How to Use Axios to Fetch Data From APIs for Svelte Apps
Fetching Data from the Frontend with Axios
This lesson preview is part of the Fullstack Svelte course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack Svelte, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
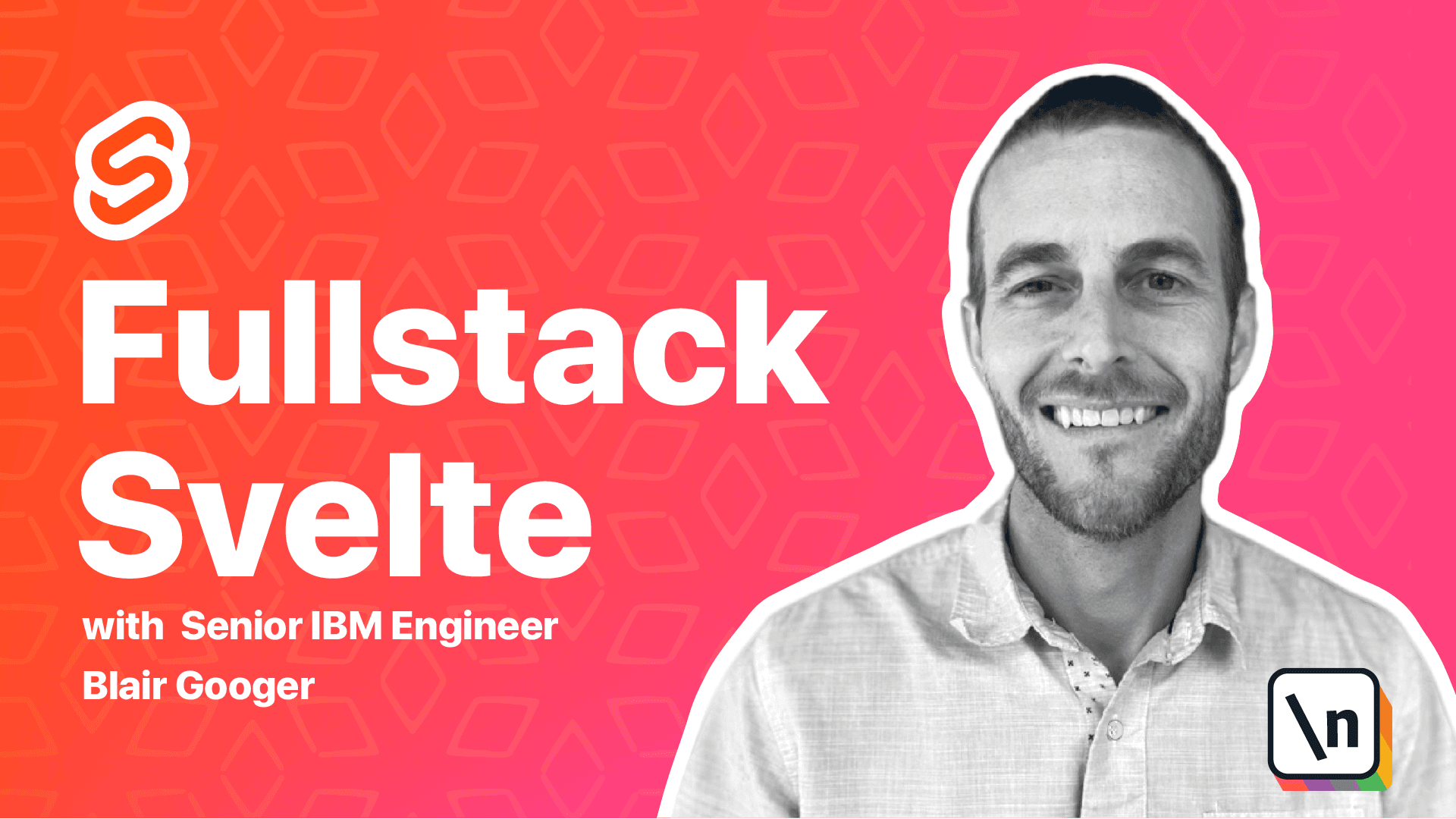
[00:00 - 02:36] Welcome back. We're glad to have you join us for another lesson. In the last lesson we worked on the Backend Express application and we created some REST API endpoints and in this lesson we're going to learn how to use our front end spelt application to call those back end endpoints and fetch some data. So a common use case in web apps is a user navigates to a route in the app. The component for that route needs data from a server so the component will show a loading spinner icon to let the user know that something is happening. Then the component triggers a network call to the server to fetch the data and when the data returns the loading spinner is hidden and the user interface is updated with the data. So there's a lot going on in those steps but smelt spelt makes it pretty easy to dynamically update the UI with the spinner icons and the data just through reactivity and then we'll also use a library called Axios that makes HTTP network calls in JavaScript and makes that part a little easier. So we're going to follow the above outline and fetch our lunch week list from the back end server and then display that in the front end. We'll take it piece by piece here so we're going to start just by displaying a simple list that will hard code in the front end. So we're going to work in lunch menu admin.spelt. So open up that file and then we'll put in this simple list lunch week list and we're just going to hard code a couple values in there and then we'll use our must-brackets or curly brackets to just display that list. This is kind of just a review of state and reactivity. So make sure that your front end application is running if not it's npm run dev to start it and then it's going to be on local host 5000. So here's my lunch week admin component and you can see that it's showing test one and test two so showing the two values from my array there. So that's just one little start there and so this is basically what it looks like.
