Testing tRPC Endpoints
Testing tRPC Endpoints
This lesson preview is part of the Fullstack Typescript with TailwindCSS and tRPC Using Modern Features of PostgreSQL course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack Typescript with TailwindCSS and tRPC Using Modern Features of PostgreSQL, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
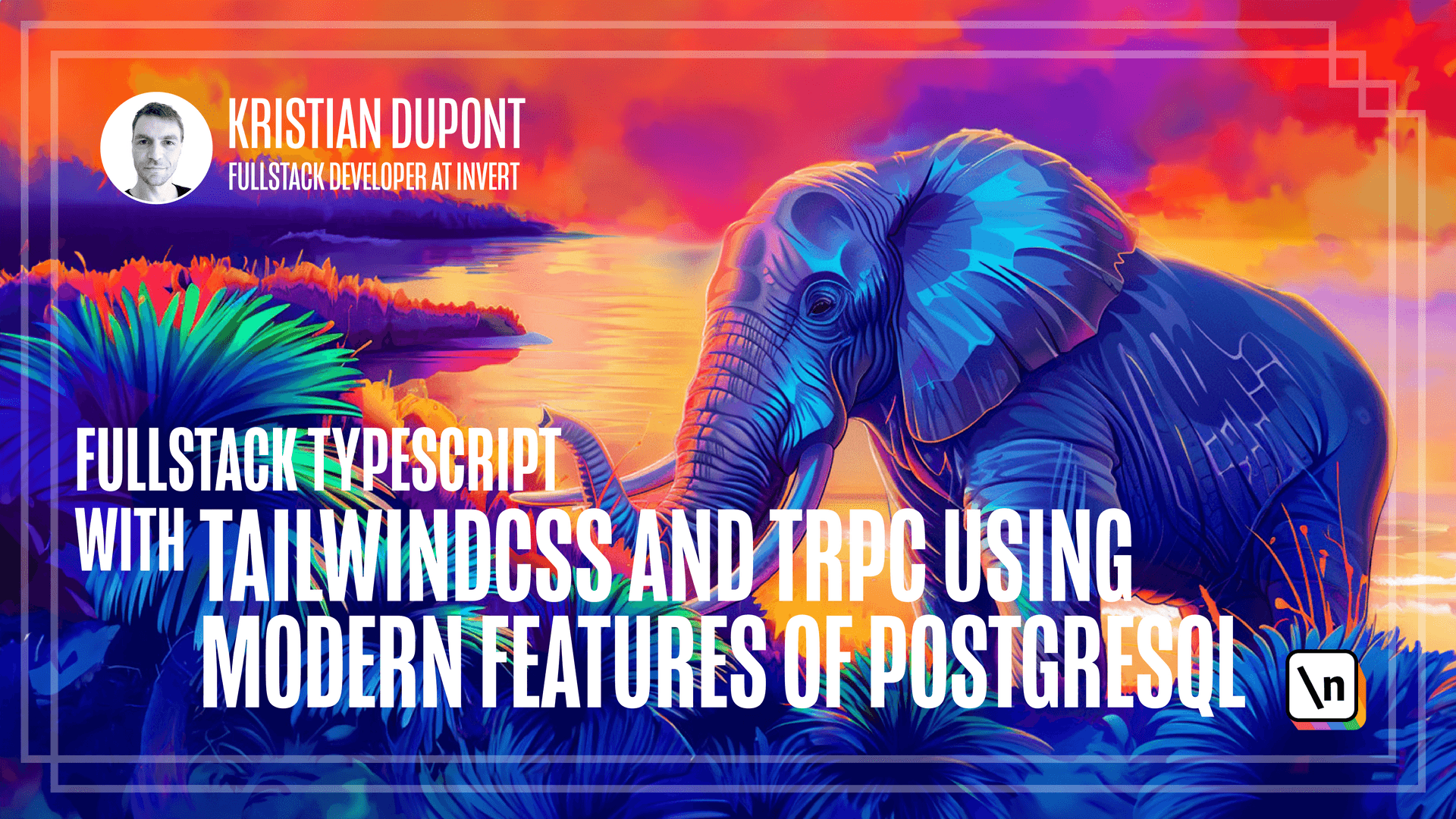
[00:00 - 00:17] Now that we have a bit of architecture in place, it's time for us to start thinking about testing. Testing is extremely important and I almost feel guilty for waiting even this long before introducing it because it's definitely something you should be thinking about from the very start when designing your architecture.
[00:18 - 00:34] There are a number of ways to think about the approach to testing and I think that the test pyramid which I believe Martin Fowler was the first one to come up with is a good place to start. It basically says that your foundation, so to speak, should be that you have a bunch of unit tests.
[00:35 - 00:46] On top of that you have some integration tests and then you have even fewer end -to-end tests. You might even have some manual tests but that depends a whole lot on what type of ab your building.
[00:47 - 01:11] I have experienced situations where it was almost impossible not to have some manual tests but obviously I would all make recommend that you go with automatic testing when you can. You can say that the tests further up in this pyramid are more valuable in that they encompass more code so they are more likely to catch a bug if one appears somewhere in your code.
[01:12 - 01:23] A unit test will only test a single unit so if the bug resides anywhere else it will go unnoticed by said particular test. From that perspective the tests at the top are most valuable.
[01:24 - 01:44] However they tend to also be the ones that are more expensive to write and they also tend to be the more expensive ones to execute in terms of CPU time on your continuous integration server. For that reason alone it's better to have more unit tests but the other reason is that unit tests are very nice for covering edge cases and sample space.
[01:45 - 02:22] If you have some piece of logic that can create a large combination of outcomes it's much better to create this piece of logic in a function or a class or something that is isolated and then test that with unit tests so that you can both run them quickly and they are fast to develop and then you don't need to go through signing up creating a user going through a bunch of form pages or whatever for some number of combination methods at the end because that would just be too expensive. For those reasons unit tests have certainly a great place in any test setup.
[02:23 - 02:42] So a back end is a very nice and testable piece of software because it exposes an API which is machine readable or usable and that is perfect for running tests. So we're going to set up first our tests which are going to be integration tests for our back end.
[02:43 - 03:03] We'll be using VTest which is a test framework created by the developers of V2 which we're already using. So you might be more familiar with Jest and this is a new test framework that has almost the same API but in my opinion is much nicer to work with and it's just faster and more pleasant to use.
[03:04 - 03:24] So let me start by installing that as a developer dependency here. And then we'll add a script to the back end package so dev will say test should be VTest run and let's get rid of that old one there.
[03:25 - 03:41] So now it will run VTest and we can configure it but let's do that in a second because the default configuration actually works quite well for us out of the box. So I'm going to create a test file here in the back end in the source folder and I'm going to call it App Router.
[03:42 - 04:06] Should put it in this folder here and I will paste this in here. So our App Router as you might recall has this ping endpoint and this test here will simply call that the ping endpoint and it will expect the result to be pong which is what comes out of the result.
[04:07 - 04:20] So a few things to note here, the describe, expect and it functions which are in the global namespace when you're using Jest have to be imported. So that is the primary difference from Jest.
[04:21 - 04:30] There are some other few small things that you need to be aware of but other than that everything should feel completely recognizable to you. Let's see what happens if I run tests.
[04:31 - 04:32] It looks like our test succeeds.