How to Use the Canvas API to Draw in a React Redux App
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
Lesson Transcript
[00:00 - 00:09] Working with Canvas, we'll use the Canvas API to handle drawing. First of all, add the Canvas styles to the index CSS file.
[00:10 - 00:32] Canvas should have transform, translate 3D - 50%, 0, 0. Cursor should be URL, icons, pencil, PNG, 0, 34, comma, or add some margin, 100 pixels, 50%.
[00:33 - 00:49] Copy the pencil PNG from the attached code example and put it into a src icons folder. After it's done, create a new folder, src, utils, and there define a file, canvas, utils, TS.
[00:50 - 00:58] First of all, we'll need a function to clear the Canvas. Expert, const, clear, canvas is a function that will receive Canvas as an argument.
[00:59 - 01:04] The type of Canvas here should be HTML, Canvas, element. We don't need to import this type.
[01:05 - 01:08] It's defined directly. Get the context from the Canvas.
[01:09 - 01:13] Context equals Canvas. Get context to D.
[01:14 - 01:17] We need to check if the context exists. If not context, return.
[01:18 - 01:33] Specify the field style, white, and then fill the react the size of the canvas. Fill, react from the 0 point till the canvas, width, and canvas height.
[01:34 - 01:43] Alright, now we have a function that will draw a rectangle the size of the canvas and fill it with white color. That's the same of just erasing everything that we have drawn.
[01:44 - 01:49] Now let's define the setCanvasSize function. Expert, const, set, canvas size.
[01:50 - 01:58] This function should also receive Canvas and also width, number, and height arguments. Those are going to specify the size of our canvas.
[01:59 - 02:13] We set canvas width to width multiplied by 2. That's because I'm using rednet display, canvas height equals height multiplied by 2 canvas style.
[02:14 - 02:21] Width equals a string saying width pixels, same with the height. Then we set canvas.
[02:22 - 02:31] Get context to D to use scale to context can be undefined. So we add optional chain here.
[02:32 - 02:35] Now go to SRCUp.tsx. Define the constants for the canvas size.
[02:36 - 02:46] Const width equals 1024, const height 768. Now let's get the canvas ref.
[02:47 - 02:56] Const, canvas ref equals use ref of type HTML canvas element. Default value is null.
[02:57 - 03:12] Below the title bar, render the canvas, pass in the canvas ref. Define a helper function, const get canvas with context is a function that will get canvas with default value canvas ref current.
[03:13 - 03:28] And then it's going to return an object with canvas and context that will be calculated from the canvas with optional chaining get context to D. And then we can define a side effect that will get the canvas with the context.
[03:29 - 03:36] Check that both exist, set canvas size and default line styles. And then clear the canvas so we can start working with it.
[03:37 - 03:41] Define use effect. This use effect should get triggered when we mount our component.
[03:42 - 03:50] So we pass an empty array. Then we get canvas and the context using the get canvas with context.
[03:51 - 04:05] If there is no canvas or there is no context, then we return. Otherwise, we set canvas size to the constants that we defined with and height.
[04:06 - 04:19] We set the default line styles context line join round context line cap round. Here we define the styles of the points where two lines will join.
[04:20 - 04:26] Round option will make our lines look smoother. The line cap defines the style of the ends of the line.
[04:27 - 04:33] Again, it's just make the lines, the strokes that we're going to be drawing a bit smoother. Then we specify the line width.
[04:34 - 04:36] I chose five. You can choose the value that you like.
[04:37 - 04:45] And actually you can enhance this application and make it possible to choose the width of the strokes. And next we set the default stroke style.
[04:46 - 04:54] We're going to use black strokes, but later we'll implement selecting colors. All right, now we have everything prepared and we can clear the canvas.
[04:55 - 04:56] All right, our canvas is ready for the first strokes.
