What We'll Build In This React Course - Hardware Handler
Get familiar with the sample React application's folder structure and current functionality.
This lesson preview is part of the The newline Guide to Modernizing an Enterprise React App course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Modernizing an Enterprise React App, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
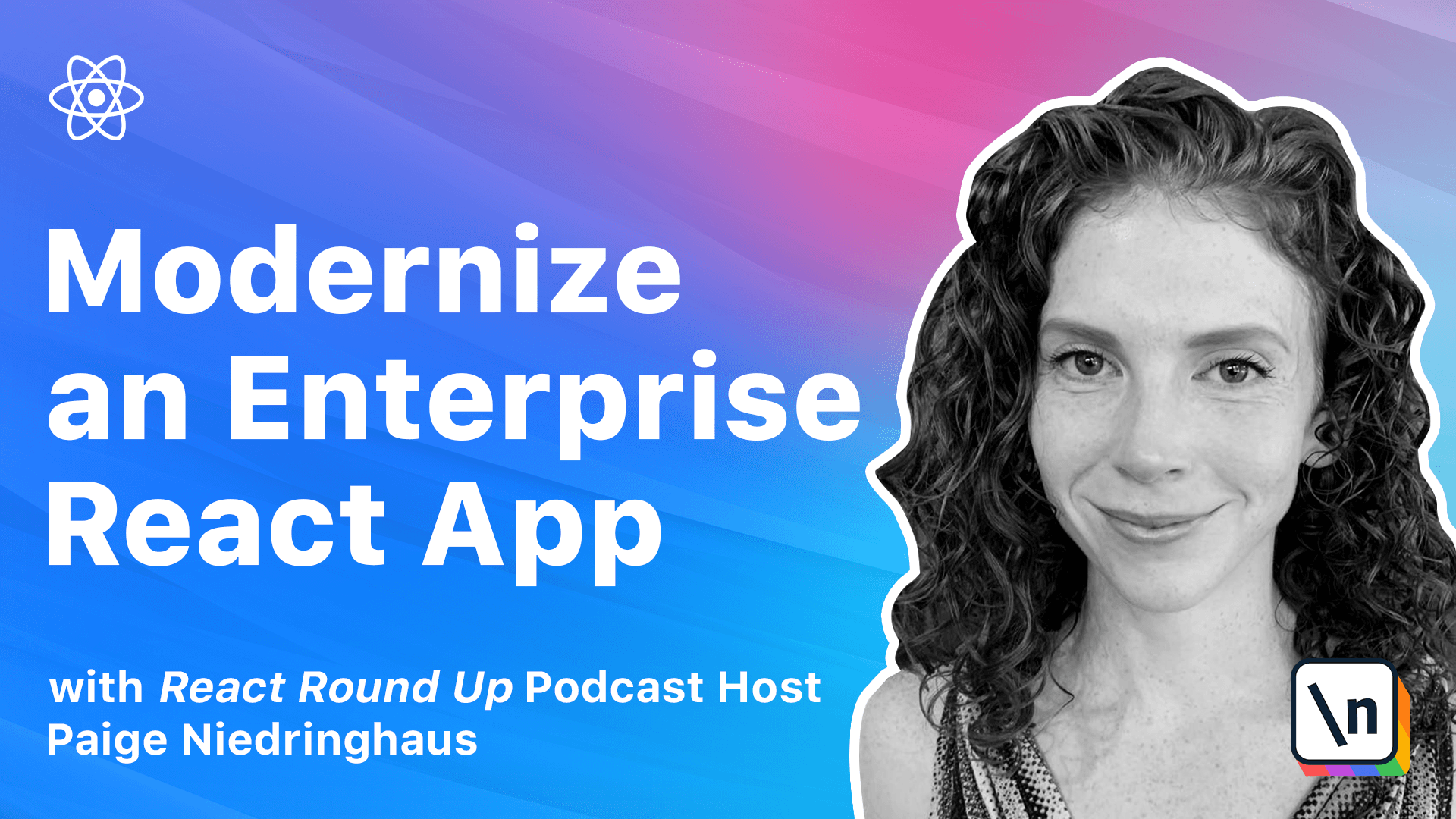
[00:00 - 00:18] Before diving into how hardware handlers built, let's take a quick tour through the application itself. This application is a sample react app built to help product managers within retail companies manage their product disorments, which are sent to retail stores to sell to customers.
