What is Redux? An Intro to React State Management
This lesson preview is part of the Fullstack React with TypeScript Masterclass course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack React with TypeScript Masterclass with a single-time purchase.
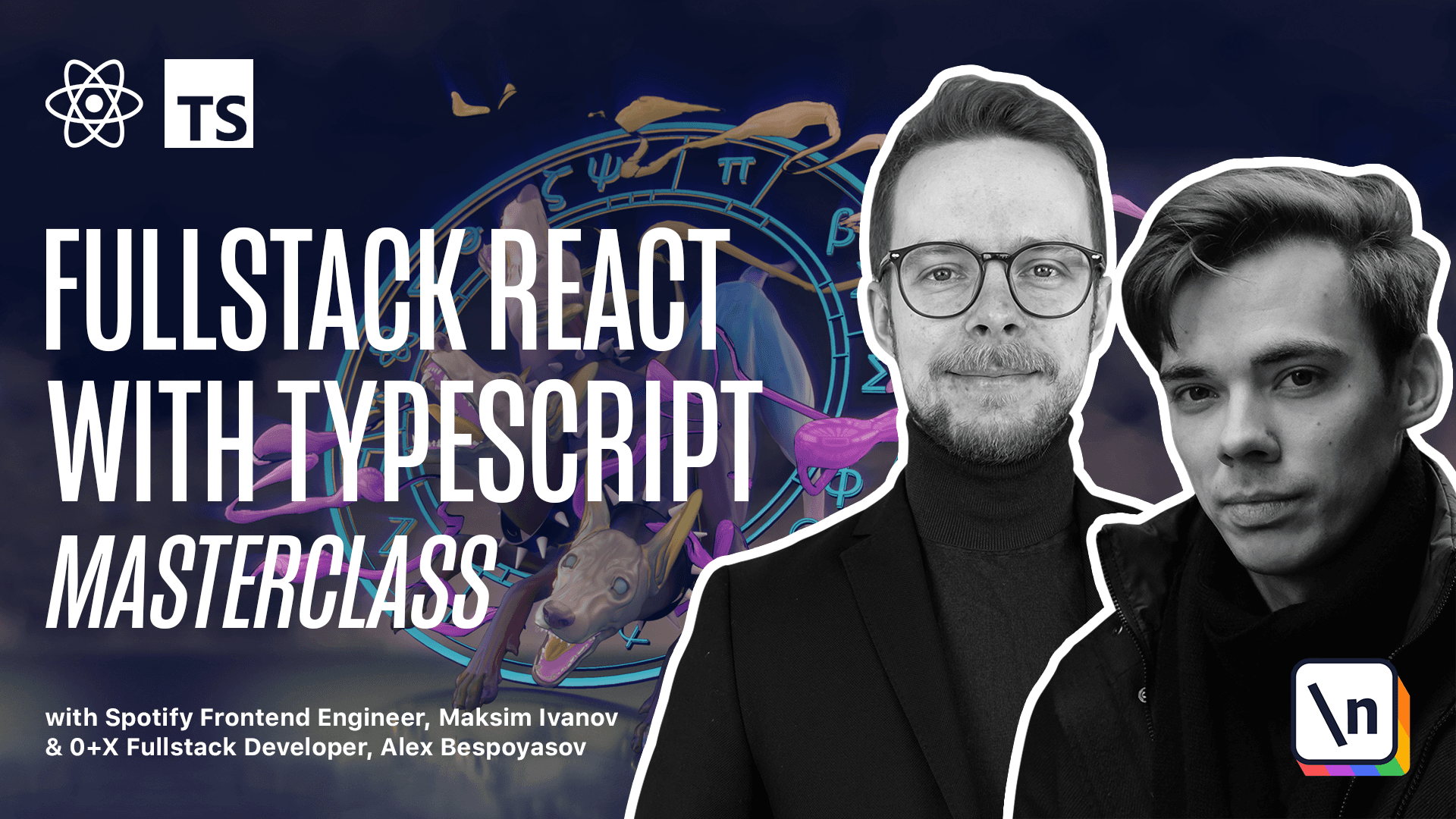
[00:00 - 00:09] What is Redux? Redux is a state management framework that is based on the idea of representing the global state of the application as a reducer function.
[00:10 - 00:16] Or as they state on the main page, it's a predictable state container for JS applications. Let's look at an example.
[00:17 - 00:24] When you work with Redux in your application, usually you start by creating a global store. You do it using a special function called CreateStore.
