How to Bootstrap a React TypeScript App With create-react-app
This lesson preview is part of the Fullstack React with TypeScript Masterclass course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack React with TypeScript Masterclass with a single-time purchase.
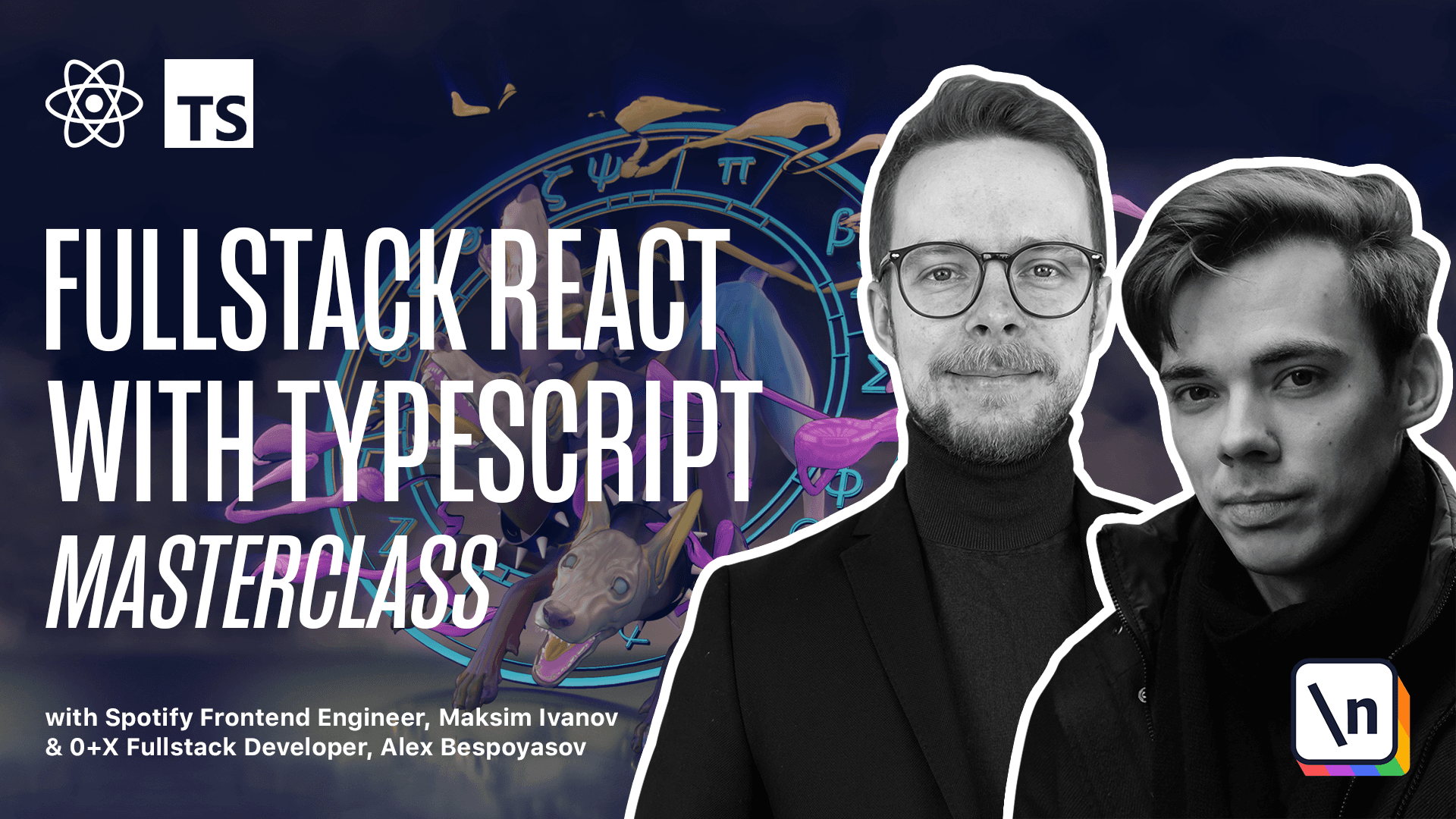
[00:00 - 00:06] How to bootstrap React and TypeScript app automatically. In this lesson, we will use an automatic CLI tool to bootstrap our project's initial structure.
[00:07 - 00:13] Why use automatic app generators? Usually, when you create a React application, you need to create a bunch of boilerplate files.
[00:14 - 00:19] First, you will need to set up a transpiler. React uses JSX syntax to describe the layout.
