React Hooks - Understanding The Basic Rules and Benefits
Learn about the motivation behind React Hooks, the benefits it can bring, and the rules that apply to all Hooks in a React application.
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the The newline Guide to Modernizing an Enterprise React App course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Modernizing an Enterprise React App, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
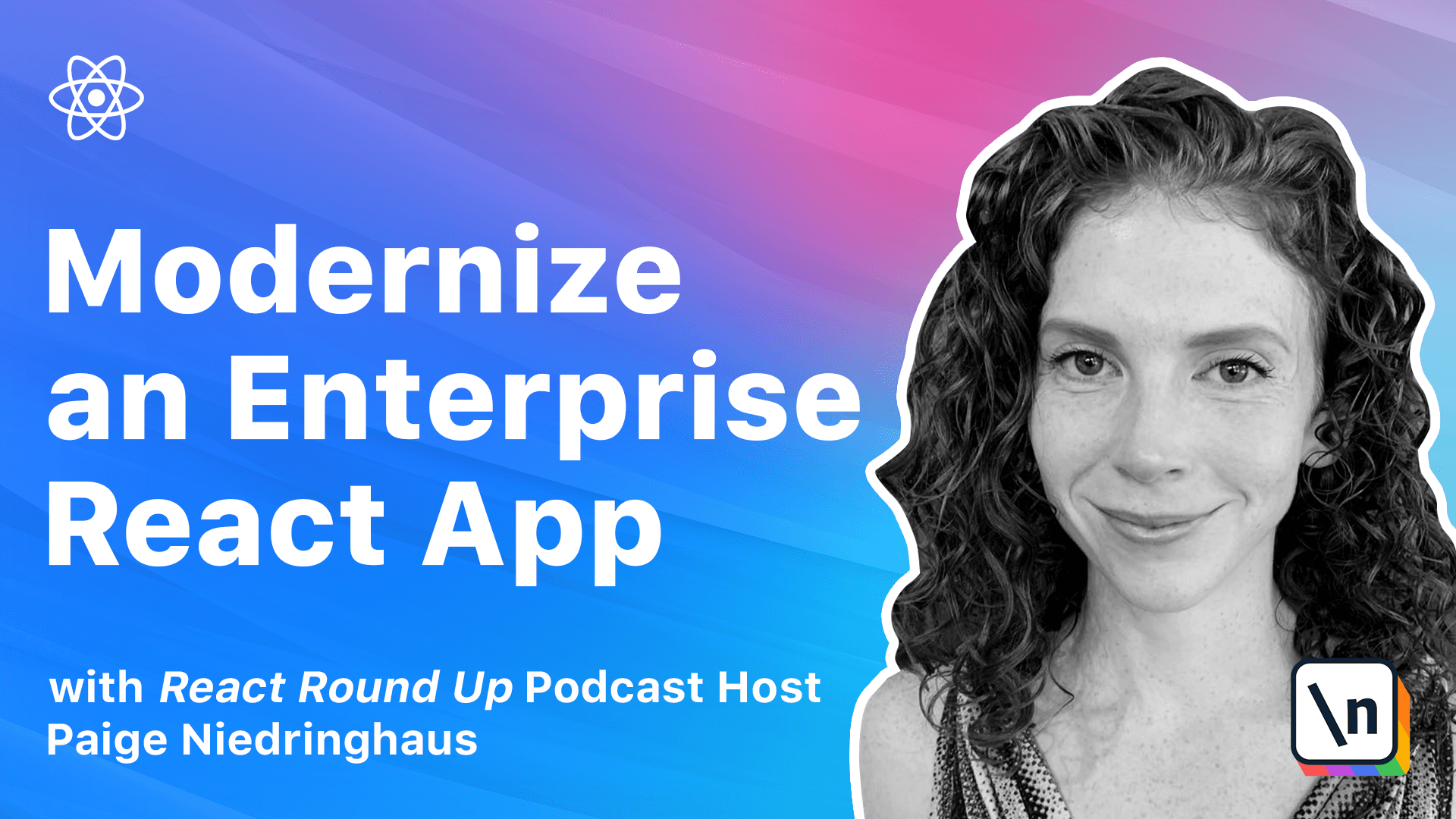
[00:00 - 00:16] Conceptually, React components have always been closer to functions. The React team freely admits this, but before Hooks arrived, these functional components, while more in line with the spirit of React, had limitations, most notably an inability to have their own state.
