Build Example Mock Data to Test a GraphQL Server
To help us get started, we'll begin by introducing a mock data array of rental listings in this lesson. This will help us get started before we begin to address more appropriate data persistence.
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
Introducing mock listings
📝 The mock listings array used in this lesson can be found - here.
📝 This lesson's quiz can be found - here.
🗒️ Solutions for This lesson's quiz can be found - here.
Let's add a little functionality to our current minimal server. To get us started, we're going to introduce a mock array of data that we'll be able to use and manipulate before we talk about and address more appropriate data persistence.
We'll introduce this mock array in a listings.ts
file within our src/
folder.
server/
// ...
src/
index.ts
listings.ts
// ...
The mock data we hope to introduce is a collection of rental listings where each listing will have a listing title
, image
, address
, price
, rating
, number of beds
, number of baths
, and permissible number of guests
. Our mock array is to have three distinct listing objects. This is purely fake data, with fake addresses, and copyright-free to use images.
We'll create and export a const
labeled listings
that is to be this mock array, in the src/listings.ts
file.
export const listings = [
{
id: "001",
title: "Clean and fully furnished apartment. 5 min away from CN Tower",
image:
"https://res.cloudinary.com/tiny-house/image/upload/v1560641352/mock/Toronto/toronto-listing-1_exv0tf.jpg",
address: "3210 Scotchmere Dr W, Toronto, ON, CA",
price: 10000,
numOfGuests: 2,
numOfBeds: 1,
numOfBaths: 2,
rating: 5
},
{
id: "002",
title: "Luxurious home with private pool",
image:
"https://res.cloudinary.com/tiny-house/image/upload/v1560645376/mock/Los%20Angeles/los-angeles-listing-1_aikhx7.jpg",
address: "100 Hollywood Hills Dr, Los Angeles, California",
price: 15000,
numOfGuests: 2,
numOfBeds: 1,
numOfBaths: 1,
rating: 4
},
{
id: "003",
title: "Single bedroom located in the heart of downtown San Fransisco",
image:
"https://res.cloudinary.com/tiny-house/image/upload/v1560646219/mock/San%20Fransisco/san-fransisco-listing-1_qzntl4.jpg",
address: "200 Sunnyside Rd, San Fransisco, California",
price: 25000,
numOfGuests: 3,
numOfBeds: 2,
numOfBaths: 2,
rating: 3
}
];
This lesson preview is part of the The newline Guide to Building Your First GraphQL Server with Node and TypeScript course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Building Your First GraphQL Server with Node and TypeScript, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
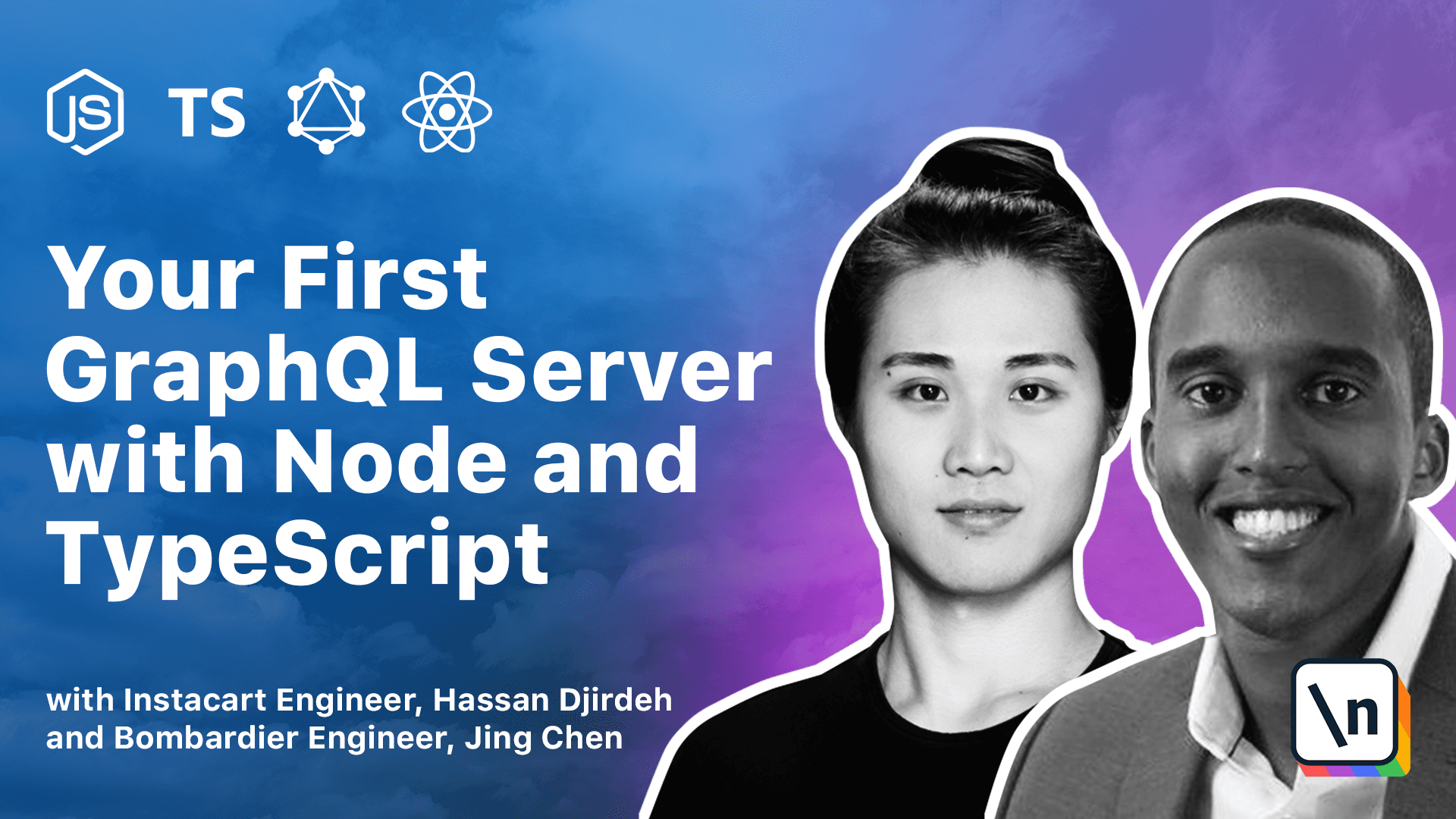