Creating REST API GET, POST, PUT, and DELETE Requests
REST API Endpoints
This lesson preview is part of the Fullstack Svelte course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack Svelte, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
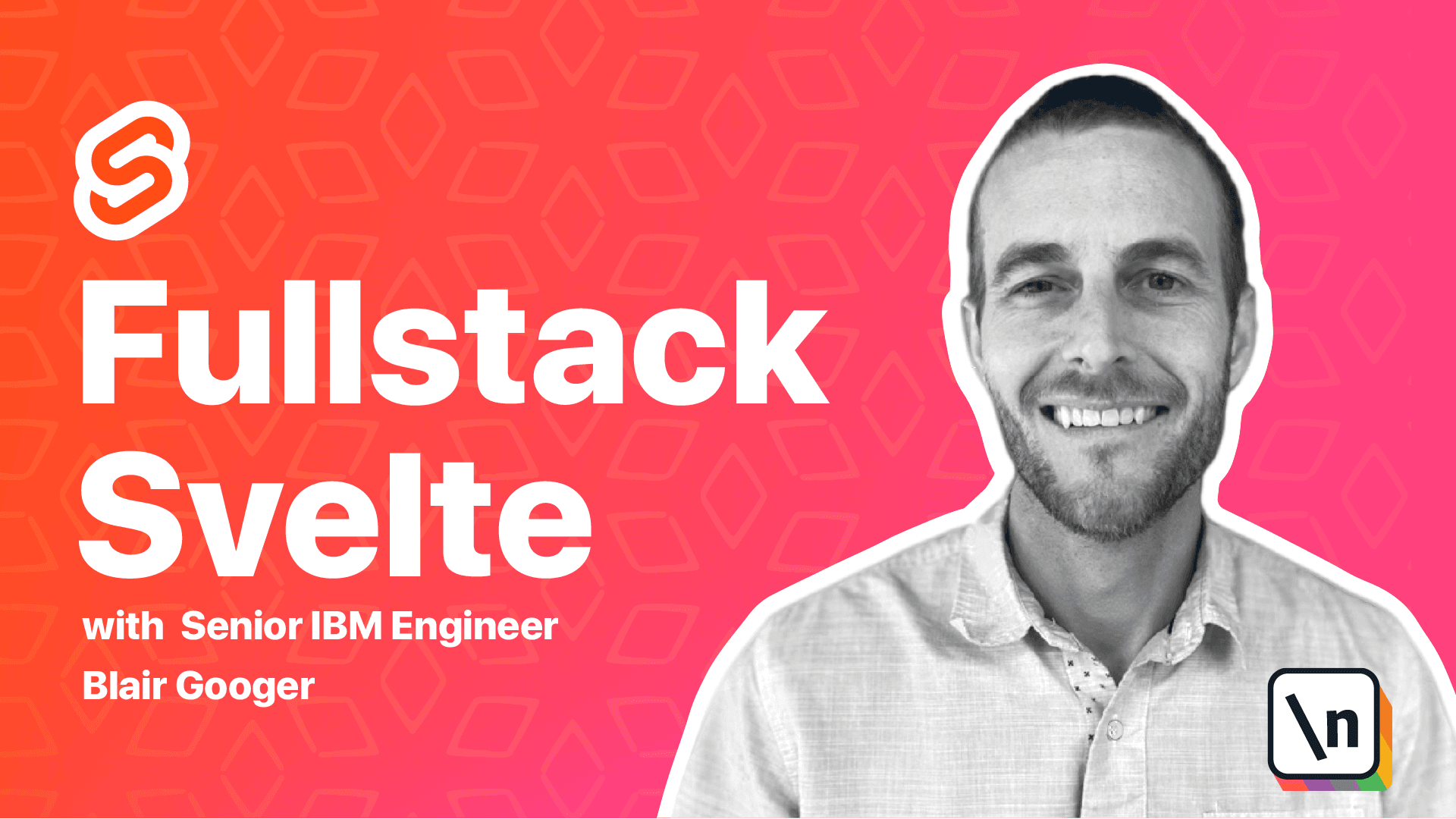
[00:00 - 01:55] Hello, welcome back. In this lesson, we're going to discuss REST API endpoints. We'll cover a few concepts about different types of HTTP requests, and then we'll update our express back end to return JSON. In the last lesson, we looked at a URL HTTP localhost 3000/users. So when we put this URL into our browser, it's going to trigger a get request, and it's going to send that request to the server specified at the beginning part of the URL. In our case, localhost just refers to the local machine, and then the port specified here. Normally on websites, we don't have a port because we use a domain name, but in the case of our local dev environment, we have localhost and then the port, and then the route of slash users. So if we look over here on the network tab, we can see that Chrome shows the request method of git, and then the response status code. So we ask for slash users, and then that gets handled by our express middleware and routed to the git handler, and back end routes users.js. This handler code that responds to an HTTP request is known as an endpoint. So it's the end destination of a network request, and it's responsible for replying back to the caller with a resource such as a file or a JSON payload. Any given route can have different endpoints that handle different HTTP methods.
