Svelte 'each' Blocks - Loop Through an Array and Update a DOM
Using Svelte Each Blocks to Iterate a List
This lesson preview is part of the Fullstack Svelte course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack Svelte, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
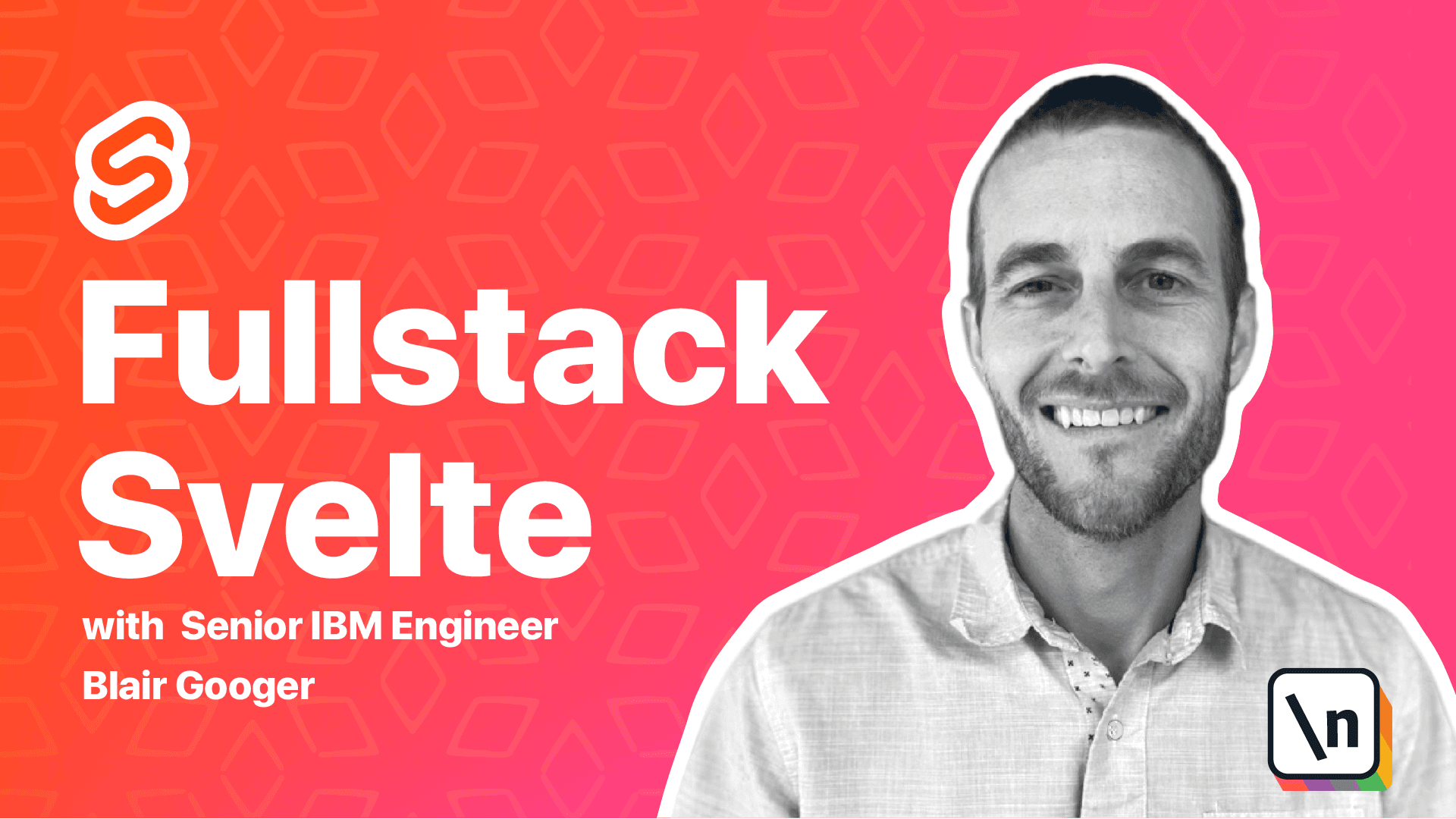
[00:00 - 00:18] Previously, we set up the LunchmanUAdmin component to fetch data from our back end, but we didn't show the data to users in a friendly format. So we just did a JSON stringify, and we're just showing the whole array of objects here on the page.
[00:19 - 00:34] Since we're fetching an array of lunchweek objects, we can use a spelt each block to loop through the array, and spelt will automatically update the DOM with properly rendered HTML. So here's a little example.
[00:35 - 00:49] Imagine we have a list with apple orange in Kiwi. So we can use an each block to iterate that list, and spelt will translate that into the following markup for the browser.
[00:50 - 01:04] So it'll translate that list into an individual li for each item in that list. And so we can use that concept for our lunchweek list in the lunchmanUAdmin.sp elt file.
[01:05 - 01:19] So instead of using an unordered list, like the example above, we'll use a table, and then we'll wrap the each block around the table rows after the header. So let's give this a try.
[01:20 - 01:34] So copy this table. And then in our lunchmanUAdmin.spelt file, where we have the JSON stringify, we 'll leave that alone for a second, and we'll paste in our table.
[01:35 - 01:51] And so what this is going to do here is add a table with a head element to it, and we'll just add column headers there. And then underneath that, we'll loop each item in the array and build a TR for each item.