[00:17 - 00:26] The 4 React 16.8 was released in order to handle state or features like lif ecycle methods. Component did mount, component will update, etc.
[00:27 - 00:41] Then React, the component utilizing these things had to be class-based. And so, we had the stateful, class-based components that were responsible for fetching data and keeping track of state, often referred to as container components.
[00:42 - 00:54] And the stateless functional components responsible for receiving data from those class components and displaying it were known as "presentational components." React Hooks turned that idea on its head, and with good reason.
[00:55 - 01:09] This lesson will dive into why Hooks were introduced to React to help us better understand the problems Hooks are designed to solve while building applications. So the first question to ask yourself is why Hooks?
[01:10 - 01:23] Why go to the trouble of basically changing the way that we have been building React up until this point? You know, class components seem to have done relatively well for us, so why should I even bother?
[01:24 - 01:42] And the motivation is kind of, it's multifaceted. But as the React docs themselves put it, the motivation behind Hooks was to solve a wide variety of seemingly unconnected problems in React that we've encountered over five years of writing and maintaining tens of thousands of components.
[01:43 - 01:57] This was from the React team themselves. This was from Facebook saying, "Hey, we've found some issues which people talk about pretty regularly or talked about, I should say, pretty regularly on Stack Over flow, on GitHub issues.
[01:58 - 02:03] There were pain points with React. There still are pain points with React, but it's continuing to improve."
[02:04 - 02:19] So although there are no plans to remove classes from React, backwards compatibility has been important from day one, and for that we are all grateful. Hooks provide a more direct API to react concepts that you're probably already familiar with.
[02:20 - 02:37] Apps, state, context, refs, life cycles, those things that you already know and use in one way or another, regardless of if you're using Hooks or if you're using class components. So if you reread that quote from above, you might think to yourself problems.
[02:38 - 02:48] What sorts of unconnected problems is the React team referring to? Well, you've probably encountered some of these problems when you stop and think about it if you've been working with React for any length of time.
[02:49 - 03:04] Things might include things like complex components that become hard to understand. As stateful components grow more complex, lifecycle methods that started out rather simple become unmanageable messes of stateful logic inside effects.
[03:05 - 03:22] And when I talk about side effects in React, I am referring to any function that modifies a variable, a state, or data that is outside of the scope of the current function that's being executed. This is also known as an impure function if you are familiar with functional programming concepts.
[03:23 - 03:31] To put that into an example, if a function modifies a global variable, it's considered a side effect. If it makes a network call, that's also a side effect.
[03:32 - 03:45] Data fetching is a side effect in React, which explains why we see references to side effects in React most often with lifecycle methods and now with the use effect hook. Now that hooks have come into Vogue.
[03:46 - 04:01] That's what I mean when I talk about side effects. So it's not uncommon for a component fetching data inside of a component did mount method to also contain unrelated logic that maybe sets up event listeners or references to DOM nodes.
[04:02 - 04:10] Likewise in the component will unmount a completely separate method. It would be responsible for cleanup of the same event listeners when the component unmounts.
[04:11 - 04:26] Mutually related code that changes together gets split apart but unrelated code and is unchammed together because of these lifecycle methods that we have to follow with class-based components. This is a recipe for bugs.
[04:27 - 04:36] It's a recipe for inconsistency. This just makes it harder to understand what is this component actually doing or what are all of the things that it's doing.
[04:37 - 05:01] So hooks let you split one large stateful component into smaller functions based on what pieces are related rather than forcing a split based on lifecycle methods. This is a huge, huge improvement and you're going to see as we get into examples and we start doing our own refactoring just how much simpler this makes some things with React.
[05:02 - 05:16] Another issue that a lot of people have brought up is that classes are confusing to both people and machines. Classes which are relatively new to JavaScript as a language are a barrier to learning React.
