How to Save React Element State on the Backend Server
This lesson preview is part of the Fullstack React with TypeScript Masterclass course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack React with TypeScript Masterclass with a single-time purchase.
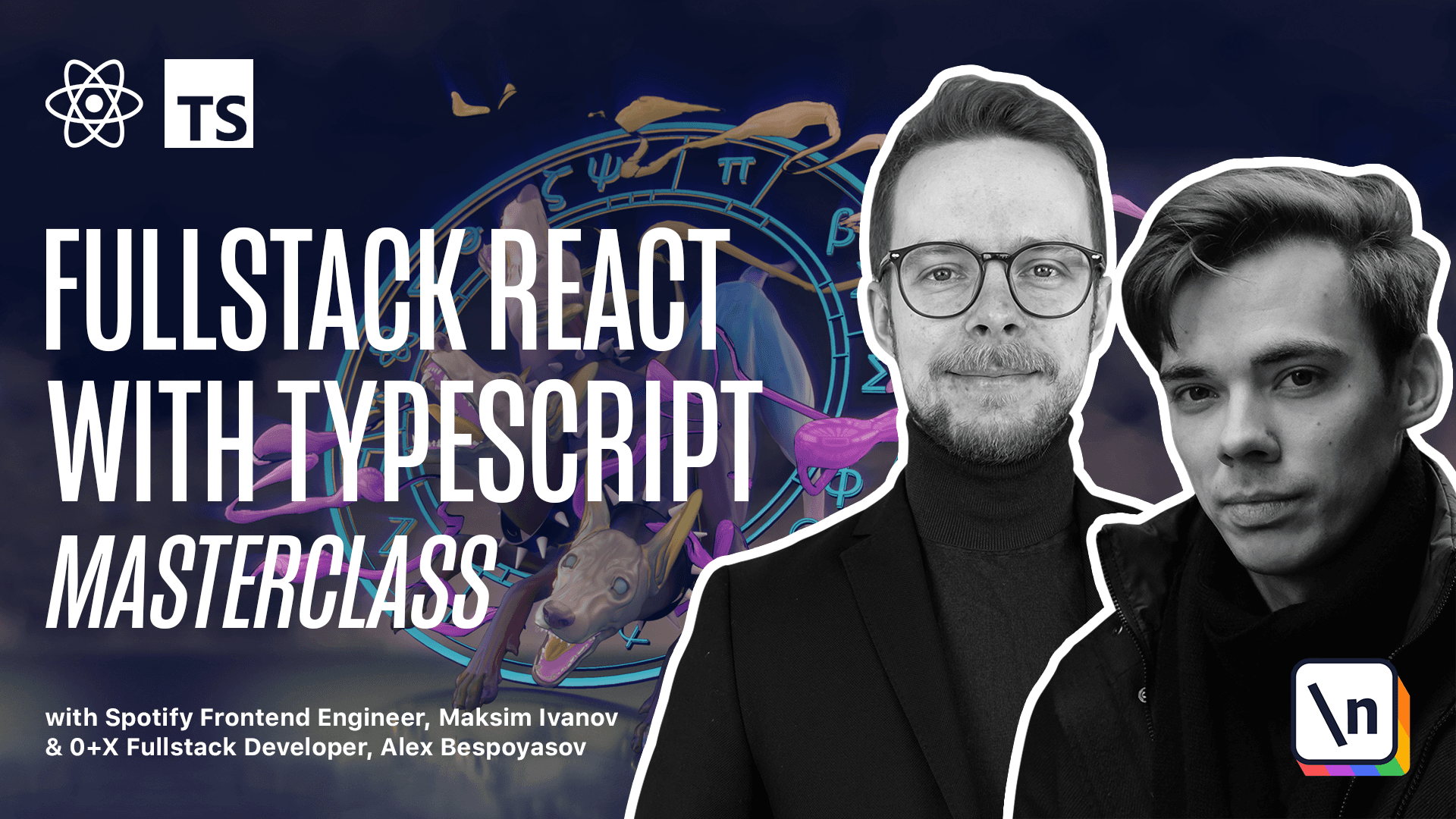
[00:00 - 00:07] Saving state on backend, how to make network requests. In this lesson we learned to work with network requests.
[00:08 - 00:20] Network requests are tricky, they're resolved only during runtime, so you have to account for that when you write your TypeScript code. In previous sections we wrote a Kanban board application where you can create tasks, organize them into lists and drag them around.
[00:21 - 00:26] Let's upgrade our app and let the users save the application state on the backend. Sample backend.
[00:27 - 00:32] I've prepared a simple backend application for this chapter. This backend will allow us to store and retrieve the application state.
[00:33 - 00:40] We'll use a naive approach and we'll send the whole state every time it changes . You will need to keep the server running for these chapter examples to work.
[00:41 - 00:53] To launch it, go to code 01 first app Trello backend, install the dependencies using Yarn and run YarnStart. Yarn and YarnStart.
[00:54 - 00:59] You should see the following message. Kanban backend running on HTTP local host port 4000.
[01:00 - 01:08] You can verify that the backend works correctly by manually sending curl requests. There are two endpoints available, one for storing data and one for retrieving.
