Code a Keyboard in React and Compose Classes with clsx
This lesson preview is part of the Fullstack React with TypeScript Masterclass course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack React with TypeScript Masterclass with a single-time purchase.
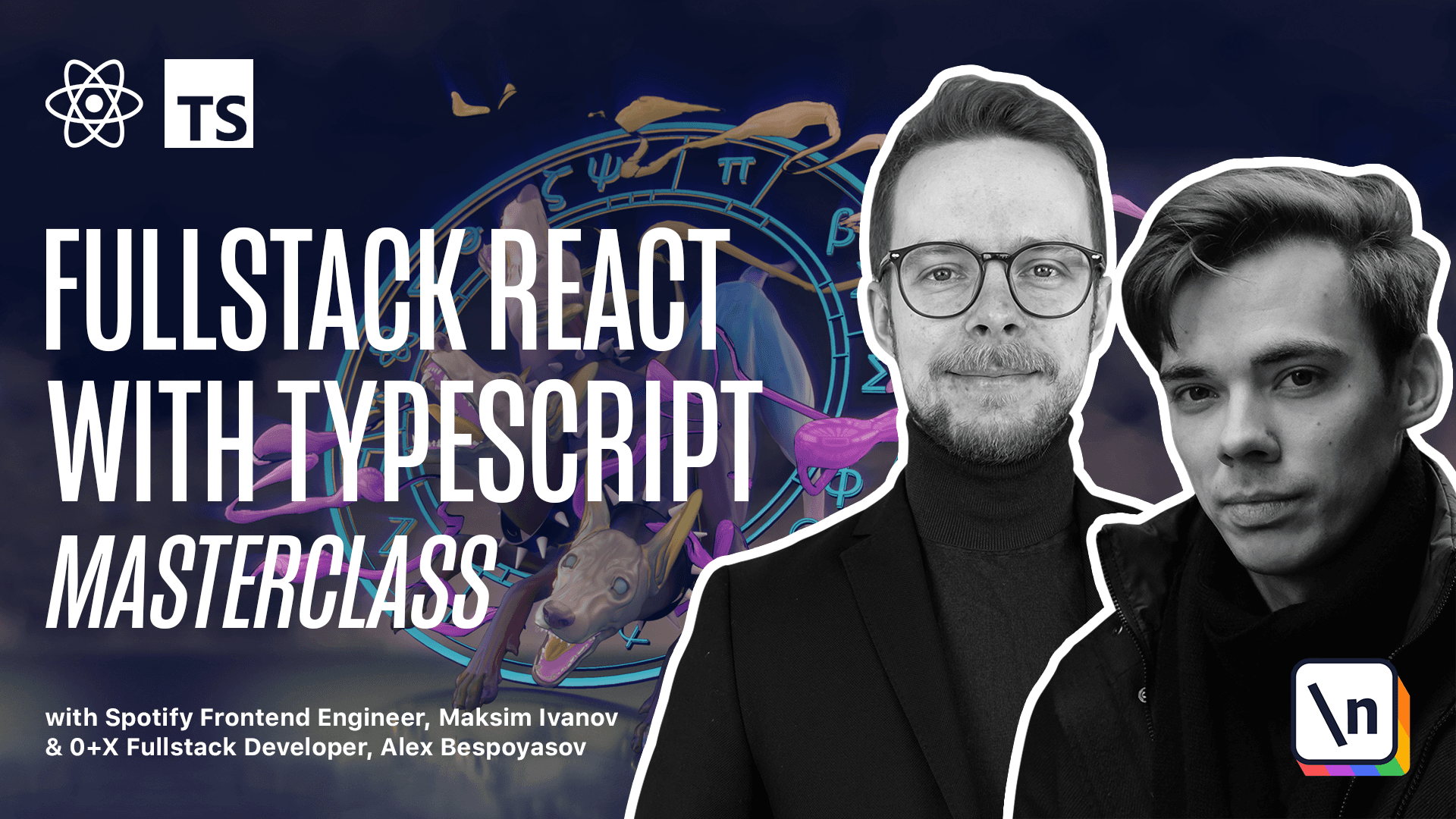
[00:00 - 00:06] Creating a keyboard. In this lesson we will implement the keyboard. We will start with the component that will render the individual keys.
[00:07 - 00:13] In this component we will need to compose different class names on the element. To make it easier let's install CLSX package.
[00:14 - 00:22] Yarn, Add, CLSX. After it's done, create a folder as your C, components, key.
[00:23 - 00:25] And first we'll define the styles. Styles for the key.
[00:26 - 00:33] Our keys will be based on the regular button element. To make it look similar in all the browsers we want to reset the default button styles.
[00:34 - 00:49] Open SRC index CSS and add the following styles. Styles for the bottom element where we reset the border with border radius, margin padding with background, appearance, color, font, line height and cursor .
[00:50 - 00:58] Basically we made the button look like a text element. We've added those styles into the SRC index.css because we want them to affect the whole application.
