How to Autogenerate GraphQL Types With Apollo CLI
In this lesson, we'll use Apollo tooling's command line interface to autogenerate static types for the GraphQL requests in our client application.
This lesson preview is part of the TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL with a single-time purchase.
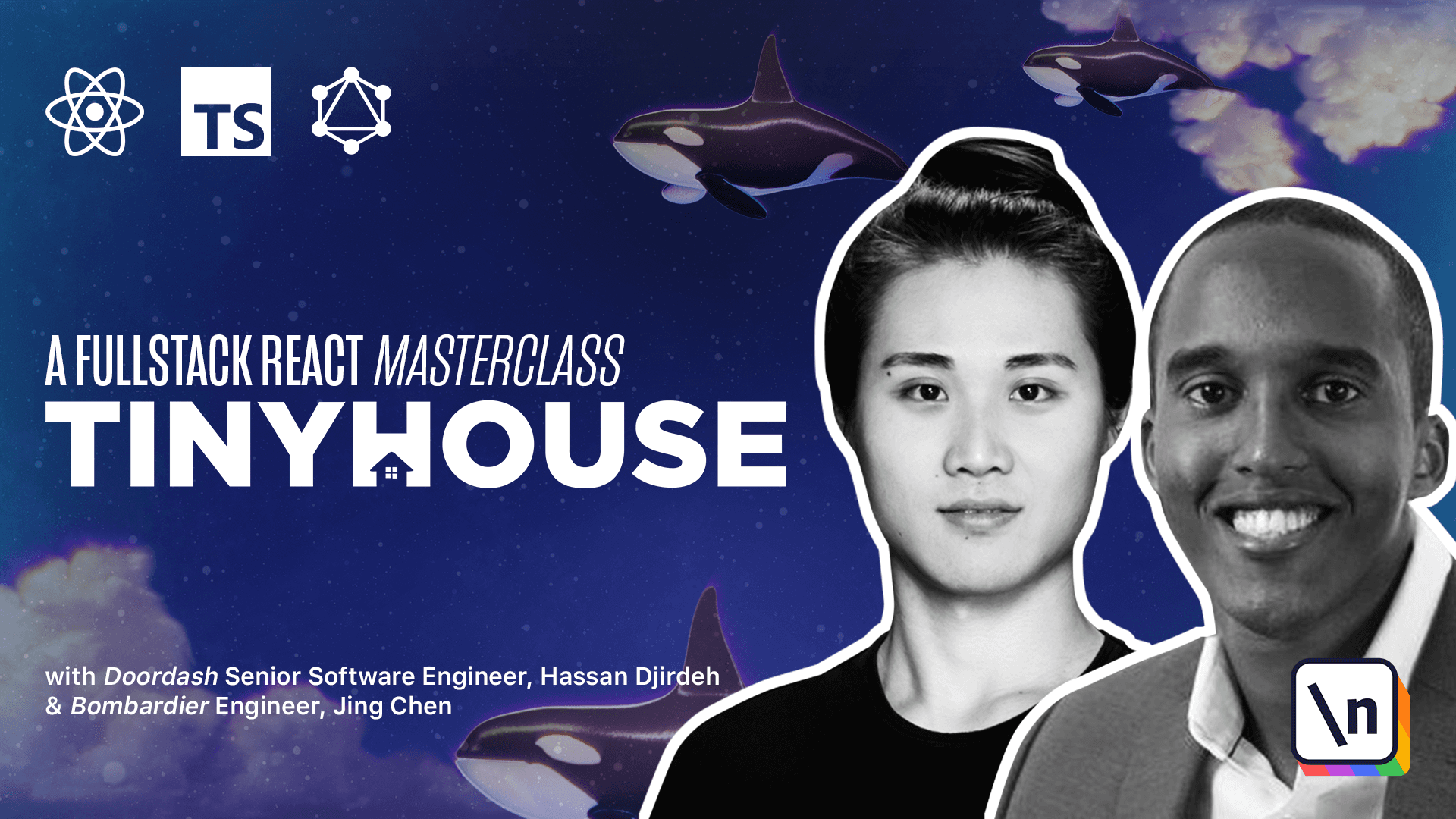
[00:00 - 00:13] In the current and last module, we've come to recognize how powerful hooks can be when it comes to making GraphQL requests. In this module, we've brought in Reactor Polo and used Reactor Polo's use query and use mutation hooks.
[00:14 - 00:35] Whether we've done it through custom means or with Reactor Polo, we've been able to pass in custom typings we've created to help shape the type of data and variables we are to use in our GraphQL requests. We've created the custom typings for our requests in the listings component in a types file in the listings folder.
[00:36 - 00:52] And we've constructed these types by looking through our GraphQL API and schema . This worked fine and there's nothing inherently wrong with this, despite having to make changes to our typings, if a type change was ever made in our server schema.
[00:53 - 01:07] Picture in a large scale production application, we could have dozens and dozens of GraphQL documents and requests everywhere. First off, it'll be a little tedious having to create the typings for all of these GraphQL requests.
[01:08 - 01:23] Second, a breaking change in the server, for example, changing the type in the server schema, will make finding and updating our client types a little difficult. And if we think about it, our GraphQL API already has its own types.
[01:24 - 01:31] Wouldn't it be cool if we can just leverage those types automatically? Well, we somewhat can with the help of code generators.
[01:32 - 01:49] The community has created a lot of different GraphQL code generators aimed at addressing this point. There's the popular GraphQL code generator library, there's typed GraphQLify, and even the Apollo CLI, or command line interface.
