How to Build a React Navbar With a Menu and Header
This lesson is going to focus on getting the menu and header completed on our mock Settings Page
This lesson is going to focus on getting the menu and header completed on our mock Settings Page.

We will be doing everything in codesandbox.io, using their React Starter Template. To ensure you are all using the identical dependency versions, I would recommend going to this sandbox that I have already made and forking it by clicking on the "Fork" button in the upper right-hand corner:

This will create your own copy so you can follow along.
Setting up the index.js
#
Let's get a few things in place so we can get started. The first thing we need to do is install styled-components
and the @bedrock-layout/css-reset
. That can be done on the left-hand side, under Dependencies
:

Just search for the package you want, like styled-components
and select it. Codesandbox will then install it for you. This is equivalent to running npm install styled-components
on your local machine.
Then in the index.js
file, I am going to import the css-reset:
xxxxxxxxxx
import "@bedrock-layout/css-reset/lib/reset.css";
import { StrictMode } from "react";
import ReactDOM from "react-dom";
While we are in this file, let's look at AppBoundary, which is something I like to add to all my projects. High-resolution screens are becoming more commonplace, and websites are much easier to use when the content width is capped at a useable width. Bedrock already has a component called AppBoundary
that limits the width at 1920px
and keeps the content centered horizontally on the screen.
So let's install @bedrock-layout/appboundary
and import it into our index.js
file:
xxxxxxxxxx
import "@bedrock-layout/css-reset/lib/reset.css";
import { StrictMode } from "react";
import ReactDOM from "react-dom";
import { AppBoundary } from "@bedrock-layout/appboundary";
Then all we need to do is wrap our App
component in index.js
in the AppBoundary
:
xxxxxxxxxx
ReactDOM.render(
<StrictMode>
<AppBoundary>
<App />
</AppBoundary>
</StrictMode>,
rootElement
);
The menu's basic layout#
In App.js
, let's start off by removing the imported styled sheet at the top and the className on the wrapper div. (Don't delete the stylesheet from the project as we will use that later.) Now our entire file looks like this:
xxxxxxxxxx
export default function App() {
return (
<div>
<h1>Hello CodeSandbox</h1>
<h2>Start editing to see some magic happen!</h2>
</div>
);
}
Before we think about layout, let's just stub out what is going to be in our MenuBar. We need a logo, a navigation area, a search input, an icon, and a profile image. Let's remove the sandbox content and add our menu content:
xxxxxxxxxx
return (
<div>
<div>
<div>Logo</div>
<nav>
<ul>
<li>Dashboard</li>
<li>Job</li>
<li>Applicants</li>
<li>Company</li>
</ul>
</nav>
<input placeholder="Search" />
<div>icon</div>
<div>profile</div>
</div>
</div>
);
What we need to do in this MenuBar is inline everything, with the nav section stretching out to use all available space. So let's bring in the Inline component by installing @bedrock-layout/inline
and importing it into the file. Just like above you install it by searching for it in the dependency list on the left.
xxxxxxxxxx
import { Inline } from "@bedrock-layout/inline";
Then we can use that as the wrapper of our MenuBar like this:
xxxxxxxxxx
<Inline gutter="lg" align="center" stretch={1}>
At this point, our MenuBar looks like this:

Our navigation list also needs to be inlined, so let's use the Inline component as a ul
with sm
gutters:
xxxxxxxxxx
<nav>
<Inline as="ul" gutter="sm">
<li>Dashboard</li>
<li>Job</li>
<li>Applicants</li>
<li>Company</li>
</Inline>
</nav>
This lesson preview is part of the Composing Layouts in React course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Composing Layouts in React, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
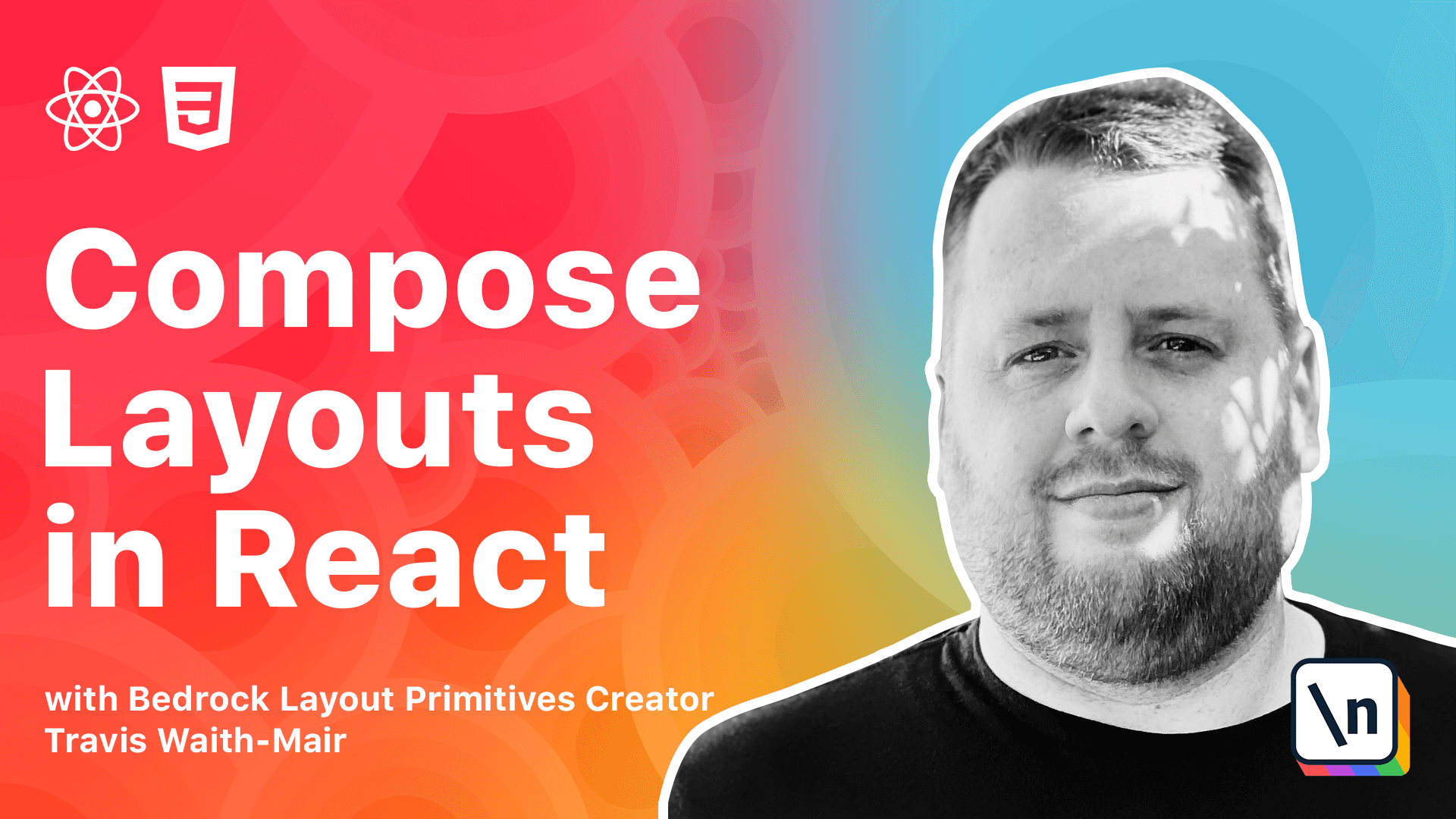