How to Export an Image From a React Redux App
This lesson preview is part of the Fullstack React with TypeScript Masterclass course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack React with TypeScript Masterclass with a single-time purchase.
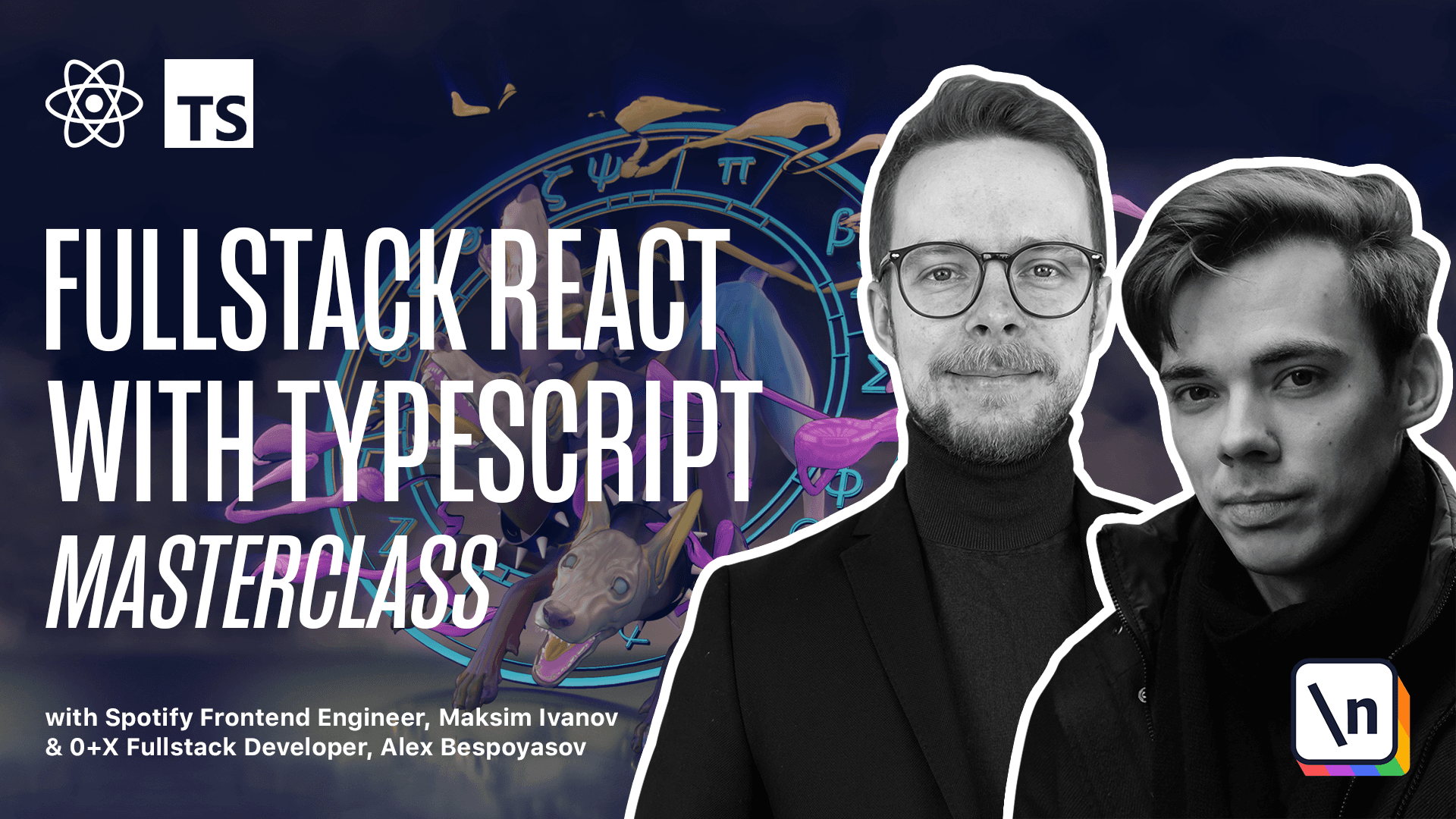
[00:00 - 00:07] Exporting an image. Let's allow exporting the picture to a file. To do this we'll need to retrieve the bitmap information from our canvas.
[00:08 - 00:14] Then we'll need to transform it into a blob and then save it as a file locally. The file saving logic will be defined in a separate component.
[00:15 - 00:22] We'll use the React context API to make the canvas reference available there. First let's define the canvas provider. Create a new file as you see.