[00:25 - 00:30] You provide the reducer to it and the initial value of your state. The initial value can be of any type.
[00:31 - 00:33] Usually it's an object. In our example, it's a string.
[00:34 - 00:46] The reducer function that you pass as a first argument accepts two values. The previous state value that Redux will continuously pass to this function, to this reducer.
[00:47 - 00:51] And the action. Think of the actions as of some sort of events.
[00:52 - 00:59] They have a mandatory field type. And then some payload, which is an optional field that can contain some additional data.
[01:00 - 01:07] So in case of the set value action, we can pass the value of this new value. So let's say we dispatch an action.
[01:08 - 01:12] As you can see, it can be just an object. The only hard requirement here is that it has that type field.
[01:13 - 01:26] This will trigger the state update, which means that Redux will call the redu cer. It will take the state value, initially it's going to be the one that will pass to the CreateStore function and an action.
[01:27 - 01:35] Inside of the reducer, we must return the new state value. Usually you will see the switch statement that will check action type.
[01:36 - 01:43] And depending on this type, we'll perform different changes on the state. We'll perform different calculations that will return the new state value.
[01:44 - 01:52] In our case, our state is a string. So if we want to set a new value and action type is set value, then we can just return action payload.
[01:53 - 02:05] Because what we have in the action payload is actually the new value that we want to store in our state. Otherwise, if we got some other type or we got an unknown action type, we just return the state unchanged.
[02:06 - 02:15] You can find this example code attached to the course. It is located in the Redux TypeScript chapter in the folder called Redux Example.
[02:16 - 02:22] You can play with this example to gain some additional practice. Try for example, dispatching some more actions and see what happens with the state.
[02:23 - 02:30] But why can't we use the useReducer hook instead of Redux? Since version 16.8 React supports hooks.
[02:31 - 02:43] One of them, useReducer, works in a very similar way to Redux. In the first part of this course we created an application managing the application state using combination of userreducer and React context API.
[02:44 - 02:52] It was a Trello clone. If you need the refresher, you can find a userreducer example in the code 01 FirstUp userreducer folder.
[02:53 - 03:03] So why do we need Redux if we have a native tool that allows us to represent the state as a reducer as well? If we make it available across the application using the context API, won't that be enough?
[03:04 - 03:10] Well, Redux provides a bunch of important advantages. One of them is the existence of the browser tools.
[03:11 - 03:16] For example, Redux DevTools. It is a great tool to debug your Redux code.
[03:17 - 03:30] It allows you to see the list of dispatched actions you can see here on the left. You can inspect the state on the right panel and you can even time travel and inspect how your state was changing after each dispatched action.
[03:31 - 03:39] Another benefit of using Redux is that it's easier to handle the side effects. With userreducer you have to invent your own ways to organize the code that performs network requests.
[03:40 - 03:50] You remember it if you went through the first lesson where we had to implement our own system to save the state on the back and end to load it again. And it was a bit cumbersome.
[03:51 - 03:58] So using Redux would actually be a bit easier there. For example, Redux provides the middleware API to handle side effects.
[03:59 - 04:17] For example, fetch data when you dispatch a certain type of action or send the data to backend and things like that. Using Redux middleware API will probably be a bit too low level and there are better tools like Redux Thunks that allow you to use functions as actions.
[04:18 - 04:28] In this case, when you dispatch such action like Thunk, it will execute this action where you can have your asynchronous logic. In this part of the course we're actually going to use Redux Thunks.
[04:29 - 04:37] Among other benefits, I would also list the ease of testing. As Redux is based on pure functions, it is easy to test.
[04:38 - 04:50] All the tests well down to checking the outputs with the given inputs. Here for example, we pass undefined as a state and empty object as an action and we expect to receive the default state.
[04:51 - 05:00] If you look at the other tests, they follow the same structure. We pass the previous state and the action and verify that the value matches with the one we expect.
[05:01 - 05:07] No side effects, no headaches. And finally I would mention patterns and code organization.
[05:08 - 05:17] Redux is well studied and there are recipes for almost all the problems. There is a methodology called Dux that you can use to organize the Redux code.
[05:18 - 05:43] Or you can go in another direction and use Redux Toolkit, a framework that is built on top of Redux and provides a higher level API which makes it much easier to work with Redux. Closer to the end of this part of the course, we will actually rewrite our application to use Redux Toolkit so that you compare how easy it is to use Redux Toolkit compared to regular bare bones vanilla Redux.