How to Replace useQuery and useMutation With Apollo Hooks
With the Apollo client available everywhere in our app, we'll replace the use of the custom useQuery and useMutation Hooks with the variations provided to us from the React Apollo library.
This lesson preview is part of the TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL with a single-time purchase.
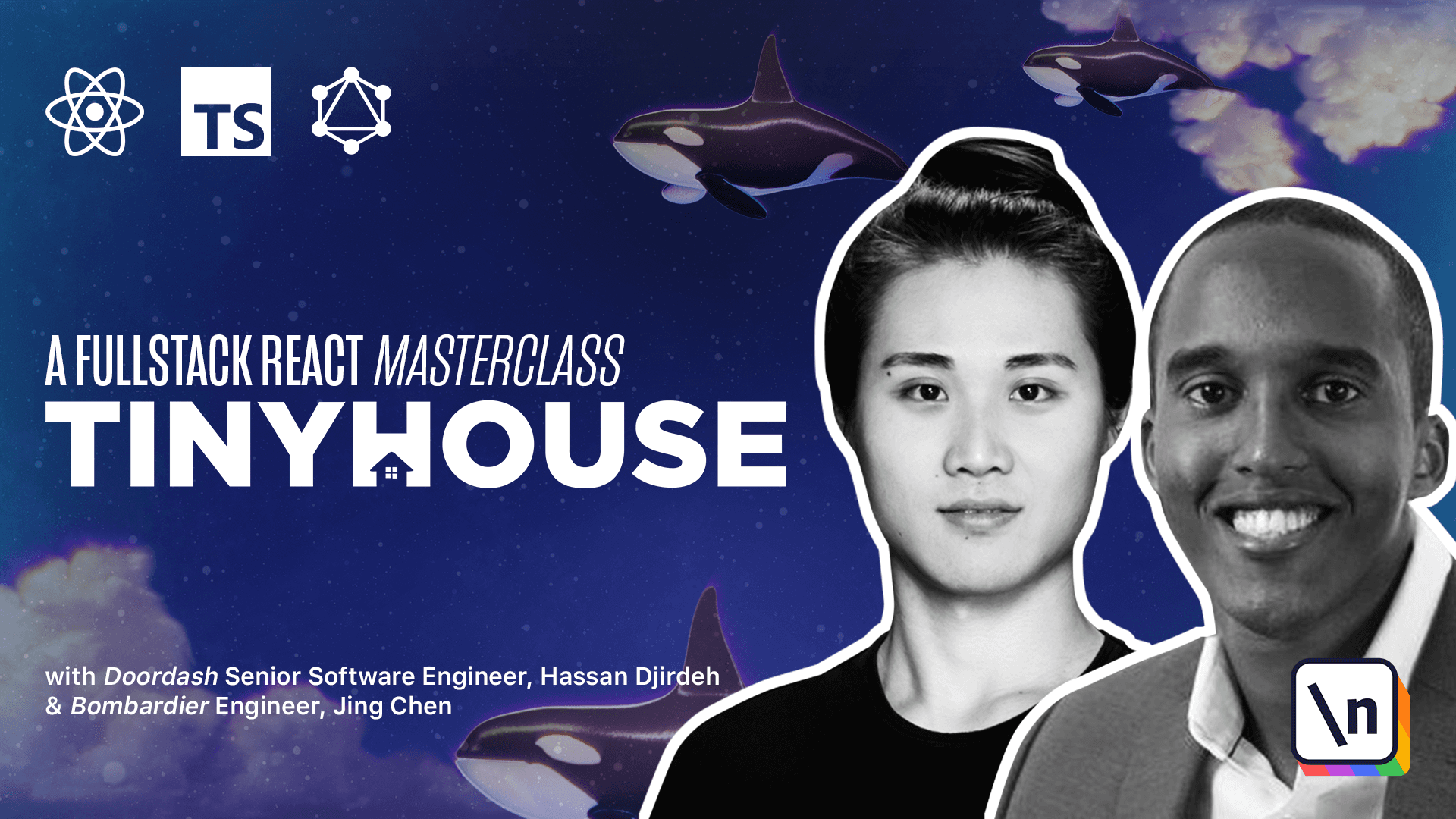
[00:00 - 00:09] We have the Apollo client created and available everywhere in our app. So let's see how we can import and use the hooks from Reactive Apollo in our Listings components.
[00:10 - 00:30] In the Listings components, we'll now import the use query and use mutation hooks from Reactive Apollo instead of our own local lib folder. Notice how the use of use query and use mutation doesn't throw any errors in our components, except for how we're passing in the GraphQL document nodes?
[00:31 - 00:49] Well, that's because we've built our custom use query and use mutation hooks to resemble very closely with how the hooks from Reactive Apollo actually work. The use query and use mutation hooks take two arguments, the query or mutation in question, and in options objects.
[00:50 - 01:10] Each hook also accepts two type variables, one to represent the shape of data to be returned, and the other to represent the shape of variables that can be passed in. The use query hook returns a series of fields within an object recognized as the query result, data, loading, error, refetch.
[01:11 - 01:33] In addition, it also returns a bunch of other fields, the Apollo client itself, the network status, a fetch more function, and so on. The use mutation hook returns a tuple, with the first value being the mutation function itself, and the second value being the mutation result options, which are similar to the options available in use query.
[01:34 - 01:40] There's still a few changes we'll need to make. The first is how we pass in the GraphQL document node.
[01:41 - 01:54] With our simple fetch function, we were able to realize that we could construct our GraphQL documents as strings. The hooks in React Apollo, however, expect our GraphQL documents to be constructed as a tree.
[01:55 - 02:11] We can do this with the help of the GQL function tag that's available to us, just like we've seen in our Apollo server application. We'll first import the GQL tag from Apollo Boost.
[02:12 - 02:35] In front of our GraphQL query documents, we'll apply the GQL tag function to have our strings be parsed as a GraphQL abstract syntax tree. With VS Code's Apollo GraphQL extension, we now get appropriate syntax highlighting for all our GraphQL documents created with the GQL tag.
[02:36 - 02:46] The next change we'll make is how we pass in variables to our use mutation hook . Before, we simply provided an object of key value pairs for every variable field.
[02:47 - 03:05] For the hooks in React Apollo, we'll need to specify an options object that has a variables field object within. This is because each hook accepts an options object where variables is just one option we can specify.
[03:06 - 03:24] And that's pretty much it. When we head to the browser, our application should work just as intended by allowing us to query listings on page load and delete listings when we click the delete button. The options we receive from our hooks, such as loading and error, dictates how we show information in the UI.
[03:25 - 03:37] When loading is true from the query, we display a message that simply says loading and nothing else. When we get an error from our query, we get an error message displayed in the UI and nothing else.
[03:38 - 03:58] Similarly, we display loading and error messages for our mutation, but at the bottom of our list. Since we're using the official React Apollo library, we can now remove the entire source lib folder since that contains the custom server fetch function and our custom use query and use mutation hooks that we're not using any longer .