[01:50 - 02:16] Apart from the Apollo CLI, which is a little unique, many of these tools have their minor differences in setup, but their main aim is often similar to essentially generate typings from a GraphQL schema. Some of these libraries like GraphQL code generator and what a policy alike can provide can help generate typings for many different languages, like Swift, Java, C#, etc.
[02:17 - 02:37] In addition, some of these libraries help generate code, like your actual queries and mutations based on the schema that you specified in your GraphQL API. Though we won't be worrying ourselves too much about that, our main focus is how we can help generate typings from the GraphQL schema.
[02:38 - 02:56] Apollo CLI is a pretty robust command line interface that allows for a variety of different things, such as schema validation, server compatibility checks, and the ability to generate static types. We'll use the official Apollo CLI to generate static types from our GraphQL API .
[02:57 - 03:23] To use the Apollo CLI, we're able to actually install the command line globally and run commands in any project we like, but what we like to do instead is set up scripts in our applications package.json file and run the commands with the NPX command, which will help us avoid the need to globally install it. There's essentially two commands we'll need to run to be able to generate static types from our schema, with the Apollo CLI.
[03:24 - 03:38] The first command will help download our GraphQL schema onto our client project and save it for Apollo to be able to then generate types. And then the second command will help generate static types from our schema.
[03:39 - 03:53] We'll set up both of these commands as two separate script commands in our package.json file. We'll label them codegen schema and codegen generate.
[03:54 - 04:03] Downloading a schema is fairly simple. Essentially, we need to run the Apollo client download schema command and specify the options we'd want.
[04:04 - 04:32] In this case, we'll specify the single minimum option we need, the endpoint and pass in a value of our local GraphQL endpoint, http localhost 9000/api. Let's run the newly created codegen schema script in our command line.
[04:33 - 04:50] After a brief period, we'll notice success messages, stating loading Apollo projects and saving schema to schema.json. As we look at our project directory, we'll notice a schema.json file that represents our entire GraphQL schema.
[04:51 - 05:04] With our GraphQL schema available in our app, we can now look to generate the static types for our application query and mutation. This can be done with the CLI client codegen command.
[05:05 - 05:24] The first option we'll specify is the local schema file option, which is used to specify the path to our local schema file. Since the schema.json file we've generated is in the root of our project directory, our value for the local schema file option will be schema.json.
[05:25 - 05:49] The second option we'll specify is the includes option, which is used to state the files that the GraphQL operations will want to generate static types for. Once all of our GraphQL requests live in TypeScript files, and in this case right now, in TSX files within our source folder, we'll want to declare a search for these files in the source folder.
[05:50 - 06:14] So here we'll specify a value of source, star, star, star.tsx, which entails looking through our entire source folder and for any files that have the .tsx file extension. The final option we'll specify is the target option, which is required and allows us to say which code generator we'd like to use.
[06:15 - 06:36] Swift, Flow, Scala are all different options, but in our case we're interested in TypeScript. For a whole list of options and what can be done with the CLI, do check out the Apollo tooling readme that will provide a link for in our documentation, but this is all we'll need.
[06:37 - 07:00] Let's now run the newly created code generate command in our command line. Upon success, we'll see the success loading Apollo project message followed by generating query files with TypeScript target wrote three files.
[07:01 - 07:22] By default, the Apollo code generator creates static types for the GraphQL documents it finds in a generated folder located within the module it found those documents. If we take a look at the source section's listings generator folder, we can notice two files were created for each GraphQL request.
[07:23 - 07:37] In the query file we can see a listings interface that represents the shape of the data to be returned from our GraphQL listings query. In the mutation file, we can see interfaces for the data that is to be returned as well as the variables that the request expects.
[07:38 - 07:49] Amazing, we can now use these static generated types. In the listings component file, we'll import the appropriate types from each generated file.
[07:50 - 08:05] Once the listings namespace is used to represent the name of the components, we 'll import the listings data interface as listings data to prevent any naming conflicts. For consistency, we'll do the same for the delete listing data interface as well.
[08:06 - 08:18] We'll also remove the imports being made from our created types file. Our hooks are already consuming these type variables so there's nothing else left for us to do.
[08:19 - 08:29] Our app now works just as before. So with that said, we can now go ahead and delete the types file we've created before since we won't need it any longer.
[08:30 - 08:45] Great. If our server now was to ever change the schema, all we'll need to do is regenerate the schema JSON file, kept in our application, and then regenerate the automatically generated static type definitions.
[08:46 - 09:01] If our server schema was to remain the same, but there was a change in how we 're querying or mutating certain fields, all we'll need to do is just simply run the code generator command to regenerate the static types. Amazing.
[09:02 - 09:15] Now there's two notes to make before we close this lesson. For the Apollo CodeGen library to pick up GraphQL documents and make static types that reference them, it requires us to name all our GraphQL requests.
[09:16 - 09:38] If we attempt to run our static type generation command without naming our documents, Apollo CodeGen will throw an error. In addition to creating static type definitions for each GraphQL document, Apollo CodeGen also creates a global types file kept in the roots of the project under a generated folder of its own.
[09:39 - 09:53] The global types file keeps a reference of all enum and input object types that can exist in our GraphQL API. We don't have any in our application as of now, so as a result, our global types file is empty.
[09:54 - 10:02] If interested, one can also delete it as well, but we're just going to leave it as is even though it's empty. And that's it.
[10:03 - 10:16] Our application hooks now uses the statically generated types that the Apollo CLI helps generate. We won't be making any more changes to how our component makes GraphQL requests , which concludes this module.
[10:17 - 10:21] The next module, we'll see how we can style what we've built to make it more presentable.