How to Create a Minimal Express Node.js Server
Web servers provide functionality for requests that can be made from client applications. Node has a built-in HTTP module that provides the capability to create a server. With this lesson, we'll look to use the popular Express framework as the replacement of the core HTTP module to create a minimal Node server, with Express routing.
Creating a minimal Node:Express Server
📝 This lesson's quiz can be found - here.
🗒️ Solutions for This lesson's quiz can be found - here.
What we've done so far is use Node to run a simple JavaScript file. Now, we'll look to create a Node server.
A server is software or even hardware that aims to provide functionality for client requests. Large scale applications we use day to day such as Airbnb, Uber, Instagram, and YouTube all have servers that serve data to the respective client apps. Client applications could be running on phones and computers to display this data to users.
Node has a built-in HTTP module that provides the capability to create a server. We'll create a very simple Node server and if you haven't done so before you'll notice how surprisingly little code is needed to make this happen.
package.json
The first thing we'll do is create a package.json
file. The package.json
file is an important element of the Node ecosystem and is where one can provide metadata about an app, list the packages the app depends on, and create scripts to run, build, or test the app.
We'll create this package.json
file at the root of our server project directory.
tinyhouse_v1
server/
index.js
package.json
A package.json
file must contain a name
and version
field. name
and version
dictate the name of the application package being built and the version of that particular package respectively. We'll name our application package tinyhouse-v1-server
and label the version as 0.1.0
.
{
"name": "tinyhouse-v1-server",
"version": "0.1.0"
}
To help us prepare a Node server, we'll install a third-party library known as Express. Express is an incredibly popular framework for Node designed for building servers and APIs. To install Express, we'll run the npm install command
followed by the name of the Express package (express
).
server $: npm install express
When complete, npm
will fetch Express from its repository and place the express
module in a folder called node_modules
in our server directory. When we now run our Node application, Node will look for modules that are required in the node_modules
folder before looking into parent directories and global installs.
We'll also notice that the package.json
file has been updated to include express
as a dependency.
{
"name": "tinyhouse-v1-server",
"version": "0.1.0",
"dependencies": {
"express": "^4.17.1"
}
}
This lesson preview is part of the The newline Guide to Building Your First GraphQL Server with Node and TypeScript course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Building Your First GraphQL Server with Node and TypeScript, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
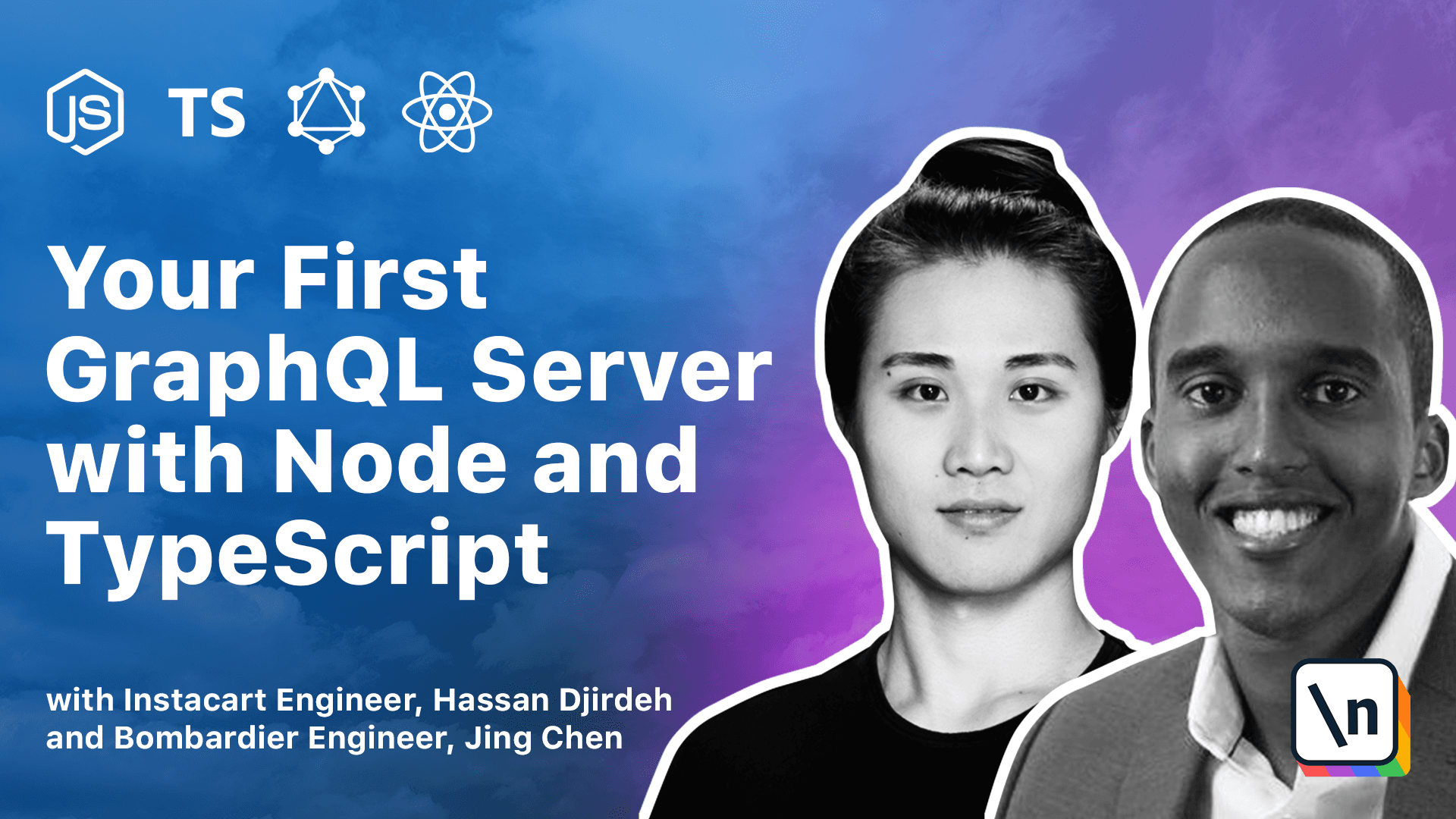
[00:00 - 00:09] What we've done so far is use a node to simply run a JavaScript file. Now, we're going to look to create a node server.