[01:09 - 01:14] Here is the command to store the data. If you run it, you should receive success.
[01:15 - 01:22] True. And then you can run another command to retrieve the data.
[01:23 - 01:27] You should receive an object with an empty list. The final result.
[01:28 - 01:35] Before we start working on our application, let's see what we are aiming to get in the end. Make sure to have your backend running in a separate tab.
[01:36 - 01:53] Go to the examples folder provided with this course and there open the code example for the lesson 01 29 loading the data. Slide this folder, install the dependencies using Yarn and then run YarnStart.
[01:54 - 02:05] Initially you should see an empty field with plus another list button. Click it, try creating a bunch of lists to do in progress, done and then a couple of cards inside of them.
[02:06 - 02:13] Learn TypeScript, write tests and maybe something else. Read the book.
[02:14 - 02:20] Now if you try to reload the page, the task that we just created will be preserved. The starting point.
[02:21 - 02:35] If you've completed the instructions from the previous lessons, then you can continue from where you left off. If you didn't follow the previous lessons, then you can use the code from the code examples chapter 01 27 drag the card to an empty column.
[02:36 - 02:41] Copy the folder somewhere into your working projects directory and use it to build upon. Using fetch with TypeScript.
[02:42 - 02:49] Browser JavaScript has a built-in fetch method that allows network requests to be made. Here is a TypeScript type declaration for this function.
[02:50 - 02:58] It says that fetch accepts two arguments. Input, of type request info, where request info is a union type defined like string or request.
[02:59 - 03:06] It means that it can be a string or an object having request type. And the init, optional argument, of type request init.
[03:07 - 03:16] This argument contains options that can control a bunch of different settings. Using this parameter you can specify request method, custom headers, request body, etc.
[03:17 - 03:21] Performing requests. Here is a typical post request performed with fetch.
[03:22 - 03:24] We specify the URL that we want to fetch. We specify the method.
[03:25 - 03:36] Here it's post. The headers, content type application JSON and the body where we send the JSON string created from the object with the field username containing string example.
[03:37 - 03:41] Working with responses. fetch returns a promise that resolves to a response type.
[03:42 - 03:48] We will usually work with JSON type responses. So to us the most interesting field is the JSON method.
[03:49 - 03:56] This method returns a promise that resolves to response body text as JSON. Unfortunately this method is not defined as generic.
[03:57 - 04:07] So we'll have to do some trickery to specify the type of the return value. Here we have to cast the return type of JSON method to a promise and then specify the type of the return value here.
[04:08 - 04:15] So let's say here we're trying to fetch the data from apigithub.com. We expect to get an object that contains field current user URL.
[04:16 - 04:25] We say that it's going to be a string. And then after we get this data and destructured the response body object we can log that type of this field and we expect that it's going to be a string.
[04:26 - 04:34] Create API module. When I work with network requests I prefer to create a separate module with asynchronous functions that abstract the actual network calls.
[04:35 - 04:40] Let's go to our app. Let's create a .n file in the root of your project with the following contents.
[04:41 - 04:46] It will hold the backend endpoint. In our case it's localhost 4000 where our backend is running.
[04:47 - 05:01] In create React app applications all the environment properties should be pref ixed with react app. So we say that react app backend endpoint equals HTTP localhost 4000.
[05:02 - 05:13] Now inside of the src folder create a new file api.ts. And here we are going to import app state from state app state reducer.
[05:14 - 05:35] Now we'll define the save function expert const save equals a function that will receive the payload of type app state as an argument. And then it's going to return fetch we get the URL from the environment use the template string process and react app backend endpoint.
[05:36 - 05:48] And then we use the save handle here. We pass the options object the method should be post headers is an object containing accept field that should be application JSON.
[05:49 - 05:56] And then the content type of the value we're sending is going to be application JSON as well. Content type is application JSON.
[05:57 - 06:08] And then we send the body body is going to be JSON string from the payload. After we perform the fetch we can call then and then here we will process the response.
[06:09 - 06:18] First of all we check if response is positive so it has status 200 then we return response JSON. Otherwise we throw a new error.
[06:19 - 06:28] We don't really care what is the error here we just care about the state not being stored. So we say error while saving the state.
[06:29 - 06:44] So what this function does it accepts the current state and sends it to the backend as JSON in case of the unsuccessful save we throw an error saving the state. We want to save our application state every time it changes.
[06:45 - 06:53] This means that every time we move the items around or create new ones we want to make a request to our backend. In our application we have a redux like architecture.
[06:54 - 07:03] It means that we have a centralized store that holds our application state. We don't really use redux but we use React built in userhood which is fairly similar.
[07:04 - 07:15] In order to save the state on the backend we will use the use effect hook. Go to SRC state upstate context and in produce effect hook from react use effect.
[07:16 - 07:30] Then scroll down and right before the upstate provide return statement add use effect here is a callback that will call save state. Every time that the state changes, import the save function from the API.
[07:31 - 07:40] The use effect hook allows us to run side effect callbacks on some value changes. So every time the state will change it will save it on the backend.
[07:41 - 07:49] Now if you drag the cards around in your application and then look at the server logs you should see the console output that should look somewhat like this.