[00:23 - 00:29] Canvas. Context. TSX. Here we want to export const canvas. Context.
[00:30 - 00:38] Defined using the create context method that we import from React. Provide the type. Here we're going to store the ref object.
[00:39 - 00:45] That will contain the HTML canvas element. We know this because we know that we're going to store canvas there.
[00:46 - 00:52] Impert the ref object type from React. Now we're going to pass an empty object as the default value for the canvas.
[00:53 - 01:08] As you know this default value is only going to be used if you don't wrap your application into the context provider. And we don't really care about this case. So we can just pass the empty object and cast it to ref object, HTML, canvas, element.
[01:09 - 01:13] Let's format the document and save it. Alright, let's define the canvas provider now.
[01:14 - 01:28] Expert const canvas. Provider is going to be a component that should receive children. We can do it by using props with children and passing an empty object there as a type of the props.
[01:29 - 01:44] You can import the props with children type from React. Alternatively, you could just define the canvas provider as FC or functional component and not pass any props either because canvas provider doesn't require any props, except for children.
[01:45 - 01:59] And then you could just remove the props with children and you would already have the type of the children prop defined. Alright, right now canvas provider is complaining that we're not returning anything from this functional component and we must return some React element.
[02:00 - 02:22] So let's continue with the layout. We're going to store the canvas ref here. So let's define it canvas ref equals use ref of type HTML canvas element, set the default value to null and now return canvas context provider inside of which render the children, provide the value and here we pass the canvas ref.
[02:23 - 02:31] Alright, import the use ref hook and format the document. Now to make it easier to use our canvas provider, let's define a helper hook called use canvas.
[02:32 - 02:41] Expert const use canvas equals a function that inside of it will call use context canvas context. Import use context from react.
[02:42 - 02:58] So we basically created a wrapper around use context that we would otherwise use in our components and now instead of using use context and we would actually have to provide the canvas context to it every time we can use use canvas without providing any additional properties. Okay, our provider will store the reference to the context.
[02:59 - 03:09] Now we need to go to index TSX and wrap our application into this provider. Import canvas provider from canvas context.
[03:10 - 03:20] Wrap the up into canvas provider. Now we can go to SRC up TSX remove the use ref and instead define the canvas ref using use canvas.
[03:21 - 03:25] Let's import it from the canvas context. Now define the get canvas image.
[03:26 - 03:36] This is the function that will get the blob from this canvas. Inside of the utils canvas utils define a new function expert const get canvas image.
[03:37 - 03:49] It's going to be a function that will receive the canvas of type HTML canvas element or now. It's going to be an async function because it will return a promise that will resolve to now or blob.
[03:50 - 04:13] Inside of this function return a new promise pass a promise callback this callback should receive resolve and reject callbacks. If there is no canvas then return reject null otherwise we convert canvas to blob and pass the resolve function as a callback to the to blob method of the canvas.
[04:14 - 04:25] If we check the to blob definition it receives a callback and there it will pass the blob value of the canvas. When we pass the promise resolve function there it will make it so that the promise will get resolved with this blob.
[04:26 - 04:45] Now let's create the file panel that will allow us to save the drawing as an image. Inside of the shared folder create new file call it file panel.tsx Here we expert const file panel that's going to be a functional component and we'll need some styles for this panel.
[04:46 - 05:11] Go to index.css and add a new class file is going to have position fixed bottom 40 pixels right 20% and that index 10. We also will need to use a package called file saver and it doesn't have types provided with it so we'll need to install the types separately using yarn add file saver.
[05:12 - 05:18] And also types for the file saver. The file saver is installed we can go back to the file panel.
[05:19 - 05:32] Here we'll get the canvas ref const canvas ref will use the use canvas that we 've defined in the beginning of this lesson. Then we define the expert to file function const expert to file.
[05:33 - 05:56] It's going to be an async function that gets the file const file equals await get canvas image from the canvas ref current and then if there is no file it just returns and does nothing. Otherwise it calls the save as method from the file saver passing the file and the file name growing PNG.
[05:57 - 06:23] Let's define the layout of this component return here we have a wrapping div with class name window and file inside of it there will be a title bar with a title bar text saying file. And then we want to have window body with field row and inside of it a button with save button class saying expert add a non click handler there that will call expert to file.
[06:24 - 06:42] Now we have the file panel component we can go to the app component scroll down to the layout and add it after the color panel file panel. Open your application try drawing something for example a little froggy here you go some cute little eyes some legs.
[06:43 - 06:48] Now let's expert it to a file and we can store it as a drawing file.