[00:19 - 00:38] Okay, now that we have hardware handler up and running, we are going to talk a little bit about how it works currently and how it is structured under the hood. So let's get right to that. When the hardware handler application first starts up, the first page that a user will see is the home page.
[00:39 - 01:07] This home page links to all of the other pages of the app and displays any items that are already present in the checkout, which you can see right up here in the corner. These pages are where a product manager in this theoretical application of ours would actually go about the business of reviewing products, adding new ones to the assortment that they manage, and looking at the list they intend to potentially ship to the stores that they are helping to supply.
[01:08 - 01:21] So let's look at the very first page, the My Products page, by clicking either the button here or the link in the nav bar. We'll just click the button for now. So this brings the user to the product page.
[01:22 - 01:39] And the first thing that we will see is users can view products that they already manage. They can filter down by name or by department. So if we click Hand Tools, we see that only the tools or the products that our hand tools are visible.
[01:40 - 01:54] Additionally, they can filter by product name. So if we add bubble scrubbers or Momo, we get more products that way. We can remove that. Basically, it is just it's a nice filtering option for our users.
[01:55 - 02:17] Unlike a typical e-commerce site though, once they have added the product once to their checkout, like this, they will see an error if they try to add it a second time. And the reasoning behind this is that typically there is more than one connected system that a product manager would be using to keep track of the products they were sending to stores.
[02:18 - 02:36] And that would be outside of the scope of this course. So they would be responsible for coordinating all the details to get the products into the stores in that downstream system. That would be where they would do things like pricing the size of the products that they were placing in stores and how many products exactly they would be shipping.
[02:37 - 02:54] So that's why they only had the option to potentially pick once and put it into their checkout. And we can see up here that every time they add something, there's a little success or error message as well as the checkout count gets updated.
[02:55 - 03:01] So add that. We will see that now they have three items in their checkout.
[03:02 - 03:11] So let's move on to the Add New Products tab. So this is a page where a product manager can add new products to the list of products that they manage.
[03:12 - 03:24] They can select from a predetermined list of departments, visible here. They can add a product name, they can add a brand for their product, they can add a description, and they can add a retail price.
[03:25 - 03:39] Once all of those things have been added, this submit button, which is currently disabled, will become enabled, and that product will be added. And then they will show up. It will show up on the My Products page.
[03:40 - 03:58] The last page is the checkout page, and this is what it sounds like. It's a summary of all the products the product manager has added to his or her checkout. It displays all of the relevant product information, suggested retail price of an individual product, and the ability to remove that product from the checkout .
[03:59 - 04:09] Just like that. In a real-world scenario, a product manager would most likely send this summary of products to another system further down in the ordering and product replen ishment process.
[04:10 - 04:26] And within that system, a product manager might determine exactly how many units of each product deliver to retail stores, as well as exact dates to ship to those stores. But as I mentioned above, all that is outside the scope of this course, so the checkout page in the summary is where this application's functionality concludes.
[04:27 - 04:36] Now, one more thing that I would like to point out is the toasts, because we saw a few of those. That's what these little guys are. They are called toasts.
[04:37 - 04:52] So in addition to the main pages and their functionality, there are these disappearing messages that are present throughout the application. These messages can alert a product manager when actions they attempt to perform , like adding new products to the system or removing items from the checkout are successful or not.
