Creating an issue
This lesson preview is part of the Fullstack React with TypeScript Masterclass course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack React with TypeScript Masterclass with a single-time purchase.
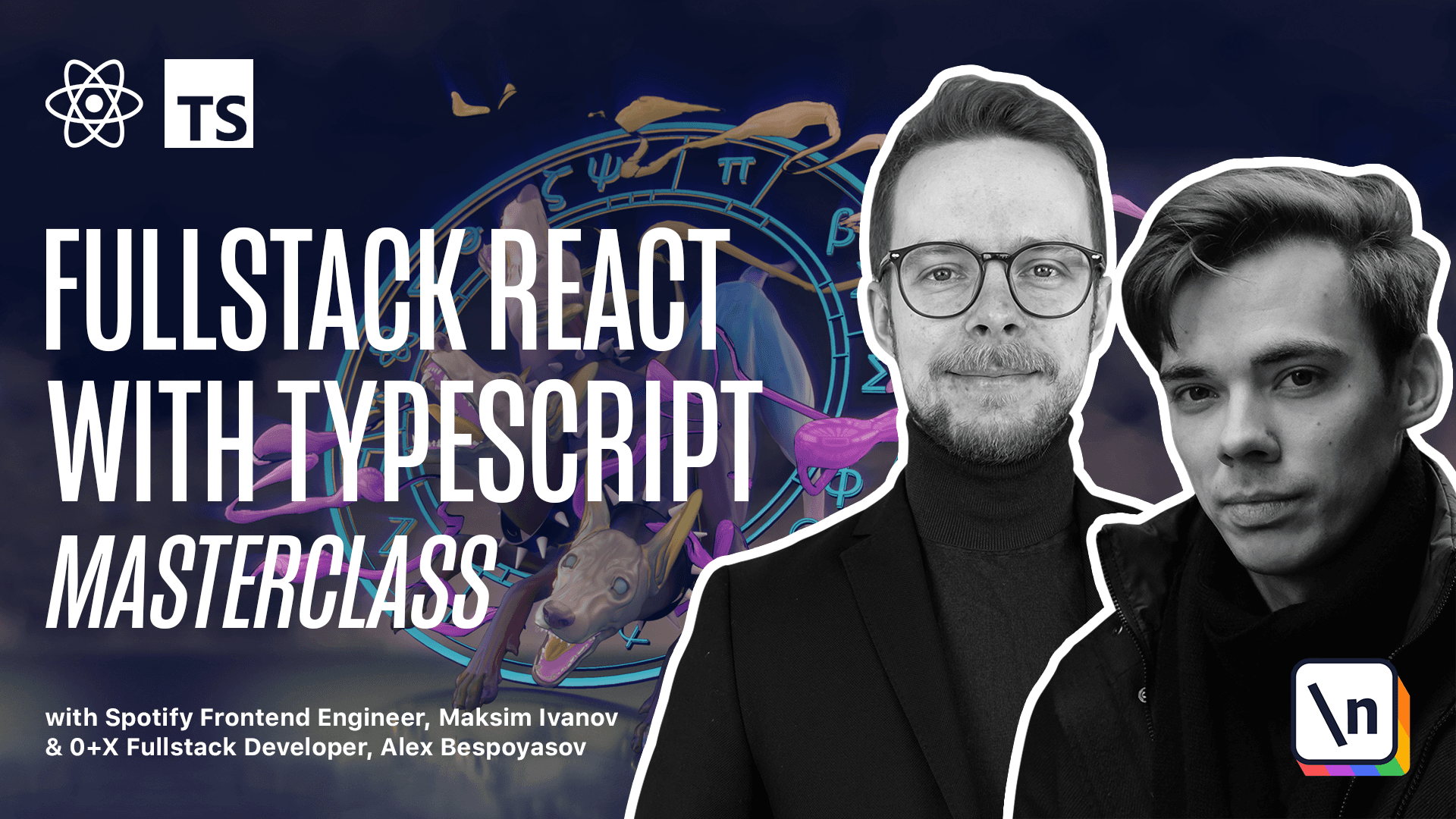
[00:00 - 00:07] Creating an issue. Inside of the src_issues, create a new file, new_issue_tsx.
[00:08 - 00:13] First, define the mutation. const create_issue = gql.
[00:14 - 00:19] Define the mutation, create new_issue. It's going to receive three arguments.
