How to Test a useCart React Hook With Multiple Functions
This lesson preview is part of the Fullstack React with TypeScript Masterclass course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack React with TypeScript Masterclass with a single-time purchase.
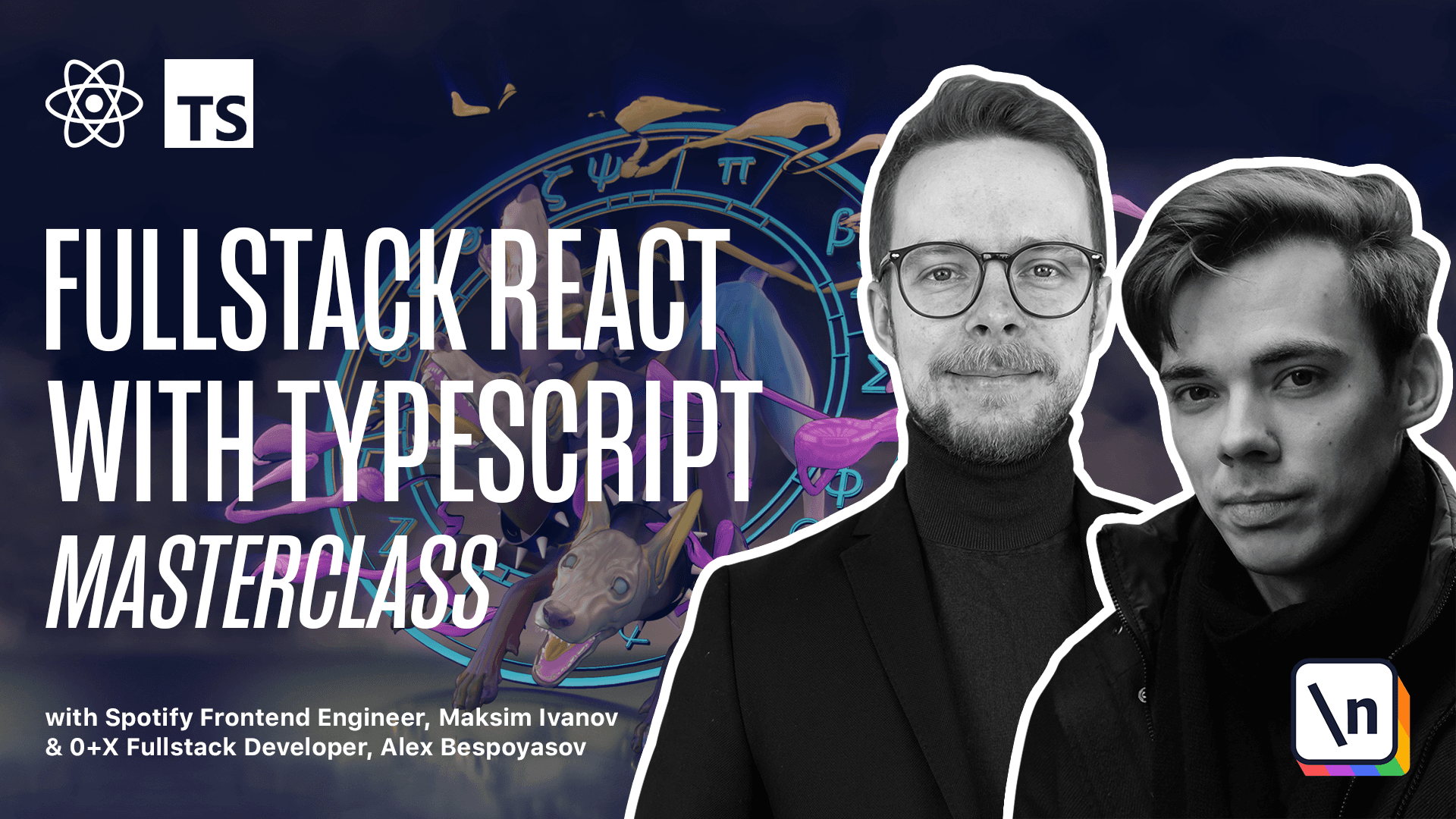
[00:00 - 00:24] Testing useCard. Another hook that we have in our application is useCard. This hook allows us to get the list of products in the card, add new products, or clear the card. This hook provides a bunch of functions and will check each of them in our tests. If we look at the useCard hook, you will see that first of all it has a use effect that triggers on mount when the component that uses this hook is mounted.
[00:25 - 00:42] It has the total price method that calculates the total price of the products, add to card that allows us to add new items to the card, remove from the card, and clear card methods. Create a new file, useCardSpec.ts and describe the useCard.
[00:43 - 00:53] Describe, useCard. Pass in a callback where we will have our pre-planned tests. We'll have a bunch of aspects that we want to test here.
[00:54 - 01:17] First we want to describe on mount. On mount. Here we'll have only one test, it to do. It loads the data from the local storage. Then we have another to do that will test the add to card method. And in this case we want to check that it adds the item to the card.