[01:56 - 02:08] So git is one example, and then we'll review the other common ones, such as post, put, and delete. Git requests are used for fetching a resource, and they shouldn't be used for modifying data.
[02:09 - 02:28] For example, this would be a bad practice for a git request. So this one's running a git request, users, and then it says create user equals true, and it gives a username and an email address. So it kind of indicates that it's trying to create a resource instead of just fetching a resource.
[02:29 - 02:53] So this is not something you would want to do. Another concept in the above example is query parameters. So git requests can have query parameters, and they provide instructions to the server. Git requests, query parameters, are directly in the URL. So there's no body. So all the information is conveyed to the server via the URL itself.
[02:54 - 03:44] So up next we have post requests, and post requests are most commonly used for creating a resource. A post request can have query parameters, but it can also have a body, and in the body, additional data is sent to the server. So for example, we might have a post request that goes to the same route. Local has 3000 users, and then it sends in a body. And so this is a better way of creating a resource. So this is real similar to what we sent here, except we actually send JSON in with the body of the post request. And express will allow us to have several different endpoints on the same route, but with different HTTP methods.
[03:45 - 04:06] So under this case, we would say post here, and we would create a resource here. And so express knows based on the request type, whether to send it to this endpoint handler code, or this endpoint handler code.
[04:07 - 04:27] Usually the response to a post request would contain the unique ID of the newly created resource. So for example, we might have something that looks like this user created successfully ID1.
[04:28 - 05:01] There are some cases where a post request can also be used to fetch a resource. This is because there's a URL length limit. So imagine if we had a real complicated query, that was many characters long, we could hit the URL length limit, and it wouldn 't be appropriate for a get request. So we could also send in that case, we could send in a post request, and our query would be represented in the body. And the endpoint would just return a response versus creating a response.
[05:02 - 05:16] Okay, up next is put request. Put requests are similar to post requests. They can have parameters in a body, but they're used to update an existing resource.
[05:17 - 05:57] So imagine that we put originally, we put email address [email protected]. If we wanted to change that resource, and we got back ID1, we wanted to change that resource, we might have a post request that looks like this. So we pass, we go to the user's route, but this time we add slash one to identify the ID. And then we have a body that says the username and the email address. And the endpoint would take this body and replace the existing resource with this new data, in which case we update the email address.
[05:58 - 07:14] And then last, we have the delete request. And this one's pretty self explanatory, it's used to delete a resource. Now there's a few other ones like patch and options, but we're not going to discuss those right now, they're less common. And we can build a whole app, whole web app with just these four. So going back to express, in the boilerplate code, the get users endpoint just responded with a string. And it said respond with a resource. But for our app, we're going to have our endpoints respond with data in JSON format. JSON is JavaScript object notation. And it looks real similar to a JavaScript object. And it's a first class citizen in both node and front end JavaScript. So it makes it real easy to send data from a front end app, like our Svelte app to a back end JavaScript app, like express. JavaScript, our JSON is so popular, though, that almost every web application framework can handle it pretty easily, whether you're using Java dot net go rust, anything like that.
[07:15 - 07:44] They all have libraries for parsing and handling JavaScript, or excuse me, JSON . So in order to return JSON from our back end express app, we just need to return JavaScript objects or arrays. So let's copy this code here and go back to our express users get endpoint. And we'll paste this in. So all we're doing here is creating a user object.
[07:45 - 07:59] And then we'll return that user object rest, which is short for response dot send user. Okay.
[08:00 - 09:12] So I need to start the back end up. So let me run npm run node mon. Remember, that's our command to start the back end. And now if we try this again, now we get a JSON response . So this is something that we can deal with programmatically in our front end app. Now I have a Chrome plugin that formats JSON years will probably look like this, it will probably be raw, which is fine too. But if you like this formatted JSON, you can find a Chrome plugin that'll make it look a little nicer. So that's it returning JSON from express is very, very simple. You just need to return a JavaScript object and express will do the rest. Let me give you one more example here. We can also return an array. And it will handle that too. So let's say const r equals 123.
[09:13 - 09:51] Now since we have node mon running, we don't need to recycle the back end. So that'll return that array in JSON format. And we could even return an array of objects. So let's say just a real simple object here.
[09:52 - 10:21] And so we'll get that response back in JSON format. That's it for this lesson. So ultimately, we're going to have a back end express app, and it's going to handle data coming from the front end for our school lunch information.
[10:22 - 10:37] And then it'll be able to get that data. We will have post endpoints to create new data, we'll have put endpoints to update data, and then delete endpoints to delete data. So hopefully the big picture of the application is starting to emerge a little bit.
[10:38 - 10:51] In the next lesson, we're going to discuss the school lunch data model. So we 'll kind of bring it back to our application, and we'll continue with express routes and end points.