[01:52 - 02:07] And so we've declared a variable called lunchweek here, and then we can access the properties from that lunchweek using our curly braces inside these TDs. So let's give that a look.
[02:08 - 02:21] Okay, so now you can see that spelt is building this table, and you can just take a look here and see the TRs here. One TR, one table row for each item in our array.
[02:22 - 02:32] So that's looking good. I'll go ahead and take away the JSON stringify part.
[02:33 - 02:53] Okay, so it looks pretty plain so far because we haven't applied the BOMA table class. So let's add class equal table to the table element.
[02:54 - 03:00] So right here at the top, add class equal table. And then that will apply the BOMA styles.
[03:01 - 03:11] So that makes it look a little bit better there. Okay, so that's better, but it's not interactive yet.
[03:12 - 03:36] And what we want to happen is when a user views this data, we want them to be able to click on a given week to drill through, and then view or update the lunch details for that week. So what we're going to do is add a click handler to those rows, and then we're going to route the users to a new component called lunch menu admin details.
[03:37 - 03:45] And the way we're going to do this is eat lunch week object has a lunch week ID property. So one, two, three, et cetera.
[03:46 - 03:57] And we're going to use that property to build a route convention that makes hierarchical sense. So for example, currently we've got admin manage menus.
[03:58 - 04:11] We route that to the lunch menu admin component. So then on this drill through, we'll have admin manage menus, week details, and then the ID of the given week that they're drilling into.
[04:12 - 04:26] And so we're going to dynamically build this as part of that table. And so when a user clicks on this router, navigates to this route, then we'll render a new lunch menu admin details component.
[04:27 - 04:38] And that'll be that detail component where they can view or update the details for a given week. So let's go ahead and create a basic lunch menu admin details file.
[04:39 - 05:00] So we're going to create this under views admin. All we're going to do right now is just import the store and have a little breadcrumb at the top.
[05:01 - 05:13] So that'll be good for now. And then what we're going to do next is add an on click event handler inside that each block.
[05:14 - 05:19] And so this is going to add that handler automatically to each row. So let's copy this here.
[05:20 - 05:32] So we're going to add it at the TR level back in lunch menu admin. So right here at the TR level, we're going to add that.
[05:33 - 05:48] And then we're going to pass down this lunch week object into a function called open lunch week details. So now we need to actually add that function here.
[05:49 - 05:57] So let's copy this part. Add it up to the script.
[05:58 - 06:06] And so what we're going to do is pass in that lunch week object. And then we'll build a route using lunch week dot lunch week ID.
[06:07 - 06:11] So this will be that new route. And then we're going to use programmatic navigation.
[06:12 - 06:26] So this is a navigate to function that we need to import as well. And so this will need to import this navigate to function from spelt router spot.
[06:27 - 06:36] So add that to our imports as well. So just to recap, each TR is going to get a click handler.
[06:37 - 06:58] When the user clicks on that TR, it's going to call our handler function, which is going to do programmatic navigation to this route that we're building right here. OK, so that's kind of ready, but we need to actually add the route to our routes JS file.
[06:59 - 07:20] And this one's going to be a little different because we're going to need to use the convention colon var name because we're passing in a dynamic variable to the routes or passing in that lunch week ID. And so we need to use that special syntax right here.
[07:21 - 07:32] And so we're going to actually use nested routes for this. And so we're going to change this around a little bit so that it looks like this.
[07:33 - 07:40] Let me copy this part. So over in routes JS.
[07:41 - 07:43] We've got nested routes index. So that's fine.
[07:44 - 07:53] That's pointing to kind of the root the route route underneath this path. And so then we're going to add our new one there.
[07:54 - 08:04] So it's going to be this new lunchman you admin details route. I'm going to split this out just to make it more readable.
[08:05 - 08:17] Add a comma there and then add the new one. Get rid of this.
[08:18 - 08:39] OK, so within this nested route, now we're going to have another sub route or nested route with weak details and then the lunch week ID. And then we need to import this component as well.
[08:40 - 08:44] So this is our new component that we just created. OK.
[08:45 - 08:56] OK, so the routing should be ready. So let's give this a try.
[08:57 - 09:05] So now if we click on one of these TRs. So we get if you notice the URL updates and we get routed to the new component.
[09:06 - 09:09] So that looks good. So this one we get ID number two.
[09:10 - 09:16] This one we get ID number three. OK, but the styles don't look very good at this point.
[09:17 - 09:25] So we're going to add a couple of styles here. So we're going to add a class called hasTextLink.
[09:26 - 09:36] And then we're going to add cursor pointer to the TR. So we'll add class equals hasTextLink and then style cursor pointer.
[09:37 - 09:52] I'll copy that part here. So down here on the TR, add those there.
[09:53 - 10:00] And now the cursor turns into a hand pointer there. And the color turns blue.
[10:01 - 10:14] So it's more obvious to the user that this is a clickable element in the app. OK, so that's a basic introduction to each block.
[10:15 - 10:35] So there's a really powerful in web apps for taking an array of objects or just a simple array and iterating those and dynamically building HTML and letting Svelte handle the rest. So Svelte will handle all the reactivity and automatically update the web page based on the data in the array.
[10:36 - 10:45] And so we use that to add an interactive table to our lunch menu admin component. And hopefully you're starting to see the power of Svelte components.
[10:46 - 10:57] In the next lesson, we'll add a loading spinner icon to our on mount function so that we can let the user know that the app is fetching data. Thanks, we'll talk to you next time.