How to Use Redux Toolkit createAction to Simplify Actions
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
Lesson Transcript
[00:00 - 00:09] Using Create Action. Right now we will find a type constant and an action creator for each action in our project. Redux Toolkit provides the Create Action method that simplifies it.
[00:10 - 00:18] When you use Create Action you only need to provide the Action Type string to it. The resulting Action Creator will set whatever arguments you pass to it as the Action Payload.
[00:19 - 00:26] In TypeScript we need to specify the form of Payload in advance. This is why we set the Payload Type as the generic argument value.
[00:27 - 00:33] We are going to start with the Endstroke Action. It is going to be used by multiple reducers, so we will define it in a Shared Module.
[00:34 - 00:44] Create a new file, SRC, Modules, Shared, Actions, TS. Here we will need to import any action and create action from Redux.js Toolkit.
[00:45 - 00:56] Impert, any action type and the Create Action method from Redux.js Toolkit. We also need to import the Stroke Type.
[00:57 - 01:03] Impert, Stroke from Utils, Types. Define the Shared Action Type.
[01:04 - 01:25] Expert Type, Shared Action equals any action or return type of the Endstroke Type. Here we combine the types of the Endstroke Action Creator and the any action so that this action is supported by our reducers.
[01:26 - 01:39] This comes from the fact that any action makes the Payload optional. And this is exactly what the reducer type, the reducer function type, expects to get according to Redux Toolkit type definitions.
[01:40 - 01:47] Now let's define the Endstroke Action. Expert, Const and Stroke equals Create Action.
[01:48 - 01:55] Here we pass in the type of the Payload. It's going to be Stroke and History Index of Type Number.
[01:56 - 02:05] Pass in the Type and Stroke. Now go to SRC Modules History Index, Actions and remake it to use Create Action .
[02:06 - 02:17] We can remove all the previous definitions and we don't need the Constance. Impert, any action, Create Action from Redux.js Toolkit.
[02:18 - 02:32] Expert, Const, Undo equals Create Action with Type Number and we call it Undo. And same with Redo but now we don't need to pass any Payload so we just pass the name Redo.
[02:33 - 02:50] Define the Action Type, Expert Type, Action equals any action or return type, type of Undo or return type, type of Redo. Don't forget to update the History Index Reducer.
[02:51 - 03:11] Impert the Action, Undo and Redo from the History Index actions and the End stroke from the Shared Actions. Now the Type here is going to be Action and the cases will use the Action Creat ors and Stroke to String, Undo to String and Redo to String.
[03:12 - 03:46] Go to Current Stroke Actions, remake it to use Create Action method, Expert, Const, Begin, Stroke equals Create Action Point and the Type is Begin Stroke Expert, Const, Update, Stroke also has Payload of Type Point but has the name Update Stroke and the Set Stroke Color is going to be Create Action, String, Set, Stroke, Color. Let's define the Action Type, Expert Type, Action.
[03:47 - 04:12] Let's define any action or return Type, Type of Begin, Stroke or Return Type, Type of Update, Stroke or Return Type, Type of Set, Stroke, Color. Alright, go to the Reducer file, remove the old Action Impert, now we'll import the Action Creators generated with Create Action.
[04:13 - 05:40] import the end stroke from shared actions and update the reducer. Now we're gonna use the action creators begin stroke to string update stroke to string set stroke color to string and and stroke to string. Now go to strokes reducer, import the end stroke and shared action from shared actions update the reducer. Now the type of the action is going to be shared action and we use the end stroke action creator as the type here. Case and stroke to string. Alright, let's update the app component, import and stroke from modules, shared actions, update the start drawing. Here we'll need to pass an object with x offset x and y offset y instead of two separate arguments. Update the end drawing. Here we also pass an object instead of two arguments. It will contain history index and stroke and also update the draw method. It's gonna also pass an object with x and y. Launch the app, yarn start, open the browser, you should be able to draw the strokes and do redo change colors and it should basically work.