[04:53 - 05:09] That's their main purpose, and they are predetermined to disappear after a certain amount of time, unless you hover over them like I'm doing right now to show you. So if we watch that little countdown bar goes down, and then the toast disappears all on its own.
[05:10 - 05:24] So now that we've talked about how hardware handler works, let's talk about how it's structured in the application. So we're back in VS code, and we're looking at how our code is actually built.
[05:25 - 05:45] As I said in the first lesson, this course is focused on modernizing an enterprise-style React application. It is built in the style of a full stack app, a root folder that holds both of the client folder in charge of our React views, and a server folder, which contains our Node.js express API.
[05:46 - 05:56] The only files that are present are a package JSON, a DB.org.json, and a DB. json, as well as a couple of Node modules.
[05:57 - 06:14] So this folder exists entirely to provide data courtesy of the JSON server and PM package, which you can see right here. To simulate the CRUD functionality that would be present, if the application was connecting to multiple REST API microservices, which is how it would normally go.
[06:15 - 06:32] So when I say CRUD, I mean create, read, update, delete, the typical things that you would want to do to data in a database somewhere. So the JSON server just provides a very quick back end for prototyping or mocking, or in this case, powering a sample application.
[06:33 - 07:03] And once the server folders JSON server starts up, the DB.eridge.json file is populated with initial data, and that's loaded into the app, so that when we're actually in the application, that's where we see these products coming from. Those are all coming from our server. And then once we have this, the DB.json is a local database file that is created each time the application starts up, and it keeps track of any updates that are made.
[07:04 - 07:39] So you know that we have one product still in our checkout, and if we look here , the DB or Ridge JSON, there's no products in our checkout, but if we look at this guy, let's scroll down to the DB.json. We see that there is actually one product in our checkout, so that is how it's being updated while the application is running, but every time the application shuts down and the server turns off, this is lost, and a fresh one is spun up based on what is in here the next time around. On to the good part, the client folder. This is the stuff that we really care about.
[07:40 - 08:00] This is where the bulk of this course focuses on. So let's open this up, and let's take a quick peek at some of the folders that we have. So if you've ever used Create React app before, you are probably pretty familiar with this. This front end is built off an older version of Create React app.
[08:01 - 08:22] If we look back here into our package JSON, we will see that we're running React 16, and we are running React scripts of 1.1.4. We are up to four, possibly five, or the Create React app scripts now, so we are quite a ways away from being current.
[08:23 - 08:45] But that's okay. So all of these versions of React are pre-react hooks, which were introduced with the release of React 16.8.0. And the code structure, which I will describe briefly below, provides an example of how a larger React application could be structured for code maintain ability and scalability as the application grows.
[08:46 - 09:04] It's a could, it's not a must. Everything that I say in this course is going to be a could, not a must, because you have to find what works best for you. With that being said, the source folder is where everything lives. It is the main star, it is where we will be doing the bulk of our work.
[09:05 - 09:21] These are some of the folders inside of source that we will be focusing on. So we'll start in the containers because those are the largest pieces of our application. And the majority of where our stateful components are filed are ease of access.
[09:22 - 09:37] State lives in class components in the source containers folders for now. So if we look for instance at our app, we will see that the main app.js file has a little bit of state, it is a class component.
[09:38 - 10:19] The line of delineation between where state should live becomes much less clear with the introduction of React hooks and the ability to have state almost anywhere within an application because of folks. But regardless, I'm still a fan of larger page style components, living in some sort of pages folder or containers folder and smaller components being injected into these larger components and living in another folder, most likely labeled components, or something along those lines. As we continue our tour into the components folder , we will see that the components folder are basically view based functional components.
[10:20 - 10:53] They don't have any state of their own, they are passed the props that they need in order to make their displays. The same comment that I made about the class based component section applies here too. But like I said, the idea of smaller components that might be useful to reuse and multiple larger container components being stored inside of a components folder is appealing to me and seems less tied to a specific page of the application unless it is very specific to a particular piece of the application.
