Creating Basket Controller
In this lesson, we're going to create basket controller
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
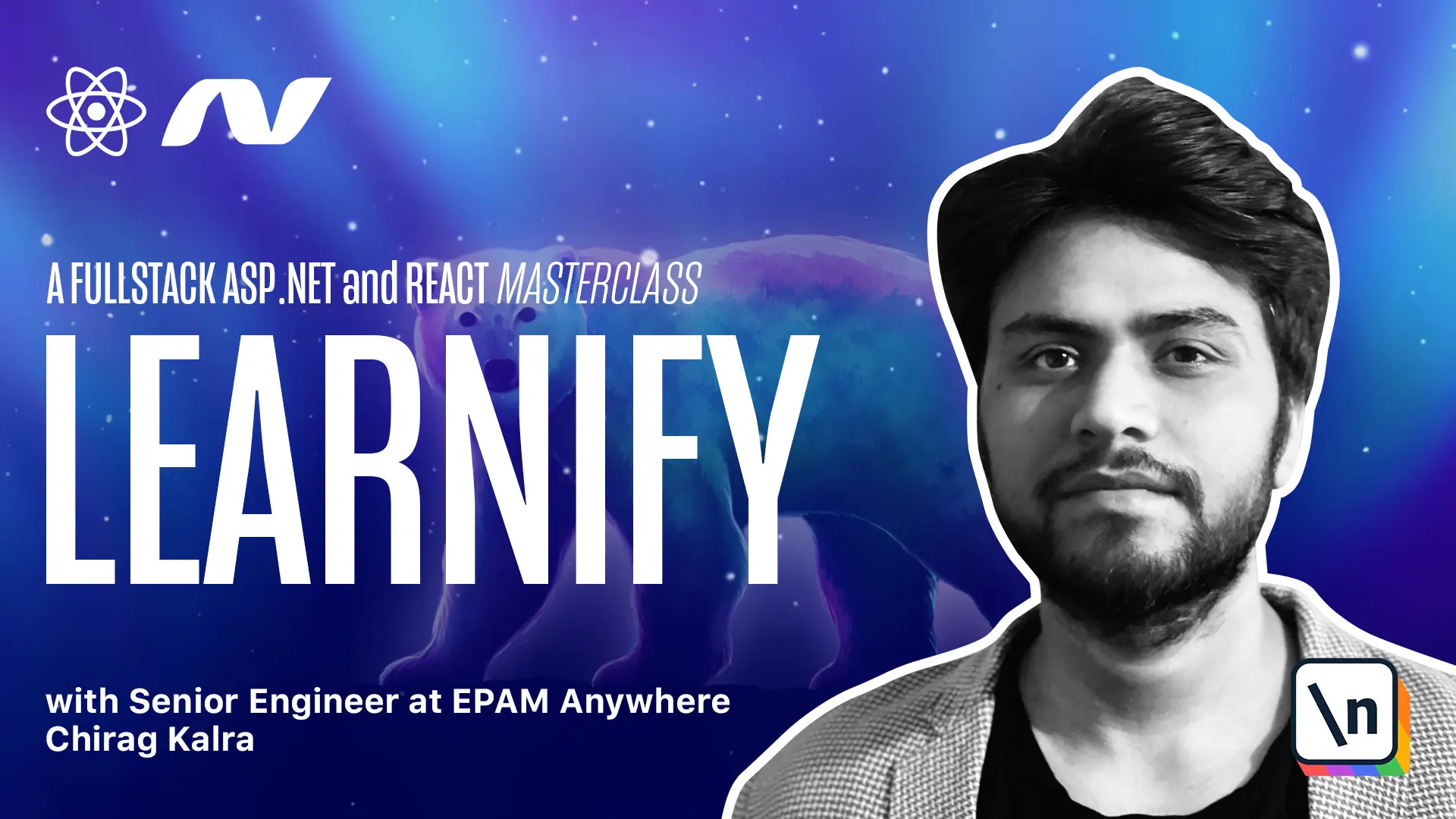
[00:00 - 00:06] We have created our basket and the basket item entity. We have created the migration as well.
[00:07 - 00:19] Let's create our basket controller now. Inside our API project and inside controllers, let's create a new class and we will call it basket controller.
[00:20 - 00:38] We will derive it from our base controller. Let's create our empty constructor and inject our store context.
[00:39 - 00:49] We can simply call it context. That's imported using infrastructure and initialize field from parameter.
[00:50 - 00:55] You must be wondering why we haven't used our generic repository for this. Well, you definitely can.
[00:56 - 01:11] But the main advantage of using the generic repository is when there are multiple use cases and when we have to repeat the code again and again. Let's see how it goes and if there is a need to create the generic repository for these methods, we'll do that as well.
[01:12 - 01:21] For now, let's proceed with the store context and create three methods. Let's start with the get basket method.
[01:22 - 01:44] So on top, we will write HTTP get. Below this, we will write public async task of type action result and here we will return a basket.
[01:45 - 02:02] Let's call this method get basket and import task using system.threading.tasks. Let's import action result using Microsoft ASP net code at NBC.
[02:03 - 02:21] We will import basket using entity. Inside the method, let's create a new variable called basket and inside we will write context.t Now, we need a unique identifier to identify the user's particular basket.
[02:22 - 02:33] How can we do it? Now, when we create a new basket, we can create an ID which we can send as a response which will be saved in a user's browser as cookies.