[00:20 - 00:25] And also, you'll probably want to use the modern JavaScript features. To do this, we'll have to install and set up Babel.
[00:26 - 00:31] It will transform our code to normal JavaScript that current and old browsers can support. You will also need a bundler.
[00:32 - 00:37] You will have plenty of different files. Your components code, styles, maybe images and fonts.
[00:38 - 00:47] To build them together into small packages, you will have to set up Webpack as your application bundler. Another example of a bundler is Parcel, Parcel.js.org.
[00:48 - 00:56] Then there are a lot of smaller things. Setting up a test runner, adding vendor prefixes to your CSS rules, setting up a linter and enabling hot reload.
[00:57 - 01:01] So you don't have to refresh the page manually every time we change the code. It will be a lot of work.
[01:02 - 01:11] To simplify the process, we'll use the create react app package. It is a tool that will generate the file structure and automatically create all the settings files for your project.
[01:12 - 01:19] This way, we will be able to focus on using react tools in the TypeScript environment. How to use create react app with TypeScript.
[01:20 - 01:31] Navigate to the folder where you keep your programming projects and run create react app. We do it using NPX, create react app, dash dash template.
[01:32 - 01:39] We select the TypeScript template, and then you specify the name of your project. In our case, it's going to be Trello clone.
[01:40 - 01:43] All right, now app is created. Let's look at the command that we've just used.
[01:44 - 01:49] Here we've used NPX to run create react app without installing it. This is the recommended way to use create react app.
[01:50 - 01:54] Read more in their getting started guide. Create react app.dev, docs getting started.
[01:55 - 02:01] We've specified an option, template, TypeScript. So our app will have all the settings needed to work with the TypeScript.
[02:02 - 02:06] The last argument is the name of our app. In our case, Trello clone.
[02:07 - 02:16] Create react app will automatically generate the Trello clone folder with all the necessary files. CD2 Trello clone and open it with your favorite code editor.
[02:17 - 02:21] Project structure generated by create react app. Let's look at the application structure.
[02:22 - 02:27] If you've used create react app before, it will look quite familiar. We have a bunch of files and folders here.
[02:28 - 02:35] Let's go through the files and see why we need them. We'll do a short overview, and then we'll go back to some of the files and talk about them a bit more.
[02:36 - 02:39] First of all, the files in the root. Here you have the README file.
[02:40 - 02:49] This is a markdown file that contains a description of your application. For example, GitHub will use this file to generate the HTML summary that you can see at the bottom of the projects.
[02:50 - 02:55] Then we have the package JSON file. This file contains metadata relevant to the project.
[02:56 - 03:03] For example, it contains the name, the version, and optionally the description of our app. It also contains the dependencies list.
[03:04 - 03:12] It has all the external libraries that our app depends on. You can find the full list of possible package JSON fields and their descriptions on the NPM website.
[03:13 - 03:17] In the docs for that package JSON. Let's take a closer look at the dependencies.
[03:18 - 03:27] You might see that some packages, like for example React, have corresponding types packages. Those types packages contain type definitions for libraries originally written in JavaScript.
[03:28 - 03:36] Why do we need them if TypeScript can parse JavaScript code as well? The problem with JavaScript is that often it's impossible to tell what types the code will work with.
[03:37 - 03:47] Let's say we have a JavaScript code with a function that accepts the data argument. TypeScript can parse this code, but it has no way of knowing what type the data attribute is restricted to.
[03:48 - 03:59] So for TypeScript, the data attribute will implicitly have the type any, and this function will accept basically any value. This type matches with absolutely anything, which defeats the purpose of type checking.
[04:00 - 04:08] If we know that the function is meant to be more specific, for instance, it only accepts the values of TypeString. We can create a dts file and describe it there manually.
[04:09 - 04:20] This dts file or a file with an extension.d.ts will contain the types for the specific module. This file name should match the module name we provide the types for.
[04:21 - 04:36] So in this save data function example, if this function comes from the save data.js module, we'll create a save data.d.ts file. We'll need to put this file where the TypeScript compiler will see it, usually in its as a C folder.
[04:37 - 04:48] This file then will contain the declaration for our save data function. Declare function save data, and then here we specify the type of the data attribute, and then we set the return value to be void.
[04:49 - 04:58] This is another way of saying that this function returns nothing or undefined, or as you can see void. We could create a package with this file and publish it to npm.
[04:59 - 05:11] This is what all those add types, and then some name packages are. They contain files with the dts extensions, and those dts files contain the declarations for the types of those libraries.
