Style React Elements With CSS, Element Styles, and Libraries
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the Fullstack React with TypeScript Masterclass course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack React with TypeScript Masterclass, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
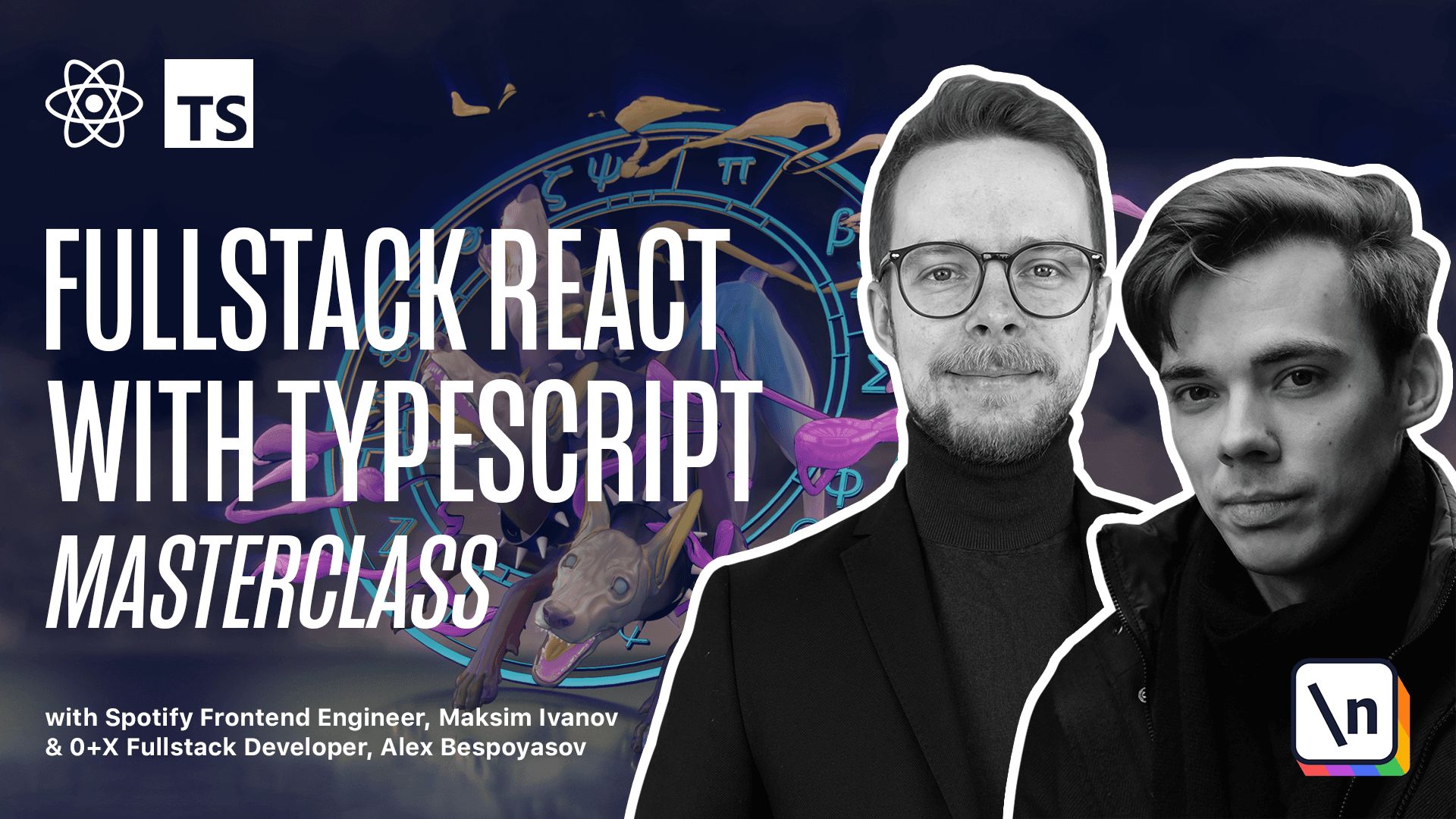
[00:00 - 00:03] How to style React components? There are several ways to style React elements.
[00:04 - 00:13] We can do it using regular CSS files, including CSS modules. We can manually specify the elements style property, and we can use external styling libraries.
[00:14 - 00:18] Let's briefly talk about each of the options. Using separate CSS files.
[00:19 - 00:26] You can have styles defined in CSS files. For example, in our application we have index CSS that we import in the index.tsx file.
[00:27 - 00:35] To use them you need a properly configured bundler, like webpack or parcel. Create React app includes a pre-configured webpack that supports loading CSS files.
[00:36 - 00:47] React elements accept class name prop that sets the class attribute of the rendered DOM node. As a result, you get a div in this case with class set to styled.
[00:48 - 00:53] You can also pass the CSS rules through the style property. For example, let's say we want to style this div.
[00:54 - 01:02] We can declare the object inline. Then we won't need to specify the type for it, but a better practice is to define styles in a separate constant.
[01:03 - 01:10] For example, here we define them in the bottom styles. Then we can apply them to our element by providing them inside of the style prop.
[01:11 - 01:19] As a bonus, we get auto-completion hints for CSS property names. It is important to note that we are not using real CSS attribute names.
[01:20 - 01:29] In React, the style properties are in camel case form. Here, for example, the background color is in camel case instead of being background-color, like in real CSS.
[01:30 - 01:36] This is crucial to remember when you work with React CSS properties. You can also use external styling libraries.
[01:37 - 01:41] There are a lot of libraries that simplify working with CSS in React. I like to use styled components.
[01:42 - 01:54] Stiled components allows you to define reusable components with attached styles like this. Here we define a bottom component that is a styled button with specified background color, border radius, border and box shadow.
[01:55 - 02:00] And then we can use it like a regular React component. Here we render a button with text "click me".
[02:01 - 02:09] We can also provide mouse events to it and overall it will work just like the regular button. In our trail application, we are going to use the style components approach.