School Lunch Data Model
School Lunch Data Model
This lesson preview is part of the Fullstack Svelte course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack Svelte, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
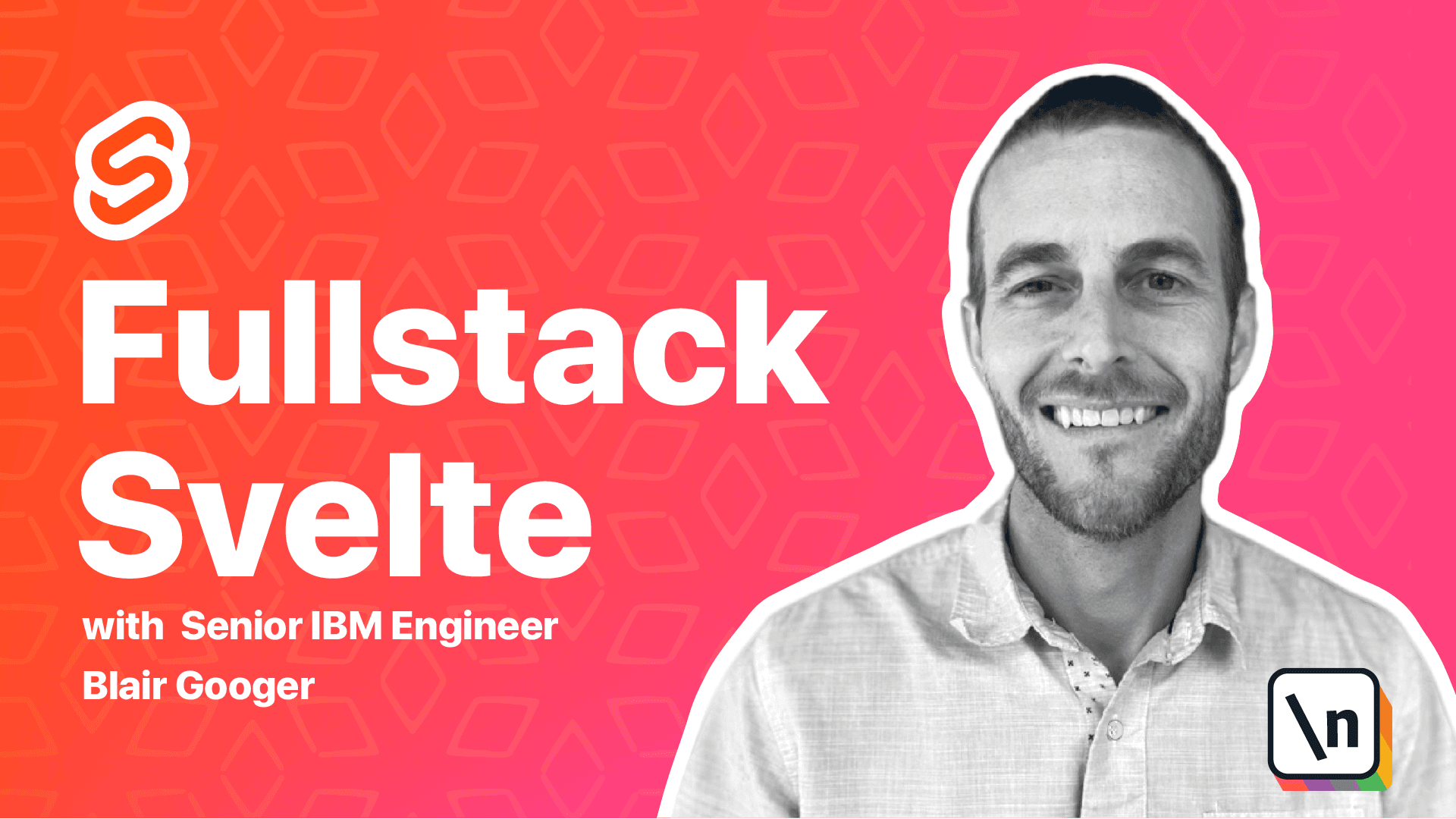
[00:00 - 00:08] In the last lesson, we talked just about concepts. We talked about REST APIs and endpoints.
[00:09 - 00:23] In this lesson, we need to actually bring it back to our application and talk about our application's data model. So the purpose of our application is to allow registered users to create and publish a weekly school lunch menu.