[05:12 - 05:20] It is a convention that all the types packages are published under the types namespace. Those packages are provided by the definitely typed repository.
[05:21 - 05:33] When you install JavaScript dependencies that don't contain type definitions, you can usually install them separately by installing a package with the same name and add types prefix. Versions for types and their corresponding packages don't have to match exactly.
[05:34 - 05:48] Here you can see that React DOM has version 17.02, and the types for it have version 17.01. Apart from dependencies, this package JSON file also contains the scripts block that helps us work with our application.
[05:49 - 05:58] We can launch it, build, test, or eject, and those scripts should be familiar to you if you already worked with a Create React App package. Let's move on to the next files.
[05:59 - 06:03] Here we have the package lock.json. Alternatively, you might have yarn.lock file.
[06:04 - 06:13] This file is generated when you install the dependencies using yarn or npm, and it's gonna be in your project truth. This file contains resolve dependencies versions along with their sub-dependencies.
[06:14 - 06:21] It is needed for consistent installations on different machines. Thing is you don't really have to read or especially edit this file.
[06:22 - 06:31] In fact, it's harmful, so don't edit it manually, but it is still good to know what this file is doing. Next, we have the TSConfig.json file, and this file contains that TypeScript configuration.
[06:32 - 06:39] We don't need to edit this file because the default settings work fine for us. You can tweak it later when you gain more confidence with TypeScript.
[06:40 - 06:46] And finally, we have the gitignore file. This file contains the list of files and folders that shouldn't end up in your git repository.
[06:47 - 06:50] So we're done with the files in the root. Let's go to the folders.
[06:51 - 06:56] First, we have the public folder. The public folder contains the aesthetic files for our app.
[06:57 - 07:05] They are not included in the compilation process and remain untouched during the build. Read more about the public folder in the create/react app documentation.
[07:06 - 07:13] In this folder, we'll have the index.html file. This file contains the special div with ID root.
[07:14 - 07:19] That is a mounting point for our React application. This folder also contains the manifest.json file.
[07:20 - 07:30] And this file provides the application metadata for the progressive web apps. For example, the file allows installation of your application on a mobile phone's home screen, similar to native apps.
[07:31 - 07:38] It contains the app name, icons, theme, colors, and other data needed to make your app installable. Next, we have a bunch of icons.
[07:39 - 07:47] We have the ICO format and two bigger icons with PNG extension. These are icons for our application.
[07:48 - 07:57] For example, the ICO is a small icon that is shown on browser tabs. Also, there are bigger icons, logo 192 and the logo 512.
[07:58 - 08:07] Those are referenced in the manifest.json and will be used on mobile devices if your app will be added to the home screen. Last file on this folder is the robots.txt.
[08:08 - 08:12] And this file tells crawlers what resources they shouldn't access. By default, it allows everything.
[08:13 - 08:19] If you need, you can read more about robot.txt on the robots.txt website. We're done with the public folder.
[08:20 - 08:26] Let's go to the SRC. Files in this folder are processed by the webpack and will be added to your app's bundle.
[08:27 - 08:40] This folder contains a bunch of files with .tsx extension, like app.tsx, index.tsx, and then we'll add more files like that. This extension means that those files contain .jsx code.
[08:41 - 08:47] As you can see, .jsx is HTML-like syntax used in React applications to describe the layout. You can read more about it in React docs.
[08:48 - 08:54] In a JavaScript React application, we could use either .jsx or .js extensions for such files. It would make no difference.
[08:55 - 09:04] With TypeScript, you should use .tsx extensions on files that have .jsx code and .ts on files that don't. Here, for example, setuptests.ts.
[09:05 - 09:13] This is important because otherwise, there can be a syntactic clash. Both TypeScript and .jsx use angle brackets, but for different purposes.
[09:14 - 09:33] For example, let's say we have a constant text and we want to make the value that we assigned to it a string. If we don't do it and we don't use this angle bracket operator here, then the type of the text constant is gonna be hello TypeScript, because string literals can be used as types.
[09:34 - 09:42] And often that's not what you want, so you will use this. Angle bracket operator to specify that the type of the text constant is just a string, so a broader type.
[09:43 - 09:57] But the problem with this operator, as you already can see, is that it is using angle brackets, just like the .jsx elements do. And in order to let TypeScript know what to expect, how will we use these angle brackets, we need to specify the file format.
