How to Create a React Column Component Layout
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
Lesson Transcript
[00:00 - 00:12] Create column components. In this section, I won't explain how React components work. If you need to pick this knowledge up, refer to the React documentation, make sure you know what props and state are and how life cycle events work.
[00:13 - 00:25] We'll start with a column component. Create a new file in the SRC folder, column TSX. Here we'll import column container, column title, and card container from the styles.
[00:26 - 00:39] Define column props, type column props. For now, it's going to be an object that will contain only the field text. It's going to be a string. We'll use it to display the column title. Expert, const, column.
[00:40 - 00:56] Here we define the column component. We'll use the text from the props, and we specify the type of the props, column props. We don't use functional component or FC type to define the column component because we don't need the children prop to be part of the props list.
[00:57 - 01:08] Return the layout, column container. Inside of it, we render column title. Here we render text that we got from the props. Then we render card container.
[01:09 - 01:28] And here we'll mock the cards for now. Generate app scaffold, and then to others with text, learn, type script, and begin to use static typing. So this component will receive the text prop and render it as a column title.
[01:29 - 01:47] Update app TSX to render the column component. Column text equals to do and import it from the column module. Remove the unused styles and we are done. How to define props? You can use a type or an interface to define the form of your props object.
[01:48 - 01:57] Most of the time, types and interfaces can be used interchangeably. We'll get to some differences later. In our column component, we define the props as a type.
[01:58 - 02:14] Or in other words, we defined a type with field text, of type string, and assigned an alias, column props to it. Now if we say that some variable has typed column props, it will mean that this variable is an object that has a field text of type string. This is what we did with the props of the column component here .
[02:15 - 02:24] To use this type for our component props, we specified it as a type of our functional component first argument. We also immediately destructured this object to get the field text from it.
[02:25 - 02:31] By default, all the fields you define on your types are required. It means that if the field will be missing, you will get a type error.
[02:32 - 02:39] To make the field optional, you can add a question mark to it. In this case, TypeScript will conclude that text can be undefined.
[02:40 - 02:45] How to accept children prop? There are several ways to define the children prop on your props type.
[02:46 - 02:54] Use the FC type for the component. The first option is to use the React functional component or its alias, Re active C, as your component type.
[02:55 - 03:08] Here the functional component or FC, it is basically the same type because FC is an alias to functional component type. And it's a generic type that receives the props to which it will add the children prop.
[03:09 - 03:19] Use props with children. Alternatively, we could use the React props with children type that can enhance your props type and add the definition for children prop.
[03:20 - 03:37] Here is how React props with children type is defined. It's a generic type that gets a type variable p and then it combines this p or props because you're supposed to pass the props to this type with the type with field children of type React React node.
[03:38 - 03:41] The letter p is called a type argument. It works similar to function arguments.
[03:42 - 04:01] We can pass an actual type with which this type will be used instead of this letter. So for example, we define column props, we use props with children to do it, and we pass an object with the field text string to props with children as letter p or as type argument p.
[04:02 - 04:18] Then the resulting type of column props is going to be an intersection type between object with text string and object with field children that is optional of type react react node. The ampersand combines two types into one.
[04:19 - 04:27] In TypeScript, this is called a type intersection. Here having the type combined from two other types is almost the same.
[04:28 - 04:35] In a lot of cases, it's practically the same as having a type having both of those fields. You can also define the children prop manually.
[04:36 - 04:42] Here is the type column props where we manually define an optional prop children of type react react node.
This lesson preview is part of the Fullstack React with TypeScript Masterclass course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack React with TypeScript Masterclass, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
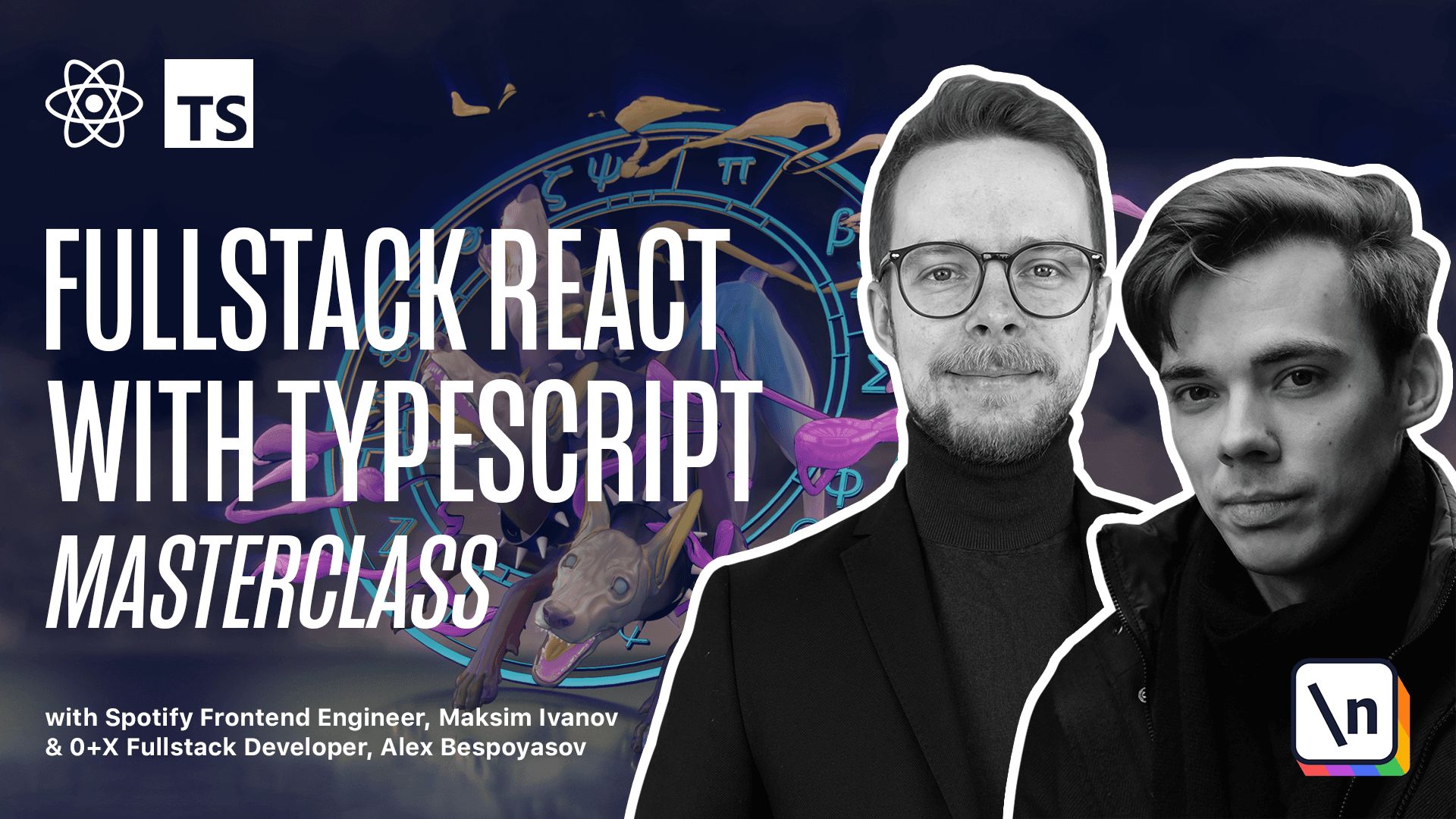