[02:34 - 03:20] Then with every request, client will send the client ID and this is how we will know to which basket customer is interacting to. Now what we can do is first or default async and check if x dot client ID which is stored in our database is equal to the client's cookie which can be checked as request dot cookies and inside we can pass client ID.
[03:21 - 03:31] Now we can import first or default async using Microsoft entity framework code. Now doing this will give us the basket ID and the client ID.
[03:32 - 03:39] But if you remember, it also has navigation properties. We have items and items have costs.
[03:40 - 03:50] Inside our generic repository, we made a specification for doing this. But since we are not using generic repository here, we can change this with the include statement.
[03:51 - 04:06] So let's take this one down and here let's include the items first. After this, we want to use another navigation property.
[04:07 - 04:21] So what we can do is rather than using include we can use then include. This one will be i goes to dot course.
[04:22 - 04:46] Now if the basket is null, we will return not found response and inside we can pass the new API response that we created with status 404. Let's import API response using API dot error response.
[04:47 - 04:58] If it's not null, we will simply return the basket. Also we need to await.
[04:59 - 05:19] Now let's write another method which is going to add the item to the basket. Now it will be HTTP post public async task of action result.
[05:20 - 05:26] And let's return basket for now. We will create basket dto shortly.
[05:27 - 05:43] Let's call it add item to basket. Our method will need cause ID as a parameter which is of type goed.
[05:44 - 05:50] And let's write cause ID. Make sure it is imported.
[05:51 - 06:00] First of all, we will check if the basket exists. We need to copy the same code as above.
[06:01 - 06:15] And paste it here. Let's create a new method by selecting this and clicking on extract method so that we don't have to create it again and again.
[06:16 - 06:33] Let's call it extract basket and copy it. Paste it here.
[06:34 - 06:44] Think this part and paste it here as well. Now we don't need this statement.
[06:45 - 06:52] What we want is if the basket exists, we will add the item to it. Otherwise we will have to create a new basket.
[06:53 - 07:08] So what we'll do is we'll check if basket is equal to null. We will create a new method which will be create basket.
[07:09 - 07:21] So let me write it here. Let's use quick fix and click on generate method.
[07:22 - 07:44] Here we need to create a client ID first. We can do it by creating a new variable called client ID and we can do it using goed.new goed and we have already imported goed using system.
[07:45 - 07:53] So that's not a problem. And since we want it to be a string, we will use the two string method.
[07:54 - 08:13] To add it to our response, we can use response dot cookies dot append. We can pass the name of the cookie, which is going to be client ID.
[08:14 - 08:28] And after this, we will pass the value, which is the client ID, which we have created right now. We can also pass some additional options to it, such as the expires property or the max age or the path.
[08:29 - 08:37] We need to set two properties. So what we'll do is write options.
[08:38 - 08:52] And it will be new key options. Let's import it using Microsoft ASP net core dot HTTP.
[08:53 - 09:09] And the first property will be is essential, which indicates if the cookie is essential for the application to function correctly. It's essential because without this user won't be able to create a basket or add items to it.
[09:10 - 09:15] So what I can do is make it true. We also need to set the expiry date for this.
[09:16 - 09:31] We have the expires property, which will be equal to date time dot now dot add days. And for now, let's keep it 10.
[09:32 - 09:40] Now we have to pass these options to our cookie. So after the client ID, I will write options.
[09:41 - 09:55] Now we have the client ID so we can create a new basket. So let me create a new variable basket and it will be equal to a new basket.
[09:56 - 10:16] And we will pass the client ID to be this client ID. We can now add this to our database using underscore context dot baskets dot add and we will simply add this basket here.
[10:17 - 10:24] Finally we will return the basket. So now we have created a new basket.
[10:25 - 10:38] Next step is to get the course. Since we have the course ID and the context, we can simply use context method rather than injecting generic repository since we want it only once.
[10:39 - 11:00] What we'll do is go back to our method. We add item to basket method and create a new variable called cause and use context dot causes dot find async and we will pass the cause ID.
[11:01 - 11:17] If the cause is null, we want to return not found with our new API response 404 . So I'll check if cause is equal to null.
[11:18 - 11:50] We will return not found and inside we will pass our new API response class that we created and we will pass the status code 404. If it's not null, we can pass it to our ad cause method, which we created in our entity basket dot add cause item and I will pass this cause.
[11:51 - 12:05] I noticed we need to make it a var and here we forgot to put a wait. And like I told you before, it's not yet stored in our database.
[12:06 - 12:14] What we have to do is use context dot save changes async to make changes to the database. We can also check if the changes were successful.
[12:15 - 12:34] The save changes async method gives us an integer on successful transaction. So if we store it inside a variable, let me create a new variable called result and here I will use context dot save changes async.
[12:35 - 12:49] And if I hover over it, you see it returns an integer. So if it is more than zero and let me put a wait here as well, I will know that the changes have been made.
[12:50 - 12:57] If no changes, it won't be more than zero. So this is a check to see if the changes are actually made.
[12:58 - 13:09] Now we can check if the result exists. So if result, we can simply return the basket.
[13:10 - 13:40] Otherwise we can return a bad request response with our new API response. Our request has status code of 400 and we can also set a message which will say problem saving item with the basket.
[13:41 - 13:48] Also we forgot to await here. So now it's sorted.
[13:49 - 13:51] In the next lesson, let's create our remove item method.