Adding Course Params to Redux
In this lesson, we're going to add Course params to Redux
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
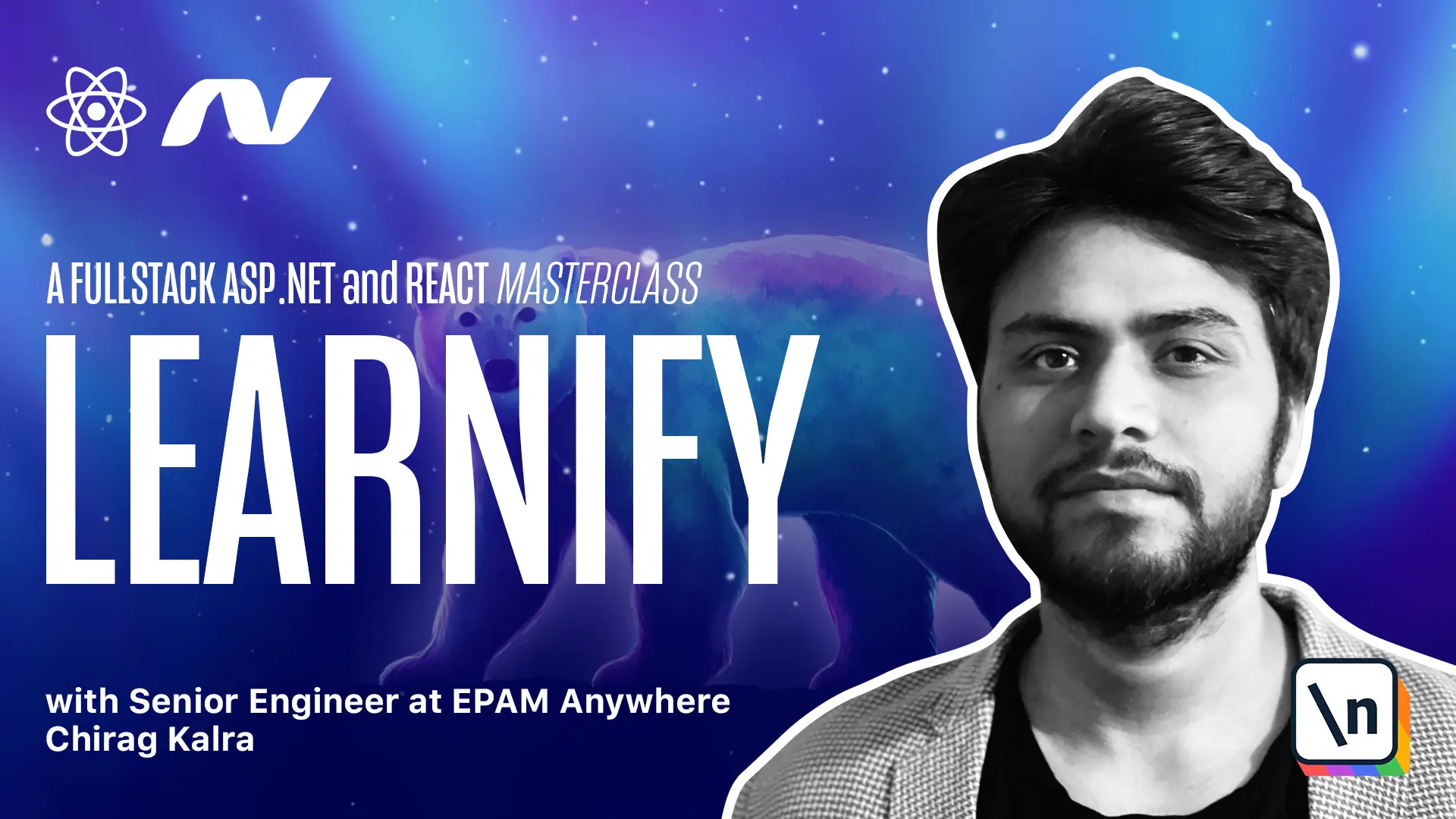
[00:00 - 00:06] Let's start centralizing cause params now. So let's close it and we can start by creating the model.
[00:07 - 00:21] So inside models and let's do it inside cause.ds file. So in the bottom, we will write export interface and let's call it cause params.
[00:22 - 00:31] Cause params. We need sort which will have a type string.
[00:32 - 00:41] We need search of type string. We are not going to have that search always so we can make it optional by using a question mark.