[00:10 - 00:28] A server is software or even hardware that aims to provide functionality for client requests. Large scale applications we use day to day, such as Airbnb, Uber, Instagram, YouTube, all have servers that serve data to the respective client apps.
[00:29 - 00:37] These could be running on their phones and even computers to display the data to users. This is a very simple explanation of what a server is.
[00:38 - 00:52] Node comes with an HTTP module that provides the capability to create a server. We're going to create a very simple node server, and if you haven't done so before, you'll notice how surprisingly little code is needed to make this happen.
[00:53 - 01:03] The first thing we're going to do is create a package.json file. The package.json file is an important element of the node ecosystem and is where one can provide metadata about an app.
[01:04 - 01:15] This the package is an app depends on and creates scripts to run, build or test the app. We'll create this package.json file at the roots of our server project directory.
[01:16 - 01:43] A package.json file must contain a name field and a version field. Name and version are pretty self-explanatory and it dictates the name of the application package being built and the version of that particular package.
[01:44 - 02:02] We'll name our application package TinyHouse v1 server and we'll give it a version number of 0.1.0. Next, to help us prepare a node server, we'll install a third-party library known as express.
[02:03 - 02:28] Express is an incredibly popular framework for Node designed for building servers and APIs. To install express, in our terminal, we'll navigate into the server folder and then run the npm install command followed by the name of the package we intend to install, which in this case is express.
[02:29 - 02:40] When complete, npm will fetch express from its repository and place the express module in a folder called node modules. We now run our node application.
[02:41 - 03:01] Node will look for the modules that are required in the node modules folder before looking into the parent directories and global installs in which packages may also be available. We can also notice that the package.json file has been updated to include express as a dependency.
[03:02 - 03:17] For anyone else downloading our directory, all they need to do is run npm install to install all the modules listed in the package.json file. You may also notice a package.lock.json file be automatically generated.
[03:18 - 03:45] The package.lock.json file stores the exact dependency tree that highlights the dependencies installed from the package.json file at a certain moment in time. This file was first introduced in npm version 5 and it serves to ensure teammates and deployment processes are all guaranteed to always install the same dependencies as well as aim to convey greater visibility in changes that are being made to the app's dependencies.
[03:46 - 03:56] The package.lock.json file should always be committed alongside all the other files to source code but will never actually directly touch this file. It is automatically generated.
[03:57 - 04:02] Cool. We'll now look to use express to instant shadon node server.
[04:03 - 04:14] We'll first move our index.js file to a source folder that's going to be located within our server application. We'll denote the source folder as SRC.
[04:15 - 04:36] Next, we're going to remove everything we've written before to have a blank slate for where we're going to create our node express server. The first thing we're going to do is we're going to require the express module and we're going to basically require that module and assign it to a variable labeled express.
[04:37 - 04:56] Next we're going to create and express server instance by running the express function and assigning that instance to a constant variable labeled app. We're then going to create a port constant variable with which we're going to specify the intended port number we'd want the server to run.
[04:57 - 05:15] We'll state this port number to be 9000. Now with app being the server instance, we can take advantage of express routing and use the app.get function to associate a path with an endpoint function.
[05:16 - 05:38] So here we can essentially say that when we receive an HTTP get request to the index routes denoted by just slash, we can attempt to respond by simply sending a hello world string. The endpoint function provides a request and response object as parameters.
[05:39 - 05:59] Request is an object containing information about the HTTP request while response is how we send back the desired response. Here we'll keep it simple by saying response.send a simple string that says hello world.
[06:00 - 06:18] We'll finally state app.listen which is a function that express provides to allow us to create the node server at a specified port. Here we can just simply pass in the port constant variable we've defined above which will say that our actual server is going to run on port 9000.
[06:19 - 06:42] And then for convenience, we'll use a simple console log message to basically tell us that our app is actually going to be successfully run in the appropriate ports. Like in the previous module, we can run this JavaScript code we've written by using the node command.
[06:43 - 06:51] So in our terminal, we're already located within the server folder. So we can now simply reference the source index.js file directly.
[06:52 - 07:00] So first we'll use the terminal clear command to clear the logs in our terminal . And now we can use the node command to run our index file.
[07:01 - 07:30] Though we can say source slash index.js to be explicit, we're also able to simply do node source with which is able to recognize that we want to run the index file within the source folder. Now that we can actually see when successfully run the console message that we 've generated, this is helpful in telling us that, hey, this server is now successfully running and you can find a server in this particular path, localhost 9000.
[07:31 - 07:43] When we head to our browser, when we actually navigate to localhost 9000 in this particular case, I have it open, so I'm simply going to refresh it. We can see the hello world message that's going to be sent back to us.
[07:44 - 08:03] This is because we're in the index route, low close 9000 slash, and we specified in our express routing that in the index routes, that is to say, when we attempt to get information from this route, we want the response to simply return the string of hello world. And we can see that this successfully works.
[08:04 - 08:28] [ Silence ]