[10:54 - 11:15] But for something like a loader or an item, those kinds of things are probably going to be reused in multiple places throughout your application. So it probably makes sense to put them into smaller reusable formats and not tie them to a particular container, but more to a generic component folder.
[11:16 - 11:33] So the next folder that we will look at is the services folder. And to me, the services folder is where all of our API calls should live. The data fetching calls that utilize the popular, promise-based HTTP library Ax ios, which you can see at work right here.
[11:34 - 11:59] These are all stored in the services folder. Keeping all the data fetching together makes it easier to keep track of and update these functions. For example, even if two or three or more components are calling the same API endpoint, by having only one API call that all the components rely on, you can easily change that one API call and know that the data supplied to all the components will change in the same way.
[12:00 - 12:16] So this is just one way to keep it more organized. And finally, the last thing that we will look at are the helpers folder and the constants folder. The helpers contains a few helper functions and constants stored in their respective folders that are invoked throughout the application.
[12:17 - 12:41] So the helpers folder has plain JavaScript functions that have no JSX or React- specific data. An example might be a function that takes in a number and formats it to be displayed like a USD constants serve a similar purpose. They are typically strings to indicate that something like a message that might be referenced or checked in multiple places throughout the application.
[12:42 - 13:07] So instead of re-typing and potentially mistyping the same string in multiple locations, like to check if a particular error message is returned from an API call, the constant for that API call can be subbed in, ensuring it will be exactly the same in any and all locations it's used. So as we go through the process of refactoring this app and upgrading it, I'll go into more detail for each particular folder and the files housed within it.
[13:08 - 13:36] We'll also add a few more folders to organize new things like custom hooks, contexts, tests, and more. So get ready, but this is a good starting point and a good basis for how you might want to organize a React application as it grows and gets bigger and more complicated. This has worked out pretty well for me and my experience. So now that we have talked about what is in this application and how it is built, let's talk about what is not included.
[13:37 - 14:01] There is no Redux. It is overkill here. Redux isn't included because it is unnecessary and the argument could be made. It's unnecessary for many React applications. It's been introduced to. Furthermore, trying to keep track of both React and Redux can muddy the waters of what's being supplied by React versus Redux, which introduces a level of complexity that won't enhance this course.
[14:02 - 14:25] With the introduction of hooks, with the introduction of the context API and its more versatile use cases, Redux just isn't necessary. So additionally, there is no SAS, there is no less for the CSS styling. Because this application is written with such an old version of Create React app, a version sold that SAS cannot easily be set up, the styling is written with vanilla CSS.
[14:26 - 14:48] In a true production app, this would almost certainly be supplemented with less SAS, CSS, and JS, you choose yours, maybe tailwind or bootstrap even. But for simplicity, and to focus more on the React part of modernizing this app , the vanilla CSS devices, and it separates the JavaScript code's logic from the app styling.
[14:49 - 14:54] So this should make understanding and refactoring things a little bit easier. There is no TypeScript.
[14:55 - 15:12] TypeScript is a superset of the JavaScript language, and while it's gaining popularity and widespread amongst the JavaScript community for its type checking capabilities, it will not be included in this course. For this course to be accessible to the widest amount of developers, I'm using vanilla JavaScript instead.
[15:13 - 15:24] If you'd like to learn more about using TypeScript, Newline offers a lot of other courses that are really great to help you get started. So now the big question is, what's next? React hooks are next.
[15:25 - 15:46] A big piece of this course is about how to upgrade a React application to use the latest the React framework has to offer, and that means React hooks, so that's what we'll get familiar with. So before we start upgrading the sample app's files, I'm going to introduce you to hooks in a friendlier manner and give some simpler examples of how they work for anyone who wants a refresher and wants to just play around.
[15:47 - 15:57] Then we'll dive into our project and start refactoring file by file to transform these class-based components into functional hooks-based components. I'll see you there.