How to Customize the React useQuery Hook
With React's useState and useEffect Hooks, we've been able to query and display listings information the moment our Listings component is mounted. In this lesson, we'll create our very own custom useQuery Hook that will consolidate the pattern of creating a state property, using the useEffect Hook, and running the server fetch() function to make a query and state update when a component mounts.
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL with a single-time purchase.
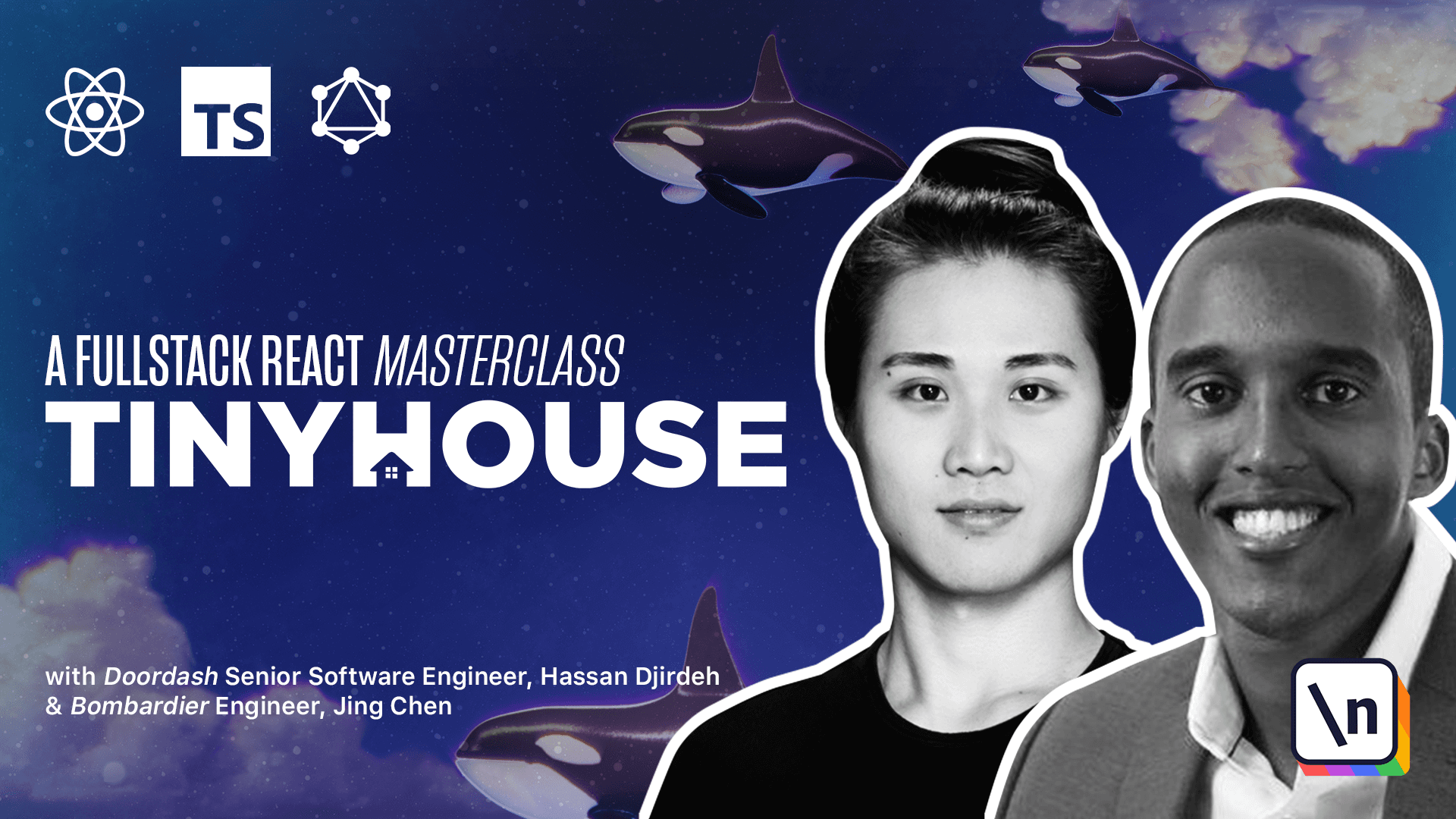