[01:18 - 01:43] Next we want to test the remove from card and it must remove the item from the card. We want to test the total price method. We expect that it returns the total products price and the clear card removes all the products from the card.
[01:44 - 01:55] Okay, first of all we'll need to mock the local storage. Conced local storage. Mock is going to be a function that will create the mock for our local storage.
[01:56 - 02:12] It's going to be self invoked. Inside of it will have the store let store. It should be mutable, we should be able to update the values there. And it will contain keys of type string and values of type string. By default the value is going to be an empty object.
[02:13 - 02:34] Now we return an object with the following fields. We want to have a function clear. It's going to remove all the items from the local storage. By assigning store the value of empty object. Then we want to have get item. It 's going to receive a key of type string and it's going to return store key.
[02:35 - 02:59] If there is no value by this key then it will return null. We want to have remove item. It is also a function that receives a key of type string. But instead of returning the store key value it will delete it. Delete store value by key. And then we want to have a set item method that will receive a key of type string and a value of type string.
[03:00 - 03:22] This method should set store key to value if it exists value then value to string. Otherwise it should set it to an empty string. Now we want to assign it to the window object. To do this we use object define property window local storage.
[03:23 - 03:45] And then we pass the value local storage mock. Here we have to use the object define property method because the window local storage field is read only window local storage. And then we command click on it you will see that it's a read only property. So as a workaround we can use object define property because then types get one complaint.
[03:46 - 04:07] One last thing before we move on to the test add the cleanup code inside of the top level describe. After each we want to call local storage mock clear. So after each test case we're going to reset the local storage mock. And we also call just restore all mocks.
[04:08 - 04:23] This method restores all the mocks back to their original value. We need to do this to reset all the counters in the spy functions. Now let's describe the on mount test. Add a callback. Here we want to verify that it lost the data from the local storage.
[04:24 - 04:48] First we need to define the products. Const products is going to be an array of type product. Here we'll have only one product. Name product full price zero image image JPEG. Then we call local storage mock set item the key is products and the value is Jason stringify products.
[04:49 - 05:15] Then we render the use card hook const result equals render hook use card. And then we expect the result current products to equal the product tree. The test is passing and we can continue. Then we want to test that we can add the items to the cart. Add a callback. Here we'll also need the product tree const product.
[05:16 - 05:50] Here we'll need to define one product const product of type product equals an object with name product full price zero and image image JPEG. Render the hook const result equals render hook use card. Then we want to get the spy function. We're going to spy on the local storage mock set item function const set item spy just spy on local storage mock set item.
[05:51 - 06:15] Spying on the function means that we will be able to track the amount of calls to this function the arguments that were passed to it count how many times this function was called and so on. In our case we want to be able to tell if the set item spy was called with the correct values. So we call act we do it because the add to cart method alters the state of our hook.
[06:16 - 06:49] So let's do it result current add to cart and here we pass the product. After we've added the product we expect result current products to equal an array with just one item product. We also expect that the set item spy was called with products that equals JSON stringify array with one product. Then we can call set item spy mock restore.
[06:50 - 07:21] So here we hard code the product render our hook call add to cart. We wrap it into act because it updates the state inside of the hook. Then we verify that the products array from our hook matches an array with our hard coded product. And finally we check that the data stored in the local storage is correct and it also matches an array with our hard coded product. Now let's move on to remove from cart remove that to do add a callback.
[07:22 - 07:48] Here we want to define a product as well const product of type product equals an object with name product full price zero image image JPEG. Here before rendering our hook we call local storage mock set item products with the JSON value of that product array. Now we have an item in the local storage.
[07:49 - 08:20] Then we render our hook const result equals render hook use cart. We'll need to spy on the set item function again const set item spy equals just spy on local storage mock set item. Then we perform an action we remove the item from the card act result current remove from cart and we pass in the product. Now we can test a bunch of expectations.
[08:21 - 09:04] First we expect that the cart is going to be empty expect result current products to equal an empty array. Then we expect local storage mock set item to have been called with the products that should have a value of an empty array. Then we reset the set item spy that has this passing we can test the total price now here we can copy the products from the previous test we call local storage mock set item products JSON stringify and we pass an array with two products.
[09:05 - 09:46] Render the hook const result equals render hook use cart and now expect that the result current total price is equal 42. The test is failing because the sum of the prices of two products is going to be zero. Let's fix it. Let's try passing 21 and run the test again. The test is passing and we can write the clear card test. Add a callback function here we'll also need the product set the local storage mock products to an array with two instances of the product spy on the set item method.
[09:47 - 10:16] Now we can call clear card act result current clear card render the use card hook const result equals render hook use cart. Then we spy on the set item method. Now we can call act where we'll call the clear card result current clear card and add the expectations expect that the products are equal an empty array.
[10:17 - 10:37] We also expect after clearing the card that the local storage mock set item was called with the products that were an empty array and then we call set item spy mock restore. The test is passing and that means that you have tested the whole application.
[10:38 - 10:45] Congratulations.