[09:58 - 10:13] So if the file format is .jsx, then TypeScript will assume that, all right, any angle brackets are gonna be .jsx elements. Or in case of .js files, it will know that there is not gonna be any .jsx, it's gonna be type assertion.
[10:14 - 10:19] You can read more about this issue in the TypeScript documentation. Now let's talk about the specific files in the src folder.
[10:20 - 10:27] Let's start with the index.tsx. It is the most important file in this src folder, because it is an entry point for our application.
[10:28 - 10:37] It means that Webpack will start to build our application from this file. And then we'll recursively include all other files using the import statements that lead to other files.
[10:38 - 10:42] So let's look at the contents. We have a bunch of imports, and first of all, we import react.
[10:43 - 10:52] We use it to set the strict mode in our application. We import react DOM to be able to render our react component tree into the root element.
[10:53 - 10:59] As you remember, it is in the public index.html divid root. Let's go back to the index.tsx.
[11:00 - 11:04] We import the styles. This file contains styles relevant to the whole application.
[11:05 - 11:09] So we import it here in the entry point. Then we import the app component.
[11:10 - 11:15] And after that, we import the report web vitals. This module can be helpful if you want to measure your app performance.
[11:16 - 11:22] It is explained in full detail in the create react app documentation. As it is not specific to TypeScript, we're not going to focus on it.
[11:23 - 11:32] After we got all the imports, we render the app using the react DOM render. Note that by default, the app component is going to be wrapped into React strict mode element.
[11:33 - 11:40] React strict mode mostly checks that no deprecated methods are being used. Also, all those checks are performed only in development mode.
[11:41 - 11:49] And it is a good practice to wrap your app into react.strict mode. React Jaz documentation contains the continuously updated list of the strict mode functionality.
[11:50 - 11:54] We're not going to change much in this file. So let's move on to the app.tsx.
[11:55 - 12:05] If you use modern create react app, this file won't be very different to the regular JavaScript version. And thing is that currently you don't really need to import react neither in JavaScript or React files.
[12:06 - 12:16] So we can safely remove this file. But previously you had to import react module because internally, all those JSX elements are just React namespace function calls.
[12:17 - 12:27] So div, for example, is react.createElement.div, header is the same for header and so on. With the modern React versions, they removed the necessity to import react from React.
[12:28 - 12:38] Apart from that, this file doesn't have much difference from the JavaScript version of this file in the regular create react app setup. The next file that might be interesting to us is react up and dts.
[12:39 - 12:55] This file references the types from React scripts. And if you command click on them, it will open this file where you will see type declarations for a bunch of image type modules like gpeg, GIF and BMP, for example.
[12:56 - 13:03] Here you might think, why do we need type declarations for the style sheets and images? And thing is that TypeScript does not even see the static resource files.
[13:04 - 13:13] It is only interested in files with .tsx.ts and d.ts extensions. With some tweaking, it will also see the .js and .jsx files.
[13:14 - 13:19] So let's get back to the app. App.tsx and here we're importing the logo from the logo.svg.
[13:20 - 13:32] Unless we would have those type definitions, TypeScript would have no way of telling what type will this logo here have. And if you look at the type definitions for the SVG, you will see that there are two options.
[13:33 - 13:38] If we are using the default import, it's gonna be a string. Let's verify it.
[13:39 - 13:44] We'll go to app.tsx and check the type of logo. And yes, indeed, logo is a string.
[13:45 - 13:51] Alternatively, we could have used the named import. And then it would be called React component.
[13:52 - 13:54] Let's change that. React component.
[13:55 - 13:58] And then we'll be able to render it as a React component. Let's check the type.
[13:59 - 14:06] And it is a component that accepts all the props of SVG element. Let's undo the changes and get back to the definitions.
[14:07 - 14:17] And this is where this React component is defined. Here it says that this React component constant should have type of function component that receives the SVG props often SVG element.
[14:18 - 14:30] And this ampersand here means that among with other props, it also should accept that title of type string. Now, this code might be too hard to understand right now before we discuss TypeScript generics and intersection types.
[14:31 - 14:48] So I suggest you go back here and check if you can understand it better after we discuss those topics later in this course. Other than that, in our SRC folder, we also have the tests defined for our application, the logo asset that we were just discussing and the helper module that helps us properly set up the tests.
[14:49 - 14:55] Let's make sure that everything works correctly and launch our application. Open the terminal and run yarn start.
[14:56 - 15:05] Or if you use NPM, you can run NPM, run start. After your app is built, it should open the local host port 3000.
[15:06 - 15:09] And if you see the same spinning React logo, Then everything is fine.