[00:20 - 00:31] Title, a required argument of type string, body, it's a description of our issue, it can be an optional string, and the repository id. Repository, it is also required field.
[00:32 - 00:39] Then we define the body of this mutation. We will call create_issue with the input.
[00:40 - 00:50] That will contain our arguments. It should be title = title, body, with value of body, and repository id with value of repository.
[00:51 - 01:02] Return issue, and from issue we want title to show it on success panel. And the URL to be able to navigate to the newly created issue on GitHub website .
[01:03 - 01:10] As you can see, this mutation accepts the repository id that we've defined in one of the previous lessons. Generate the types for the mutation.
[01:11 - 01:18] Run the code generator to get the types for this query. Run, yarn, run, Apollo, code, generate, with the following parameters.
[01:19 - 01:27] After it's done, import the generated types, along with the get repository query and types for it. Define the component.
[01:28 - 01:35] Now we're ready to define the new issue component itself. Expert const, new_issue = functional component.
[01:36 - 01:51] Here we use our mutation const create_issue = use_mutation. Provide the types, create new_issue, and type for the values, create new_issue variables.
[01:52 - 01:59] Pass in the create_issue, graph_kill query. Then let's define the onSubmit function.
[02:00 - 02:07] Const on submit = async. This function will receive values of type form values.
[02:08 - 02:33] And then here we'll have repo, title, and body from the values text box. We can get the owner and the name using the repository by splitting it with / owner_name = repo_split/ Alright, just like in the new repository component, we'll need two states, one for the error and one for the created issue.
[02:34 - 02:54] Const, error, set error = use_state = type error or null and then const, issue, set_issue = state. That has create new issue, create issue, issue, type.
[02:55 - 03:02] This is the type that graph_kill generated for us. Default value, don't forget to make it optional by providing an alternative null to it.
[03:03 - 03:23] Define the layout, return a panel, it should have top 25% left center and height 12 pixels. Inside of this panel we'll render the text, it's going to be the title left center and it's going to say new_issue.
[03:24 - 03:38] Below the text we're going to add a form and as you remember it's a render_prop component. So inside of it we need to pass a function that receives trigger_submit callback and there we return a fragment with a bunch of fields.
[03:39 - 03:45] We'll need repo, title and body fields. Each of them will trigger the trigger_submit function.
[03:46 - 03:54] Below the form add the instructional buttons. The text element should say tab to select next field and the button should say enter to submit.
[03:55 - 04:05] Don't forget to define the on-press_prop with an on-submit handler. Now to actually perform this mutation we'll need the reference to the client.
[04:06 - 04:23] const client = use_apolo client. Now inside of the on-submit function we can check if there is no owner or there is no name. Then we set error new error repository.
[04:24 - 04:33] Name should have owner name format. Actually we're going to use the same message for a bunch of entities that we're going to create.
[04:34 - 04:39] We'll need the same for the pull request for example. So it makes sense to store it in some constant.
[04:40 - 04:46] Then if we set the error we also need to return from this function. So we don't send anything anywhere.
[04:47 - 04:58] Next we receive the data using the await client query. Here we'll get the repository because we need the repository to be able to create issues.
[04:59 - 05:10] Get repository, get repository variables. Here we pass the configuration query get repository variables owner and name.
[05:11 - 05:26] That's enough to get the repository ID. Then if there is no data or no data repository then we return.
[05:27 - 05:40] Otherwise we try we must define a catch block here catch_e set error. Then we create a await create issue with variables title body and repository.
[05:41 - 05:52] That comes from data repository ID. You might think why didn't we use optional chaining here but we want to not even send anything if there is no data or data repository.
[05:53 - 06:02] Otherwise of course we could use optional chaining. Now we got the result let's set issue to result data create issue issue.
[06:03 - 06:29] Render the success and error results scroll bit down and before the layout if there is an issue then we return new entity success pass in the title new issue created pass in the URL issue URL and the on close handler. It's going to be a function that will set issue to null.
[06:30 - 06:42] Alright now let's process the error if error will return new entity error. We pass in the error that on close and that's it.
[06:43 - 06:56] On close we'll set error to null. Now go to issues TSX remove the new issue and import the proper one from the new issue module new issue from new issue.
[06:57 - 07:05] Alright run the app yarn start go to the issues press I if you press C you should be able to create a new issue now.