[00:59 - 01:12] Now inside of the key folder in the components, create a new file, key, module, CSS and define the key class there. It should have position, relative, font size.
[01:13 - 01:25] We take from the variable font, size, border, radius, 0, 0 and we take value of the variable radius. And here as well, var radius.
[01:26 - 01:34] Text, transform, upper case, user, select, none. Also define the variables for the key class.
[01:35 - 01:46] The radius that we use for the border radius is two pixels, font size, 0.6 rem and so on. Our keys will have natural, sharp and flat modifiers.
[01:47 - 01:57] Natural, should have width and height, defined in the white key width and white key height. Variables, padding top also comes from the variables.
[01:58 - 02:14] It should have solid one pixel, semi transparent black border, should have gray , font color, small margin right and that index one. Sharp and flat have similar styles because both will be just black keys on the top of the keyboard.
[02:15 - 02:28] Sharp, flat, the width and height come from the black key width and height variables, same with the padding top. The ground color is a very, very dark gray, text color is white and margin comes from the variables as well.
[02:29 - 02:41] That index should be two to overlap the natural keys. Now let's add the modifiers for the pressed keys. You will need natural, active and pressed, where we set the background color to be a bit darker.
[02:42 - 02:51] And for the sharp and flat keys, it's going to be a bit lighter instead. We also need some modifiers for the disabled keys.
[02:52 - 03:02] Here's where we specify the background color and special cursor form. We also need to add disabled styles specifically for natural, sharp and flat styles.
[03:03 - 03:13] Let's define the media queries to support different screen sizes. Here is one for the smallest screens. Here we just override the values of the variables so we don't need to override all the styles.
[03:14 - 03:25] This is the convenience of using variables because now we can easily adjust our styles. Define another one for the bigger screen, some versions for tablets and the biggest version for the desktop.
[03:26 - 03:33] Define the key component. Inside of the C components key, create new file, key. tsx.
[03:34 - 03:46] Add the imports, we'll need the function component from React, CLSX from the C LSX library we've installed in the beginning of this lesson. Note type from the note domain and styles from the key module CSS.
[03:47 - 04:02] Now define the key props. Type, key props. They will contain the type, note, type. As you remember it can be natural, flat or sharp. Then we'll have a label, string and disable, optional flag of type Boolean.
[04:03 - 04:16] Expert const key, that's going to be our component, function component with props coming from key props. Define the function of this component, the props now have type key props.
[04:17 - 04:33] We can get type, label and the rest from them and return the layout. Here we'll render a button. Inside of it we'll render the label. We'll specify the class name and we'll combine the class names using the CLSX package.
[04:34 - 04:47] We'll combine styles key with styles and the type. This is how we apply the styles for the flat, sharp or natural keys. We also specify the field type to be bottom.
[04:48 - 05:00] Then we pass the rest of the props. Create an index.tsx.tsx and re-expert everything from the key. Expert everything from key. Create the keyboard component.
[05:01 - 05:16] Inside of the components folder, create a new folder, keyboard and inside of it create a new file, keyboard, module, CSS. Here we define the styles for the keyboard. Keyboard, display, flex, that's it.
[05:17 - 05:41] Now we define the keyboard.tsx file, new file, keyboard, tss. Here we'll need the notes from the note domain, key from the key component folder and styles from the keyboard module. We define the keyboard component. Expert const, keyboard, it's a functional component. We return div with class name, styles, keyboard.
[05:42 - 06:01] Inside of it we map through the notes map and for each note we break it down into MIDI, type, index and octave. Then we get the label, const label equals select key. Select key will select a letter label for the given key.
[06:02 - 06:18] We will write this function in a second. It will receive the octave number and the index of the key inside of this octave. Now we return a key. The key of this element is going to be the MIDI type, is going to be type and label is going to be label.
[06:19 - 06:34] Let's define the select key function. Inside of the domain folder create a new file, keyboard.ts and here we define the select key. First we import octave index and pitch index from note.
[06:35 - 07:03] We define an expert the type key, it's just an alias for the string type and the type keys. Expert, type, keys, that's going to be an array of type key. Expert, three constants, one for the top row, it's going to be an array of keys and we define it as an array from Q2W3ER5T6Y7U.
[07:04 - 07:33] Define the bottom row, Z, S, X, D, C, V, G, B, H, N, J, M and now expert const change row at, it's going to be an octave index, the value is 5. Now create an expert the select key function. Expert, function, select key, it 's going to receive an octave index, octave of type, octave index and the index of the note, pitch index.
[07:34 - 08:04] As a result to return the key, as you remember it's just a string. Now we get the keys row equals octave, if it's smaller than change row at, then it's going to be top row, otherwise it's going to be bottom row. Now that we have the keys row, we can return keys row and take an element by the index from there. Alright, go to the keyboard, import the select key and now let's update the main component.
[08:05 - 08:25] Go to components main, main TSX and use the real keyboard instead of the dummy that we used before, import keyboard from the keyboard. Let's run the application. Yarn, start. If everything is fine, then you should see your keyboard, you should be able to press the keys and they should change their colors slightly.