[00:24 - 00:41] So in order to accomplish that, we're going to need a lunch week entity that will have a list of lunch day entities for each school day. So just imagine the data that we're going to need and this is kind of the model that we'll use to record that data.
[00:42 - 00:52] The lunch week entity, it'll have a lunch week ID so we can track unique lunch weeks. It'll have a week of property and that'll be the start date of the school week.
[00:53 - 01:04] That'll be a date property. It'll have an is published property and that will denote whether or not the week is finally published or if it's in a draft status.
[01:05 - 01:13] And then we'll have an array of lunch day's child objects. So the lunch day object will have a lunch day ID.
[01:14 - 01:25] That's just a primary key for tracking and we'll talk about that a little bit later when we talk about persistence. Then it'll have the lunch week ID of the parent.
[01:26 - 01:36] It'll have the day which will be the date of the given lunch day and then it'll have the menu details. So what's being served for that school lunch for that day.
[01:37 - 01:49] So after modeling, here's a representative JavaScript object example that puts it all together. So we've got a main lunch week object at the top.
[01:50 - 01:53] It's got an ID. It's got the week and whether or not it's published.
[01:54 - 01:57] And then it's got a couple of lunch days. So normally there would be five of these.
[01:58 - 02:06] In this example, there's only two. And so each of these lunch day objects has a parent lunch week ID.
[02:07 - 02:20] So both of those are one because the parent is one in both of these cases and then it has its own unique ID. And then it's got the day and the menu details for that day.
[02:21 - 02:26] So we're kind of keeping it simple. Our menu details property is just a single string.
[02:27 - 02:40] We might discover later that we need more ways to subdivide it. Maybe we need to have the snack, the entree and a drink and kind of subdivide that into three different fields.
[02:41 - 02:46] But I think at this point it's a lot better to keep those separate. It's easy enough to add things later.
[02:47 - 02:49] Something small like that. So we'll keep it simple for now.
[02:50 - 03:05] And we'll defer that decision until later. The next thing we need to tackle is creating the lunch week route in Express so that we can handle the create, read, update and delete operations.
[03:06 - 03:17] Create, read, update, delete is oftentimes just known by the acronym CRUD. So we're going to handle the CRUD operations for those entities.
[03:18 - 03:40] So we can start off by creating a file called lunchweek.js in our routes directory. So over in VS code, make sure you're under the back end and create a new file under the routes directory, lunchweek.js.
[03:41 - 03:53] And we'll go ahead and just stub out an empty, get, well not an empty, but a basic get endpoint. Okay.
[03:54 - 04:09] So once we have that route file in place, we need to import that route into app .js and then update it to include that in the Express routing middleware. So we need to import it.
[04:10 - 04:18] So we'll copy this statement right here. Now on back end node applications, we're importing with require syntax.
[04:19 - 04:32] So this is similar to using the import syntax that we did on the front end spell application. So find app.js and put this near the other routers.
[04:33 - 04:44] Now I haven't modified this file yet. So you see the semicolons, as soon as I hit save, my prettier JS formatting is going to kick in and it's going to remove those semicolons.
[04:45 - 04:54] And you can also notice that this lunchweek router is kind of grade color. That's because I have this variable in here, but I haven't actually used it anywhere.
[04:55 - 05:15] And so it's just a nice formatting thing that VS code is letting me know I haven't used this. So we imported it above and then we need to use it right here and that will wire it up into the Express app.
[05:16 - 05:27] Okay. So we can go over to the browser and give that a try.
[05:28 - 05:53] Okay, so we just see our static string response that says lunchweek data at the lunch week or out. So eventually we're going to have a Postgres database and we're going to put our lunchweek data into that database.
[05:54 - 06:01] So we'll store it in there, we'll retrieve it back out when we need to respond to a Git request. But for now we'll just hard code it.
[06:02 - 06:13] And obviously this won't work in production, but I think it'll help us learn some of the concepts faster. So copy this list right here.
[06:14 - 06:20] Go back to the lunchweek route file. We'll just add it at the top as a global variable here.
[06:21 - 06:38] And then we'll return this lunchweek list. So that's going to return JSON and it's going to return this array of lunch weeks.
[06:39 - 06:40] Give that a try. Okay.
