fast we can build something.
[08:50 - 08:52] And I'll see you on the next lesson.