[02:37 - 08:40] Now let's go one step further. So previously we talked about the on-mount life cycle function and on-mount is a good place for a component to initialize itself and this is a good spot to do something like fetching data from a server. So we're going to add on-mount in here and then we'll set the lunch week variable to an empty array and then in the on-mount function we'll actually set it to a value. Now eventually in a minute we're going to fetch this down from a server but we're getting there piece by piece. So let's add the on-mount life cycle function there and then we're gonna say on-mount a sink and then inside there we'll actually set the lunch week list but we need to initialize it first so let's move this up here. Okay so now when the component's ready and on- mounted we'll actually set that list to a value. So give that a try and you can see with the hot loading that's updated there so the on-mount life cycle functions working. Okay so now let's talk about Axios. So the Axios library gives us a pretty convenient syntax to fetch data from an API. So we import Axios and then we can call Axios git and then we put the API route and then the response from that call will contain the JSON from the REST API. So in this case we say response.data and that will contain the JSON. Okay so that looks pretty easy but there's a lot going on there and there's some complexity lurking under the covers and one important concept with the above code is the usage of async await syntax. So we've got the async keyword before our function signature and then that allows us to await asynchronous calls and you may have heard of promises this is a modern way of dealing with promises and so what async await does in this case is that it blocks until this call is finished before moving on to the next line of code. So here's an example of some similar code but without the await keyword. So what would happen if we set up our code this way is the Axios git call would fire and that would run in the background and then immediately the JavaScript runtime would console log response which would be empty because it won't block it won't wait until this call is finished. So you got to be careful and make sure when dealing with asynchronous network calls to use the await keyword. Okay so let's go ahead and install Axios in the front end project. So I'm going to stop the front end dev environment npm install Axios and then start it up again. Okay and then let's go ahead and import it and we'll use it to fetch from our API. So import Axios here and then we're gonna assign response.data to our lunchweek list variable. Since we're dealing with a network call and something that's going over a network to a server this could have a failure point in it so it's a good idea to wrap this with a try catch that way we can deal with errors more gracefully. So for example we might want to show our users an error message that says error fetching data from server or something like that. So we'll add a try catch to it as well. For now we'll just console log the error if we get one. Okay so let's give this a try now we're just hard coding our back end route for now and we'll we'll deal with externalizing that route the local host 3000 part later. So this will just get us to where we're going for now. So let's give this a try and it looks like it's not working so let's take a look at dev tools here and look at the console let's look at the network calls so if we refresh this and if you choose XHR this will show all the API rest API calls and so this is this is failing right now I don't think I have my back end running and so this is probably gonna happen to you you'll either have your front end not running or your back end not running but we need to run them both. So from here out in this course we're gonna mostly be running both at the same time.
[08:41 - 10:23] So I'll just start a new browser tab here and go to my workspace in my school lunch directory and then go to back end and then we want to run npm run node mon that's how we start our back end. Okay so now refresh this and give it another try and it's still failing there and what you can see is that our request has been blocked by core's policy. No access control allow or origin header is present so as I was building this course I encountered the same error and I decided that it might be neat to just work through this as we got the error together because this is kind of something that would commonly happen to you in the real world. So this error deals with cross origin resource sharing and this error prevents a web page on one domain in our case localhost 5000 from originating a request to a resource on another domain in this case localhost 3000. So maybe another example would be imagine we owned the domain great email dot com and we tried to fetch data from a domain that we didn't own mail dot Google dot com and in that case Google's server side course policy would probably kick in and prevent that from working.
[10:24 - 12:29] But for our case we own both the front end and the back end for our school lunch application and so we can set up a course policy in express to allow certain types of cross origin requests. So we're gonna switch gears and put our back end hat on and deal with this problem now. So firstly we need to NPM install cores in the back end project. So since both our front end and back end or JavaScript got to be careful that you make sure you're in the right place at the right time. So I'm in the back end directory here and I'll do NPM install cores. Okay and then we're gonna update app.js to add the cores middleware. So add this piece in there. So here's app.js for the back end. And I think we should need just these two pieces. So under where app is declared we'll add those two pieces. So there's another little complexity here. Certain cores requests such as post put in delete are considered complex and they require an initial an additional options request called the pre flight request. And so that's why we need this app.options star cores. And we're gonna later on need post put in delete. So we'll go ahead and put it in there now. And so here's a look at the full app.js with the cores updates. So I think this will get us past that error . Go ahead and start the back end up again NPM run nodemon.
[12:30 - 13:50] And then refresh this page again and we'll see what happens. Cool. So now go look at the network tab and you'll see that we got a 200 OK status. And if you click on this and go to preview you can see the JSON that was returned from the server. Now when we bound that list to Svelte it just shows it as object object. So it 's not going to directly show a list of objects on the screen. I'll show you one more technique you can use if you do want to see the contents of those objects and have Svelte render it onto the web page. So back in our Svelte file inside the curly braces we can run any JavaScript we want. So we could run JSON dot string ify and stringify that list. And when we do that Svelte will render it onto the page so we can actually see the contents of those lists. Now of course this is just for testing and we'll clean this up in the next lesson. So that's it for this one.
[13:51 - 14:33] We learned about the on mount lifecycle function and we installed Axios and discussed async await syntax. And then we even had to troubleshoot a cores issue to get our front end to be able to make network calls to the back end. So in the next lesson we're going to talk about Svelte each blocks and we'll use those to iterate through our lunch week list. And then we'll use that to update the user interface and show more organized data instead of just object object or JSON stringifying that data. So thank you for joining us for this lesson and we'll see you next time.