[06:41 - 06:47] So there's our response there. Okay.
[06:48 - 06:56] Express dynamic routes. So we're going to have a couple of different routes here.
[06:57 - 07:14] We're going to have a route that returns a list of all the lunch weeks and then we'll have a route that will return just the individual lunchweek. And we'll use the ID number to find that individual lunchweek.
[07:15 - 07:22] Okay. And in order to accomplish that, we need to use dynamic routes.
[07:23 - 07:38] So back end routing, we can do that with the convention semicolor, excuse me, colon and then variable name. So we're going to create another route in the lunchweek router.
[07:39 - 07:52] And we'll return the details of a specific lunchweek based on the lunchweek ID parameter. So copy this over.
[07:53 - 08:12] Paste this below. So both of these endpoints can live in the same file and they're not going to conflict with each other because one is just lunchweek get slash lunchweek and the other one will be get slash lunchweek slash ID number.
[08:13 - 08:20] Let's look at this code a little bit. So when parameters come in, express treats them as strings.
[08:21 - 08:29] So in some cases, you might need to convert them to a different type. So we're going to convert this lunchweek ID into an integer.
[08:30 - 08:42] And the way we access it is via rec.perams.peramid. So we'll convert rec.perams.lunchweekid to an integer.
[08:43 - 08:54] And then we've got, so imagine that we've got the ID to passed in. We need to find the object in this array that's identified by lunchweek ID to.
[08:55 - 09:18] And so we can use the JavaScript array.prototype.find method and we'll find the first matching entity. So we'll do lunchweek list dot find where lunchweek ID from the object inside the list equals the ID that was passed in.
[09:19 - 09:31] And then it's a good idea to do some error checking and error handling in the back end endpoints. So we'll check and make sure that this is populated, that this is not a fault C variable.
[09:32 - 09:41] And so if it's not faulty, then we'll return the one we found. Otherwise we'll return a 404 not found status.
[09:42 - 09:52] Okay, so let's give this a try. So now we should be able to say slash two.
[09:53 - 10:00] And then we get just that one object from this endpoint. And these Chrome dev tools are really nice.
[10:01 - 10:07] So you can also see information about the network call here. So you can see that it was a git request.
[10:08 - 10:18] You can see the URL that was used. You can even hit preview and see the JSON response and then hit response and see the raw response.
[10:19 - 10:25] So if you remember, we only have three options in our list. So let's say we tried four.
[10:26 - 10:32] Now this ID doesn't exist. So if we do that, we're going to get our 404 error code.
[10:33 - 10:36] And you can see it over here. It says 404 not found.
[10:37 - 10:55] So this is our error handling and it lets the caller know that that resource doesn't exist. So this section here is talking about Chrome dev tools, which we've already been using and we've covered quite a lot.
[10:56 - 11:04] So let's tackle one more thing before we finish this module. Our routes right now don't say that they're API routes.
[11:05 - 11:20] And this could be a little bit confusing and it might even conflict with say a route that returns HTML. So we're going to add an API prefix, just the word API to our routes.
[11:21 - 11:34] So we can do this by making a few changes in app.js. Right now we have lunch week router equals require the route and then app.use the route.
[11:35 - 12:00] So we're going to change that to bar router equals express router, then we'll use router.use lunch week router and then we'll use API router.use app.use API router. So it kind of layers the pieces together.
[12:01 - 12:34] So let's see if we can change this piecemeal and we'll be able to track what we 're doing a little bit better. So we're going to declare a new router here and then we'll say router.use router.use and then we'll say app.use/api router.
[12:35 - 12:48] Let's give that a try. We're going to go back to one that actually exists.
[12:49 - 13:06] So now our routes, all of our endpoint, all of our API endpoint routes will now have the prefix API in them. This is kind of just a nice to have but it helps clearly distinguish that this is an API route that's going to return JSON.
[13:07 - 13:22] All right. So we've got our back end application coming together now and we created some API endpoints that serve JSON based on our lunch week data model.
[13:23 - 13:36] So hopefully everything's starting to make more and more sense. And we're going to switch back to the front end for the next module and we're going to learn how to fetch data from our Svelte front end.
[13:37 - 13:44] We're going to trigger fetches from our Svelte front end and pull that data down from the express back end. So we look forward to seeing you next time.