How to Load Project Files From a React Redux Server
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
Lesson Transcript
[00:00 - 00:18] Load the project. In this lesson we'll make it possible to load the projects from the server. First we'll update the types. Open SRC, Utils, Types and define a project type here. Expert, Type, Project = an object with fields image of type string, name of type string and ID of type string.
[00:19 - 01:06] Update the root state as well. It will now have the projects list field, projects list and it's gonna have three fields, error, string, Boolean flag pending, Boolean and projects array of type project. We have the error and the pending fields because we're gonna fetch the projects from the backend. So we'll need to manage those states somehow. Define the API module. All our project loading logic will reside in a separate module. Create a new folder, SRC, modules, project, projects list and here create a new file api.ts. Define a function fetch project list, expert const fetch projects list. It's gonna be a function that we'll call fetch, hdp, local host, 4000 projects. After that we get the response and parse it as JSON.
[01:07 - 08:35] Create a project list slice. Inside of the project list folder, create a new file, slice.ts. Add the imports, we'll need create slice and create a syncthunk from Redux toolkit, root state from the types and fetch project list from the API module we've just defined. Define the initial state, const initial state is gonna be root state field projects list. It's gonna be an object with fields error undefined by default pending as you remember it's a Boolean flag and by default it's false and projects it's gonna be an empty array. Now define the slice, const slice equals create slice, provide an options object with name, projects list initial state reducers is gonna be an empty object and extra reducers is gonna be a function that receives a builder and then let's add cases for each of the actions we're gonna process builder add case. Here we are gonna be processing the get project list thunk that will have pending fulfilled and rejected states. Let's define the thunk first, export const get projects list create a syncthunk the type is get projects list it's an async thunk and inside of it we return fetch projects list we don't have to do anything else async thanks provided by Redux toolkit are quite smart so if our fetch project list function will fail or will reject the thunk will reject as well and it will dispatch the rejected action otherwise it's gonna be fulfilled and will dispatch the fulfilled action and in the meantime while it's loading it will dispatch the pending action. Now let's add the cases first case is gonna be for the pending state get projects list pending here will receive the state and we 'll set state pending to true. Next let's add the fulfilled state builder add case get projects list fulfilled here we receive the state and the action we set state pending to false state projects to action payload and state error to undefined and finally the rejected state this is where something went wrong and we got an error builder add case get projects list rejected again we receive a state we don't care about the action we set state pending to false and state error here we can set it to something went wrong now let's export the reducer expert const projects list equals slice reducer and expert a bunch of selectors expert const projects list selector equals a function that receives state of type root state and returns state projects list projects we also need to define the projects pending selector here we return projects list pending and projects list error selector by the way projects a list pending selector should be the name of the previous selector so projects list error selector returns project list error go to the store import the projects list reducer from the modules projects list slice added to the reducer projects list and now we are