Build a Keyboard App With a React Hook and the Web Audio API
This lesson preview is part of the Fullstack React with TypeScript Masterclass course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack React with TypeScript Masterclass with a single-time purchase.
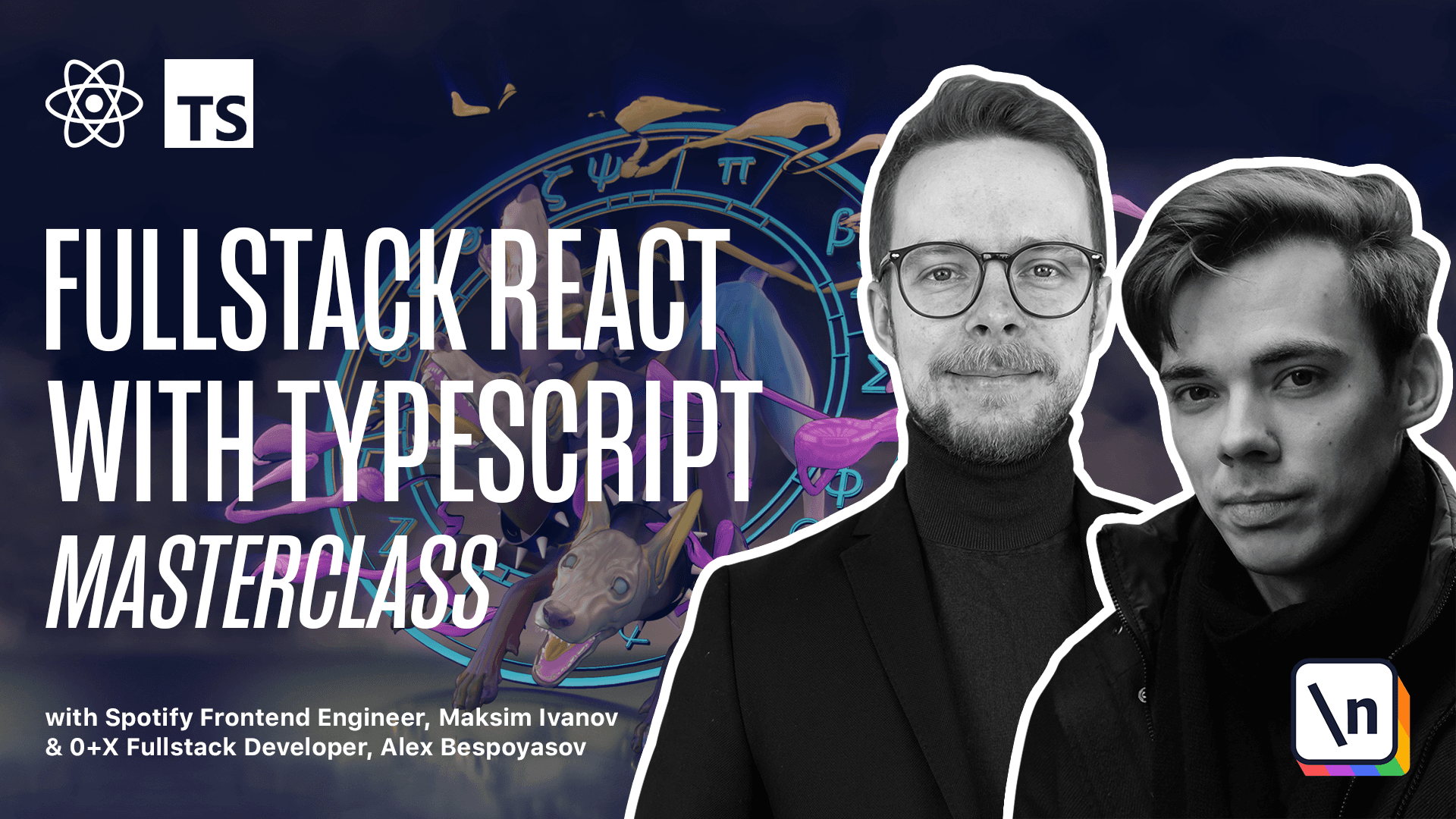
[00:00 - 00:07] The third-party API and the browser API. We're going to use the audio API and the third-party API called sound font to create a sound.
[00:08 - 00:12] So let's talk a bit about the integration of those APIs. The web audio API.
[00:13 - 00:20] For starters, let's figure out what's required to create a sound in a browser in the first place. Modern web browsers support audio API.
[00:21 - 00:30] It uses an audio context to handle audio operations such as playing musical tracks, creating oscillators, etc. This audio context has nothing to do with React context, that we saw earlier.
[00:31 - 00:40] They only have similar names, but audio context is an interface that provides access to the browser's audio API. We can access audio context via window audio context.
[00:41 - 00:50] The problem is that not every browser has this property. The majority of modern browsers do, but we cannot rely on the assumption that the user's browser has it.
[00:51 - 00:56] Let's ensure that the browser supports audio context. Create a helper function that will check if our browser supports audio context.
[00:57 - 01:07] Inside of the SRC domain, create a new file audio TS. Here we want to get the optional type, import, optional, from types.
[01:08 - 01:14] Now we want to define a function called access context. Expert, function, access, context.
[01:15 - 01:33] This function is going to return an optional audio context, and here we'll do the following. We either return window audio context, if it exists, or window, webkit, audio context, or, in worst case, we return nothing.
[01:34 - 01:43] So now that you know how access context is supposed to work, let's define the missing parts. Create a new file, types, .ts, and define an expert and optional type.
[01:44 - 01:59] Expert, type, optional, that will receive t, entity, type variable, and then it will return either t, entity, or now. So it will make this t, entity optional by returning a union type between the entity and now.
[02:00 - 02:11] Now let's provide the audio context types. Open React app and dts and define the type audio context type equals type of audio context.
[02:12 - 02:29] And we also want to extend the window interface, interface window extends window with webkit audio context. It will have the audio context type. Now we can get back to the audio.ts and all the errors should be done.
[02:30 - 02:57] Now what we just did, here we first created an audio context type, which is equal to type of audio context. This may seem a bit confusing, but technically it means that our custom type, audio context type, is literally a type of window audio context. Audio context is not a type per set, but a constructor function. So to make type script understand what type do we want to declare, we explicitly define it as type of audio context. Below we can see how we extend the window interface.
[02:58 - 03:13] Here we make it include the webkit audio context field that we specify as audio context type. This is required for now because TypeScript by default doesn't include some vendor properties and methods on window. Now we're done with the audio context and we can move on to sound font.
[03:14 - 03:41] Sound font is a framework agnostic loader and player which has a pack of pre- rendered sounds of many instruments. It is an external package and it comes with typings for integration with TypeScript projects. Let's begin integrating sound font with our project by installing it from NPM. Yarn. Add sound font player. Sound font player. After the package is installed, create a new file in the domain folder and call it sound.ts.
[03:42 - 04:07] Here we want to import instrument name from sound font player. And then we export a default instrument of type instrument name that is called acoustic grand piano. For now we're done with third party integration. In our app we will actually use those APIs through adapters. We will learn about them in the next lessons.