This lesson preview is part of the Fullstack React with TypeScript Masterclass course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack React with TypeScript Masterclass, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
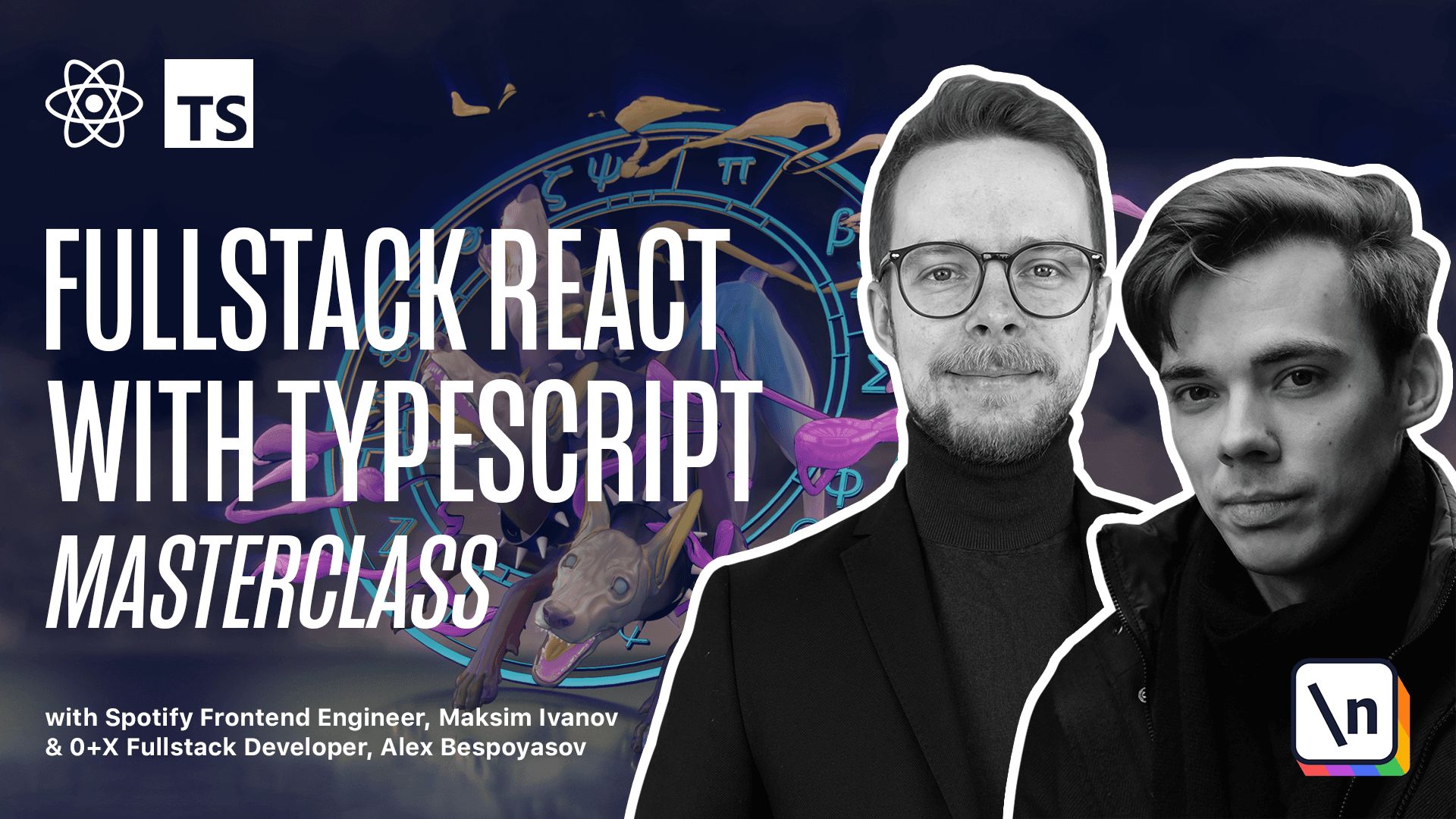