[05:17 - 05:32] Even though they've been around since ES6 which was introduced back in 2015, I haven't seen them widely used in the JavaScript community outside of React. With classes things like how this keyword works in JavaScript is important to understand.
[05:33 - 05:51] Going to bind event handlers has to happen and while people might well understand props, state and the top down unidirectional data flow of React, they can still struggle with wrapping their head around classes. Even experienced developers have problems with classes sometimes.
[05:52 - 06:12] And even the distinction about when to use function based components or class based components could lead to disagreements between experienced developers. On top of all, React is committed to staying relevant with the other options available today like view, angular, spelled and the team found that class based components can encourage unintentional patterns.
[06:13 - 06:25] The code doesn't minify so well and it makes hot reloading which is one of my favorite benefits of React flaky and unreliable. Whereas hooks let you use more of React's features without classes.
[06:26 - 06:43] Hooks embrace functions while still providing a good development experience that doesn't require UBA functional programming or reactive programming master. That is the main thing is that hooks are easier to use once you start to understand how they're supposed to be used.
[06:44 - 07:04] So the last thing that a lot of people pointed to when they were talking about why hooks is hard to re-use stateful logic between components when you're talking about class based components. For hooks React didn't offer a straightforward way to have reusable behavior within a component.
[07:05 - 07:23] Even though both a component did mount and a component should update method might call the same API endpoint at different times, there was no good way to prevent dupl icating that same code for each lifecycle method. So the principle of dry code or do not repeat yourself was being violated even within the same component.
[07:24 - 07:36] Patterns like render props and higher order components attempted to solve this, but they might require restructuring components and they tend to make the code harder to follow. To the React core team this indicated an underlying problem.
[07:37 - 07:50] React needed a better primitive for sharing stateful logic. So hooks introduces the ability to extract stateful logic from a component so it can be tested independently and re-used without changing your component hierarchy.
[07:51 - 08:06] This makes it easier to share hooks among many components within a project or even within the larger development community. So now that we've talked a little bit about why hooks, let's talk about some of the rules of hooks and the problems that they aim to solve.
[08:07 - 08:35] So if these rules are violated, don't worry, you will know immediately your console and your browser will light up with errors and the app will probably break while you're doing local development when this happens. So just try to keep them in mind as we look at various hook examples in the next few lessons and all of these rules that I'm going to go over might not immediately make sense, but once we begin upgrading our own application, you'll get a better feel for these rules and how to work with hooks to avoid breaking them.
[08:36 - 08:51] So all of these rules were taken directly from the React documentation themselves. I linked to the rules of hooks if you ever want to check the docs and get more information about them, but this is where you can find them.
[08:52 - 09:08] So the first rule, only call hooks at the top level, don't call them inside loops, conditions or nested function. So following this rule ensures that hooks are always called in the same order each time a component renders and this is incredibly important for React.
[09:09 - 09:19] React relies on the order in which these hooks are called. So if a hook was nested inside of an if statement, it might not run on every render, which could lead to very hard to locate bugs.
[09:20 - 09:34] One solution, if you do need to have some sort of a conditional with a hook, would be to put the if statement inside of the hook, but you cannot do it the other way around, React does not stand for that. The next rule, only call hooks from React functions or functional components.
[09:35 - 09:47] You cannot call them from regular JavaScript functions or class-based components, it just doesn't work. So following this rule ensures that all stateful logic in a component is clearly visible from its source code.
[09:48 - 10:01] And then the third rule is that custom hooks are functions that call other hooks. This takes a little bit of time to get your head around, but as a best practice , custom hooks should always be denoted with the term use before the hook name.
[10:02 - 10:18] So if you had a custom hook that was involved with your products, you might call it use products, or if you had a custom hook that was just for running intervals within your application, you might call it use interval. So it's just use something and that's the name of your hook.
[10:19 - 10:34] We will definitely be doing some work with our own custom hooks later on. So in the next lesson, we'll dive deep into the use state hook, the first and most frequently used hook, and the closest to the state you already know from class-based components.
[10:35 - 10:35] That's coming up next.