able to load the projects list let's add the code to load the individual project go to src modules strokes api ts and define a new function expert const get project it's going to be a function that will receive project id of type string and it will call fetch http local host 4 000 projects and then pass in the project id after we got the response we parse it as json go to the slice and define a funk that will call this fetch function import the get project from the api and define the funk expert const load project equals create a sync funk of type load project and we pass in a callback a sync project id is a string and it will try to get the project using await get project using the project id that will pass as an argument project id if it's successful then it will return project strokes otherwise if we catch an error then we'll just log this error scroll to the slice and add another extra reducer builder add case load project fulfilled here we want state and the action and in the body of the handler we return action payload now we can load the list of the projects and the individual project let's work on the UI first we want to present the user with the list of loaded projects open src index css and add the following styles projects container here we want to have overflow aura so we can scroll if there are a lot of projects we set the max width the height display flex with justify content flex start and we set the padding and the width of this element next we'll need the styles for the individual project cards project card those are going to be square 100 by 100 pixels with margin 20 pixels and cursor pointer so that we show that they're clickable and text align should be center and we also specify the styles for the image project card img it should be a square image with margin bottom 10 pixels now let's update the project's model component open projects model tss and get the projects list in the components body const projects list equals use selector projects list selector imprint the projects list selector and the use selector hook and define a use effect use effect that when the component will be mounted passing an empty dependency array we will call dispatch get projects list imp reduce effect and the get projects list action creator now when the project model is going to be shown it will trigger the use effect that will trigger dispatch and dispatch the get project list action and then the project list is going to be updated after we get the data from the backend now we'll need to define the onload project event handler define const on load project it's a function that will receive project ID of type string and then here we'll dispatch the load project action with the project ID import the project ID action creator and then we'll also dispatch height to hide this model scroll down instead of the projects list text we will now map through the projects list or an empty array just in case and here we map and for each project we're gonna render div where key of which div is going to be project ID and on click is going to be a function that will call onload project and passing the project ID and then we render the project image img as our c equals project image and alt should be thumbnail render another div that will say project name and then add a class name to the alt rediv it should be project card now go to the app tss find the use effect that updates the canvas depending on the history index and add the strokes to the dependencies array launch the app to verify that you can save and load the project yarn dev open the app try to load the project and it should work congratulations you just wrote your own drawing application using Redux and TypeScript
This lesson preview is part of the Fullstack React with TypeScript Masterclass course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Fullstack React with TypeScript Masterclass, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
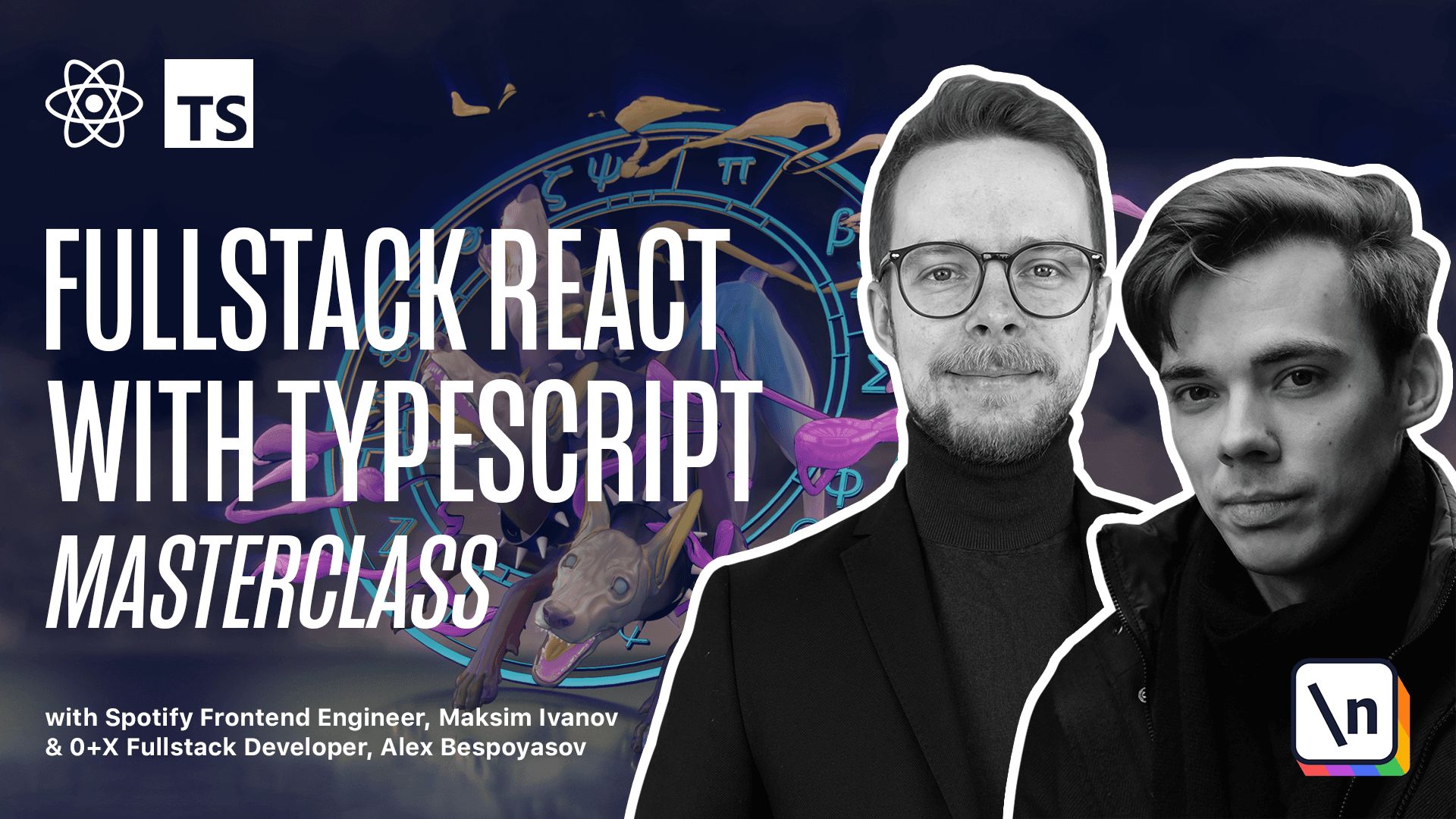