[00:42 - 00:55] But we always want a page index, page index type number. We will need page size, page size of type number.
[00:56 - 01:04] And finally, we also need category which will be again optional. So let me make it optional by using a question mark.
[01:05 - 01:11] And this category will be number because we are using the ID. Let's go to the cause slice file now.
[01:12 - 01:22] So course slice on top of the cause slice. Let's create a function and let's call it get params.
[01:23 - 01:31] So function get params. This will have all the initial value that we want to make.
[01:32 - 01:47] So let's return the initial values and we are going to return the page index, which will be always one. So whenever we initialize the application, the page index will be one, which means we will always fetch the first page.
[01:48 - 01:57] And let's make the page size to be pre for now. And the sort criteria can be by title.
[01:58 - 02:07] So let me write title. And if you remember, we have used title as the value and we also have price descending and price ascending.
[02:08 - 02:15] But by default, we will use sort by title. Now inside the initial state, we can add cost params.
[02:16 - 02:27] So here, that's right. Cause params and the value can be the get params function that we just created.
[02:28 - 02:37] This is basically the default value for our cause parameters. Now inside reducers, we can create a method, which will be called set cause params.
[02:38 - 02:55] And we will call this method whenever we choose any filter. So inside reducers, let me write a function and let's call it set cause params.
[02:56 - 03:09] And like any other reducer function, it will have state and an action. Inside this function, first of all, we will make the courses loaded property to be false.
[03:10 - 03:26] So let's write state dot courses loaded to be false because we want to call the courses again. After that, we will set state dot cause params.
[03:27 - 03:40] And inside, we can use a spread operator for the existing cause parameters, because we might not have all the values inside the cause params to be changed. So I can write state dot cause params.
[03:41 - 03:50] And after that, we can spread action dot payload. This means the parameters that are being changed.
[03:51 - 03:58] And whenever we call this function, we want the page index to be one. So let me write page index to be one.
[03:59 - 04:11] We will make a cause params object with this, but we need to shape it in a way that our server understands it. So what I can do is let's create a new function and which will be called get x use params.
[04:12 - 04:27] So on top, let's create the function. Let's call it get x use params.
[04:28 - 04:49] And this function will take as a parameter cause params, which will have a type cause params like the one we created here. First of all, inside the function, we will initialize the parameters and we can use URL search params that is given by default.
[04:50 - 05:04] So I can write const params is equal to new URL search params. Now we can append all the cost parameters to the params.
[05:05 - 05:10] And to do this, we have the append method. Let's start by appending the page index.
[05:11 - 05:18] So what I'll do is params dot append. And the property is page index.
[05:19 - 05:30] So I will write page index. And as a second parameter, we have the value, so it will be cause params dot page index.
[05:31 - 05:40] And since it only accepts string, we will convert it into a string. Next is the page size.
[05:41 - 05:56] So rather than writing it all over again, let's copy it, paste it. And this should be page size and the value should be page size as well.
[05:57 - 06:00] Next is sort. So let's copy it one more time.
[06:01 - 06:13] And key is sort and the value is cause params dot sort. And we don't have to convert it into a string because it is already a string.
[06:14 - 06:22] Now categories and search are optional. So what we can do is we can check if cause params have category or search.
[06:23 - 06:35] So let's start with the category. We will check if cause params dot category, which means if it is available, only then we want to add it to our URL search param.
[06:36 - 06:51] So the same thing, it will be category ID and cause params dot category dot two string. And the same goes for the search.
[06:52 - 07:09] So I will write if cause params dot search, we can add search to our search params. It should be search and we don't want to convert it into a string because it is already.
[07:10 - 07:22] And finally, from this function, we want to return the params. Now inside the get cause is async function, we can get all the params.
[07:23 - 07:44] We can use the same get access params function and we need to access the cause params state. So for that, we will need the thunk API here, since we don't need the first parameter and we can pass an underscore and the second one can be thunk API.
[07:45 - 08:03] To access all the params, we can write function params, which will be equal to get exus params, the function that we just created. And if you remember this get access params function needs cause params, which we will get using the thunk API.
[08:04 - 08:14] So inside we can write thunk API dot get state. And from the state, we have cost slice.
[08:15 - 08:23] And from the cost slice, we have cause params. We see an error because we haven't defined the state.
[08:24 - 08:40] So here, let's write state to be the root state, which will resolve this one. Now we can pass the params to agent dot courses dot list.
[08:41 - 08:49] Let's see what's causing this error inside causes and list. We have to make the params to be a part of this.