This lesson preview is part of the Fullstack React with TypeScript Masterclass course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack React with TypeScript Masterclass, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
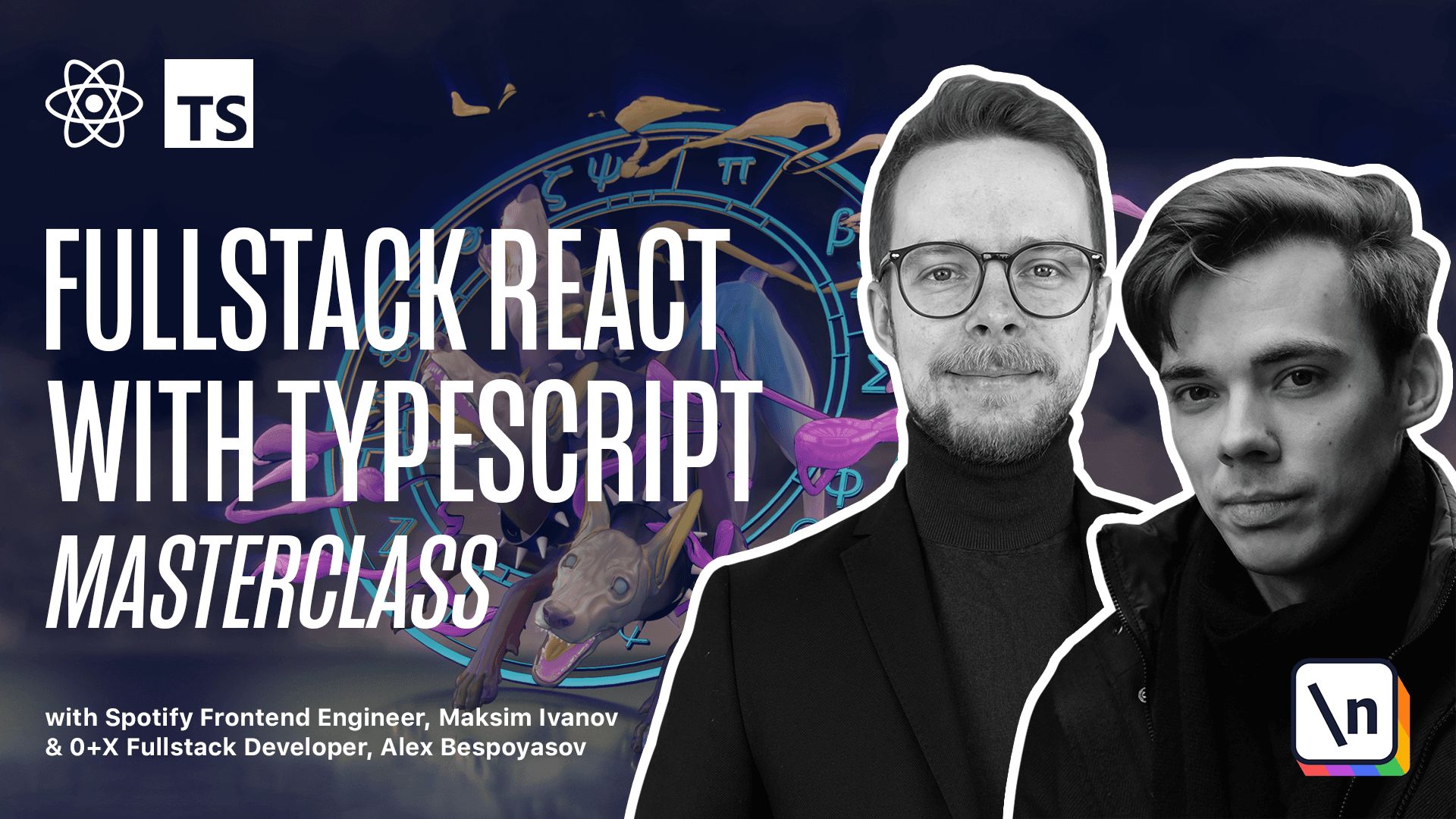