[08:50 - 09:01] So what we can do is make params and make them optional. And the type can be URL search params.
[09:02 - 09:06] This will resolve this error. We can now export this function.
[09:07 - 09:26] So in the bottom, I can write export const and this function is set cause params. And this will be destructured from cause slice dot actions.
[09:27 - 09:32] We can now go back to the homepage. And here we need to import cause params.
[09:33 - 09:40] So on top, we already have courses loaded. We also need cause params.
[09:41 - 09:58] And our radio buttons can now have the value cause params dot sort for the sorting options and cause params dot category for category options. So for this radio dot group, let's keep it down.
[09:59 - 10:11] The value can be cause params dot sort. And for the category radio button, let's keep it down as well.
[10:12 - 10:22] The value will be cause params dot category. We see an error because we haven't given type to our cause params state.
[10:23 - 10:31] So let's do one thing. For now, inside we get initial state, let's make it any so that anything is acceptable.
[10:32 - 10:38] And later on, we will create a separate state for our cause. So for now, let's keep it to any.
[10:39 - 10:49] And here the value is cause params dot category, which is the selected filter for categories. Now we want to pass the data selected to the cause params.
[10:50 - 10:56] For this, we can use on change event. And let's start here.
[10:57 - 11:05] This is on change. We will have access to the event.
[11:06 - 11:19] So let's write small e. And this on change function will dispatch set cause params function.
[11:20 - 11:27] Let's import it. And this is for sorting.
[11:28 - 11:39] So sort will be equal to e dot target dot value. The same way we will pass the category inside category radio group.
[11:40 - 11:54] So what I can do is I can copy it and paste it here. And this one will have category instead rest.
[11:55 - 11:59] Everything can stay the same. Since we are here, let's make the search power work too.
[12:00 - 12:11] If we go to the navigation file, let's go to the navigation. And here we can create a new state called search text.
[12:12 - 12:20] So here, let's write search text. And this will be set search text.
[12:21 - 12:29] We will use use state and the initial value will be an empty string. Let's create a function called handle change.
[12:30 - 12:46] So let me create a function here. Let's call it handle change, which will take the value and store it inside the search text state.
[12:47 - 12:54] We will have access to the event. So let me write e and this event will be a change event.
[12:55 - 13:01] So let's use the change event. This change event is HTML input element.
[13:02 - 13:19] So we can give it a type of HTML input element inside. We can set search text to be e dot target dot value.
[13:20 - 13:27] We can use this function to the on change event to the input. And we also need a function that will dispatch this to the set cause params function.
[13:28 - 13:48] So below this, let's create another function and let's call it on search. This will take an event e and this will be a synthetic event.
[13:49 - 14:04] First of all, if you don't want to submit it and that's why we will use the e dot prevent default, which will just prevent the default behavior of submitting the form. And after this, we will dispatch a function, which will need a dispatch.
[14:05 - 14:39] So here let's use dispatch from use dispatch. And inside this function, we can use dispatch to use the set cause params function, which will simply take search with value search text, which is our internal state.
[14:40 - 14:54] So inside the form, we can use the on change event. This will be equal to the handle change function that we created.
[14:55 - 15:09] And the value will be the search text, which is the internal state. And when they submit the form, so on submit, we want to use the on search function.
[15:10 - 15:21] On search, this is the function that we created here. This will simply dispatch the set cause params with the search value with this search text.
[15:22 - 15:38] And now for all this to work, we need to add optional params parameter to our get request for which we can go to the agent dot t s file. And inside the get request here, we can also pass another argument, which will be params.
[15:39 - 15:47] And since this should be optional, we can add a question mark. And this will have a type URL search params.
[15:48 - 16:03] And now as a second property, we will pass params inside an object. Now our causes dot list method, we can pass params as well.
[16:04 - 16:10] And now we can try and run this inside the browser. Make sure the servers are running.
[16:11 - 16:24] Now if you select any other sorting option, let's say price high to low, you see the cost with the highest price is coming first. And if we select price low to high, we see the cause with the lowest cost is coming first.
[16:25 - 16:30] Now let's also try if the search box is working. Let's search for AWS and press enter.
[16:31 - 16:40] And as you can see, we have a cause which has that AWS keyword. If we refresh it and let's try data.
[16:41 - 16:48] Now if we click data and run see, we see the cause that has data keyword in it. So now that is working fine.
[16:49 - 16:50] Let's start working on the pagination in the next class.